Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / ObjectToken / RegistryDataKey.cs / 1 / RegistryDataKey.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // Encapsulation of the Registry Key. // // History: // 7/1/2004 [....] //--------------------------------------------------------------------------- using Microsoft.Win32; using System; using System.IO; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Security; using System.Security.AccessControl; using System.Text; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. using RegistryEntry = System.Collections.Generic.KeyValuePair; namespace System.Speech.Internal.ObjectTokens { /// /// Summary description for SpRegDataKey. /// #if VSCOMPILE [DebuggerDisplay ("{Name}")] #endif internal class RegistryDataKey : ISpDataKey, IEnumerable, IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors protected RegistryDataKey (string keyId, RegistryKey hkey) { // Set the name _sKeyId = keyId; _hkey = hkey; } internal static RegistryDataKey Create (string tokenId, bool fCreateIfNotExist) { // Sanity check if (string.IsNullOrEmpty (tokenId)) { return null; } // If the last character is a '\', get rid of it string id = (string) tokenId.Clone (); // Create the registry entry key RegistryKey hkey = CreateKey (tokenId, fCreateIfNotExist, out id); if (hkey == null) { return null; } return new RegistryDataKey (id, hkey); } internal static RegistryDataKey Create (string keyId, RegistryKey hkey) { return new RegistryDataKey (keyId, hkey); } internal static RegistryKey CreateKey (string tokenId, bool fCreateIfNotExist, out string id) { // If the last character is a '\', get rid of it id = (string) tokenId.Clone (); if (string.IsNullOrEmpty (tokenId)) { return null; } int cLen = id.Length; if (cLen > 0 && id [cLen - 1] == '\\') { id = id.Substring (0, cLen - 1); } // Create the registry entry key return RegistryPathToHkey (tokenId, fCreateIfNotExist, false); } /// /// Needed by IEnumerable!!! /// public void Dispose () { Dispose (true); } ~RegistryDataKey () { Dispose (false); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region internal Methods #region ISpDataKey Implementation // ISpDataKey Methods ////// Writes the specified binary data to the registry. /// /// /// /// ///[PreserveSig] public int SetData ([MarshalAs (UnmanagedType.LPWStr)] string valueName, UInt32 cbData, [MarshalAs (UnmanagedType.LPArray, SizeParamIndex = 1)] Byte [] data) { int error = -1; if (_rwKey || MakeKeyWrite ()) { try { _hkey.SetValue (valueName, data, RegistryValueKind.Binary); error = 0; } catch (ArgumentException) { error = (int) SAPIErrorCodes.SPERR_NOT_FOUND; } catch (Exception e) { if (!CanSwallowRegistryException (e)) { throw; } error = -1; } } return error; } /// /// Reads the specified binary data from the registry. /// /// /// /// ///[PreserveSig] public int GetData ([MarshalAs (UnmanagedType.LPWStr)] string valueName, ref UInt32 pcbData, [MarshalAs (UnmanagedType.LPArray, SizeParamIndex = 1), Out] Byte [] data) { int error = -1; if (data != null) { object result = null; error = GetRegistryValue (valueName, ref result); if (error == 0) { byte [] dataResult = result as byte []; if (result == null || dataResult == null) { error = (int) SAPIErrorCodes.SPERR_NOT_FOUND; } else if (dataResult.Length > (int) pcbData) { error = (int) SAPIErrorCodes.ERROR_MORE_DATA; } else { pcbData = (uint) dataResult.Length; Array.Copy (dataResult, data, dataResult.Length); error = 0; } } } return error; } /// /// Writes the specified string value from the registry. If valueName /// is NULL then the default value of the registry key is read. /// /// /// ///[PreserveSig] public int SetStringValue ([MarshalAs (UnmanagedType.LPWStr)] string valueName, [MarshalAs (UnmanagedType.LPWStr)] string value) { int error = -1; if (_rwKey || MakeKeyWrite ()) { error = SetString (valueName, value) ? 