Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Documents / FixedTextContainer.cs / 2 / FixedTextContainer.cs
//---------------------------------------------------------------------------- //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // Description: // Implements the FixedTextContainer // // History: // 10/29/2003 - [....] ([....]) - Created. // //--------------------------------------------------------------------------- #pragma warning disable 1634, 1691 // To enable presharp warning disables (#pragma suppress) below. namespace System.Windows.Documents { using MS.Internal; using MS.Internal.Documents; using MS.Utility; using System.Windows; // DependencyID etc. using System.Windows.Controls; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Shapes; using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Windows.Threading; // Dispatcher //===================================================================== ////// FixedTextContainer is an implementaiton of TextContainer for Fixed Documents /// internal sealed class FixedTextContainer : ITextContainer { //------------------------------------------------------------------- // // Connstructors // //---------------------------------------------------------------------- #region Constructors ////// FixedTextContainer constructor that sets up the default backing store /// /// /// The object that will be parent of the top level content elements /// internal FixedTextContainer(DependencyObject parent) { Debug.Assert(parent != null && parent is FixedDocument); _parent = parent; _CreateEmptyContainer(); } #endregion Constructors //------------------------------------------------------------------- // // Public Methods // //---------------------------------------------------------------------- #region Public Methods // // This is readonly Text OM. All modification methods returns false // void ITextContainer.BeginChange() { } ////// void ITextContainer.BeginChangeNoUndo() { // We don't support undo, so follow the BeginChange codepath. ((ITextContainer)this).BeginChange(); } ////// /// void ITextContainer.EndChange() { ((ITextContainer)this).EndChange(false /* skipEvents */); } ////// void ITextContainer.EndChange(bool skipEvents) { } // Allocate a new ITextPointer at the specified offset. // Equivalent to this.Start.CreatePointer(offset), but does not // necessarily allocate this.Start. ITextPointer ITextContainer.CreatePointerAtOffset(int offset, LogicalDirection direction) { return ((ITextContainer)this).Start.CreatePointer(offset, direction); } // Not Implemented. ITextPointer ITextContainer.CreatePointerAtCharOffset(int charOffset, LogicalDirection direction) { throw new NotImplementedException(); } ITextPointer ITextContainer.CreateDynamicTextPointer(StaticTextPointer position, LogicalDirection direction) { return ((ITextPointer)position.Handle0).CreatePointer(direction); } StaticTextPointer ITextContainer.CreateStaticPointerAtOffset(int offset) { return new StaticTextPointer(this, ((ITextContainer)this).CreatePointerAtOffset(offset, LogicalDirection.Forward)); } TextPointerContext ITextContainer.GetPointerContext(StaticTextPointer pointer, LogicalDirection direction) { return ((ITextPointer)pointer.Handle0).GetPointerContext(direction); } int ITextContainer.GetOffsetToPosition(StaticTextPointer position1, StaticTextPointer position2) { return ((ITextPointer)position1.Handle0).GetOffsetToPosition((ITextPointer)position2.Handle0); } int ITextContainer.GetTextInRun(StaticTextPointer position, LogicalDirection direction, char[] textBuffer, int startIndex, int count) { return ((ITextPointer)position.Handle0).GetTextInRun(direction, textBuffer, startIndex, count); } object ITextContainer.GetAdjacentElement(StaticTextPointer position, LogicalDirection direction) { return ((ITextPointer)position.Handle0).GetAdjacentElement(direction); } DependencyObject ITextContainer.GetParent(StaticTextPointer position) { return null; } StaticTextPointer ITextContainer.CreatePointer(StaticTextPointer position, int offset) { return new StaticTextPointer(this, ((ITextPointer)position.Handle0).CreatePointer(offset)); } StaticTextPointer ITextContainer.GetNextContextPosition(StaticTextPointer position, LogicalDirection direction) { return new StaticTextPointer(this, ((ITextPointer)position.Handle0).GetNextContextPosition(direction)); } int ITextContainer.CompareTo(StaticTextPointer position1, StaticTextPointer position2) { return ((ITextPointer)position1.Handle0).CompareTo((ITextPointer)position2.Handle0); } int