Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Misc / GDI / WindowsPen.cs / 1305376 / WindowsPen.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #if [....]_NAMESPACE namespace System.Windows.Forms.Internal #elif DRAWING_NAMESPACE namespace System.Drawing.Internal #else namespace System.Experimental.Gdi #endif { using System; using System.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Drawing2D; using System.Globalization; using System.Runtime.Versioning; ////// #if [....]_PUBLIC_GRAPHICS_LIBRARY public #else internal #endif sealed partial class WindowsPen : MarshalByRefObject, ICloneable, IDisposable { // // Handle to the native Windows pen object. // private IntPtr nativeHandle; private const int dashStyleMask = 0x0000000F; private const int endCapMask = 0x00000F00; private const int joinMask = 0x0000F000; private DeviceContext dc; // // Fields with default values // private WindowsBrush wndBrush; private WindowsPenStyle style; private Color color; private int width; private const int cosmeticPenWidth = 1; // Cosmetic pen width. #if GDI_FINALIZATION_WATCH private string AllocationSite = DbgUtil.StackTrace; #endif [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc) : this( dc, WindowsPenStyle.Default, cosmeticPenWidth, Color.Black ) { } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc, Color color ) : this( dc, WindowsPenStyle.Default, cosmeticPenWidth, color ) { } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc, WindowsBrush windowsBrush ) : this( dc, WindowsPenStyle.Default, cosmeticPenWidth, windowsBrush ) { } [ResourceExposure(ResourceScope.Process)] public WindowsPen(DeviceContext dc, WindowsPenStyle style, int width, Color color) { this.style = style; this.width = width; this.color = color; this.dc = dc; // CreatePen() created on demand. } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc, WindowsPenStyle style, int width, WindowsBrush windowsBrush ) { Debug.Assert(windowsBrush != null, "null windowsBrush" ); this.style = style; this.wndBrush = (WindowsBrush) windowsBrush.Clone(); this.width = width; this.color = windowsBrush.Color; this.dc = dc; // CreatePen() created on demand. } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] private void CreatePen() { if (this.width > 1) // Geometric pen. { // From MSDN: if width > 1, the style must be PS_NULL, PS_SOLID, or PS_INSIDEFRAME. this.style |= WindowsPenStyle.Geometric | WindowsPenStyle.Solid; } if (this.wndBrush == null) { this.nativeHandle = IntSafeNativeMethods.CreatePen((int) this.style, this.width, ColorTranslator.ToWin32(this.color) ); } else { IntNativeMethods.LOGBRUSH lb = new IntNativeMethods.LOGBRUSH(); lb.lbColor = ColorTranslator.ToWin32( this.wndBrush.Color ); lb.lbStyle = IntNativeMethods.BS_SOLID; lb.lbHatch = 0; // // Note: We currently don't support custom styles, that's why 0 and null for last two params. this.nativeHandle = IntSafeNativeMethods.ExtCreatePen((int)this.style, this.width, lb, 0, null ); } } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public object Clone() { return (this.wndBrush != null) ? new WindowsPen(this.dc, this.style, this.width, (WindowsBrush) this.wndBrush.Clone()) : new WindowsPen(this.dc, this.style, this.width, this.color); } ~WindowsPen() { Dispose(false); } public void Dispose() { Dispose(true); } void Dispose(bool disposing) { if (this.nativeHandle != IntPtr.Zero && dc != null) { DbgUtil.AssertFinalization(this, disposing); dc.DeleteObject(this.nativeHandle, GdiObjectType.Pen); this.nativeHandle = IntPtr.Zero; } if (this.wndBrush != null) { this.wndBrush.Dispose(); this.wndBrush = null; } if (disposing) { GC.SuppressFinalize(this); } } public IntPtr HPen { get { if( this.nativeHandle == IntPtr.Zero ) { CreatePen(); } return this.nativeHandle; } } public override string ToString() { return String.Format( CultureInfo.InvariantCulture, "{0}: Style={1}, Color={2}, Width={3}, Brush={4}", this.GetType().Name, this.style, this.color, this.width, this.wndBrush != null ? this.wndBrush.ToString() : "null" ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Encapsulates a GDI Pen object. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #if [....]_NAMESPACE namespace System.Windows.Forms.Internal #elif DRAWING_NAMESPACE namespace System.Drawing.Internal #else namespace System.Experimental.Gdi #endif { using System; using System.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Drawing; using System.Drawing.Drawing2D; using System.Globalization; using System.Runtime.Versioning; ////// #if [....]_PUBLIC_GRAPHICS_LIBRARY public #else internal #endif sealed partial class WindowsPen : MarshalByRefObject, ICloneable, IDisposable { // // Handle to the native Windows pen object. // private IntPtr nativeHandle; private const int dashStyleMask = 0x0000000F; private const int endCapMask = 0x00000F00; private const int joinMask = 0x0000F000; private DeviceContext dc; // // Fields with default values // private WindowsBrush wndBrush; private WindowsPenStyle style; private Color color; private int width; private const int cosmeticPenWidth = 1; // Cosmetic pen width. #if GDI_FINALIZATION_WATCH private string AllocationSite = DbgUtil.StackTrace; #endif [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc) : this( dc, WindowsPenStyle.Default, cosmeticPenWidth, Color.Black ) { } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc, Color color ) : this( dc, WindowsPenStyle.Default, cosmeticPenWidth, color ) { } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc, WindowsBrush windowsBrush ) : this( dc, WindowsPenStyle.Default, cosmeticPenWidth, windowsBrush ) { } [ResourceExposure(ResourceScope.Process)] public WindowsPen(DeviceContext dc, WindowsPenStyle style, int width, Color color) { this.style = style; this.width = width; this.color = color; this.dc = dc; // CreatePen() created on demand. } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public WindowsPen(DeviceContext dc, WindowsPenStyle style, int width, WindowsBrush windowsBrush ) { Debug.Assert(windowsBrush != null, "null windowsBrush" ); this.style = style; this.wndBrush = (WindowsBrush) windowsBrush.Clone(); this.width = width; this.color = windowsBrush.Color; this.dc = dc; // CreatePen() created on demand. } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] private void CreatePen() { if (this.width > 1) // Geometric pen. { // From MSDN: if width > 1, the style must be PS_NULL, PS_SOLID, or PS_INSIDEFRAME. this.style |= WindowsPenStyle.Geometric | WindowsPenStyle.Solid; } if (this.wndBrush == null) { this.nativeHandle = IntSafeNativeMethods.CreatePen((int) this.style, this.width, ColorTranslator.ToWin32(this.color) ); } else { IntNativeMethods.LOGBRUSH lb = new IntNativeMethods.LOGBRUSH(); lb.lbColor = ColorTranslator.ToWin32( this.wndBrush.Color ); lb.lbStyle = IntNativeMethods.BS_SOLID; lb.lbHatch = 0; // // Note: We currently don't support custom styles, that's why 0 and null for last two params. this.nativeHandle = IntSafeNativeMethods.ExtCreatePen((int)this.style, this.width, lb, 0, null ); } } [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public object