Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / AccessibleTech / longhorn / Automation / UIAutomationTypes / System / Windows / Automation / AutomationIdentifier.cs / 1 / AutomationIdentifier.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Base class for Automation Idenfitiers (Property, Event, etc.) // // History: // 06/02/2003 : [....] Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Diagnostics; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Base class for object identity based identifiers. /// Implement ISerializable to ensure that it remotes propertly /// This class is effectively abstract, only derived classes are /// instantiated. /// #if (INTERNAL_COMPILE) internal class AutomationIdentifier : IComparable #else public class AutomationIdentifier : IComparable #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Internal so only our own derived classes can actually // use this class. (3rd party classes can try deriving, // but the internal ctor will prevent instantiation.) internal AutomationIdentifier(UiaCoreTypesApi.AutomationIdType type, int id, Guid guid, string programmaticName) { Debug.Assert(id != 0); _id = id; _type = type; _guid = guid; _programmaticName = programmaticName; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// Returns underlying identifier as used by provider interfaces. /// ////// Use LookupById method to convert back from Id to an /// AutomationIdentifier /// public int Id { get { return _id; } } ////// Returns the programmatic name passed in on registration. /// ////// Appends the type to the programmatic name. /// public string ProgrammaticName { get { return _programmaticName; } } #endregion Public Properties //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Tests whether two AutomationIdentifier objects are equivalent /// public override bool Equals( object obj ) { return obj == (object)this; } ////// Overrides Object.GetHashCode() /// public override int GetHashCode() { return base.GetHashCode(); } ////// For IComparable() /// public int CompareTo(object obj) { Debug.Assert(obj != null, "Null obj!"); if (obj == null) throw new ArgumentNullException("obj"); // Ordering allows arrays of references to these to be sorted - though the sort order is undefined. Debug.Assert(obj is AutomationIdentifier, "CompareTo called with unexpected type"); return GetHashCode() - obj.GetHashCode(); } #endregion Public Methods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static AutomationIdentifier Register(UiaCoreTypesApi.AutomationIdType type, Guid guid, string programmaticName) { // Get internal id from the guid... int id = UiaCoreTypesApi.UiaLookupId(type, ref guid); if (id == 0) { Debug.Assert(id != 0, "Identifier not recognized by core - " + guid); return null; } lock (_idTable) { // See if instance already exists... AutomationIdentifier autoid = (AutomationIdentifier)_idTable[guid]; if (autoid != null) { return autoid; } // If not, create one... switch (type) { case UiaCoreTypesApi.AutomationIdType.Property: autoid = new AutomationProperty(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.Event: autoid = new AutomationEvent(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.TextAttribute: autoid = new AutomationTextAttribute(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.Pattern: autoid = new AutomationPattern(id, guid, programmaticName); break; case UiaCoreTypesApi.AutomationIdType.ControlType: autoid = new ControlType(id, guid, programmaticName); break; default: Debug.Assert(false, "Invalid type specified for AutomationIdentifier"); throw new InvalidOperationException("Invalid type specified for AutomationIdentifier"); } _idTable[id] = autoid; return autoid; } } internal static AutomationIdentifier LookupById(UiaCoreTypesApi.AutomationIdType type, int id) { AutomationIdentifier autoid; lock (_idTable) { autoid = (AutomationIdentifier) _idTable[id]; } if(autoid == null) { return null; } if(autoid._type != type) { return null; } return autoid; } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private Guid _guid; private UiaCoreTypesApi.AutomationIdType _type; private int _id; // value used in core private string _programmaticName; // As of 8/18/03 there were 187 entries added in a normal loading private static Hashtable _idTable = new Hashtable(200,1.0f); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
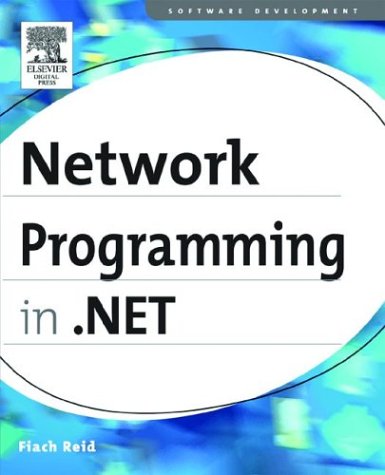
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CollectionViewSource.cs
- HelpProvider.cs
- CodeIdentifiers.cs
- GlyphRunDrawing.cs
- RefreshPropertiesAttribute.cs
- _UriTypeConverter.cs
- NamedObject.cs
- ConfigurationSettings.cs
- DeflateEmulationStream.cs
- QueryCoreOp.cs
- _BaseOverlappedAsyncResult.cs
- URLString.cs
- DependencySource.cs
- CustomAttributeBuilder.cs
- ProcessHostFactoryHelper.cs
- Win32PrintDialog.cs
- UpdateCommandGenerator.cs
- HttpClientChannel.cs
- AdornerPresentationContext.cs
- HMACMD5.cs
- Font.cs
- EntityRecordInfo.cs
- CompilationPass2Task.cs
- TabItemAutomationPeer.cs
- SerialReceived.cs
- XmlSchemaObjectTable.cs
- Size3DValueSerializer.cs
- Panel.cs
- ConfigViewGenerator.cs
- FlowNode.cs
- MembershipPasswordException.cs
- DecimalConstantAttribute.cs
- VisualStyleTypesAndProperties.cs
- UpdateRecord.cs
- CompositeScriptReference.cs
- ActionNotSupportedException.cs
- RtfToXamlReader.cs
- DetailsViewInsertEventArgs.cs
- ButtonBaseAdapter.cs
- DataKeyCollection.cs
- IndentedWriter.cs
- VideoDrawing.cs
- Point4D.cs
- FixedDocumentSequencePaginator.cs
- _ChunkParse.cs
- DataFormats.cs
- AnnotationResourceCollection.cs
- ToolStripGripRenderEventArgs.cs
- TreeNodeBindingCollection.cs
- WebPartDeleteVerb.cs
- SimpleHandlerFactory.cs
- PropertyOverridesDialog.cs
- SoapSchemaImporter.cs
- ListBox.cs
- shaperfactoryquerycacheentry.cs
- AutomationTextAttribute.cs
- List.cs
- EncoderExceptionFallback.cs
- MobileRedirect.cs
- HtmlElementErrorEventArgs.cs
- Path.cs
- TypeElement.cs
- UriSection.cs
- CollectionConverter.cs
- odbcmetadatacollectionnames.cs
- FlowDocumentReader.cs
- ProfilePropertyNameValidator.cs
- CompilationPass2TaskInternal.cs
- StyleSelector.cs
- XmlSchemaComplexType.cs
- ValidationSummary.cs
- ProcessHostFactoryHelper.cs
- LayoutSettings.cs
- Control.cs
- BypassElement.cs
- PlainXmlSerializer.cs
- Compiler.cs
- XmlLinkedNode.cs
- UInt64Converter.cs
- BinaryNode.cs
- BindingCompleteEventArgs.cs
- BuildManagerHost.cs
- XDRSchema.cs
- EnumBuilder.cs
- DecimalKeyFrameCollection.cs
- StorageMappingItemLoader.cs
- NullPackagingPolicy.cs
- Converter.cs
- precedingsibling.cs
- SystemDropShadowChrome.cs
- LinqDataSourceDeleteEventArgs.cs
- Deflater.cs
- TextProviderWrapper.cs
- WeakReference.cs
- SymLanguageVendor.cs
- DashStyles.cs
- ComponentCollection.cs
- ExpressionVisitor.cs
- DBConcurrencyException.cs
- WmpBitmapDecoder.cs