Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Documents / TextTreeUndo.cs / 1305600 / TextTreeUndo.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Helper class for TextContainer, handles all undo operations. // // History: // 03/03/2004 : [....] - Created // //--------------------------------------------------------------------------- using MS.Internal.Documents; namespace System.Windows.Documents { // This static class is logically an extension of TextContainer. It contains // TextContainer undo related helpers. internal static class TextTreeUndo { //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods // Adds a TextTreeInsertUndoUnit to the open parent undo unit, if any. // Called from TextContainer.InsertText and TextContainer.InsertEmbeddedObject. internal static void CreateInsertUndoUnit(TextContainer tree, int symbolOffset, int symbolCount) { UndoManager undoManager; undoManager = GetOrClearUndoManager(tree); if (undoManager == null) return; undoManager.Add(new TextTreeInsertUndoUnit(tree, symbolOffset, symbolCount)); } // Adds a TextTreeInsertElementUndoUnit to the open parent undo unit, if any. // Called from TextContainer.InsertElement. internal static void CreateInsertElementUndoUnit(TextContainer tree, int symbolOffset, bool deep) { UndoManager undoManager; undoManager = GetOrClearUndoManager(tree); if (undoManager == null) return; undoManager.Add(new TextTreeInsertElementUndoUnit(tree, symbolOffset, deep)); } // Adds a TextTreePropertyUndoUnit to the open parent undo unit, if any. // Called by TextElement's property change listener. internal static void CreatePropertyUndoUnit(TextElement element, DependencyPropertyChangedEventArgs e) { UndoManager undoManager; PropertyRecord record; TextContainer textContainer = element.TextContainer; undoManager = GetOrClearUndoManager(textContainer); if (undoManager == null) return; record = new PropertyRecord(); record.Property = e.Property; record.Value = e.OldValueSource == BaseValueSourceInternal.Local ? e.OldValue : DependencyProperty.UnsetValue; undoManager.Add(new TextTreePropertyUndoUnit(textContainer, element.TextElementNode.GetSymbolOffset(textContainer.Generation) + 1, record)); } // Adds a DeleteContentUndoUnit to the open parent undo unit, if any. // Called by TextContainer.DeleteContent. internal static TextTreeDeleteContentUndoUnit CreateDeleteContentUndoUnit(TextContainer tree, TextPointer start, TextPointer end) { UndoManager undoManager; TextTreeDeleteContentUndoUnit undoUnit; if (start.CompareTo(end) == 0) return null; undoManager = GetOrClearUndoManager(tree); if (undoManager == null) return null; undoUnit = new TextTreeDeleteContentUndoUnit(tree, start, end); undoManager.Add(undoUnit); return undoUnit; } // Adds a TextTreeExtractElementUndoUnit to the open parent undo unit, if any. // Called by TextContainer.ExtractElement. internal static TextTreeExtractElementUndoUnit CreateExtractElementUndoUnit(TextContainer tree, TextTreeTextElementNode elementNode) { UndoManager undoManager; TextTreeExtractElementUndoUnit undoUnit; undoManager = GetOrClearUndoManager(tree); if (undoManager == null) return null; undoUnit = new TextTreeExtractElementUndoUnit(tree, elementNode); undoManager.Add(undoUnit); return undoUnit; } // Returns the local UndoManager. // Returns null if there is no undo service or if the service exists // but is disabled or if there is no open parent undo unit. internal static UndoManager GetOrClearUndoManager(ITextContainer textContainer) { UndoManager undoManager; undoManager = textContainer.UndoManager; if (undoManager == null) return null; if (!undoManager.IsEnabled) return null; if (undoManager.OpenedUnit == null) { // There's no parent undo unit, so we can't open a child. // // Clear the undo stack -- since we depend on symbol offsets // matching the original document state when an undo unit is // executed, any of our units currently in the stack will be // corrupted after we finished the operation in progress. undoManager.Clear(); return null; } return undoManager; } #endregion Internal methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Helper class for TextContainer, handles all undo operations. // // History: // 03/03/2004 : [....] - Created // //--------------------------------------------------------------------------- using MS.Internal.Documents; namespace System.Windows.Documents { // This static class is logically an extension of TextContainer. It contains // TextContainer undo related helpers. internal static class TextTreeUndo { //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods // Adds a TextTreeInsertUndoUnit to the open parent undo unit, if any. // Called from TextContainer.InsertText and TextContainer.InsertEmbeddedObject. internal static void CreateInsertUndoUnit(TextContainer tree, int symbolOffset, int symbolCount) { UndoManager undoManager; undoManager = GetOrClearUndoManager(tree); if (undoManager == null) return; undoManager.Add(new TextTreeInsertUndoUnit(tree, symbolOffset, symbolCount)); } // Adds a TextTreeInsertElementUndoUnit to the open parent undo unit, if any. // Called from TextContainer.InsertElement. internal static void CreateInsertElementUndoUnit(TextContainer tree, int symbolOffset, bool deep) { UndoManager undoManager; undoManager = GetOrClearUndoManager(tree); if (undoManager == null) return; undoManager.Add(new TextTreeInsertElementUndoUnit(tree, symbolOffset, deep)); } // Adds a TextTreePropertyUndoUnit to the open parent undo unit, if any. // Called by TextElement's property change listener. internal static void CreatePropertyUndoUnit(TextElement element, DependencyPropertyChangedEventArgs e) { UndoManager undoManager; PropertyRecord record; TextContainer textContainer = element.TextContainer; undoManager = GetOrClearUndoManager(textContainer); if (undoManager == null) return; record = new PropertyRecord(); record.Property = e.Property; record.Value = e.OldValueSource == BaseValueSourceInternal.Local ? e.OldValue : DependencyProperty.UnsetValue; undoManager.Add(new TextTreePropertyUndoUnit(textContainer, element.TextElementNode.GetSymbolOffset(textContainer.Generation) + 1, record)); } // Adds a DeleteContentUndoUnit to the open parent undo unit, if any. // Called by TextContainer.DeleteContent. internal static TextTreeDeleteContentUndoUnit CreateDeleteContentUndoUnit(TextContainer tree, TextPointer start, TextPointer end) { UndoManager undoManager; TextTreeDeleteContentUndoUnit undoUnit; if (start.CompareTo(end) == 0) return null; undoManager = GetOrClearUndoManager(tree); if (undoManager == null) return null; undoUnit = new TextTreeDeleteContentUndoUnit(tree, start, end); undoManager.Add(undoUnit); return undoUnit; } // Adds a TextTreeExtractElementUndoUnit to the open parent undo unit, if any. // Called by TextContainer.ExtractElement. internal static TextTreeExtractElementUndoUnit CreateExtractElementUndoUnit(TextContainer tree, TextTreeTextElementNode elementNode) { UndoManager undoManager; TextTreeExtractElementUndoUnit undoUnit; undoManager = GetOrClearUndoManager(tree); if (undoManager == null) return null; undoUnit = new TextTreeExtractElementUndoUnit(tree, elementNode); undoManager.Add(undoUnit); return undoUnit; } // Returns the local UndoManager. // Returns null if there is no undo service or if the service exists // but is disabled or if there is no open parent undo unit. internal static UndoManager GetOrClearUndoManager(ITextContainer textContainer) { UndoManager undoManager; undoManager = textContainer.UndoManager; if (undoManager == null) return null; if (!undoManager.IsEnabled) return null; if (undoManager.OpenedUnit == null) { // There's no parent undo unit, so we can't open a child. // // Clear the undo stack -- since we depend on symbol offsets // matching the original document state when an undo unit is // executed, any of our units currently in the stack will be // corrupted after we finished the operation in progress. undoManager.Clear(); return null; } return undoManager; } #endregion Internal methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
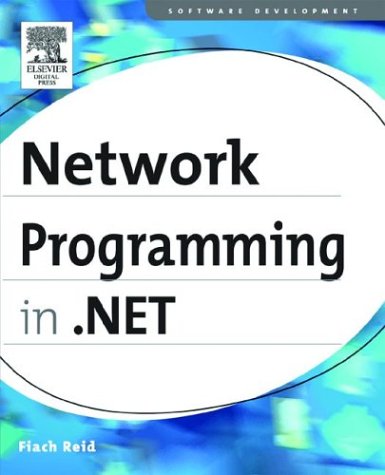
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymmetricCryptoHandle.cs
- LinqDataSourceStatusEventArgs.cs
- DataGridTable.cs
- ChildrenQuery.cs
- ScriptServiceAttribute.cs
- DefaultParameterValueAttribute.cs
- QueryCursorEventArgs.cs
- GridViewRow.cs
- ObjectFullSpanRewriter.cs
- Vector3DAnimationBase.cs
- DoubleLinkListEnumerator.cs
- TableLayoutSettingsTypeConverter.cs
- _DisconnectOverlappedAsyncResult.cs
- XamlReaderConstants.cs
- HashMembershipCondition.cs
- ChineseLunisolarCalendar.cs
- DrawingContextWalker.cs
- DataDocumentXPathNavigator.cs
- EdmConstants.cs
- SinglePageViewer.cs
- CapiSafeHandles.cs
- GridViewUpdateEventArgs.cs
- WSSecurityOneDotOneSendSecurityHeader.cs
- ProgressPage.cs
- TiffBitmapDecoder.cs
- TrackingMemoryStreamFactory.cs
- BodyGlyph.cs
- StorageScalarPropertyMapping.cs
- BuildManager.cs
- IndentTextWriter.cs
- SemanticResolver.cs
- BaseDataListPage.cs
- InternalConfigSettingsFactory.cs
- BufferAllocator.cs
- ServiceDesigner.cs
- SourceSwitch.cs
- PrintDocument.cs
- ArgumentNullException.cs
- BrowserCapabilitiesFactory.cs
- CroppedBitmap.cs
- NetworkCredential.cs
- FrugalMap.cs
- LocationSectionRecord.cs
- AllowedAudienceUriElement.cs
- TransformValueSerializer.cs
- SimpleBitVector32.cs
- RotationValidation.cs
- Message.cs
- LogManagementAsyncResult.cs
- _NegoStream.cs
- XAMLParseException.cs
- EntityWithKeyStrategy.cs
- SynchronizedInputAdaptor.cs
- TextSelectionHighlightLayer.cs
- FontWeightConverter.cs
- Base64Decoder.cs
- PathFigure.cs
- StrongNamePublicKeyBlob.cs
- ProviderConnectionPoint.cs
- PrincipalPermission.cs
- DateTimeOffset.cs
- ConfigurationLocation.cs
- NetworkInformationPermission.cs
- TrackingParameters.cs
- ChangePassword.cs
- InfoCardKeyedHashAlgorithm.cs
- ProfileSettings.cs
- MsmqIntegrationProcessProtocolHandler.cs
- StopRoutingHandler.cs
- Line.cs
- MailMessageEventArgs.cs
- ImageListDesigner.cs
- Preprocessor.cs
- OSFeature.cs
- DashStyle.cs
- ProviderException.cs
- JournalNavigationScope.cs
- CloseSequenceResponse.cs
- XmlCustomFormatter.cs
- MetadataUtilsSmi.cs
- _DomainName.cs
- Int64KeyFrameCollection.cs
- DBPropSet.cs
- WebPartConnectionCollection.cs
- ReplyChannelBinder.cs
- PlainXmlWriter.cs
- ChangePassword.cs
- AnimationClock.cs
- KeyValuePairs.cs
- IISMapPath.cs
- BinaryKeyIdentifierClause.cs
- AncillaryOps.cs
- MissingMethodException.cs
- OdbcFactory.cs
- ToolStripControlHost.cs
- EntityDataSourceDataSelection.cs
- TaskForm.cs
- ReplyChannelBinder.cs
- IisTraceListener.cs
- SpellerInterop.cs