Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / UniqueEventHelper.cs / 1 / UniqueEventHelper.cs
using System; using System.Collections; using System.Diagnostics; namespace System.Windows.Media { ////// Aids in making events unique. i.e. you register the same function for /// the same event twice, but it will only be called once for each time /// the event is invoked. /// /// UniqueEventHelper should only be accessed from the UI thread so that /// handlers that throw exceptions will crash the app, making the developer /// aware of the problem. /// internal class UniqueEventHelperwhere TEventArgs : EventArgs { /// /// Add the handler to the list of handlers associated with this event. /// If the handler has already been added, we simply increment the ref /// count. That way if this same handler has already been added, it /// won't be invoked multiple times when the event is raised. /// internal void AddEvent(EventHandlerhandler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] == null) { _htDelegates.Add(handler, 1); } else { int refCount = (int)_htDelegates[handler] + 1; _htDelegates[handler] = refCount; } } /// /// Removed the handler from the list of handlers associated with this /// event. If the handler has been added multiple times (more times than /// it has been removed), we simply decrement its ref count. /// internal void RemoveEvent(EventHandlerhandler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] != null) { int refCount = (int)_htDelegates[handler]; if (refCount == 1) { _htDelegates.Remove(handler); } else { _htDelegates[handler] = refCount - 1; } } } /// /// Enumerates all the keys in the hashtable, which must be EventHandlers, /// and invokes them. /// /// The sender for the callback. /// The args object to be sent by the delegate internal void InvokeEvents(object sender, TEventArgs args) { Debug.Assert((sender != null), "Sender is null"); if (_htDelegates != null) { Hashtable htDelegates = (Hashtable)_htDelegates.Clone(); foreach (EventHandlerhandler in htDelegates.Keys) { Debug.Assert((handler != null), "Event handler is null"); handler(sender, args); } } } /// /// Clones the event helper /// internal UniqueEventHelperClone() { UniqueEventHelper ueh = new UniqueEventHelper (); if (_htDelegates != null) { ueh._htDelegates = (Hashtable)_htDelegates.Clone(); } return ueh; } /// /// Ensures Hashtable is created so that event handlers can be added/removed /// private void EnsureEventTable() { if (_htDelegates == null) { _htDelegates = new Hashtable(); } } private Hashtable _htDelegates; } // internal class UniqueEventHelper { ////// Add the handler to the list of handlers associated with this event. /// If the handler has already been added, we simply increment the ref /// count. That way if this same handler has already been added, it /// won't be invoked multiple times when the event is raised. /// internal void AddEvent(EventHandler handler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] == null) { _htDelegates.Add(handler, 1); } else { int refCount = (int)_htDelegates[handler] + 1; _htDelegates[handler] = refCount; } } ////// Removed the handler from the list of handlers associated with this /// event. If the handler has been added multiple times (more times than /// it has been removed), we simply decrement its ref count. /// internal void RemoveEvent(EventHandler handler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] != null) { int refCount = (int)_htDelegates[handler]; if (refCount == 1) { _htDelegates.Remove(handler); } else { _htDelegates[handler] = refCount - 1; } } } ////// Enumerates all the keys in the hashtable, which must be EventHandlers, /// and invokes them. /// /// The sender for the callback. /// The args object to be sent by the delegate internal void InvokeEvents(object sender, EventArgs args) { Debug.Assert((sender != null), "Sender is null"); if (_htDelegates != null) { Hashtable htDelegates = (Hashtable)_htDelegates.Clone(); foreach (EventHandler handler in htDelegates.Keys) { Debug.Assert((handler != null), "Event handler is null"); handler(sender, args); } } } ////// Clones the event helper /// internal UniqueEventHelper Clone() { UniqueEventHelper ueh = new UniqueEventHelper(); if (_htDelegates != null) { ueh._htDelegates = (Hashtable)_htDelegates.Clone(); } return ueh; } ////// Ensures Hashtable is created so that event handlers can be added/removed /// private void EnsureEventTable() { if (_htDelegates == null) { _htDelegates = new Hashtable(); } } private Hashtable _htDelegates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
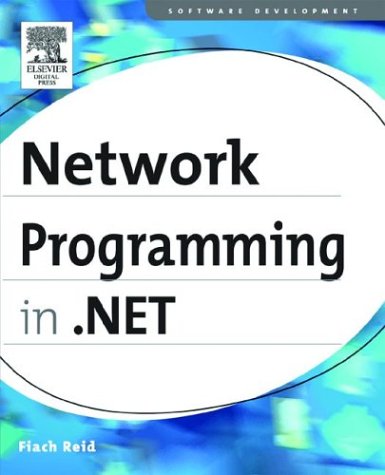
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BamlLocalizer.cs
- TCPListener.cs
- TableHeaderCell.cs
- ServiceErrorHandler.cs
- UpdateCommandGenerator.cs
- PipelineModuleStepContainer.cs
- MSHTMLHostUtil.cs
- X509ServiceCertificateAuthenticationElement.cs
- SystemException.cs
- TypeResolver.cs
- ValidationHelper.cs
- ExtendedProperty.cs
- RelationshipNavigation.cs
- SessionPageStatePersister.cs
- ToolStripPanelRenderEventArgs.cs
- MenuStrip.cs
- Range.cs
- CellConstantDomain.cs
- SqlClientWrapperSmiStream.cs
- SimpleMailWebEventProvider.cs
- Constants.cs
- MemberMaps.cs
- ClientApiGenerator.cs
- Socket.cs
- InputQueue.cs
- HttpWebResponse.cs
- DependencyObjectValidator.cs
- Keyboard.cs
- SliderAutomationPeer.cs
- HttpListenerResponse.cs
- TypeDescriptorContext.cs
- Viewport2DVisual3D.cs
- ActivityCodeDomReferenceService.cs
- X509InitiatorCertificateServiceElement.cs
- BooleanConverter.cs
- PropertyMappingExceptionEventArgs.cs
- DesignerCalendarAdapter.cs
- Walker.cs
- AsymmetricKeyExchangeDeformatter.cs
- RoutedCommand.cs
- LineSegment.cs
- FilteredDataSetHelper.cs
- AttachedAnnotation.cs
- _ShellExpression.cs
- FilterElement.cs
- TcpTransportBindingElement.cs
- HandlerFactoryWrapper.cs
- X509ChainPolicy.cs
- CompositeDesignerAccessibleObject.cs
- MessageQueueTransaction.cs
- OutOfMemoryException.cs
- ImageMapEventArgs.cs
- OverlappedAsyncResult.cs
- SqlConnectionPoolProviderInfo.cs
- ProgressiveCrcCalculatingStream.cs
- CustomAttributeSerializer.cs
- CustomExpressionEventArgs.cs
- GraphicsPathIterator.cs
- SortAction.cs
- PageContent.cs
- SignatureHelper.cs
- GregorianCalendar.cs
- FontWeightConverter.cs
- MaskedTextProvider.cs
- GC.cs
- MonitorWrapper.cs
- X509Extension.cs
- tooltip.cs
- ZipFileInfo.cs
- UnauthorizedWebPart.cs
- Table.cs
- BitmapEffectGroup.cs
- ProtocolViolationException.cs
- Object.cs
- DateTimeFormatInfo.cs
- ConfigurationManagerInternal.cs
- MemberRestriction.cs
- XmlWrappingReader.cs
- CultureSpecificStringDictionary.cs
- ContractMethodInfo.cs
- WebBrowserNavigatingEventHandler.cs
- DataServiceKeyAttribute.cs
- FastEncoder.cs
- RtfToXamlLexer.cs
- DrawingContextDrawingContextWalker.cs
- ArraySubsetEnumerator.cs
- ObjectKeyFrameCollection.cs
- SiteMapDataSource.cs
- RichTextBox.cs
- WebPartManager.cs
- OrthographicCamera.cs
- FilterException.cs
- SerializableAttribute.cs
- ValuePattern.cs
- VectorConverter.cs
- RegexNode.cs
- XmlSubtreeReader.cs
- SqlClientMetaDataCollectionNames.cs
- CreateUserWizardStep.cs
- ParameterReplacerVisitor.cs