Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / Binding / BindingUtils.cs / 1305376 / BindingUtils.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Utilities for binding related operations // // //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Reflection; using System.Collections; #endregion ///Utilities for binding related operations internal static class BindingUtils { ////// Throw if the entity set name is null or empty /// /// entity set name. /// entity instance for which the entity set name is generated. internal static void ValidateEntitySetName(string entitySetName, object entity) { if (String.IsNullOrEmpty(entitySetName)) { throw new InvalidOperationException(Strings.DataBinding_Util_UnknownEntitySetName(entity.GetType().FullName)); } } ////// Given a collection type, gets it's entity type /// /// Input collection type ///Generic type argument for the collection internal static Type GetCollectionEntityType(Type collectionType) { while (collectionType != null) { if (collectionType.IsGenericType && WebUtil.IsDataServiceCollectionType(collectionType.GetGenericTypeDefinition())) { return collectionType.GetGenericArguments()[0]; } collectionType = collectionType.BaseType; } return null; } ///Verifies the absence of observer for an DataServiceCollection ///Type of DataServiceCollection /// Non-typed collection object /// Collection property of the source object which is being assigned to /// Type of the source object internal static void VerifyObserverNotPresent(object oec, string sourceProperty, Type sourceType) { Debug.Assert(BindingEntityInfo.IsDataServiceCollection(oec.GetType()), "Must be an DataServiceCollection."); DataServiceCollection typedCollection = oec as DataServiceCollection ; if (typedCollection.Observer != null) { throw new InvalidOperationException(Strings.DataBinding_CollectionPropertySetterValueHasObserver(sourceProperty, sourceType)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
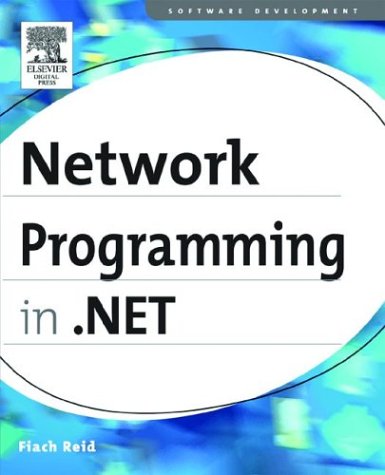
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WpfXamlType.cs
- ConstraintStruct.cs
- IPipelineRuntime.cs
- Trace.cs
- MailAddressCollection.cs
- LambdaCompiler.ControlFlow.cs
- ListenerSingletonConnectionReader.cs
- _DomainName.cs
- DeferrableContentConverter.cs
- Operators.cs
- WindowsPrincipal.cs
- RotateTransform3D.cs
- SqlInfoMessageEvent.cs
- File.cs
- MultiBinding.cs
- GridViewSortEventArgs.cs
- ContentPosition.cs
- XmlSchemaInclude.cs
- BitmapEditor.cs
- HtmlTitle.cs
- ByteStreamMessageEncoderFactory.cs
- MimeTypeAttribute.cs
- Matrix.cs
- Literal.cs
- ConnectionStringsExpressionBuilder.cs
- DateTimeParse.cs
- SqlTrackingQuery.cs
- HtmlImage.cs
- EntityContainerAssociationSet.cs
- EventHandlersStore.cs
- DmlSqlGenerator.cs
- ThreadStateException.cs
- DataTable.cs
- propertytag.cs
- LabelEditEvent.cs
- RijndaelManaged.cs
- UTF7Encoding.cs
- SettingsPropertyCollection.cs
- RangeBaseAutomationPeer.cs
- IisNotInstalledException.cs
- HtmlInputRadioButton.cs
- GroupItemAutomationPeer.cs
- HwndHostAutomationPeer.cs
- HtmlFormParameterReader.cs
- BindToObject.cs
- ConversionContext.cs
- XmlEntity.cs
- MethodBody.cs
- DataControlFieldCollection.cs
- EmptyCollection.cs
- Pen.cs
- Oci.cs
- HostedController.cs
- ClientEventManager.cs
- FloaterParaClient.cs
- WithStatement.cs
- HttpRequest.cs
- StylusEditingBehavior.cs
- TypeRestriction.cs
- SecurityUtils.cs
- AcceleratedTokenProvider.cs
- RowUpdatedEventArgs.cs
- AccessDataSource.cs
- ReceiveContextCollection.cs
- TextTreeUndoUnit.cs
- ColorMap.cs
- InvalidateEvent.cs
- DataRelationPropertyDescriptor.cs
- followingsibling.cs
- LocalFileSettingsProvider.cs
- _CommandStream.cs
- CaseInsensitiveComparer.cs
- InlinedAggregationOperatorEnumerator.cs
- ImageButton.cs
- AppDomain.cs
- Int16.cs
- WindowsAuthenticationEventArgs.cs
- FunctionCommandText.cs
- TextTreeUndo.cs
- CatalogZoneBase.cs
- TableRow.cs
- StructuralCache.cs
- TrustLevelCollection.cs
- CultureTableRecord.cs
- InternalsVisibleToAttribute.cs
- TableDetailsCollection.cs
- TextTreeTextElementNode.cs
- PagesChangedEventArgs.cs
- EventLogPropertySelector.cs
- TransportationConfigurationTypeInstallComponent.cs
- ConfigurationValidatorBase.cs
- MergablePropertyAttribute.cs
- XPathBinder.cs
- ScriptingWebServicesSectionGroup.cs
- InfoCardSchemas.cs
- SqlErrorCollection.cs
- IdentifierElement.cs
- QueryComponents.cs
- RangeValidator.cs
- tibetanshape.cs