Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / TextFormatting / DrawingState.cs / 1 / DrawingState.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: DrawingState.cs // // Contents: Drawing state of full text // // Created: 1-29-2005 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Media; namespace MS.Internal.TextFormatting { ////// Formatting state of full text /// internal sealed class DrawingState : IDisposable { private TextMetrics.FullTextLine _currentLine; // full text line currently formatted private DrawingContext _drawingContext; // current drawing context private Point _lineOrigin; // line origin XY relative to drawing context reference location private Point _vectorToLineOrigin; // vector to line origin in UV relative to paragraph start private MatrixTransform _antiInversion; // anti-inversion transform applied on drawing surface private bool _overrideBaseGuidelineY; // a flag indicating whether a new guideline overrides the line's Y guideline private double _baseGuidelineY; // the Y guideline of the text line. ////// Construct drawing state for full text /// internal DrawingState( DrawingContext drawingContext, Point lineOrigin, MatrixTransform antiInversion, TextMetrics.FullTextLine currentLine ) { _drawingContext = drawingContext; _antiInversion = antiInversion; _currentLine = currentLine; if (antiInversion == null) { _lineOrigin = lineOrigin; } else { _vectorToLineOrigin = lineOrigin; } if (_drawingContext != null) { // LineServices draws GlyphRun and TextDecorations in multiple // callbacks and GlyphRuns may have different baselines. Pushing guideline // for each DrawGlyphRun are too costly. We optimize for the common case where // GlyphRuns and TextDecorations in the TextLine share the same baseline. _baseGuidelineY = lineOrigin.Y + currentLine.Baseline; _drawingContext.PushGuidelineY1(_baseGuidelineY); } } ////// Set guideline Y for a drawing operation if necessary. It is a no-op if the Y value is the same /// as the guideline Y of the line. Otherwise, it will push the Y to override the guideline of the line. /// A SetGuidelineY() must be paired with an UnsetGuidelineY() to ensure balanced push and pop. /// internal void SetGuidelineY(double runGuidelineY) { if (_drawingContext == null) return; Invariant.Assert(!_overrideBaseGuidelineY); if (runGuidelineY != _baseGuidelineY) { // Push a new guideline to override the line's guideline _drawingContext.PushGuidelineY1(runGuidelineY); _overrideBaseGuidelineY = true; // the new Guideline Y overrides the line's guideline until next unset. } } ////// Unset guideline Y for a drawing operation if necessary. It is a no-op if the Y value is the same /// as the guideline Y of the line. Otherwise, it will push the Y to override the guideline of the line. /// A SetGuidelineY() must be paired with an UnsetGuidelineY() to ensure balanced push and pop. /// internal void UnsetGuidelineY() { if (_overrideBaseGuidelineY) { _drawingContext.Pop(); _overrideBaseGuidelineY = false; } } ////// Clean up internal state. /// public void Dispose() { // clear the guideline at line's baseline if (_drawingContext != null) { _drawingContext.Pop(); } } ////// Current drawing context /// internal DrawingContext DrawingContext { get { return _drawingContext; } } ////// Anti-inversion transform applied on drawing surface /// internal MatrixTransform AntiInversion { get { return _antiInversion; } } ////// Origin XY of the current line relative to the drawing context reference location /// internal Point LineOrigin { get { return _lineOrigin; } } ////// Vector to line origin in UV relative to paragraph start /// internal Point VectorToLineOrigin { get { return _vectorToLineOrigin; } } ////// Line currently being drawn /// internal TextMetrics.FullTextLine CurrentLine { get { return _currentLine; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
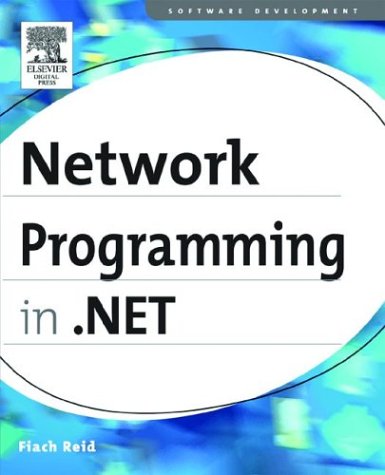
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateGroupCollection.cs
- CodeValidator.cs
- GeometryGroup.cs
- ProfileSettings.cs
- ByteStreamMessage.cs
- EnumBuilder.cs
- TabItemWrapperAutomationPeer.cs
- ListViewCancelEventArgs.cs
- HMACSHA384.cs
- WebBrowserBase.cs
- DropShadowBitmapEffect.cs
- TextOutput.cs
- ValueOfAction.cs
- FolderLevelBuildProvider.cs
- Collection.cs
- SystemWebCachingSectionGroup.cs
- SystemSounds.cs
- ApplicationCommands.cs
- KnownTypes.cs
- NameNode.cs
- DynamicResourceExtensionConverter.cs
- WindowsFormsHost.cs
- AnnotationService.cs
- DocumentGrid.cs
- HttpContext.cs
- BitmapDownload.cs
- PageCache.cs
- EventsTab.cs
- WindowsSecurityToken.cs
- RtfControls.cs
- CompressEmulationStream.cs
- DecimalAverageAggregationOperator.cs
- Compilation.cs
- ContainerSelectorActiveEvent.cs
- ObjectView.cs
- TableCell.cs
- MessageContractImporter.cs
- Sorting.cs
- PrintingPermissionAttribute.cs
- NameTable.cs
- EncryptedType.cs
- DoWorkEventArgs.cs
- ApplicationHost.cs
- activationcontext.cs
- HtmlElementErrorEventArgs.cs
- MenuScrollingVisibilityConverter.cs
- Control.cs
- glyphs.cs
- MsmqReceiveHelper.cs
- XmlMtomReader.cs
- ImportCatalogPart.cs
- DesignTimeTemplateParser.cs
- StorageModelBuildProvider.cs
- AlphabeticalEnumConverter.cs
- NamespaceTable.cs
- RulePatternOps.cs
- Token.cs
- StoragePropertyMapping.cs
- WebPartConnectionsDisconnectVerb.cs
- DataGridRow.cs
- VisualBrush.cs
- Comparer.cs
- FileCodeGroup.cs
- ReadWriteSpinLock.cs
- StorageTypeMapping.cs
- ApplicationServiceManager.cs
- ExceptionList.cs
- ParenthesizePropertyNameAttribute.cs
- ExplicitDiscriminatorMap.cs
- Validator.cs
- _HelperAsyncResults.cs
- DataServiceException.cs
- MemberRelationshipService.cs
- BrowserCapabilitiesCompiler.cs
- DataRowCollection.cs
- AttachmentCollection.cs
- EncryptedReference.cs
- XmlSchemaChoice.cs
- ManualResetEvent.cs
- TextTreeInsertUndoUnit.cs
- LocatorGroup.cs
- MobileControlsSection.cs
- WebPartZoneBaseDesigner.cs
- ServiceModelConfigurationSectionCollection.cs
- ModelItemCollectionImpl.cs
- ExtendedProperty.cs
- WebPartCollection.cs
- Set.cs
- SecurityPermission.cs
- MessageDesigner.cs
- FormViewInsertEventArgs.cs
- DataServiceConfiguration.cs
- DBDataPermission.cs
- SystemEvents.cs
- LookupNode.cs
- StopStoryboard.cs
- WindowInteropHelper.cs
- SymDocumentType.cs
- InheritanceUI.cs
- IdentitySection.cs