Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Image.cs / 1305600 / Image.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: Image.cs // // Description: Contains the Image class. // Layout Spec at http://avalon/layout/Specs/Image.xml // // History: // 06/02/2003 : greglett - Added to WCP branch, layout functionality (was Image.cs in old branch) // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationFramework; using MS.Utility; using System.Diagnostics; using System.ComponentModel; #if OLD_AUTOMATION using System.Windows.Automation.Provider; #endif using System.Windows.Threading; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Documents; using System.Windows.Markup; using System.Windows.Navigation; using System; namespace System.Windows.Controls { ////// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] #if OLD_AUTOMATION [Automation(AccessibilityControlType = "Image")] #endif public class Image : FrameworkElement, IUriContext, IProvidePropertyFallback { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public Image() : base() { } #endregion //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- //-------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ////// Gets/Sets the Source on this Image. /// /// The Source property is the ImageSource that holds the actual image drawn. /// public ImageSource Source { get { return (ImageSource) GetValue(SourceProperty); } set { SetValue(SourceProperty, value); } } ////// Gets/Sets the Stretch on this Image. /// The Stretch property determines how large the Image will be drawn. /// ///public Stretch Stretch { get { return (Stretch) GetValue(StretchProperty); } set { SetValue(StretchProperty, value); } } /// /// Gets/Sets the stretch direction of the Viewbox, which determines the restrictions on /// scaling that are applied to the content inside the Viewbox. For instance, this property /// can be used to prevent the content from being smaller than its native size or larger than /// its native size. /// ///public StretchDirection StretchDirection { get { return (StretchDirection)GetValue(StretchDirectionProperty); } set { SetValue(StretchDirectionProperty, value); } } /// /// DependencyProperty for Image Source property. /// ///[CommonDependencyProperty] public static readonly DependencyProperty SourceProperty = DependencyProperty.Register( "Source", typeof(ImageSource), typeof(Image), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender, new PropertyChangedCallback(OnSourceChanged), null), null); /// /// DependencyProperty for Stretch property. /// ///[CommonDependencyProperty] public static readonly DependencyProperty StretchProperty = Viewbox.StretchProperty.AddOwner(typeof(Image)); /// /// DependencyProperty for StretchDirection property. /// ///public static readonly DependencyProperty StretchDirectionProperty = Viewbox.StretchDirectionProperty.AddOwner(typeof(Image)); /// /// ImageFailedEvent is a routed event. /// public static readonly RoutedEvent ImageFailedEvent = EventManager.RegisterRoutedEvent( "ImageFailed", RoutingStrategy.Bubble, typeof(EventHandler), typeof(Image)); /// /// Raised when there is a failure in image. /// public event EventHandlerImageFailed { add { AddHandler(ImageFailedEvent, value); } remove { RemoveHandler(ImageFailedEvent, value); } } #endregion //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods /// /// Creates AutomationPeer ( protected override System.Windows.Automation.Peers.AutomationPeer OnCreateAutomationPeer() { return new System.Windows.Automation.Peers.ImageAutomationPeer(this); } ///) /// /// Updates DesiredSize of the Image. Called by parent UIElement. This is the first pass of layout. /// ////// Image will always return its natural size, if it fits within the constraint. If not, it will return /// as large a size as it can. Remember that image can later arrange at any size and stretch/align. /// /// Constraint size is an "upper limit" that Image should not exceed. ///Image's desired size. protected override Size MeasureOverride(Size constraint) { return MeasureArrangeHelper(constraint); } ////// Override for protected override Size ArrangeOverride(Size arrangeSize) { return MeasureArrangeHelper(arrangeSize); } // // protected override void OnArrange(Size arrangeSize) // Because Image does not have children and it is inexpensive to compute it's alignment/size, // it does not need an OnArrange override. It will simply use its own RenderSize (set when its // Arrange is called) in OnRender. // ///. /// /// The Stretch property determines how large the Image will be drawn. The values for Stretch are: /// protected override void OnRender(DrawingContext dc) { ImageSource imageSource = Source; if (imageSource == null) { return; } //computed from the ArrangeOverride return size dc.DrawImage(imageSource, new Rect(new Point(), RenderSize)); } #endregion Protected Methods #region IUriContext implementation ////// AlignmentX and AlignmentY properties are used to position the Image when its size /// is different the RenderSize. ///
- None
- Image draws at natural size and will clip / overdraw if too large.
