Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / FeatureSupport.cs / 1305376 / FeatureSupport.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Configuration.Assemblies; using System.Diagnostics; using System; using System.Reflection; using System.Security; using System.Security.Permissions; ////// /// public abstract class FeatureSupport : IFeatureSupport { ///Provides ///methods for retrieving feature information from the /// current system. /// /// public static bool IsPresent(string featureClassName, string featureConstName) { return IsPresent(featureClassName, featureConstName, new Version(0, 0, 0, 0)); } ///Determines whether any version of the specified feature /// is installed in the system. This method is ///. /// /// public static bool IsPresent(string featureClassName, string featureConstName, Version minimumVersion) { object featureId = null; IFeatureSupport featureSupport = null; //APPCOMPAT: If Type.GetType() throws, we want to return //null to preserve Everett behavior. Type c = null; try { c = Type.GetType(featureClassName); } catch (ArgumentException) {} if (c != null) { FieldInfo fi = c.GetField(featureConstName); if (fi != null) { featureId = fi.GetValue(null); } } if (featureId != null && typeof(IFeatureSupport).IsAssignableFrom(c)) { featureSupport = (IFeatureSupport) SecurityUtils.SecureCreateInstance(c); if (featureSupport != null) { return featureSupport.IsPresent(featureId, minimumVersion); } } return false; } ///Determines whether the specified or newer version of the specified feature is /// installed in the system. This method is ///. /// /// public static Version GetVersionPresent(string featureClassName, string featureConstName) { object featureId = null; IFeatureSupport featureSupport = null; //APPCOMPAT: If Type.GetType() throws, we want to return //null to preserve Everett behavior. Type c = null; try { c = Type.GetType(featureClassName); } catch (ArgumentException) {} if (c != null) { FieldInfo fi = c.GetField(featureConstName); if (fi != null) { featureId = fi.GetValue(null); } } if (featureId != null) { featureSupport = (IFeatureSupport) SecurityUtils.SecureCreateInstance(c); if (featureSupport != null) { return featureSupport.GetVersionPresent(featureId); } } return null; } ///Gets the version of the specified feature that is available on the system. ////// /// public virtual bool IsPresent(object feature) { return IsPresent(feature, new Version(0, 0, 0, 0)); } ///Determines whether any version of the specified feature /// is installed in the system. ////// /// public virtual bool IsPresent(object feature, Version minimumVersion) { Version ver = GetVersionPresent(feature); if (ver != null) { return ver.CompareTo(minimumVersion) >= 0; } return false; } ///Determines whether the specified or newer version of the /// specified feature is installed in the system. ////// /// public abstract Version GetVersionPresent(object feature); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.When overridden in a derived class, gets the version of the specified /// feature that is available on the system. ///
Link Menu
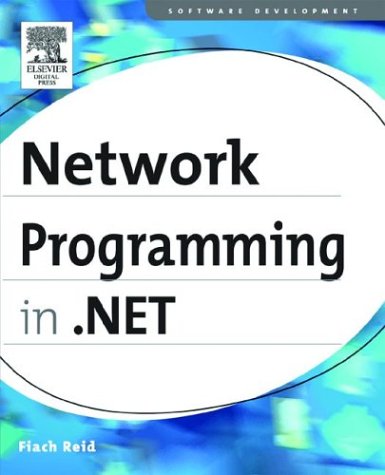
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TransactionChannelListener.cs
- DataGridViewCellCollection.cs
- AlternateViewCollection.cs
- FormViewDeletedEventArgs.cs
- ApplicationInfo.cs
- HtmlValidatorAdapter.cs
- EditCommandColumn.cs
- StickyNoteAnnotations.cs
- AppDomainFactory.cs
- CngKey.cs
- DesignerActionList.cs
- ToolBar.cs
- TcpTransportSecurityElement.cs
- ConfigXmlReader.cs
- ValidatorCompatibilityHelper.cs
- autovalidator.cs
- UriParserTemplates.cs
- SizeChangedInfo.cs
- WebPartMenu.cs
- SessionStateModule.cs
- FixedTextContainer.cs
- CodeAttributeDeclarationCollection.cs
- HtmlInputText.cs
- SecurityTokenContainer.cs
- DataGridViewRowConverter.cs
- UserPreferenceChangingEventArgs.cs
- TableProviderWrapper.cs
- FormattedText.cs
- CompModSwitches.cs
- ProfileParameter.cs
- ExpressionList.cs
- XNameConverter.cs
- ColumnClickEvent.cs
- DebuggerAttributes.cs
- HitTestWithPointDrawingContextWalker.cs
- TextElementEnumerator.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- BridgeDataReader.cs
- FileVersion.cs
- XmlSchemaInferenceException.cs
- BigIntegerStorage.cs
- ProfileProvider.cs
- VScrollBar.cs
- OutputCacheProfile.cs
- CardSpacePolicyElement.cs
- EmptyElement.cs
- UnmanagedMarshal.cs
- SeparatorAutomationPeer.cs
- TextTreePropertyUndoUnit.cs
- TreeNodeMouseHoverEvent.cs
- TextParagraphProperties.cs
- ISAPIApplicationHost.cs
- ColumnMapProcessor.cs
- OleDbRowUpdatingEvent.cs
- HiddenFieldPageStatePersister.cs
- StreamHelper.cs
- ObjectViewEntityCollectionData.cs
- rsa.cs
- RequiredFieldValidator.cs
- List.cs
- SHA512Managed.cs
- TrustLevelCollection.cs
- MessageQuerySet.cs
- AspNetSynchronizationContext.cs
- ExpressionConverter.cs
- FixedTextBuilder.cs
- SystemColors.cs
- BitmapImage.cs
- Token.cs
- SQLResource.cs
- AudienceUriMode.cs
- ResizingMessageFilter.cs
- Vector.cs
- BufferModesCollection.cs
- TableLayoutPanelCellPosition.cs
- ProfileSettings.cs
- Bidi.cs
- SubqueryRules.cs
- EndpointNotFoundException.cs
- TemplatedMailWebEventProvider.cs
- SqlGatherProducedAliases.cs
- ConfigPathUtility.cs
- ReachDocumentReferenceCollectionSerializer.cs
- StatusBarPanelClickEvent.cs
- IImplicitResourceProvider.cs
- ValidationRuleCollection.cs
- FileSecurity.cs
- SoapFormatter.cs
- ColumnMapProcessor.cs
- RoleExceptions.cs
- FormViewUpdatedEventArgs.cs
- IIS7UserPrincipal.cs
- ConnectionOrientedTransportChannelFactory.cs
- DLinqTableProvider.cs
- TextElement.cs
- Triplet.cs
- WebServiceHandler.cs
- FtpWebResponse.cs
- IteratorFilter.cs
- EntityContainerAssociationSet.cs