ITextContainer.CompareTo(StaticTextPointer position1, ITextPointer position2) { return ((ITextPointer)position1.Handle0).CompareTo(position2); } object ITextContainer.GetValue(StaticTextPointer position, DependencyProperty formattingProperty) { return ((ITextPointer)position.Handle0).GetValue(formattingProperty); } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //--------------------------------------------------------------------- #region Public Properties ////// Specifies whether or not the content of this TextContainer may be /// modified. /// ////// True if content may be modified, false otherwise. /// ////// This TextContainer implementation always returns true. /// bool ITextContainer.IsReadOnly { get { return true; } } ////// ITextPointer ITextContainer.Start { get { Debug.Assert(_start != null); return _start; } } ////// /// ITextPointer ITextContainer.End { get { Debug.Assert(_end != null); return _end; } } ////// /// Autoincremented counter of content changes in this TextContainer /// uint ITextContainer.Generation { get { // For read-only content, return some constant value. return 0; } } ////// Collection of highlights applied to TextContainer content. /// Highlights ITextContainer.Highlights { get { return this.Highlights; } } ////// DependencyObject ITextContainer.Parent { get { return _parent; } } // TextEditor owns setting and clearing this property inside its // ctor/OnDetach methods. ITextSelection ITextContainer.TextSelection { get { return this.TextSelection;} set { _textSelection = value; } } // Optional undo manager, always null for this ITextContainer. UndoManager ITextContainer.UndoManager { get { return null; } } ///// 0) { //Document end boundary node pageNumber = this.FixedDocument.PageCount - 1; } else { //Document start boundary node pageNumber = 0; } } else if (flowNode.Type == FlowNodeType.Virtual || flowNode.Type == FlowNodeType.Noop) { pageNumber = (int)flowNode.Cookie; } else if (fixedElement != null) { pageNumber = (int)fixedElement.PageIndex; } else { FixedPosition fixPos; bool res = FixedTextBuilder.GetFixedPosition(fixedTextPointer.FlowPosition, fixedTextPointer.LogicalDirection, out fixPos); Debug.Assert(res); if (res) { pageNumber = fixPos.Page; } } } } return pageNumber; } // Get the highlights, in Glyphs granularity, that covers this range internal void GetMultiHighlights(FixedTextPointer start, FixedTextPointer end, Dictionary highlights, FixedHighlightType t, Brush foregroundBrush, Brush backgroundBrush) { Debug.Assert(highlights != null); if (start.CompareTo(end) > 0) { // make sure start <= end FixedTextPointer temp = start; start = end; end = temp; } FixedSOMElement[] elements; //Start and end indices in selection for first and last FixedSOMElements respectively int startIndex = 0; int endIndex = 0; if (_GetFixedNodesForFlowRange(start, end, out elements, out startIndex, out endIndex)) { for(int i=0; i /// Collection of highlights applied to TextContainer content. /// internal Highlights Highlights { get { if (_highlights == null) { _highlights = new Highlights(this); } return _highlights; } } // TextSelection associated with this container. internal ITextSelection TextSelection { get { return _textSelection; } } #endregion Internal Properties //------------------------------------------------------------------- // // Private Methods // //---------------------------------------------------------------------- #region Private Methods //------------------------------------------------------------------- // Initilization //--------------------------------------------------------------------- private void _CreateEmptyContainer() { // new text builder with map _fixedTextBuilder = new FixedTextBuilder(this); // create initial TextPointer and container element _start = new FixedTextPointer(false, LogicalDirection.Backward, new FlowPosition(this, this.FixedTextBuilder.FixedFlowMap.FlowStartEdge, 1)); _end = new FixedTextPointer(false, LogicalDirection.Forward, new FlowPosition(this, this.FixedTextBuilder.FixedFlowMap.FlowEndEdge, 0)); _containerElement = new FixedElement(FixedElement.ElementType.Container, _start, _end, int.MaxValue); _start.FlowPosition.AttachElement(_containerElement); _end.FlowPosition.AttachElement(_containerElement); } internal void OnNewFlowElement(FixedElement parentElement, FixedElement.ElementType elementType, FlowPosition pStart, FlowPosition pEnd, Object source, int pageIndex) { FixedTextPointer eStart = new FixedTextPointer(false, LogicalDirection.Backward, pStart); FixedTextPointer eEnd = new FixedTextPointer(false, LogicalDirection.Forward, pEnd); FixedElement e = new FixedElement(elementType, eStart, eEnd, pageIndex); if (source != null) { e.Object = source; } // hook up logical tree parentElement.Append(e); // attach element to flownode for faster lookup later. pStart.AttachElement(e); pEnd.AttachElement(e); } //------------------------------------------------------------------- // TextContainer Element //---------------------------------------------------------------------- // given a TextPointer range, find out all fixed position included in this range and // offset into the begin and end fixed element private bool _GetFixedNodesForFlowRange(ITextPointer start, ITextPointer end, out FixedSOMElement[] elements, out int startIndex, out int endIndex) { Debug.Assert(start.CompareTo(end) <= 0); elements = null; startIndex = 0; endIndex = 0; if (start.CompareTo(end) == 0) { return false; } FixedTextPointer pStart = (FixedTextPointer)start; FixedTextPointer pEnd = (FixedTextPointer)end; return this.FixedTextBuilder.GetFixedNodesForFlowRange(pStart.FlowPosition, pEnd.FlowPosition, out elements, out startIndex, out endIndex); } //endofGetFixedNodes #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //---------------------------------------------------------------------- #region Private Fields /// /// Cache for document content provider /// private FixedDocument _fixedPanel; // the fixed document ////// Fixed To Flow Implemenation /// private FixedTextBuilder _fixedTextBuilder; // heuristics to build text stream from fixed document ////// Text OM /// private DependencyObject _parent; private FixedElement _containerElement; private FixedTextPointer _start; private FixedTextPointer _end; // Collection of highlights applied to TextContainer content. private Highlights _highlights; private ITextSelection _textSelection; // TextView associated with this TextContainer. private ITextView _textview; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
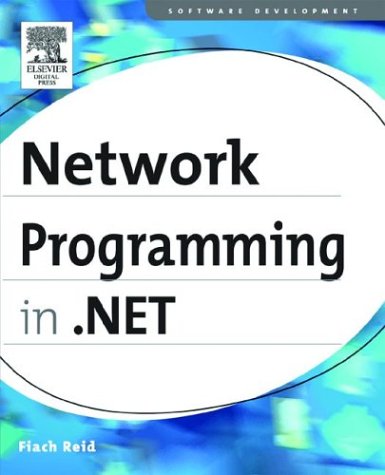
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListBox.cs
- GenericNameHandler.cs
- WebEventTraceProvider.cs
- ColumnResult.cs
- SendMessageContent.cs
- DoubleLinkListEnumerator.cs
- PerformanceCounterCategory.cs
- ChangeDirector.cs
- DomainUpDown.cs
- DrawingContextDrawingContextWalker.cs
- ChangesetResponse.cs
- BitmapImage.cs
- Stylesheet.cs
- UrlPath.cs
- UInt16Storage.cs
- TypeDescriptionProvider.cs
- SpotLight.cs
- ProfileSection.cs
- AnalyzedTree.cs
- DataGridViewLayoutData.cs
- UnknownBitmapEncoder.cs
- KeyProperty.cs
- MetadataItemSerializer.cs
- AuthenticationModuleElement.cs
- ProfileSettingsCollection.cs
- IImplicitResourceProvider.cs
- BaseCollection.cs
- BitmapSourceSafeMILHandle.cs
- DataColumnChangeEvent.cs
- TextParaLineResult.cs
- SqlDataSourceStatusEventArgs.cs
- SignedInfo.cs
- StringAnimationBase.cs
- DataGridViewCellValueEventArgs.cs
- LayoutTableCell.cs
- PlatformNotSupportedException.cs
- PolicyException.cs
- _ContextAwareResult.cs
- SafeMILHandle.cs
- SourceFilter.cs
- BindingGraph.cs
- OleDbDataReader.cs
- CommandExpr.cs
- DesignTimeType.cs
- RepeatButton.cs
- UnsafeNativeMethods.cs
- HtmlTitle.cs
- ProxyAttribute.cs
- BitmapEffect.cs
- WebPartConnectionsCloseVerb.cs
- PeerOutputChannel.cs
- Point3D.cs
- JavaScriptSerializer.cs
- _ConnectOverlappedAsyncResult.cs
- OdbcError.cs
- DataGridItemCollection.cs
- TreeIterator.cs
- ReturnType.cs
- XmlChildEnumerator.cs
- Tool.cs
- MediaElementAutomationPeer.cs
- KnownColorTable.cs
- PipelineComponent.cs
- TreeNodeStyleCollection.cs
- FloaterParagraph.cs
- EmptyControlCollection.cs
- ScrollViewer.cs
- EFDataModelProvider.cs
- MemberAssignment.cs
- EventDescriptorCollection.cs
- WpfXamlMember.cs
- DiscoveryServerProtocol.cs
- linebase.cs
- SamlSerializer.cs
- CaseInsensitiveHashCodeProvider.cs
- ConvertTextFrag.cs
- _LocalDataStore.cs
- PropertyChangedEventManager.cs
- StatusBarItem.cs
- LineServices.cs
- DataSourceView.cs
- HtmlFormAdapter.cs
- ParserOptions.cs
- SqlTopReducer.cs
- TextCompositionEventArgs.cs
- IndexerHelper.cs
- FocusTracker.cs
- CellConstantDomain.cs
- WebPartsPersonalizationAuthorization.cs
- UInt32Converter.cs
- ToolBarOverflowPanel.cs
- AsyncPostBackErrorEventArgs.cs
- SerTrace.cs
- SystemColors.cs
- SqlBulkCopy.cs
- PiiTraceSource.cs
- WebPartMinimizeVerb.cs
- MaskedTextBoxTextEditor.cs
- IUnknownConstantAttribute.cs
- PriorityBinding.cs