Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Compilation / CompilerTypeWithParams.cs / 1305376 / CompilerTypeWithParams.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Security.Permissions; using System.IO; using System.Collections; using System.Globalization; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Hosting; using System.Web.Util; using System.Web.UI; using System.Web.Configuration; /* * This class describes a CodeDom compiler, along with the parameters that it uses. * The reason we need this class is that if two files both use the same language, * but ask for different command line options (e.g. debug vs retail), we will not * be able to compile them together. So effectively, we need to treat them as * different languages. */ public sealed class CompilerType { private Type _codeDomProviderType; public Type CodeDomProviderType { get { return _codeDomProviderType; } } private CompilerParameters _compilParams; public CompilerParameters CompilerParameters { get { return _compilParams; } } internal CompilerType(Type codeDomProviderType, CompilerParameters compilParams) { Debug.Assert(codeDomProviderType != null); _codeDomProviderType = codeDomProviderType; if (compilParams == null) _compilParams = new CompilerParameters(); else _compilParams = compilParams; } internal CompilerType Clone() { // Clone the CompilerParameters to make sure the original is untouched return new CompilerType(_codeDomProviderType, CloneCompilerParameters()); } private CompilerParameters CloneCompilerParameters() { CompilerParameters copy = new CompilerParameters(); copy.IncludeDebugInformation = _compilParams.IncludeDebugInformation; copy.TreatWarningsAsErrors = _compilParams.TreatWarningsAsErrors; copy.WarningLevel = _compilParams.WarningLevel; copy.CompilerOptions = _compilParams.CompilerOptions; return copy; } public override int GetHashCode() { return _codeDomProviderType.GetHashCode(); } public override bool Equals(Object o) { CompilerType other = o as CompilerType; if (o == null) return false; return _codeDomProviderType == other._codeDomProviderType && _compilParams.WarningLevel == other._compilParams.WarningLevel && _compilParams.IncludeDebugInformation == other._compilParams.IncludeDebugInformation && _compilParams.CompilerOptions == other._compilParams.CompilerOptions; } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies) { return CreateAssemblyBuilder(compConfig, referencedAssemblies, null /*generatedFilesDir*/, null /*outputAssemblyName*/); } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, string generatedFilesDir, string outputAssemblyName) { // Create a special AssemblyBuilder when we're only supposed to generate // source files but not compile them (for ClientBuildManager.GetCodeDirectoryInformation) if (generatedFilesDir != null) { return new CbmCodeGeneratorBuildProviderHost(compConfig, referencedAssemblies, this, generatedFilesDir, outputAssemblyName); } return new AssemblyBuilder(compConfig, referencedAssemblies, this, outputAssemblyName); } private static CompilerType GetDefaultCompilerTypeWithParams( CompilationSection compConfig, VirtualPath configPath) { // By default, use C# when no provider is asking for a specific language return CompilationUtil.GetCSharpCompilerInfo(compConfig, configPath); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string outputAssemblyName) { return GetDefaultAssemblyBuilder(compConfig, referencedAssemblies, configPath, null /*generatedFilesDir*/, outputAssemblyName); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string generatedFilesDir, string outputAssemblyName) { CompilerType ctwp = GetDefaultCompilerTypeWithParams(compConfig, configPath); return ctwp.CreateAssemblyBuilder(compConfig, referencedAssemblies, generatedFilesDir, outputAssemblyName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
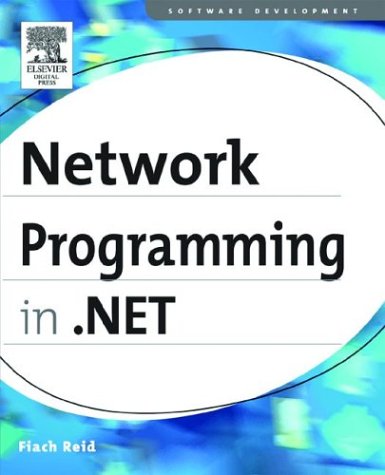
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DependencyProperty.cs
- TemplateNameScope.cs
- ObjectListSelectEventArgs.cs
- TreeViewImageKeyConverter.cs
- DataServiceEntityAttribute.cs
- DynamicAttribute.cs
- HtmlElement.cs
- UpdateManifestForBrowserApplication.cs
- TextDecoration.cs
- TextParagraph.cs
- ComponentResourceKeyConverter.cs
- EtwTrackingBehavior.cs
- TdsParserStaticMethods.cs
- ViewValidator.cs
- TagPrefixInfo.cs
- PerformanceCounterNameAttribute.cs
- Vector3D.cs
- ColorMap.cs
- XslTransform.cs
- TextOptionsInternal.cs
- BindingsCollection.cs
- IsolatedStorageFile.cs
- FormattedText.cs
- ObjectDataSourceFilteringEventArgs.cs
- Vector3DCollectionConverter.cs
- BaseTreeIterator.cs
- DataColumnChangeEvent.cs
- DescendantOverDescendantQuery.cs
- FlowNode.cs
- LambdaExpression.cs
- ResourcePool.cs
- DesigntimeLicenseContext.cs
- ConfigurationHandlersInstallComponent.cs
- EmptyEnumerable.cs
- ObjectNotFoundException.cs
- MemberNameValidator.cs
- UpdateTracker.cs
- DetailsViewDeleteEventArgs.cs
- PropertyPath.cs
- QueryableDataSourceEditData.cs
- AssemblyUtil.cs
- PrintingPermissionAttribute.cs
- TableAdapterManagerGenerator.cs
- XmlCharCheckingWriter.cs
- WithParamAction.cs
- MatrixCamera.cs
- XmlTextWriter.cs
- AttachedPropertyBrowsableAttribute.cs
- InputProcessorProfilesLoader.cs
- SecureUICommand.cs
- Timer.cs
- _NestedMultipleAsyncResult.cs
- StorageComplexPropertyMapping.cs
- SqlServices.cs
- CodeTypeOfExpression.cs
- InheritedPropertyChangedEventArgs.cs
- ProtocolsConfiguration.cs
- HyperLinkStyle.cs
- ButtonStandardAdapter.cs
- SecurityRuntime.cs
- EditorZoneBase.cs
- ReadOnlyHierarchicalDataSource.cs
- ThreadSafeList.cs
- SQLByteStorage.cs
- ForceCopyBuildProvider.cs
- DynamicILGenerator.cs
- AmbientLight.cs
- TaskForm.cs
- MediaScriptCommandRoutedEventArgs.cs
- DoubleSumAggregationOperator.cs
- Internal.cs
- PropertyPushdownHelper.cs
- CatalogZone.cs
- TemplateInstanceAttribute.cs
- RemoteCryptoSignHashRequest.cs
- AstTree.cs
- GuidelineSet.cs
- WebBrowserUriTypeConverter.cs
- InputEventArgs.cs
- ProcessModelInfo.cs
- DbConnectionStringCommon.cs
- PageAsyncTask.cs
- Memoizer.cs
- Model3DCollection.cs
- ComponentDispatcher.cs
- ConstructorNeedsTagAttribute.cs
- ChannelServices.cs
- ErrorReporting.cs
- BufferedReadStream.cs
- DictionarySectionHandler.cs
- Helper.cs
- DataError.cs
- Ppl.cs
- BitmapFrame.cs
- Compiler.cs
- BinaryKeyIdentifierClause.cs
- Deflater.cs
- TextServicesHost.cs
- SchemaReference.cs
- StateMachine.cs