0 : -1; } return error; } /// /// Reads the specified string value to the registry. If valueName is /// NULL then the default value of the registy key is read. /// /// /// ///[PreserveSig] public int GetStringValue ([MarshalAs (UnmanagedType.LPWStr)] string valueName, [MarshalAs (UnmanagedType.LPWStr)] out string value) { value = null; int error = 0; if (!TryGetString (valueName, out value)) { error = (int) SAPIErrorCodes.SPERR_NOT_FOUND; } return error; } /// /// Writes the specified DWORD to the registry. /// /// /// ///[PreserveSig] public int SetDWORD ([MarshalAs (UnmanagedType.LPWStr)] string valueName, UInt32 value) { int error = -1; if (_rwKey || MakeKeyWrite ()) { try { _hkey.SetValue (valueName, unchecked ((int) value), RegistryValueKind.DWord); error = 0; } catch (Exception e) { if (!CanSwallowRegistryException (e)) { throw; } error = -1; } } return error; } /// /// Reads the specified DWORD from the registry. /// /// /// ///[PreserveSig] public int GetDWORD ([MarshalAs (UnmanagedType.LPWStr)] string valueName, ref UInt32 pdwValue) { int error = 0; if (!TryGetDWORD (valueName, ref pdwValue)) { error = (int) SAPIErrorCodes.SPERR_NOT_FOUND; } return error; } /// /// Opens a sub-key and returns a new object which supports SpDataKey /// for the specified sub-key. /// /// /// ///[PreserveSig] public int OpenKey ([MarshalAs (UnmanagedType.LPWStr)] string subKeyName, out ISpDataKey ppSubKey) { int error = 0; ppSubKey = null; RegistryDataKey key; if (TryOpenKey (subKeyName, out key)) { ppSubKey = (ISpDataKey) key; } else { error = (int) SAPIErrorCodes.SPERR_NOT_FOUND; } return error; } /// /// Creates a sub-key and returns a new object which supports SpDataKey /// for the specified sub-key. /// /// /// ///[PreserveSig] public int CreateKey ([MarshalAs (UnmanagedType.LPWStr)] string subKeyName, out ISpDataKey ppSubKey) { int error = 0; ppSubKey = null; try { RegistryDataKey key = CreateKey (subKeyName); ppSubKey = (ISpDataKey) key; } #pragma warning disable 56500 // Transferring exceptions to the native world catch { error = -1; } return error; } #pragma warning restore 56500 /// /// Deletes the specified key. /// /// ///[PreserveSig] public int DeleteKey ([MarshalAs (UnmanagedType.LPWStr)] string subKeyName) { int error = -1; try { if (_rwKey || MakeKeyWrite ()) { _hkey.DeleteSubKey (subKeyName); error = 0; } } catch (ArgumentException) { error = (int) SAPIErrorCodes.SPERR_NOT_FOUND; } catch (Exception e) { if (!CanSwallowRegistryException (e)) { throw; } error = -1; } return error; } /// /// Deletes the specified value from the key. /// /// ///[PreserveSig] public int DeleteValue ([MarshalAs (UnmanagedType.LPWStr)] string valueName) { int error = -1; if (_rwKey || MakeKeyWrite ()) { try { _hkey.DeleteValue (valueName, true); } catch (ArgumentException) { error = (int) SAPIErrorCodes.SPERR_NOT_FOUND; } catch (Exception e) { if (!CanSwallowRegistryException (e)) { throw; } error = -1; } } return error; } /// /// Retrieve a key name by index /// /// /// ///[PreserveSig] public int EnumKeys (UInt32 index, [MarshalAs (UnmanagedType.LPWStr)] out string ppszSubKeyName) { int error = 0; ppszSubKeyName = null; try { ppszSubKeyName = _hkey.GetSubKeyNames () [index]; } catch (Exception e) { if (!CanSwallowRegistryException (e)) { throw; } error = -1; } return error; } /// /// Retreives a key value