Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / _NetRes.cs / 1305376 / _NetRes.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Diagnostics; using System.Globalization; internal class NetRes { /*++ Constructor This is the constructor, marked private because this class shouldn't be instantiated. --*/ private NetRes() { } /*++ GetWebStatusString - Get a WebExceptionStatus-specific resource string This method takes an input string and a WebExceptionStatus. We use the input string as a key to find a status message and the webStatus to produce a status-specific message, then we combine the two. Input: Res - Id for resource string. Status - The WebExceptionStatus to be formatted. Returns: string for localized message. --*/ public static string GetWebStatusString(string Res, WebExceptionStatus Status) { string Msg; string StatusMsg; StatusMsg = SR.GetString(WebExceptionMapping.GetWebStatusString(Status)); // Get the base status. Msg = SR.GetString(Res); // Format the status specific message into the base status and return // that return String.Format(CultureInfo.CurrentCulture, Msg, StatusMsg); } public static string GetWebStatusString(WebExceptionStatus Status) { return SR.GetString(WebExceptionMapping.GetWebStatusString(Status)); } /*++ GetWebStatusCodeString - Get a StatusCode-specific resource string This method is used to map a HTTP status code to a specific user readable error code. Input: statusCode - Id for resource string. Status - The WebExceptionStatus to be formatted. Returns: string for localized message. --*/ public static string GetWebStatusCodeString(HttpStatusCode statusCode, string statusDescription) { string webStatusCode = "(" + ((int)statusCode).ToString(NumberFormatInfo.InvariantInfo) + ")"; string statusMessage = null; // // Now combine the label with the base enum key and look up the status msg. // try { // // convert the HttpStatusCode to its label and look it up. // statusMessage = SR.GetString("net_httpstatuscode_" + statusCode.ToString(), null); } catch { } if (statusMessage!=null && statusMessage.Length>0) { webStatusCode += " " + statusMessage; } else { // // Otherwise try to map the base status. // if (statusDescription!=null && statusDescription.Length>0) { webStatusCode += " " + statusDescription; } } return webStatusCode; } public static string GetWebStatusCodeString(FtpStatusCode statusCode, string statusDescription) { string webStatusCode = "(" + ((int)statusCode).ToString(NumberFormatInfo.InvariantInfo) + ")"; string statusMessage = null; // // Now combine the label with the base enum key and look up the status msg. // try { // // convert the HttpStatusCode to its label and look it up. // statusMessage = SR.GetString("net_ftpstatuscode_" + statusCode.ToString(), null); } catch { } if (statusMessage!=null && statusMessage.Length>0) { webStatusCode += " " + statusMessage; } else { // // Otherwise try to map the base status. // if (statusDescription!=null && statusDescription.Length>0) { webStatusCode += " " + statusDescription; } } return webStatusCode; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
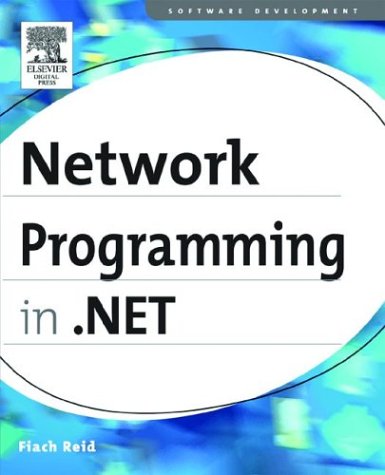
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NumericUpDown.cs
- UdpDiscoveryEndpointProvider.cs
- MultipartContentParser.cs
- DataGridCellEditEndingEventArgs.cs
- BuildProvider.cs
- Grammar.cs
- ClusterSafeNativeMethods.cs
- AssemblyCacheEntry.cs
- IdleTimeoutMonitor.cs
- OleDbConnection.cs
- ActivityValidator.cs
- ModifierKeysConverter.cs
- IntSecurity.cs
- KeyNotFoundException.cs
- ApplicationTrust.cs
- Codec.cs
- CodeArrayCreateExpression.cs
- MobileUserControl.cs
- documentsequencetextcontainer.cs
- Delegate.cs
- NamedPipeTransportSecurity.cs
- TextTreeUndoUnit.cs
- XamlWriterExtensions.cs
- PersonalizationAdministration.cs
- ScrollItemProviderWrapper.cs
- Pair.cs
- HandlerWithFactory.cs
- SecurityVerifiedMessage.cs
- NoneExcludedImageIndexConverter.cs
- AllMembershipCondition.cs
- GroupedContextMenuStrip.cs
- PropertyPathWorker.cs
- MethodBuilderInstantiation.cs
- Visitors.cs
- UserControl.cs
- XmlSchemaInferenceException.cs
- MenuItemBinding.cs
- NativeCppClassAttribute.cs
- FixedTextSelectionProcessor.cs
- IntSecurity.cs
- DynamicILGenerator.cs
- WebResourceUtil.cs
- FileDialog.cs
- DataGridBoolColumn.cs
- DbDeleteCommandTree.cs
- TextUtf8RawTextWriter.cs
- InvalidOleVariantTypeException.cs
- SmtpFailedRecipientException.cs
- CryptoHelper.cs
- Exceptions.cs
- StoreUtilities.cs
- ZipIORawDataFileBlock.cs
- QuadraticBezierSegment.cs
- DetailsViewActionList.cs
- ValidatingReaderNodeData.cs
- Token.cs
- SamlAuthorityBinding.cs
- BuildManager.cs
- EmissiveMaterial.cs
- IsolatedStorageFileStream.cs
- ZipArchive.cs
- X509AsymmetricSecurityKey.cs
- SystemTcpConnection.cs
- ButtonField.cs
- TraceHandlerErrorFormatter.cs
- StorageConditionPropertyMapping.cs
- ServiceOperationParameter.cs
- SortQuery.cs
- RSACryptoServiceProvider.cs
- XmlHierarchicalEnumerable.cs
- XmlBinaryReader.cs
- ListCollectionView.cs
- AppDomainShutdownMonitor.cs
- Triangle.cs
- RemoveFromCollection.cs
- Error.cs
- EncoderFallback.cs
- HostDesigntimeLicenseContext.cs
- InheritanceAttribute.cs
- GZipDecoder.cs
- ClientScriptManager.cs
- SemanticResolver.cs
- HttpCachePolicy.cs
- PageSettings.cs
- WsatStrings.cs
- Span.cs
- CollectionViewGroupInternal.cs
- VerticalAlignConverter.cs
- AlphaSortedEnumConverter.cs
- Metafile.cs
- ToolStripItemImageRenderEventArgs.cs
- BufferedMessageData.cs
- SplitterEvent.cs
- FileDialogCustomPlacesCollection.cs
- NegatedCellConstant.cs
- RegistryKey.cs
- BooleanProjectedSlot.cs
- HandlerMappingMemo.cs
- StringFunctions.cs
- AnnotationHelper.cs