Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / DataGridTextColumn.cs / 1305600 / DataGridTextColumn.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; namespace System.Windows.Controls { ////// A column that displays editable text. /// public class DataGridTextColumn : DataGridBoundColumn { static DataGridTextColumn() { ElementStyleProperty.OverrideMetadata(typeof(DataGridTextColumn), new FrameworkPropertyMetadata(DefaultElementStyle)); EditingElementStyleProperty.OverrideMetadata(typeof(DataGridTextColumn), new FrameworkPropertyMetadata(DefaultEditingElementStyle)); } #region Styles ////// The default value of the ElementStyle property. /// This value can be used as the BasedOn for new styles. /// public static Style DefaultElementStyle { get { if (_defaultElementStyle == null) { Style style = new Style(typeof(TextBlock)); // Use the same margin used on the TextBox to provide space for the caret style.Setters.Add(new Setter(TextBlock.MarginProperty, new Thickness(2.0, 0.0, 2.0, 0.0))); style.Seal(); _defaultElementStyle = style; } return _defaultElementStyle; } } ////// The default value of the EditingElementStyle property. /// This value can be used as the BasedOn for new styles. /// public static Style DefaultEditingElementStyle { get { if (_defaultEditingElementStyle == null) { Style style = new Style(typeof(TextBox)); style.Setters.Add(new Setter(TextBox.BorderThicknessProperty, new Thickness(0.0))); style.Setters.Add(new Setter(TextBox.PaddingProperty, new Thickness(0.0))); style.Seal(); _defaultEditingElementStyle = style; } return _defaultEditingElementStyle; } } #endregion #region Element Generation ////// Creates the visual tree for text based cells. /// protected override FrameworkElement GenerateElement(DataGridCell cell, object dataItem) { TextBlock textBlock = new TextBlock(); SyncProperties(textBlock); ApplyStyle(/* isEditing = */ false, /* defaultToElementStyle = */ false, textBlock); ApplyBinding(textBlock, TextBlock.TextProperty); return textBlock; } ////// Creates the visual tree for text based cells. /// protected override FrameworkElement GenerateEditingElement(DataGridCell cell, object dataItem) { TextBox textBox = new TextBox(); SyncProperties(textBox); ApplyStyle(/* isEditing = */ true, /* defaultToElementStyle = */ false, textBox); ApplyBinding(textBox, TextBox.TextProperty); return textBox; } private void SyncProperties(FrameworkElement e) { DataGridHelper.SyncColumnProperty(this, e, TextElement.FontFamilyProperty, FontFamilyProperty); DataGridHelper.SyncColumnProperty(this, e, TextElement.FontSizeProperty, FontSizeProperty); DataGridHelper.SyncColumnProperty(this, e, TextElement.FontStyleProperty, FontStyleProperty); DataGridHelper.SyncColumnProperty(this, e, TextElement.FontWeightProperty, FontWeightProperty); DataGridHelper.SyncColumnProperty(this, e, TextElement.ForegroundProperty, ForegroundProperty); } protected internal override void RefreshCellContent(FrameworkElement element, string propertyName) { DataGridCell cell = element as DataGridCell; if (cell != null) { FrameworkElement textElement = cell.Content as FrameworkElement; if (textElement != null) { switch (propertyName) { case "FontFamily": DataGridHelper.SyncColumnProperty(this, textElement, TextElement.FontFamilyProperty, FontFamilyProperty); break; case "FontSize": DataGridHelper.SyncColumnProperty(this, textElement, TextElement.FontSizeProperty, FontSizeProperty); break; case "FontStyle": DataGridHelper.SyncColumnProperty(this, textElement, TextElement.FontStyleProperty, FontStyleProperty); break; case "FontWeight": DataGridHelper.SyncColumnProperty(this, textElement, TextElement.FontWeightProperty, FontWeightProperty); break; case "Foreground": DataGridHelper.SyncColumnProperty(this, textElement, TextElement.ForegroundProperty, ForegroundProperty); break; } } } base.RefreshCellContent(element, propertyName); } #endregion #region Editing ////// Called when a cell has just switched to edit mode. /// /// A reference to element returned by GenerateEditingElement. /// The event