by index /// /// /// ///[PreserveSig] public int EnumValues (UInt32 index, [MarshalAs (UnmanagedType.LPWStr)] out string valueName) { int error = 0; valueName = null; try { valueName = _hkey.GetValueNames () [index]; } catch (Exception e) { if (!CanSwallowRegistryException (e)) { throw; } error = -1; } return error; } #endregion /// /// Full path and name for the key /// internal string Id { get { return _sKeyId; } } ////// Key Name (no path) /// internal string Name { get { int iPosSlash = _sKeyId.LastIndexOf ('\\'); return _sKeyId.Substring (iPosSlash + 1); } } ////// Writes the specified string value from the registry. If valueName /// is NULL then the default value of the registry key is read. /// /// /// internal bool SetString (string valueName, string sValue) { bool result = false; try { _hkey.SetValue (valueName, sValue, RegistryValueKind.String); result = true; } catch (Exception e) { if (!CanSwallowRegistryException (e)) { throw; } result = false; } return result; } // Disable parameter validation check #pragma warning disable 56507 ////// Reads the specified string value to the registry. If valueName is /// NULL then the default value of the registy key is read. /// /// /// ///internal bool TryGetString (string valueName, out string value) { bool success = false; object result = null; value = null; if (GetRegistryValue (valueName, ref result) == 0) { value = result as string; string [] aValues = result as string []; if (result == null && value == null && aValues == null) { return false; } if (aValues != null) { StringBuilder sb = new StringBuilder (); foreach (string s in aValues) { sb.Append (s); } value = sb.ToString (); } success = true; } return success; } #pragma warning restore 56507 /// /// Opens a sub-key and returns a new object which supports SpDataKey /// for the specified sub-key. /// /// ///internal bool HasValue (string valueName) { object value = null; return GetRegistryValue (valueName, ref value) == 0 && value != null; } /// /// Reads the specified DWORD from the registry. /// /// /// ///internal bool TryGetDWORD (string valueName, ref UInt32 value) { object result = value; bool success = false; if (GetRegistryValue (valueName, ref result) == 0) { if (result != null && result is int) { value = unchecked ((UInt32) (int) result); success = true; } } return success; } /// /// Opens a sub-key and returns a new object which supports SpDataKey /// for the specified sub-key. /// /// ///internal RegistryDataKey OpenKey (string keyName) { Helpers.ThrowIfEmptyOrNull (keyName, "keyName"); return RegistryDataKey.Create (_sKeyId + @"\" + keyName, false); } /// /// Creates a sub-key and returns a new object which supports SpDataKey /// for the specified sub-key. /// /// ///internal RegistryDataKey CreateKey (string keyName) { Helpers.ThrowIfEmptyOrNull (keyName, "keyName"); return RegistryDataKey.Create (_sKeyId + @"\" + keyName, true); } #if VSCOMPILE /// /// Delete a registry key based on a registry path (string) /// /// /// internal static void DeleteRegistryPath (string sRegPath, string keyName) { // We may need to parse sRegPath for the root and the sub key ... string dstrRegPathRoot; if (keyName == null) { // To best understand the parsing, here's an example: // // RegistryKey\Key1\Key2\Key3 // sRegPath ^ // sLastSlash ^ // // sLastSlash - sRegPath = 14; // dstrRegPathRoot = "RegistryKey\Key1\Key2" // // keyName = "Key3" // To find the root, and the keyname, we first need to find // the last slash int iLastSlash = sRegPath.LastIndexOf ('\\'); int cRegPath = sRegPath.Length; if (iLastSlash < 0 || iLastSlash == cRegPath - 