Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Adapters / HtmlCommandAdapter.cs / 1305376 / HtmlCommandAdapter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Specialized; using System.Diagnostics; using System.IO; using System.Web; using System.Web.UI; using System.Web.UI.MobileControls; using System.Drawing; using System.Security.Permissions; #if COMPILING_FOR_SHIPPED_SOURCE namespace System.Web.UI.MobileControls.ShippedAdapterSource #else namespace System.Web.UI.MobileControls.Adapters #endif { /* * HtmlCommandAdapter class. * * Copyright (c) 2000 Microsoft Corporation */ ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class HtmlCommandAdapter : HtmlControlAdapter { /// protected new Command Control { get { return (Command)base.Control; } } /// public override void Render(HtmlMobileTextWriter writer) { bool renderLink = false; bool renderImage = false; // If image is defined and renderable, just do it. Otherwise, // render as a link if specified. if (!String.IsNullOrEmpty(Control.ImageUrl) && Device.SupportsImageSubmit) { renderImage = true; } else if (Control.Format == CommandFormat.Link && Device.JavaScript) { renderLink = true; } if (renderLink) { writer.EnterStyle(Style); Form form = Control.Form; if (form.Action.Length > 0) { writer.Write(""); writer.WriteText(Control.Text, true); writer.WriteEndTag("a"); } else { RenderBeginLink(writer, Constants.FormIDPrefix + form.UniqueID); writer.WriteText(Control.Text, true); RenderEndLink(writer); } writer.ExitStyle(Style, Control.BreakAfter); } else { writer.EnterLayout(Style); writer.WriteBeginTag("input"); writer.WriteAttribute("name", Control.UniqueID); if (renderImage) { writer.WriteAttribute("type", "image"); writer.WriteAttribute("src", Control.ResolveUrl(Control.ImageUrl), true); writer.WriteAttribute("alt", Control.Text, true); } else { writer.WriteAttribute("type", "submit"); writer.Write(" value=\""); writer.WriteText(Control.Text, true); writer.Write("\""); } AddAttributes(writer); writer.Write("/>"); writer.ExitLayout(Style, Control.BreakAfter); } } /// public override bool LoadPostData(String key, NameValueCollection data, Object controlPrivateData, out bool dataChanged) { dataChanged = false; // HTML input tags of type image postback with the coordinates // of the click rather than the name of the control. String name = Control.UniqueID; String postX = data[name + ".x"]; String postY = data[name + ".y"]; if (postX != null && postY != null && postX.Length > 0 && postY.Length > 0) { // set dataChannged to cause RaisePostDataChangedEvent() dataChanged = true; return true; } // For other command control, defer to default logic in control. return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
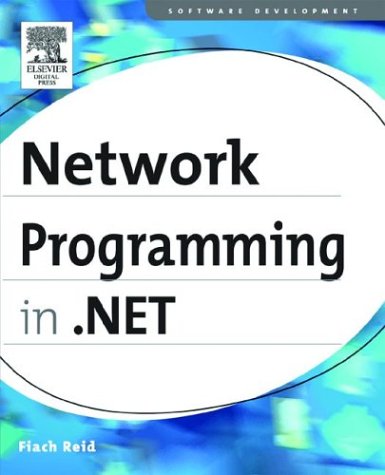
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GrammarBuilderWildcard.cs
- WebServiceBindingAttribute.cs
- JavaScriptString.cs
- ProgressBar.cs
- SizeIndependentAnimationStorage.cs
- ExtentKey.cs
- ConnectionInterfaceCollection.cs
- SamlSecurityTokenAuthenticator.cs
- MeasureItemEvent.cs
- PlainXmlWriter.cs
- UnionCodeGroup.cs
- Metafile.cs
- DropDownHolder.cs
- ObjectItemAttributeAssemblyLoader.cs
- PolyLineSegment.cs
- AppDomainManager.cs
- FormatterConverter.cs
- InputLanguageEventArgs.cs
- HwndSubclass.cs
- PassportPrincipal.cs
- GeneratedCodeAttribute.cs
- FilePrompt.cs
- OdbcConnectionString.cs
- ObjectRef.cs
- FillErrorEventArgs.cs
- NativeMethods.cs
- DataGridViewCellStyleConverter.cs
- ConfigurationStrings.cs
- MethodToken.cs
- ClientEventManager.cs
- LogLogRecordHeader.cs
- WebServiceData.cs
- BitmapEffectvisualstate.cs
- ColumnClickEvent.cs
- IPAddress.cs
- FunctionImportMapping.cs
- ListView.cs
- QueryInterceptorAttribute.cs
- ProcessingInstructionAction.cs
- ElementHostPropertyMap.cs
- ColorKeyFrameCollection.cs
- SiteMap.cs
- DictationGrammar.cs
- SeparatorAutomationPeer.cs
- InstalledVoice.cs
- DataGrid.cs
- DataTableMapping.cs
- TypeProvider.cs
- Main.cs
- HttpProfileGroupBase.cs
- ProfileModule.cs
- UIElementParagraph.cs
- AutoScrollExpandMessageFilter.cs
- WindowCollection.cs
- Line.cs
- XmlDataSourceNodeDescriptor.cs
- EncodingInfo.cs
- _emptywebproxy.cs
- LogAppendAsyncResult.cs
- X509Certificate.cs
- ReachDocumentSequenceSerializerAsync.cs
- ProfilePropertySettingsCollection.cs
- IpcManager.cs
- Verify.cs
- XmlSchemaSequence.cs
- OptimizedTemplateContentHelper.cs
- Rights.cs
- StateRuntime.cs
- Int32.cs
- DesignRelation.cs
- X509RawDataKeyIdentifierClause.cs
- ToolBarButtonDesigner.cs
- PageThemeCodeDomTreeGenerator.cs
- ReflectPropertyDescriptor.cs
- PolyBezierSegment.cs
- SqlProviderServices.cs
- CancelEventArgs.cs
- IndicShape.cs
- AlgoModule.cs
- BehaviorEditorPart.cs
- SubclassTypeValidatorAttribute.cs
- PasswordTextNavigator.cs
- XmlCharType.cs
- PreparingEnlistment.cs
- UnsafePeerToPeerMethods.cs
- XamlTreeBuilder.cs
- BitmapEffectInput.cs
- CryptoProvider.cs
- EventMappingSettings.cs
- Stacktrace.cs
- MessageSecurityProtocol.cs
- SQLDateTimeStorage.cs
- HtmlWindowCollection.cs
- OptimalTextSource.cs
- WebPartDeleteVerb.cs
- AuthenticationSection.cs
- FrameworkRichTextComposition.cs
- TreeView.cs
- CurrentTimeZone.cs
- TreeNodeBinding.cs