args of the input event that caused the cell to go into edit mode. May be null. ///The unedited value of the cell. protected override object PrepareCellForEdit(FrameworkElement editingElement, RoutedEventArgs editingEventArgs) { TextBox textBox = editingElement as TextBox; if (textBox != null) { textBox.Focus(); string originalValue = textBox.Text; TextCompositionEventArgs textArgs = editingEventArgs as TextCompositionEventArgs; if (textArgs != null) { // If text input started the edit, then replace the text with what was typed. string inputText = textArgs.Text; textBox.Text = inputText; // Place the caret after the end of the text. textBox.Select(inputText.Length, 0); } else { // If a mouse click started the edit, then place the caret under the mouse. MouseButtonEventArgs mouseArgs = editingEventArgs as MouseButtonEventArgs; if ((mouseArgs == null) || !PlaceCaretOnTextBox(textBox, Mouse.GetPosition(textBox))) { // If the mouse isn't over the textbox or something else started the edit, then select the text. textBox.SelectAll(); } } return originalValue; } return null; } private static bool PlaceCaretOnTextBox(TextBox textBox, Point position) { int characterIndex = textBox.GetCharacterIndexFromPoint(position, /* snapToText = */ false); if (characterIndex >= 0) { textBox.Select(characterIndex, 0); return true; } return false; } internal override void OnInput(InputEventArgs e) { // Text input will start an edit. // Escape is meant to be for CancelEdit. But DataGrid // may not handle KeyDown event for Escape if nothing // is cancelable. Such KeyDown if unhandled by others // will ultimately get promoted to TextInput and be handled // here. But BeginEdit on escape could be confusing to the user. // Hence escape key is special case and BeginEdit is performed if // there is atleast one non espace key character. if (DataGridHelper.HasNonEscapeCharacters(e as TextCompositionEventArgs)) { BeginEdit(e); } } #endregion #region Element Properties ////// The DependencyProperty for the FontFamily property. /// Flags: Can be used in style rules /// Default Value: System Dialog Font /// public static readonly DependencyProperty FontFamilyProperty = TextElement.FontFamilyProperty.AddOwner( typeof(DataGridTextColumn), new FrameworkPropertyMetadata(SystemFonts.MessageFontFamily, FrameworkPropertyMetadataOptions.Inherits, DataGridColumn.NotifyPropertyChangeForRefreshContent)); ////// The font family of the desired font. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// public FontFamily FontFamily { get { return (FontFamily)GetValue(FontFamilyProperty); } set { SetValue(FontFamilyProperty, value); } } ////// The DependencyProperty for the FontSize property. /// Flags: Can be used in style rules /// Default Value: System Dialog Font Size /// public static readonly DependencyProperty FontSizeProperty = TextElement.FontSizeProperty.AddOwner( typeof(DataGridTextColumn), new FrameworkPropertyMetadata(SystemFonts.MessageFontSize, FrameworkPropertyMetadataOptions.Inherits, DataGridColumn.NotifyPropertyChangeForRefreshContent)); ////// The size of the desired font. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// [TypeConverter(typeof(FontSizeConverter))] [Localizability(LocalizationCategory.None)] public double FontSize { get { return (double)GetValue(FontSizeProperty); } set { SetValue(FontSizeProperty, value); } } ////// The DependencyProperty for the FontStyle property. /// Flags: Can be used in style rules /// Default Value: System Dialog Font Style /// public static readonly DependencyProperty FontStyleProperty = TextElement.FontStyleProperty.AddOwner( typeof(DataGridTextColumn), new FrameworkPropertyMetadata(SystemFonts.MessageFontStyle, FrameworkPropertyMetadataOptions.Inherits, DataGridColumn.NotifyPropertyChangeForRefreshContent)); ////// The style of the desired font. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// public FontStyle FontStyle { get { return (FontStyle)GetValue(FontStyleProperty); } set { SetValue(FontStyleProperty, value); } } ////// The DependencyProperty for the FontWeight property. /// Flags: Can be used in style rules /// Default Value: System Dialog Font Weight /// public static readonly DependencyProperty FontWeightProperty = TextElement.FontWeightProperty.AddOwner( typeof(DataGridTextColumn), new FrameworkPropertyMetadata(SystemFonts.MessageFontWeight, FrameworkPropertyMetadataOptions.Inherits, DataGridColumn.NotifyPropertyChangeForRefreshContent)); ////// The weight or thickness of the desired font. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// public FontWeight FontWeight { get { return (FontWeight)GetValue(FontWeightProperty); } set { SetValue(FontWeightProperty, value); } } ////// The DependencyProperty for the Foreground property. /// Flags: Can be used in style rules /// Default Value: System Font Color /// public static readonly DependencyProperty ForegroundProperty = TextElement.ForegroundProperty.AddOwner( typeof(DataGridTextColumn), new FrameworkPropertyMetadata(SystemColors.ControlTextBrush, FrameworkPropertyMetadataOptions.Inherits, DataGridColumn.NotifyPropertyChangeForRefreshContent)); ////// An brush that describes the foreground color. /// This will only affect controls whose template uses the property /// as a parameter. On other controls, the property will do nothing. /// public Brush Foreground { get { return (Brush)GetValue(ForegroundProperty); } set { SetValue(ForegroundProperty, value); } } #endregion #region Data private static Style _defaultElementStyle; private static Style _defaultEditingElementStyle; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
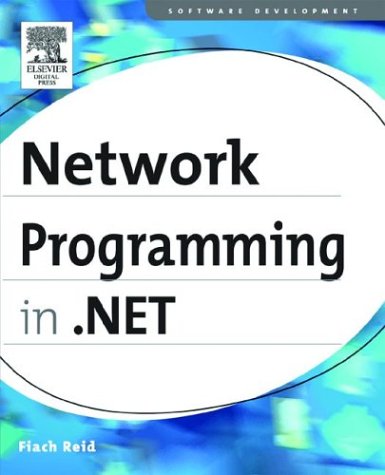
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RelationshipConstraintValidator.cs
- Errors.cs
- ImageButton.cs
- ArgumentsParser.cs
- CellParaClient.cs
- NullRuntimeConfig.cs
- DefaultObjectMappingItemCollection.cs
- GlobalItem.cs
- ModelUIElement3D.cs
- SqlCacheDependencyDatabase.cs
- StandardToolWindows.cs
- X509Utils.cs
- QueryExecutionOption.cs
- BitmapMetadata.cs
- Assert.cs
- Int64Animation.cs
- GifBitmapDecoder.cs
- CDSCollectionETWBCLProvider.cs
- OracleMonthSpan.cs
- ToolStripManager.cs
- Axis.cs
- JsonStringDataContract.cs
- ToolStripControlHost.cs
- Condition.cs
- IProvider.cs
- AttachedPropertyMethodSelector.cs
- TerminateWorkflow.cs
- WindowsSecurityToken.cs
- PostBackTrigger.cs
- QilNode.cs
- Rules.cs
- XmlSerializerFactory.cs
- _IPv6Address.cs
- MailSettingsSection.cs
- SqlColumnizer.cs
- RowsCopiedEventArgs.cs
- UnsafeNativeMethods.cs
- Transform3DGroup.cs
- TemplateInstanceAttribute.cs
- DiscoveryReferences.cs
- TemplatePagerField.cs
- WorkflowElementDialog.cs
- Substitution.cs
- RemotingConfiguration.cs
- ToolStripItemBehavior.cs
- ScrollPattern.cs
- NamedPipeHostedTransportConfiguration.cs
- HtmlImage.cs
- DeviceContext2.cs
- COM2TypeInfoProcessor.cs
- PasswordDeriveBytes.cs
- ResourceContainer.cs
- CompositeCollection.cs
- ObjectStorage.cs
- VectorConverter.cs
- AtomContentProperty.cs
- XmlQueryCardinality.cs
- ProjectionCamera.cs
- AncestorChangedEventArgs.cs
- Byte.cs
- SqlDependency.cs
- CqlErrorHelper.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- ApplicationManager.cs
- StringPropertyBuilder.cs
- SqlClientWrapperSmiStreamChars.cs
- ExeContext.cs
- FileDialog.cs
- WSFederationHttpBindingCollectionElement.cs
- Logging.cs
- InputBinder.cs
- SqlDataSourceCache.cs
- DiagnosticEventProvider.cs
- CaseInsensitiveComparer.cs
- GeometryDrawing.cs
- ReferenceConverter.cs
- AddInBase.cs
- UserUseLicenseDictionaryLoader.cs
- KnownColorTable.cs
- DbMetaDataColumnNames.cs
- RouteParser.cs
- ToolCreatedEventArgs.cs
- MobileControlsSectionHelper.cs
- ContainsSearchOperator.cs
- ProviderConnectionPoint.cs
- PathSegmentCollection.cs
- Overlapped.cs
- WebPartCollection.cs
- SessionSwitchEventArgs.cs
- NamespaceQuery.cs
- ImplicitInputBrush.cs
- FixedTextContainer.cs
- InvalidDataContractException.cs
- UnsafeNativeMethods.cs
- WebPartActionVerb.cs
- UpdateTracker.cs
- CollectionViewGroupRoot.cs
- _ConnectOverlappedAsyncResult.cs
- FormViewPagerRow.cs
- ParsedAttributeCollection.cs