1) { throw new FormatException (SR.Get (SRID.InvalidTokenId)); } keyName = sRegPath.Substring (iLastSlash + 1, cRegPath - (iLastSlash + 1)); dstrRegPathRoot = sRegPath.Substring (0, iLastSlash); } else { // No need to parse, the caller passed both in ... dstrRegPathRoot = sRegPath; } // Try to convert the regpath into an actual key RegistryKey hkeyRoot = RegistryPathToHkey (dstrRegPathRoot, false, true); if (hkeyRoot == null) { throw new FormatException (SR.Get (SRID.InvalidTokenId)); } hkeyRoot.DeleteSubKeyTree (keyName); } #endif ////// returns the name for all the values in this registry entry /// ///internal string [] GetValueNames () { return _hkey.GetValueNames (); } #region IEnumerable implementation IEnumerator IEnumerable .GetEnumerator () { string [] keys = null; try { keys = _hkey.GetSubKeyNames (); } catch (Exception e) { if (!CanSwallowRegistryException (e)) { throw; } } if (keys != null) { foreach (string keyName in keys) { yield return RegistryDataKey.Create (_sKeyId + @"\" + keyName, false); } } } IEnumerator IEnumerable.GetEnumerator () { return ((IEnumerable ) this).GetEnumerator (); } #endregion #endregion //******************************************************************* // // Protected Methods // //******************************************************************* #region Protected Methods /// /// TODOC /// /// protected virtual void Dispose (bool disposing) { if (disposing && _hkey != null) { ((IDisposable) _hkey).Dispose (); _hkey = null; } // Suppress finalization of this disposed instance. if (disposing) { GC.SuppressFinalize (this); } } #endregion //******************************************************************* // // Private Methods // //******************************************************************* #region Private Methods ////// Initialize the token for the specified static token. For example: /// /// sCategoryId = HKEY...\Recognizers /// tokenId = HKEY...\Recognizer\Tokens\MSASR English /// private static RegistryKey RegistryPathToHkey (RegistryKey hkeyRoot, string sRegPath, bool fCreateIfNotExist, bool openReadWrite) { RegistryKey hkey = null; int iPosSlash = sRegPath.IndexOf ('\\'); int cRegPath = sRegPath.Length; if (iPosSlash < 0 || iPosSlash >= cRegPath) { return null; } sRegPath = sRegPath.Substring (iPosSlash + 1, cRegPath - (iPosSlash + 1)); // Try to create/open the key with read / write access try { if (fCreateIfNotExist) { hkey = hkeyRoot.CreateSubKey (sRegPath); } else { hkey = hkeyRoot.OpenSubKey (sRegPath, openReadWrite); } } catch (Exception e) { if (!CanSwallowRegistryException (e)) { throw; } return null; } return hkey; } private static RegistryKey RegistryPathToHkey (string sRegPath, bool fCreateIfNotExist, bool openReadWrite) { RegistryKey hkey = null; RegistryKey [] registryClasses = new RegistryKey [] { Registry.ClassesRoot, Registry.LocalMachine, Registry.CurrentUser, #if !_WIN32_WCE Registry.CurrentConfig #endif // _WIN32_WCE }; // Loop thru the different keys we know about for (int i = 0; hkey == null && i < registryClasses.Length; i++) { // If we've foudn the match string s = registryClasses [i].ToString (); if (sRegPath.IndexOf (s, StringComparison.Ordinal) == 0) { hkey = RegistryPathToHkey (registryClasses [i], sRegPath, fCreateIfNotExist, openReadWrite); } } return hkey; } ////// Try to open a sub-key and returns a new object which supports SpDataKey /// for the specified sub-key. /// /// /// ///internal bool TryOpenKey (string keyName, out RegistryDataKey key) { key = null; if (string.IsNullOrEmpty (keyName)) { return false; } string keyId = _sKeyId + @"\" + keyName; // If