///- Fill
- Image will draw at RenderSize. Aspect Ratio may be distorted.
///- Uniform
- Image will scale uniformly up or down to fit within RenderSize.
///- UniformFill
- Image will scale uniformly to fill RenderSize, clipping / overdrawing in the larger dimension.
/// Accessor for the base uri of the Image /// Uri IUriContext.BaseUri { get { return BaseUri; } set { BaseUri = value; } } ////// Implementation for BaseUri /// protected virtual Uri BaseUri { get { return (Uri)GetValue(BaseUriHelper.BaseUriProperty); } set { SetValue(BaseUriHelper.BaseUriProperty, value); } } #endregion IUriContext implementation //------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods ////// Contains the code common for MeasureOverride and ArrangeOverride. /// /// input size is the parent-provided space that Image should use to "fit in", according to other properties. ///Image's desired size. private Size MeasureArrangeHelper(Size inputSize) { ImageSource imageSource = Source; Size naturalSize = new Size(); if (imageSource == null) { return naturalSize; } try { UpdateBaseUri(this, imageSource); naturalSize = imageSource.Size; } catch(Exception e) { SetCurrentValue(SourceProperty, null); RaiseEvent(new ExceptionRoutedEventArgs(ImageFailedEvent, this, e)); } //get computed scale factor Size scaleFactor = Viewbox.ComputeScaleFactor(inputSize, naturalSize, this.Stretch, this.StretchDirection); // Returns our minimum size & sets DesiredSize. return new Size(naturalSize.Width * scaleFactor.Width, naturalSize.Height * scaleFactor.Height); } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 19; } } #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields private WeakBitmapSourceEvents _weakBitmapSourceEvents; #endregion Private Fields //------------------------------------------------------------------- // // Static Constructors & Delegates // //-------------------------------------------------------------------- #region Static Constructors & Delegates static Image() { Style style = CreateDefaultStyles(); StyleProperty.OverrideMetadata(typeof(Image), new FrameworkPropertyMetadata(style)); // // The Stretch & StretchDirection properties are AddOwner'ed from a class which is not // base class for Image so the metadata with flags get lost. We need to override them // here to make it work again. // StretchProperty.OverrideMetadata( typeof(Image), new FrameworkPropertyMetadata( Stretch.Uniform, FrameworkPropertyMetadataOptions.AffectsMeasure ) ); StretchDirectionProperty.OverrideMetadata( typeof(Image), new FrameworkPropertyMetadata( StretchDirection.Both, FrameworkPropertyMetadataOptions.AffectsMeasure ) ); } private static Style CreateDefaultStyles() { Style style = new Style(typeof(Image), null); style.Setters.Add (new Setter(FlowDirectionProperty, FlowDirection.LeftToRight)); style.Seal(); return style; } private void OnSourceDownloaded(object sender, EventArgs e) { InvalidateMeasure(); InvalidateVisual(); //ensure re-rendering } private void OnSourceFailed(object sender, ExceptionEventArgs e) { SetCurrentValue(SourceProperty, null); RaiseEvent(new ExceptionRoutedEventArgs(ImageFailedEvent, this, e.ErrorException)); } private static void OnSourceChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { Image image = (Image)d; ImageSource oldValue = (ImageSource)e.OldValue; ImageSource newValue = (ImageSource)e.NewValue; UpdateBaseUri(d, newValue); // Detach existing bitmap source events. if (image._weakBitmapSourceEvents != null) { image._weakBitmapSourceEvents.Dispose(); image._weakBitmapSourceEvents = null; } BitmapSource newBitmapSource = newValue as BitmapSource; if (newBitmapSource != null && !newBitmapSource.IsFrozen) { image._weakBitmapSourceEvents = new WeakBitmapSourceEvents(image, newBitmapSource); } } } private static void UpdateBaseUri(DependencyObject d, ImageSource source) { if ((source is IUriContext) && (!source.IsFrozen) && (((IUriContext)source).BaseUri == null)) { Uri baseUri = BaseUriHelper.GetBaseUriCore(d); if (baseUri != null) { ((IUriContext)source).BaseUri = BaseUriHelper.GetBaseUriCore(d); } } } #endregion #region IProvidePropertyFallback ////// Says if the type can provide fallback value for the given property /// bool IProvidePropertyFallback.CanProvidePropertyFallback(string property) { if (String.CompareOrdinal(property, "Source") == 0) { return true; } return false; } ////// Returns the fallback value for the given property. /// object IProvidePropertyFallback.ProvidePropertyFallback(string property, Exception cause) { if (String.CompareOrdinal(property, "Source") == 0) { RaiseEvent(new ExceptionRoutedEventArgs(ImageFailedEvent, this, cause)); } // For now we do not have a static that represents a bad-image, so just return a null. return null; } #endregion IProvidePropertyFallback #region WeakBitmapSourceEvents ////// WeakBitmapSourceEvents acts as a proxy between events on BitmapSource /// and handlers on Image. It is used to respond to events on BitmapSource /// without preventing Image's to be collected. /// private class WeakBitmapSourceEvents : WeakReference { public WeakBitmapSourceEvents(Image image, BitmapSource bitmapSource) : base(image) { _bitmapSource = bitmapSource; _bitmapSource.DownloadCompleted += this.OnSourceDownloaded; _bitmapSource.DownloadFailed += this.OnSourceFailed; _bitmapSource.DecodeFailed += this.OnSourceFailed; } public void OnSourceDownloaded(object sender, EventArgs e) { Image image = this.Target as Image; if (null != image) { image.OnSourceDownloaded(image, e); } else { Dispose(); } } public void OnSourceFailed(object sender, ExceptionEventArgs e) { Image image = this.Target as Image; if (null != image) { image.OnSourceFailed(image, e); } else { Dispose(); } } public void Dispose() { // We can't remove hanlders from frozen sources. if (!_bitmapSource.IsFrozen) { _bitmapSource.DownloadCompleted -= this.OnSourceDownloaded; _bitmapSource.DownloadFailed -= this.OnSourceFailed; _bitmapSource.DecodeFailed -= this.OnSourceFailed; } } private BitmapSource _bitmapSource; } #endregion WeakBitmapSourceEvents } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: Image.cs // // Description: Contains the Image class. // Layout Spec at http://avalon/layout/Specs/Image.xml // // History: // 06/02/2003 : greglett - Added to WCP branch, layout functionality (was Image.cs in old branch) // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationFramework; using MS.Utility; using System.Diagnostics; using System.ComponentModel; #if OLD_AUTOMATION using System.Windows.Automation.Provider; #endif using System.Windows.Threading; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Documents; using System.Windows.Markup; using System.Windows.Navigation; using System; namespace System.Windows.Controls { ////// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] #if OLD_AUTOMATION [Automation(AccessibilityControlType = "Image")] #endif public class Image : FrameworkElement, IUriContext, IProvidePropertyFallback { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public Image() : base() { } #endregion //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- //-------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ////// Gets/Sets the Source on this Image. /// /// The Source property is the ImageSource that holds the actual image drawn. /// public ImageSource Source { get { return (ImageSource) GetValue(SourceProperty); } set { SetValue(SourceProperty, value); } } ////// Gets/Sets the Stretch on this Image. /// The Stretch property determines how large the Image will be drawn. /// ///public Stretch Stretch { get { return (Stretch) GetValue(StretchProperty); } set { SetValue(StretchProperty, value); } } /// /// Gets/Sets the stretch direction of the Viewbox, which determines the restrictions on /// scaling that are applied to the content inside the Viewbox. For instance, this property /// can be used to prevent the content from being smaller than its native size or larger than /// its native size. /// ///public StretchDirection StretchDirection { get { return (StretchDirection)GetValue(StretchDirectionProperty); } set { SetValue(StretchDirectionProperty, value); } } /// /// DependencyProperty for Image Source property. /// ///[CommonDependencyProperty] public static readonly DependencyProperty SourceProperty = DependencyProperty.Register( "Source", typeof(ImageSource), typeof(Image), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender, new PropertyChangedCallback(OnSourceChanged), null), null); /// /// DependencyProperty for Stretch property. /// ///[CommonDependencyProperty] public static readonly DependencyProperty StretchProperty = Viewbox.StretchProperty.AddOwner(typeof(Image)); /// /// DependencyProperty for StretchDirection property. /// ///public static readonly DependencyProperty StretchDirectionProperty = Viewbox.StretchDirectionProperty.AddOwner(typeof(Image)); /// /// ImageFailedEvent is a routed event. /// public static readonly RoutedEvent ImageFailedEvent = EventManager.RegisterRoutedEvent( "ImageFailed", RoutingStrategy.Bubble, typeof(EventHandler), typeof(Image)); /// /// Raised when there is a failure in image. /// public event EventHandlerImageFailed { add { AddHandler(ImageFailedEvent, value); } remove { RemoveHandler(ImageFailedEvent, value); } } #endregion //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods /// /// Creates AutomationPeer ( protected override System.Windows.Automation.Peers.AutomationPeer OnCreateAutomationPeer() { return new System.Windows.Automation.Peers.ImageAutomationPeer(this); } ///) /// /// Updates DesiredSize of the Image. Called by parent UIElement. This is the first pass of layout. /// ////// Image will always return its natural size, if it fits within the constraint. If not, it will return /// as large a size as it can. Remember that image can later arrange at any size and stretch/align. /// /// Constraint size is an "upper limit" that Image should not exceed. ///Image's desired size. protected override Size MeasureOverride(Size constraint) { return MeasureArrangeHelper(constraint); } ////// Override for protected override Size ArrangeOverride(Size arrangeSize) { return MeasureArrangeHelper(arrangeSize); } // // protected override void OnArrange(Size arrangeSize) // Because Image does not have children and it is inexpensive to compute it's alignment/size, // it does not need an OnArrange override. It will simply use its own RenderSize (set when its // Arrange is called) in OnRender. // ///. /// /// The Stretch property determines how large the Image will be drawn. The values for Stretch are: /// protected override void OnRender(DrawingContext dc) { ImageSource imageSource = Source; if (imageSource == null) { return; } //computed from the ArrangeOverride return size dc.DrawImage(imageSource, new Rect(new Point(), RenderSize)); } #endregion Protected Methods #region IUriContext implementation ////// AlignmentX and AlignmentY properties are used to position the Image when its size /// is different the RenderSize. ///
- None
- Image draws at natural size and will clip / overdraw if too large.
///- Fill
- Image will draw at RenderSize. Aspect Ratio may be distorted.
///- Uniform
- Image will scale uniformly up or down to fit within RenderSize.
///- UniformFill
- Image will scale uniformly to fill RenderSize, clipping / overdrawing in the larger dimension.
