Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Serializers / BatchWriter.cs / 1305376 / BatchWriter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a base class for DataWeb services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { #region Namespaces. using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; #endregion Namespaces. ////// Static helper class to write responses for batch requests /// internal static class BatchWriter { ////// Writes the start of the changeset response /// /// writer to which the response needs to be written /// batch boundary /// changeset boundary internal static void WriteStartBatchBoundary(StreamWriter writer, string batchBoundary, string changesetBoundary) { WriterStartBoundary(writer, batchBoundary); writer.WriteLine( "{0}: {1}; {2}={3}", XmlConstants.HttpContentType, XmlConstants.MimeMultiPartMixed, XmlConstants.HttpMultipartBoundary, changesetBoundary); writer.WriteLine(); // NewLine to seperate the header from message } ///Write the boundary and header information. /// writer to which the response needs to be written /// host containing the value of the response headers /// content-id string that needs to be written /// boundary string that needs to be written internal static void WriteBoundaryAndHeaders(StreamWriter writer, IDataServiceHost2 host, string contentId, string boundary) { Debug.Assert(writer != null, "writer != null"); Debug.Assert(host != null, "host != null"); Debug.Assert(boundary != null, "boundary != null"); WriterStartBoundary(writer, boundary); // First write the headers to indicate that the payload below is a http request WriteHeaderValue(writer, XmlConstants.HttpContentType, XmlConstants.MimeApplicationHttp); WriteHeaderValue(writer, XmlConstants.HttpContentTransferEncoding, XmlConstants.BatchRequestContentTransferEncoding); writer.WriteLine(); // NewLine to seperate the batch headers from http headers // In error cases, we create a dummy host, which has no request header information. // Hence we need to handle the case here. writer.WriteLine("{0} {1} {2}", XmlConstants.HttpVersionInBatching, host.ResponseStatusCode, WebUtil.GetStatusCodeText(host.ResponseStatusCode)); if (null != contentId) { WriteHeaderValue(writer, XmlConstants.HttpContentID, contentId); } System.Net.WebHeaderCollection responseHeaders = host.ResponseHeaders; foreach (string header in responseHeaders.AllKeys) { WriteHeaderValue(writer, header, responseHeaders[header]); } writer.WriteLine(); // NewLine to seperate the header from message } ////// Write the end boundary /// /// writer to which the response needs to be written /// end boundary string. internal static void WriteEndBoundary(StreamWriter writer, string boundary) { writer.WriteLine("--{0}--", boundary); } ////// Write the start boundary /// /// writer to which the response needs to be written /// boundary string. private static void WriterStartBoundary(StreamWriter writer, string boundary) { writer.WriteLine("--{0}", boundary); } ////// Write the header name and value /// /// writer to which the response needs to be written /// name of the header whose value needs to be written. /// value of the header that needs to be written. private static void WriteHeaderValue(StreamWriter writer, string headerName, object headerValue) { if (headerValue != null) { string text = Convert.ToString(headerValue, System.Globalization.CultureInfo.InvariantCulture); if (!String.IsNullOrEmpty(text)) { writer.WriteLine("{0}: {1}", headerName, text); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a base class for DataWeb services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { #region Namespaces. using System; using System.Collections.Generic; using System.Diagnostics; using System.IO; #endregion Namespaces. ////// Static helper class to write responses for batch requests /// internal static class BatchWriter { ////// Writes the start of the changeset response /// /// writer to which the response needs to be written /// batch boundary /// changeset boundary internal static void WriteStartBatchBoundary(StreamWriter writer, string batchBoundary, string changesetBoundary) { WriterStartBoundary(writer, batchBoundary); writer.WriteLine( "{0}: {1}; {2}={3}", XmlConstants.HttpContentType, XmlConstants.MimeMultiPartMixed, XmlConstants.HttpMultipartBoundary, changesetBoundary); writer.WriteLine(); // NewLine to seperate the header from message } ///Write the boundary and header information. /// writer to which the response needs to be written /// host containing the value of the response headers /// content-id string that needs to be written /// boundary string that needs to be written internal static void WriteBoundaryAndHeaders(StreamWriter