the last character is a '\', get rid of it int cLen = keyId.Length; if (cLen > 0 && keyId [cLen - 1] == '\\') { keyId = keyId.Substring (0, cLen - 1); } // Convert the token id into a datakey // Convert the string to an hkey RegistryKey hkey = RegistryPathToHkey (keyId, false, false); // If we got the data key, assign the category and token id if (hkey == null) { return false; } key = new RegistryDataKey (keyId, hkey); return true; } private int GetRegistryValue (string valueName, ref object value) { int error = -1; try { value = _hkey.GetValue (valueName); error = 0; } catch (Exception e) { if (!CanSwallowRegistryException (e)) { throw; } error = -1; } return error; } /// /// Checks for one of the expected exception on can't read a registry entry /// /// ///static private bool CanSwallowRegistryException (Exception e) { return e is ArgumentException || e is IOException || e is SecurityException || e is UnauthorizedAccessException || e is IndexOutOfRangeException; } #endregion //******************************************************************** // // Internal Fields // //******************************************************************** #region Internal Fields internal string _sKeyId; internal RegistryKey _hkey; internal bool _rwKey; #endregion //******************************************************************* // // Private Method // //******************************************************************** #region Private Method private bool MakeKeyWrite () { bool ok = false; RegistryKey key = RegistryPathToHkey (Id, false, true); if (key != null) { _hkey.Close (); _hkey = key; _rwKey = true; ok = true; } return ok; } #endregion //******************************************************************* // // Private Types // //******************************************************************* #region private Types internal enum SAPIErrorCodes { STG_E_FILENOTFOUND = -2147287038, // 0x80030002 SPERR_UNSUPPORTED_FORMAT = -2147201021, // 0x80045003 SPERR_DEVICE_BUSY = -2147201018, // 0x80045006 SPERR_DEVICE_NOT_SUPPORTED = -2147201017, // 0x80045007 SPERR_DEVICE_NOT_ENABLED = -2147201016, // 0x80045008 SPERR_NO_DRIVER = -2147201015, // 0x80045009 SPERR_TOO_MANY_GRAMMARS = -2147200990, // 0x80045022 SPERR_INVALID_IMPORT = -2147200988, // 0x80045024 SPERR_AUDIO_BUFFER_OVERFLOW = -2147200977, // 0x8004502F SPERR_NO_AUDIO_DATA = -2147200976, // 0x80045030 SPERR_NO_MORE_ITEMS = -2147200967, // 0x80045039 SPERR_NOT_FOUND = -2147200966, // 0x8004503A SPERR_GENERIC_MMSYS_ERROR = -2147200964, // 0x8004503C SPERR_NOT_TOPLEVEL_RULE = -2147200940, // 0x80045054 SPERR_NOT_ACTIVE_SESSION = -2147200925, // 0x80045063 SPERR_SML_GENERATION_FAIL = -2147200921, // 0x80045067 SPERR_SHARED_ENGINE_DISABLED = -2147200906, // 0x80045076 SPERR_RECOGNIZER_NOT_FOUND = -2147200905, // 0x80045077 SPERR_AUDIO_NOT_FOUND = -2147200904, // 0x80045078 S_OK = 0, // 0x00000000 S_FALSE = 1, // 0x00000001 SP_NO_RULES_TO_ACTIVATE = 282747, // 0x0004507B ERROR_MORE_DATA = 0x50EA, } #endregion } #region SAPI Interface [ComImport, Guid ("14056581-E16C-11D2-BB90-00C04F8EE6C0"), InterfaceType (ComInterfaceType.InterfaceIsIUnknown)] internal interface ISpDataKey { // ISpDataKey Methods [PreserveSig] int SetData ([MarshalAs (UnmanagedType.LPWStr)] string valueName, UInt32 cbData, [MarshalAs (UnmanagedType.LPArray, SizeParamIndex = 1)] Byte [] data); [PreserveSig] int GetData ([MarshalAs (UnmanagedType.LPWStr)] string valueName, ref UInt32 pcbData, [MarshalAs (UnmanagedType.LPArray, SizeParamIndex = 1), Out] Byte [] data); [PreserveSig] int SetStringValue ([MarshalAs (UnmanagedType.LPWStr)] string