/// Accessor for the base uri of the Image /// Uri IUriContext.BaseUri { get { return BaseUri; } set { BaseUri = value; } } ////// Implementation for BaseUri /// protected virtual Uri BaseUri { get { return (Uri)GetValue(BaseUriHelper.BaseUriProperty); } set { SetValue(BaseUriHelper.BaseUriProperty, value); } } #endregion IUriContext implementation //------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods ////// Contains the code common for MeasureOverride and ArrangeOverride. /// /// input size is the parent-provided space that Image should use to "fit in", according to other properties. ///Image's desired size. private Size MeasureArrangeHelper(Size inputSize) { ImageSource imageSource = Source; Size naturalSize = new Size(); if (imageSource == null) { return naturalSize; } try { UpdateBaseUri(this, imageSource); naturalSize = imageSource.Size; } catch(Exception e) { SetCurrentValue(SourceProperty, null); RaiseEvent(new ExceptionRoutedEventArgs(ImageFailedEvent, this, e)); } //get computed scale factor Size scaleFactor = Viewbox.ComputeScaleFactor(inputSize, naturalSize, this.Stretch, this.StretchDirection); // Returns our minimum size & sets DesiredSize. return new Size(naturalSize.Width * scaleFactor.Width, naturalSize.Height * scaleFactor.Height); } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 19; } } #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields private WeakBitmapSourceEvents _weakBitmapSourceEvents; #endregion Private Fields //------------------------------------------------------------------- // // Static Constructors & Delegates // //-------------------------------------------------------------------- #region Static Constructors & Delegates static Image() { Style style = CreateDefaultStyles(); StyleProperty.OverrideMetadata(typeof(Image), new FrameworkPropertyMetadata(style)); // // The Stretch & StretchDirection properties are AddOwner'ed from a class which is not // base class for Image so the metadata with flags get lost. We need to override them // here to make it work again. // StretchProperty.OverrideMetadata( typeof(Image), new FrameworkPropertyMetadata( Stretch.Uniform, FrameworkPropertyMetadataOptions.AffectsMeasure ) ); StretchDirectionProperty.OverrideMetadata( typeof(Image), new FrameworkPropertyMetadata( StretchDirection.Both, FrameworkPropertyMetadataOptions.AffectsMeasure ) ); } private static Style CreateDefaultStyles() { Style style = new Style(typeof(Image), null); style.Setters.Add (new Setter(FlowDirectionProperty, FlowDirection.LeftToRight)); style.Seal(); return style; } private void OnSourceDownloaded(object sender, EventArgs e) { InvalidateMeasure(); InvalidateVisual(); //ensure re-rendering } private void OnSourceFailed(object sender, ExceptionEventArgs e) { SetCurrentValue(SourceProperty, null); RaiseEvent(new ExceptionRoutedEventArgs(ImageFailedEvent, this, e.ErrorException)); } private static void OnSourceChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { if (!e.IsASubPropertyChange) { Image image = (Image)d; ImageSource oldValue = (ImageSource)e.OldValue; ImageSource newValue = (ImageSource)e.NewValue; UpdateBaseUri(d, newValue); // Detach existing bitmap source events. if (image._weakBitmapSourceEvents != null) { image._weakBitmapSourceEvents.Dispose(); image._weakBitmapSourceEvents = null; } BitmapSource newBitmapSource = newValue as BitmapSource; if (newBitmapSource != null && !newBitmapSource.IsFrozen) { image._weakBitmapSourceEvents = new WeakBitmapSourceEvents(image, newBitmapSource); } } } private static void UpdateBaseUri(DependencyObject d, ImageSource source) { if ((source is IUriContext) && (!source.IsFrozen) && (((IUriContext)source).BaseUri == null)) { Uri baseUri = BaseUriHelper.GetBaseUriCore(d); if (baseUri != null) { ((IUriContext)source).BaseUri = BaseUriHelper.GetBaseUriCore(d); } } } #endregion #region IProvidePropertyFallback ////// Says if the type can provide fallback value for the given property /// bool IProvidePropertyFallback.CanProvidePropertyFallback(string property) { if (String.CompareOrdinal(property, "Source") == 0) { return true; } return false; } ////// Returns the fallback value for the given property. /// object IProvidePropertyFallback.ProvidePropertyFallback(string property, Exception cause) { if (String.CompareOrdinal(property, "Source") == 0) { RaiseEvent(new