Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / IntMinMaxAggregationOperator.cs / 1305376 / IntMinMaxAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // IntMinMaxAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined min/max aggregation and its enumerator, for ints. /// internal sealed class IntMinMaxAggregationOperator : InlinedAggregationOperator{ private readonly int m_sign; // The sign (-1 for min, 1 for max). //---------------------------------------------------------------------------------------- // Constructs a new instance of a min/max associative operator. // internal IntMinMaxAggregationOperator(IEnumerable child, int sign) : base(child) { Contract.Assert(sign == -1 || sign == 1, "invalid sign"); m_sign = sign; } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override int InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // Throw an error for empty results. if (!enumerator.MoveNext()) { singularExceptionToThrow = new InvalidOperationException(SR.GetString(SR.NoElements)); return default(int); } int best = enumerator.Current; // Based on the sign, do either a min or max reduction. if (m_sign == -1) { while (enumerator.MoveNext()) { int current = enumerator.Current; if (current < best) { best = current; } } } else { while (enumerator.MoveNext()) { int current = enumerator.Current; if (current > best) { best = current; } } } return best; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new IntMinMaxAggregationOperatorEnumerator (source, index, m_sign, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class IntMinMaxAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The source data. private readonly int m_sign; // The sign for comparisons (-1 means min, 1 means max). //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal IntMinMaxAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, int sign, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; m_sign = sign; } //--------------------------------------------------------------------------------------- // Tallies up the min/max of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref int currentElement) { // Based on the sign, do either a min or max reduction. QueryOperatorEnumerator source = m_source; TKey keyUnused = default(TKey); if (source.MoveNext(ref currentElement, ref keyUnused)) { int i = 0; // We just scroll through the enumerator and find the min or max. if (m_sign == -1) { int elem = default(int); while (source.MoveNext(ref elem, ref keyUnused)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (elem < currentElement) { currentElement = elem; } } } else { int elem = default(int); while (source.MoveNext(ref elem, ref keyUnused)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (elem > currentElement) { currentElement = elem; } } } // The sum has been calculated. Now just return. return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // IntMinMaxAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined min/max aggregation and its enumerator, for ints. /// internal sealed class IntMinMaxAggregationOperator : InlinedAggregationOperator{ private readonly int m_sign; // The sign (-1 for min, 1 for max). //---------------------------------------------------------------------------------------- // Constructs a new instance of a min/max associative operator. // internal IntMinMaxAggregationOperator(IEnumerable child, int sign) : base(child) { Contract.Assert(sign == -1 || sign == 1, "invalid sign"); m_sign = sign; } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override int InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // Throw an error for empty results. if (!enumerator.MoveNext()) { singularExceptionToThrow = new InvalidOperationException(SR.GetString(SR.NoElements)); return default(int); } int best = enumerator.Current; // Based on the sign, do either a min or max reduction. if (m_sign == -1) { while (enumerator.MoveNext()) { int current = enumerator.Current; if (current < best) { best = current; } } } else { while (enumerator.MoveNext()) { int current = enumerator.Current; if (current > best) { best = current; } } } return best; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new IntMinMaxAggregationOperatorEnumerator (source, index, m_sign, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class IntMinMaxAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The source data. private readonly int m_sign; // The sign for comparisons (-1 means min, 1 means max). //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal IntMinMaxAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, int sign, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; m_sign = sign; } //--------------------------------------------------------------------------------------- // Tallies up the min/max of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref int currentElement) { // Based on the sign, do either a min or max reduction. QueryOperatorEnumerator source = m_source; TKey keyUnused = default(TKey); if (source.MoveNext(ref currentElement, ref keyUnused)) { int i = 0; // We just scroll through the enumerator and find the min or max. if (m_sign == -1) { int elem = default(int); while (source.MoveNext(ref elem, ref keyUnused)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (elem < currentElement) { currentElement = elem; } } } else { int elem = default(int); while (source.MoveNext(ref elem, ref keyUnused)) { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); if (elem > currentElement) { currentElement = elem; } } } // The sum has been calculated. Now just return. return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
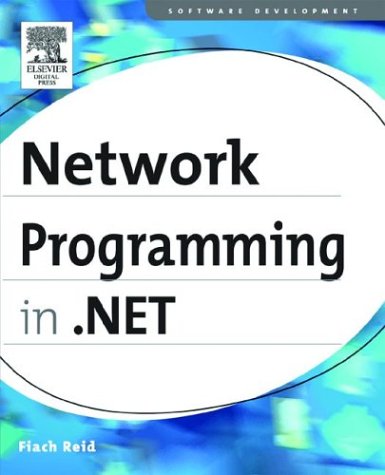
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BinHexDecoder.cs
- regiisutil.cs
- BuildProviderUtils.cs
- Listbox.cs
- ConfigXmlWhitespace.cs
- MarkupCompilePass2.cs
- SqlCachedBuffer.cs
- ServiceRoute.cs
- ManagedIStream.cs
- MobileControlBuilder.cs
- DataGridViewComboBoxCell.cs
- ScrollBar.cs
- SystemIcons.cs
- ObjectConverter.cs
- XPathBuilder.cs
- SqlTriggerAttribute.cs
- RegexRunnerFactory.cs
- XmlElementList.cs
- SqlConnectionFactory.cs
- SqlGenericUtil.cs
- IdentityModelDictionary.cs
- RelationshipEnd.cs
- BinaryObjectWriter.cs
- StorageMappingFragment.cs
- UnsafeNativeMethods.cs
- XmlStreamStore.cs
- EntityDataSourceQueryBuilder.cs
- WebBrowserUriTypeConverter.cs
- SQLBytesStorage.cs
- _ContextAwareResult.cs
- Int32CAMarshaler.cs
- DiscoveryViaBehavior.cs
- SystemTcpStatistics.cs
- ReadOnlyDataSourceView.cs
- ProfileProvider.cs
- HttpCachePolicyBase.cs
- PrintDialog.cs
- RemotingAttributes.cs
- SQLGuidStorage.cs
- SplashScreen.cs
- IdentityReference.cs
- dtdvalidator.cs
- ModelItem.cs
- TreeNode.cs
- RtfFormatStack.cs
- CookieParameter.cs
- connectionpool.cs
- PreviewPageInfo.cs
- XsdDateTime.cs
- DataConnectionHelper.cs
- CodeSnippetStatement.cs
- FixedSOMElement.cs
- InputManager.cs
- ProgressChangedEventArgs.cs
- AdCreatedEventArgs.cs
- MenuStrip.cs
- DataGridViewCellPaintingEventArgs.cs
- StyleCollectionEditor.cs
- XLinq.cs
- CssStyleCollection.cs
- Grid.cs
- NodeLabelEditEvent.cs
- PageRequestManager.cs
- Inline.cs
- Underline.cs
- WindowsListBox.cs
- RedBlackList.cs
- ListChangedEventArgs.cs
- JsonFaultDetail.cs
- SQLUtility.cs
- MemberRelationshipService.cs
- SupportingTokenBindingElement.cs
- UnitySerializationHolder.cs
- StaticTextPointer.cs
- HttpWebRequestElement.cs
- RangeValidator.cs
- TranslateTransform.cs
- Assert.cs
- SettingsPropertyValueCollection.cs
- SmtpClient.cs
- DataTableNewRowEvent.cs
- TreeNodeBindingCollection.cs
- GZipUtils.cs
- DeflateStream.cs
- SerialReceived.cs
- MaskInputRejectedEventArgs.cs
- XmlMtomWriter.cs
- StorageInfo.cs
- InvalidPrinterException.cs
- CompositeScriptReferenceEventArgs.cs
- XmlEventCache.cs
- ScriptManagerProxy.cs
- UrlParameterReader.cs
- DbConnectionInternal.cs
- DataGridViewComboBoxCell.cs
- Enumerable.cs
- UrlMappingCollection.cs
- CLSCompliantAttribute.cs
- GeometryModel3D.cs
- HtmlGenericControl.cs