valueName, [MarshalAs (UnmanagedType.LPWStr)] string value); [PreserveSig] int GetStringValue ([MarshalAs (UnmanagedType.LPWStr)] string valueName, [MarshalAs (UnmanagedType.LPWStr)] out string value); [PreserveSig] int SetDWORD ([MarshalAs (UnmanagedType.LPWStr)] string valueName, UInt32 dwValue); [PreserveSig] int GetDWORD ([MarshalAs (UnmanagedType.LPWStr)] string valueName, ref UInt32 pdwValue); [PreserveSig] int OpenKey ([MarshalAs (UnmanagedType.LPWStr)] string subKeyName, out ISpDataKey ppSubKey); [PreserveSig] int CreateKey ([MarshalAs (UnmanagedType.LPWStr)] string subKey, out ISpDataKey ppSubKey); [PreserveSig] int DeleteKey ([MarshalAs (UnmanagedType.LPWStr)] string subKey); [PreserveSig] int DeleteValue ([MarshalAs (UnmanagedType.LPWStr)] string valueName); [PreserveSig] int EnumKeys (UInt32 index, [MarshalAs (UnmanagedType.LPWStr)] out string ppszSubKeyName); [PreserveSig] int EnumValues (UInt32 index, [MarshalAs (UnmanagedType.LPWStr)] out string valueName); } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
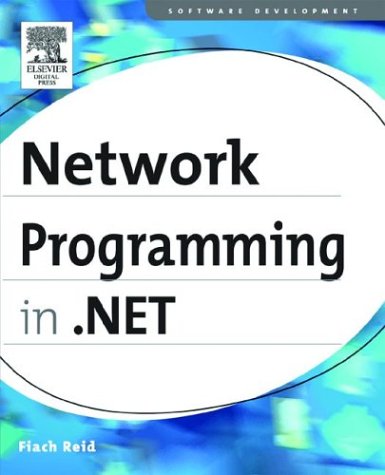
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewTextBoxCell.cs
- ValidationEventArgs.cs
- TaiwanLunisolarCalendar.cs
- PagedDataSource.cs
- TraceHandlerErrorFormatter.cs
- ResourceReferenceKeyNotFoundException.cs
- Image.cs
- DataControlFieldCollection.cs
- IgnoreFileBuildProvider.cs
- ProgressChangedEventArgs.cs
- AutomationPatternInfo.cs
- OleDbException.cs
- HandleValueEditor.cs
- DataGridViewSelectedColumnCollection.cs
- FrameworkContextData.cs
- NativeMethods.cs
- AccessDataSourceView.cs
- CustomAttributeSerializer.cs
- HttpAsyncResult.cs
- XmlFormatExtensionPrefixAttribute.cs
- IndicFontClient.cs
- SafeFileMapViewHandle.cs
- UrlMappingCollection.cs
- DataGrid.cs
- SiteMap.cs
- HttpHostedTransportConfiguration.cs
- UrlMappingsSection.cs
- ImageInfo.cs
- DelegatingTypeDescriptionProvider.cs
- UpdatePanelTriggerCollection.cs
- EntitySetBaseCollection.cs
- BitFlagsGenerator.cs
- ISFTagAndGuidCache.cs
- XmlSchemaAnnotated.cs
- Random.cs
- AttributeData.cs
- RewritingProcessor.cs
- RayHitTestParameters.cs
- StringPropertyBuilder.cs
- ThemeInfoAttribute.cs
- Propagator.cs
- HashHelpers.cs
- DropTarget.cs
- FormatterServices.cs
- StringKeyFrameCollection.cs
- GroupQuery.cs
- XmlSerializerFactory.cs
- X509UI.cs
- LockCookie.cs
- Point3DAnimationBase.cs
- ConstantSlot.cs
- ReadOnlyDictionary.cs
- SafeCoTaskMem.cs
- VirtualStackFrame.cs
- ExpressionPrefixAttribute.cs
- Point.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- WebPartConnectionsCancelEventArgs.cs
- WindowsScroll.cs
- InputQueue.cs
- odbcmetadatafactory.cs
- CommandSet.cs
- XomlCompilerParameters.cs
- OpacityConverter.cs
- TextTreeExtractElementUndoUnit.cs
- EntityDataSourceChangingEventArgs.cs
- AnnotationObservableCollection.cs
- InvalidateEvent.cs
- ProcessRequestArgs.cs
- Pen.cs
- EditorZone.cs
- DataControlCommands.cs
- CompositionTarget.cs
- CodeTypeDeclarationCollection.cs
- ButtonAutomationPeer.cs
- SQLGuidStorage.cs
- GestureRecognizer.cs
- Simplifier.cs
- Encoding.cs
- SeekableReadStream.cs
- EmbeddedMailObject.cs
- UserInitiatedNavigationPermission.cs
- SessionEndingEventArgs.cs
- TextBox.cs
- CustomBinding.cs
- PropertyDescriptorGridEntry.cs
- ColorConvertedBitmap.cs
- ScriptMethodAttribute.cs
- FactoryRecord.cs
- ZipIOFileItemStream.cs
- Point3DCollectionValueSerializer.cs
- MailAddressCollection.cs
- AddInProcess.cs
- DelimitedListTraceListener.cs
- processwaithandle.cs
- NameValuePair.cs
- PersistenceProviderDirectory.cs
- ColumnCollectionEditor.cs
- Triplet.cs
- ListViewInsertedEventArgs.cs