Clone() { return (this.wndBrush != null) ? new WindowsPen(this.dc, this.style, this.width, (WindowsBrush) this.wndBrush.Clone()) : new WindowsPen(this.dc, this.style, this.width, this.color); } ~WindowsPen() { Dispose(false); } public void Dispose() { Dispose(true); } void Dispose(bool disposing) { if (this.nativeHandle != IntPtr.Zero && dc != null) { DbgUtil.AssertFinalization(this, disposing); dc.DeleteObject(this.nativeHandle, GdiObjectType.Pen); this.nativeHandle = IntPtr.Zero; } if (this.wndBrush != null) { this.wndBrush.Dispose(); this.wndBrush = null; } if (disposing) { GC.SuppressFinalize(this); } } public IntPtr HPen { get { if( this.nativeHandle == IntPtr.Zero ) { CreatePen(); } return this.nativeHandle; } } public override string ToString() { return String.Format( CultureInfo.InvariantCulture, "{0}: Style={1}, Color={2}, Width={3}, Brush={4}", this.GetType().Name, this.style, this.color, this.width, this.wndBrush != null ? this.wndBrush.ToString() : "null" ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Encapsulates a GDI Pen object. /// ///
Link Menu
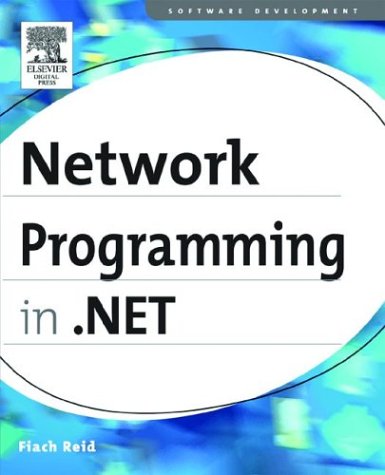
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Util.cs
- HttpValueCollection.cs
- MouseButton.cs
- TdsParserSessionPool.cs
- VBIdentifierDesigner.xaml.cs
- CompositeFontFamily.cs
- ServiceEndpointCollection.cs
- GeneralTransform3DGroup.cs
- AuthenticationException.cs
- OleDbSchemaGuid.cs
- TextSelectionHighlightLayer.cs
- HelpProvider.cs
- X509ChainPolicy.cs
- DurableTimerExtension.cs
- oledbmetadatacollectionnames.cs
- LinqDataSourceValidationException.cs
- DependencyPropertyKind.cs
- WebControl.cs
- UrlPropertyAttribute.cs
- ClientCultureInfo.cs
- TaiwanCalendar.cs
- SHA512Managed.cs
- WebPartCollection.cs
- WebCategoryAttribute.cs
- ArcSegment.cs
- CapabilitiesUse.cs
- WasHostedComPlusFactory.cs
- Stackframe.cs
- WebPartDisplayMode.cs
- WrappedIUnknown.cs
- SinglePageViewer.cs
- LOSFormatter.cs
- HttpDebugHandler.cs
- ItemType.cs
- ApplicationInfo.cs
- CssTextWriter.cs
- WindowsListViewItemStartMenu.cs
- Switch.cs
- FormatterServices.cs
- XmlDataProvider.cs
- EntityClassGenerator.cs
- PerformanceCounterPermissionAttribute.cs
- MarkupProperty.cs
- WindowInteractionStateTracker.cs
- CapiNative.cs
- QilTypeChecker.cs
- SectionInformation.cs
- DoWhile.cs
- DesignerDataView.cs
- entitydatasourceentitysetnameconverter.cs
- PolygonHotSpot.cs
- WsdlInspector.cs
- XmlFormatExtensionPointAttribute.cs
- Font.cs
- MatrixTransform3D.cs
- Attributes.cs
- Control.cs
- BroadcastEventHelper.cs
- HierarchicalDataSourceIDConverter.cs
- OleDbStruct.cs
- ExpressionEvaluator.cs
- EntityContainer.cs
- CatalogPartChrome.cs
- FontInfo.cs
- Stroke.cs
- _AcceptOverlappedAsyncResult.cs
- LocatorPart.cs
- VirtualDirectoryMapping.cs
- HashAlgorithm.cs
- OperandQuery.cs
- OracleParameterBinding.cs
- DBPropSet.cs
- StorageConditionPropertyMapping.cs
- AppModelKnownContentFactory.cs
- AudioDeviceOut.cs
- precedingquery.cs
- CookieParameter.cs
- PrivilegeNotHeldException.cs
- LabelExpression.cs
- ListItem.cs
- DetailsViewCommandEventArgs.cs
- XmlArrayItemAttributes.cs
- XPathCompileException.cs
- AssemblyBuilder.cs
- WorkflowInstanceTerminatedRecord.cs
- Span.cs
- ExpressionHelper.cs
- ByteArrayHelperWithString.cs
- MemberRestriction.cs
- StreamResourceInfo.cs
- EventListenerClientSide.cs
- Axis.cs
- ObjectListDataBindEventArgs.cs
- IndependentAnimationStorage.cs
- KerberosReceiverSecurityToken.cs
- BufferedGraphics.cs
- WebPartManager.cs
- XmlDeclaration.cs
- AnnotationResourceCollection.cs
- TextEditorSelection.cs