ExceptionRoutedEventArgs(ImageFailedEvent, this, cause)); } // For now we do not have a static that represents a bad-image, so just return a null. return null; } #endregion IProvidePropertyFallback #region WeakBitmapSourceEvents ////// WeakBitmapSourceEvents acts as a proxy between events on BitmapSource /// and handlers on Image. It is used to respond to events on BitmapSource /// without preventing Image's to be collected. /// private class WeakBitmapSourceEvents : WeakReference { public WeakBitmapSourceEvents(Image image, BitmapSource bitmapSource) : base(image) { _bitmapSource = bitmapSource; _bitmapSource.DownloadCompleted += this.OnSourceDownloaded; _bitmapSource.DownloadFailed += this.OnSourceFailed; _bitmapSource.DecodeFailed += this.OnSourceFailed; } public void OnSourceDownloaded(object sender, EventArgs e) { Image image = this.Target as Image; if (null != image) { image.OnSourceDownloaded(image, e); } else { Dispose(); } } public void OnSourceFailed(object sender, ExceptionEventArgs e) { Image image = this.Target as Image; if (null != image) { image.OnSourceFailed(image, e); } else { Dispose(); } } public void Dispose() { // We can't remove hanlders from frozen sources. if (!_bitmapSource.IsFrozen) { _bitmapSource.DownloadCompleted -= this.OnSourceDownloaded; _bitmapSource.DownloadFailed -= this.OnSourceFailed; _bitmapSource.DecodeFailed -= this.OnSourceFailed; } } private BitmapSource _bitmapSource; } #endregion WeakBitmapSourceEvents } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
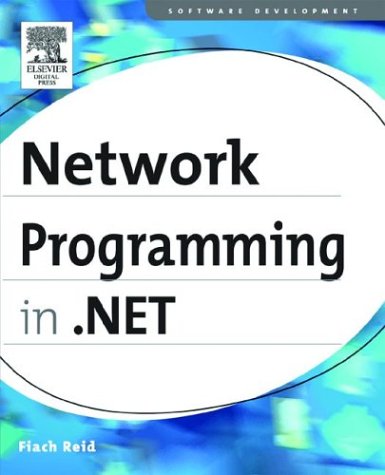
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StatusStrip.cs
- TreeViewBindingsEditorForm.cs
- TextTreeTextNode.cs
- ConfigXmlAttribute.cs
- GridViewSortEventArgs.cs
- DataGridParentRows.cs
- StyleCollection.cs
- HttpServerVarsCollection.cs
- WebConfigurationManager.cs
- MSHTMLHost.cs
- XpsFilter.cs
- HttpValueCollection.cs
- ReceiveActivityDesigner.cs
- JavaScriptString.cs
- DeobfuscatingStream.cs
- WebBrowserNavigatingEventHandler.cs
- PropertiesTab.cs
- SimpleLine.cs
- Canvas.cs
- IssuedTokenParametersElement.cs
- BaseCAMarshaler.cs
- ScalarConstant.cs
- PeerEndPoint.cs
- UdpTransportSettings.cs
- SamlAdvice.cs
- HttpCachePolicyElement.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- Console.cs
- InkCanvasAutomationPeer.cs
- MissingFieldException.cs
- XmlSchemaObject.cs
- OdbcRowUpdatingEvent.cs
- ConditionedDesigner.cs
- StateDesigner.cs
- ReflectTypeDescriptionProvider.cs
- CurrencyManager.cs
- DoubleLinkList.cs
- SecureConversationDriver.cs
- FormatPage.cs
- BroadcastEventHelper.cs
- CompareInfo.cs
- GridView.cs
- ICollection.cs
- GPPOINT.cs
- XmlSchemaAppInfo.cs
- BufferedStream2.cs
- Animatable.cs
- CodeMemberProperty.cs
- AncillaryOps.cs
- XmlImplementation.cs
- ItemCheckedEvent.cs
- InputLanguageCollection.cs
- TransformerInfo.cs
- HttpHeaderCollection.cs
- GraphicsPath.cs
- MetadataStore.cs
- TransformProviderWrapper.cs
- RepeaterItem.cs
- InkCollectionBehavior.cs
- AddInIpcChannel.cs
- ArgumentNullException.cs
- UInt64Storage.cs
- CacheDict.cs
- BamlMapTable.cs
- WebBrowserDocumentCompletedEventHandler.cs
- QueryableFilterRepeater.cs
- CodeMemberProperty.cs
- XmlEntityReference.cs
- DocumentSchemaValidator.cs
- DataViewManagerListItemTypeDescriptor.cs
- XmlTextEncoder.cs
- DataRelationPropertyDescriptor.cs
- DataObjectAttribute.cs
- OptimalBreakSession.cs
- DBSchemaTable.cs
- PropertyManager.cs
- ExclusiveCanonicalizationTransform.cs
- DetailsViewDeleteEventArgs.cs
- Console.cs
- OracleRowUpdatedEventArgs.cs
- SafeFindHandle.cs
- RTLAwareMessageBox.cs
- FileUtil.cs
- Util.cs
- _NativeSSPI.cs
- ProviderUtil.cs
- SortDescriptionCollection.cs
- WorkflowShape.cs
- XPathBinder.cs
- LayoutDump.cs
- SafeEventLogWriteHandle.cs
- FormatConvertedBitmap.cs
- SubpageParaClient.cs
- DataObjectEventArgs.cs
- HtmlElement.cs
- OperatingSystemVersionCheck.cs
- RequestCacheManager.cs
- ToolStripRenderEventArgs.cs
- CellCreator.cs
- Table.cs