Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Shared / MS / Utility / ItemMap.cs / 1305600 / ItemMap.cs
using System; using System.Collections; using System.Diagnostics; namespace MS.Utility { // // ItemStructMap// // Disable warnings about fields never being assigned to // This struct is designed to function with its fields starting at // their default values and without the need of surfacing a constructor // other than the deafult #pragma warning disable 649 internal struct ItemStructMap { public int EnsureEntry(int key) { int index = Search(key); if (index < 0) { // Not found, add Entry // Create initial capacity, if necessary if (Entries == null) { Entries = new Entry[SearchTypeThreshold]; } // Convert not-found index to insertion point index = ~index; Entry[] destEntries = Entries; // Increase capacity, if necessary if ((Count + 1) > Entries.Length) { destEntries = new Entry[Entries.Length * 2]; // Initialize start of new array Array.Copy(Entries, 0, destEntries, 0, index); } // Shift entries to make room for new key at provided insertion point Array.Copy(Entries, index, destEntries, index + 1, Count - index); // Ensure Source and Destination arrays are the same Entries = destEntries; // Clear new entry Entries[index] = EmptyEntry; // Set Entries[index].Key = key; Count++; } return index; } public int Search(int key) { int keyPv = Int32.MaxValue; int iPv = 0; // Use fastest search based on size if (Count > SearchTypeThreshold) { // Binary Search int iLo = 0; int iHi = Count - 1; while (iLo <= iHi) { iPv = (iHi + iLo) / 2; keyPv = Entries[iPv].Key; if (key == keyPv) { return iPv; } if (key < keyPv) { iHi = iPv - 1; } else { iLo = iPv + 1; } } } else { // Linear search for (int i = 0; i < Count; i++) { iPv = i; keyPv = Entries[iPv].Key; if (key == keyPv) { return iPv; } if (key < keyPv) { break; } } } // iPv and keyPv will match and have the last pivot check // Return a negative value whose bitwise compliment // is this index of the first Entry that is greater // than the key passed in (sorted insertion point) if (key > keyPv) { iPv++; } return ~iPv; } private const int SearchTypeThreshold = 4; public Entry[] Entries; public int Count; public struct Entry { public int Key; public T Value; } private static Entry EmptyEntry; } #pragma warning restore 649 } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Diagnostics; namespace MS.Utility { // // ItemStructMap // // Disable warnings about fields never being assigned to // This struct is designed to function with its fields starting at // their default values and without the need of surfacing a constructor // other than the deafult #pragma warning disable 649 internal struct ItemStructMap { public int EnsureEntry(int key) { int index = Search(key); if (index < 0) { // Not found, add Entry // Create initial capacity, if necessary if (Entries == null) { Entries = new Entry[SearchTypeThreshold]; } // Convert not-found index to insertion point index = ~index; Entry[] destEntries = Entries; // Increase capacity, if necessary if ((Count + 1) > Entries.Length) { destEntries = new Entry[Entries.Length * 2]; // Initialize start of new array Array.Copy(Entries, 0, destEntries, 0, index); } // Shift entries to make room for new key at provided insertion point Array.Copy(Entries, index, destEntries, index + 1, Count - index); // Ensure Source and Destination arrays are the same Entries = destEntries; // Clear