writer, IDataServiceHost2 host, string contentId, string boundary) { Debug.Assert(writer != null, "writer != null"); Debug.Assert(host != null, "host != null"); Debug.Assert(boundary != null, "boundary != null"); WriterStartBoundary(writer, boundary); // First write the headers to indicate that the payload below is a http request WriteHeaderValue(writer, XmlConstants.HttpContentType, XmlConstants.MimeApplicationHttp); WriteHeaderValue(writer, XmlConstants.HttpContentTransferEncoding, XmlConstants.BatchRequestContentTransferEncoding); writer.WriteLine(); // NewLine to seperate the batch headers from http headers // In error cases, we create a dummy host, which has no request header information. // Hence we need to handle the case here. writer.WriteLine("{0} {1} {2}", XmlConstants.HttpVersionInBatching, host.ResponseStatusCode, WebUtil.GetStatusCodeText(host.ResponseStatusCode)); if (null != contentId) { WriteHeaderValue(writer, XmlConstants.HttpContentID, contentId); } System.Net.WebHeaderCollection responseHeaders = host.ResponseHeaders; foreach (string header in responseHeaders.AllKeys) { WriteHeaderValue(writer, header, responseHeaders[header]); } writer.WriteLine(); // NewLine to seperate the header from message } ////// Write the end boundary /// /// writer to which the response needs to be written /// end boundary string. internal static void WriteEndBoundary(StreamWriter writer, string boundary) { writer.WriteLine("--{0}--", boundary); } ////// Write the start boundary /// /// writer to which the response needs to be written /// boundary string. private static void WriterStartBoundary(StreamWriter writer, string boundary) { writer.WriteLine("--{0}", boundary); } ////// Write the header name and value /// /// writer to which the response needs to be written /// name of the header whose value needs to be written. /// value of the header that needs to be written. private static void WriteHeaderValue(StreamWriter writer, string headerName, object headerValue) { if (headerValue != null) { string text = Convert.ToString(headerValue, System.Globalization.CultureInfo.InvariantCulture); if (!String.IsNullOrEmpty(text)) { writer.WriteLine("{0}: {1}", headerName, text); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
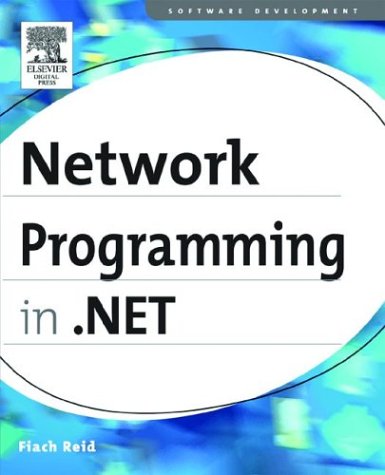
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IPEndPoint.cs
- WebPartConnectionsConfigureVerb.cs
- EntityModelBuildProvider.cs
- ObjectComplexPropertyMapping.cs
- SMSvcHost.cs
- WindowsStatusBar.cs
- TrackingDataItem.cs
- UrlEncodedParameterWriter.cs
- XslCompiledTransform.cs
- SecurityHelper.cs
- OutOfProcStateClientManager.cs
- DictationGrammar.cs
- DispatcherOperation.cs
- EntityProviderServices.cs
- RectangleGeometry.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- AlignmentXValidation.cs
- KeyValuePairs.cs
- SimpleRecyclingCache.cs
- SymbolPair.cs
- DriveInfo.cs
- ProxyAttribute.cs
- XmlSortKeyAccumulator.cs
- DataTableTypeConverter.cs
- XsltLoader.cs
- StorageMappingItemCollection.cs
- EntityContainer.cs
- DataError.cs
- wmiprovider.cs
- DirectionalLight.cs
- MimeBasePart.cs
- ExpressionList.cs
- LocationChangedEventArgs.cs
- KeyConverter.cs
- FSWPathEditor.cs
- FieldReference.cs
- OnOperation.cs
- SemaphoreSecurity.cs
- OdbcCommand.cs
- DesignerAttribute.cs
- DbParameterCollectionHelper.cs
- CodeAttachEventStatement.cs
- BaseHashHelper.cs
- MustUnderstandSoapException.cs
- DriveInfo.cs
- ToolStripSeparator.cs
- ToolStripMenuItem.cs
- Triplet.cs
- RowsCopiedEventArgs.cs
- FtpCachePolicyElement.cs
- WebFormDesignerActionService.cs
- GlyphElement.cs
- DocumentReferenceCollection.cs
- TextCompositionManager.cs
- UndoManager.cs
- DependencyObjectCodeDomSerializer.cs
- PackageDigitalSignatureManager.cs
- ElementAction.cs
- DataGridRowDetailsEventArgs.cs
- DispatcherSynchronizationContext.cs
- Win32PrintDialog.cs
- CFStream.cs
- ColumnWidthChangedEvent.cs
- RectAnimationBase.cs
- CalendarData.cs
- ChtmlTextWriter.cs
- SqlNodeAnnotation.cs
- VisualProxy.cs
- CellCreator.cs
- SQLMembershipProvider.cs
- PropertyMapper.cs
- DeviceContext2.cs
- EntityDataSourceQueryBuilder.cs
- XmlnsDictionary.cs
- DataGridTableCollection.cs
- SafeRightsManagementEnvironmentHandle.cs
- TextDecorationCollection.cs
- SevenBitStream.cs
- AutomationTextAttribute.cs
- _IPv6Address.cs
- GlyphRunDrawing.cs
- DataGrid.cs
- Encoding.cs
- EntityClassGenerator.cs
- HMAC.cs
- ThreadStartException.cs
- AutomationProperties.cs
- Array.cs
- XmlSignatureManifest.cs
- HitTestParameters3D.cs
- WindowsScroll.cs
- DeferredReference.cs
- XmlDataImplementation.cs
- LabelEditEvent.cs
- XNodeValidator.cs
- XmlMemberMapping.cs
- PocoEntityKeyStrategy.cs
- Comparer.cs
- SelectedPathEditor.cs
- EpmContentSerializerBase.cs