new entry Entries[index] = EmptyEntry; // Set Entries[index].Key = key; Count++; } return index; } public int Search(int key) { int keyPv = Int32.MaxValue; int iPv = 0; // Use fastest search based on size if (Count > SearchTypeThreshold) { // Binary Search int iLo = 0; int iHi = Count - 1; while (iLo <= iHi) { iPv = (iHi + iLo) / 2; keyPv = Entries[iPv].Key; if (key == keyPv) { return iPv; } if (key < keyPv) { iHi = iPv - 1; } else { iLo = iPv + 1; } } } else { // Linear search for (int i = 0; i < Count; i++) { iPv = i; keyPv = Entries[iPv].Key; if (key == keyPv) { return iPv; } if (key < keyPv) { break; } } } // iPv and keyPv will match and have the last pivot check // Return a negative value whose bitwise compliment // is this index of the first Entry that is greater // than the key passed in (sorted insertion point) if (key > keyPv) { iPv++; } return ~iPv; } private const int SearchTypeThreshold = 4; public Entry[] Entries; public int Count; public struct Entry { public int Key; public T Value; } private static Entry EmptyEntry; } #pragma warning restore 649 } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
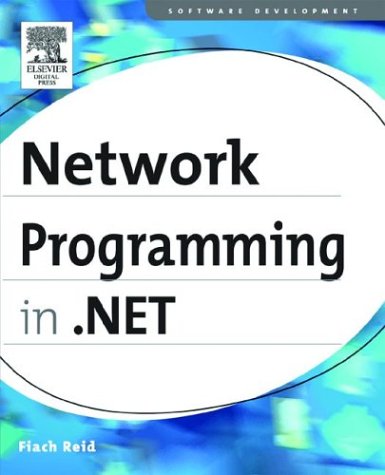
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConstraintStruct.cs
- CurrencyManager.cs
- StylusPointCollection.cs
- SafePointer.cs
- cookiecollection.cs
- login.cs
- Label.cs
- PostBackOptions.cs
- Form.cs
- X509CertificateValidator.cs
- LicenseProviderAttribute.cs
- XmlValueConverter.cs
- SourceFileInfo.cs
- PortCache.cs
- Errors.cs
- SHA512Cng.cs
- ActivityDefaults.cs
- Propagator.JoinPropagator.cs
- SoapElementAttribute.cs
- WindowsMenu.cs
- SessionIDManager.cs
- SystemIPInterfaceStatistics.cs
- ProviderSettingsCollection.cs
- CommandDesigner.cs
- C14NUtil.cs
- COAUTHIDENTITY.cs
- ModelPerspective.cs
- Label.cs
- ClockController.cs
- SHA512Managed.cs
- IDQuery.cs
- ListViewCancelEventArgs.cs
- ManagedWndProcTracker.cs
- SoundPlayer.cs
- InternalBase.cs
- LinqDataSourceEditData.cs
- SHA256Managed.cs
- RegisteredArrayDeclaration.cs
- TdsEnums.cs
- SingleTagSectionHandler.cs
- LogReservationCollection.cs
- SyntaxCheck.cs
- OleDbPropertySetGuid.cs
- ColorConverter.cs
- ConversionValidationRule.cs
- WebDescriptionAttribute.cs
- SettingsPropertyValue.cs
- OleDbException.cs
- DataFormats.cs
- ExpandedWrapper.cs
- BitArray.cs
- WorkflowShape.cs
- RadioButton.cs
- FunctionUpdateCommand.cs
- ListViewEditEventArgs.cs
- PropertyRecord.cs
- ControlUtil.cs
- ExpressionConverter.cs
- DataKeyArray.cs
- DropTarget.cs
- RemoteWebConfigurationHost.cs
- ReservationCollection.cs
- Form.cs
- Size3DConverter.cs
- ActiveXContainer.cs
- ChannelEndpointElementCollection.cs
- PolyBezierSegment.cs
- COM2PictureConverter.cs
- StringInfo.cs
- InvalidPipelineStoreException.cs
- ThreadStartException.cs
- Mappings.cs
- ToolStripScrollButton.cs
- KnownTypesHelper.cs
- PartManifestEntry.cs
- DtrList.cs
- DetailsViewModeEventArgs.cs
- TypeSemantics.cs
- MenuItem.cs
- XmlReflectionImporter.cs
- DocumentViewerBase.cs
- EndPoint.cs
- PointCollection.cs
- IconConverter.cs
- FileEnumerator.cs
- UnsafeMethods.cs
- ParserStack.cs
- CreateRefExpr.cs
- ProxyWebPartManager.cs
- MsmqBindingFilter.cs
- DbProviderServices.cs
- ErrorRuntimeConfig.cs
- GridItemProviderWrapper.cs
- ApplicationGesture.cs
- MDIWindowDialog.cs
- WebSysDescriptionAttribute.cs
- FragmentQuery.cs
- ControlAdapter.cs
- SQLDoubleStorage.cs
- TextCompositionManager.cs