Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Synthesis / WaveHeader.cs / 1 / WaveHeader.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // This class defines the header used to identify a waveform-audio // buffer. // // with the CLR 607 // History: // 2/1/2005 [....] Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Speech.Synthesis.TtsEngine; namespace System.Speech.Internal.Synthesis { ////// /// internal sealed class WaveHeader : IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// Initialize an instance of a byte array. /// /// ///MMSYSERR.NOERROR if successful internal WaveHeader (byte [] buffer) { _dwBufferLength = buffer.Length; _gcHandle = GCHandle.Alloc (buffer, GCHandleType.Pinned); } ////// Frees any memory allocated for the buffer. /// ~WaveHeader () { Dispose (false); } ////// TODOC /// public void Dispose () { Dispose (true); GC.SuppressFinalize (this); } ////// Frees any memory allocated for the buffer. /// private void Dispose (bool disposing) { if (disposing) { ReleaseData (); if (_gcHandleWaveHdr.IsAllocated) { _gcHandleWaveHdr.Free (); } } } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods internal void ReleaseData () { if (_gcHandle.IsAllocated) { _gcHandle.Free (); } } #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Properties internal GCHandle WAVEHDR { get { if (!_gcHandleWaveHdr.IsAllocated) { _waveHdr.lpData = _gcHandle.AddrOfPinnedObject (); _waveHdr.dwBufferLength = (uint) _dwBufferLength; _waveHdr.dwBytesRecorded = 0; _waveHdr.dwUser = 0; _waveHdr.dwFlags = 0; _waveHdr.dwLoops = 0; _waveHdr.lpNext = IntPtr.Zero; _gcHandleWaveHdr = GCHandle.Alloc (_waveHdr, GCHandleType.Pinned); } return _gcHandleWaveHdr; } } internal int SizeHDR { get { return Marshal.SizeOf (_waveHdr); } } #endregion //******************************************************************* // // Internal Fields // //******************************************************************** #region Internal Fields ////// Used by dwFlags in WaveHeader /// Set by the device driver to indicate that it is finished with the buffer /// and is returning it to the application. /// internal const int WHDR_DONE = 0x00000001; ////// Used by dwFlags in WaveHeader /// Set by Windows to indicate that the buffer has been prepared with the /// waveInPrepareHeader or waveOutPrepareHeader function. /// internal const int WHDR_PREPARED = 0x00000002; ////// Used by dwFlags in WaveHeader /// This buffer is the first buffer in a loop. This flag is used only with /// output buffers. /// internal const int WHDR_BEGINLOOP = 0x00000004; ////// Used by dwFlags in WaveHeader /// This buffer is the last buffer in a loop. This flag is used only with /// output buffers. /// internal const int WHDR_ENDLOOP = 0x00000008; ////// Used by dwFlags in WaveHeader /// Set by Windows to indicate that the buffer is queued for playback. /// internal const int WHDR_INQUEUE = 0x00000010; ////// Set in WaveFormat.wFormatTag to specify PCM data. /// internal const int WAVE_FORMAT_PCM = 1; #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region private Fields ////// Long pointer to the address of the waveform buffer. This buffer must /// be block-aligned according to the nBlockAlign member of the /// WaveFormat structure used to open the device. /// private GCHandle _gcHandle = new GCHandle (); private GCHandle _gcHandleWaveHdr = new GCHandle (); private WAVEHDR _waveHdr = new WAVEHDR (); ////// Specifies the length, in bytes, of the buffer. /// internal int _dwBufferLength; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
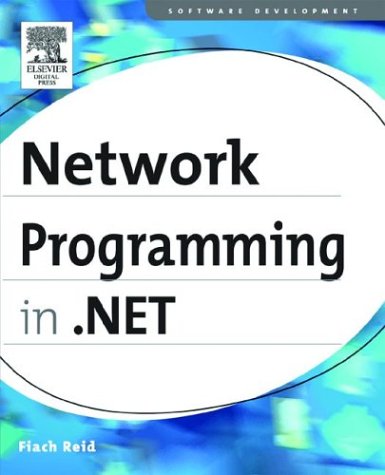
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FontNamesConverter.cs
- QueuePropertyVariants.cs
- LookupTables.cs
- ContentControl.cs
- AxisAngleRotation3D.cs
- RichTextBoxAutomationPeer.cs
- XamlStream.cs
- HyperLinkField.cs
- BoundColumn.cs
- OptimalBreakSession.cs
- ObjectStateManager.cs
- DependencyPropertyConverter.cs
- Win32Exception.cs
- PlatformCulture.cs
- _LocalDataStore.cs
- JsonStringDataContract.cs
- ComboBoxItem.cs
- SelectionBorderGlyph.cs
- BindingContext.cs
- DrawingCollection.cs
- RevocationPoint.cs
- ParagraphResult.cs
- RowSpanVector.cs
- NegotiateStream.cs
- ContainerControl.cs
- WebPartMenu.cs
- GuidTagList.cs
- VsPropertyGrid.cs
- InputReportEventArgs.cs
- Filter.cs
- GenericRootAutomationPeer.cs
- DataGridViewMethods.cs
- DataGridViewCellValueEventArgs.cs
- XmlSiteMapProvider.cs
- ObjectQuery_EntitySqlExtensions.cs
- columnmapkeybuilder.cs
- CacheVirtualItemsEvent.cs
- ResourcePart.cs
- FilterableAttribute.cs
- ListItemConverter.cs
- ConnectionManagementElement.cs
- RenderTargetBitmap.cs
- Converter.cs
- ManipulationLogic.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- GlyphCache.cs
- XmlSchemaSimpleContentExtension.cs
- SequenceDesigner.cs
- HMACMD5.cs
- KeyPullup.cs
- SmtpAuthenticationManager.cs
- ThreadStateException.cs
- GraphicsState.cs
- CancellationToken.cs
- ScrollBarRenderer.cs
- TemplateBuilder.cs
- BrowserDefinitionCollection.cs
- BrowserDefinitionCollection.cs
- ProcessThreadCollection.cs
- AddressingProperty.cs
- MessageDirection.cs
- DataException.cs
- CodeExporter.cs
- UpdatePanelTrigger.cs
- TearOffProxy.cs
- TextWriterEngine.cs
- FunctionNode.cs
- IsolatedStorageFile.cs
- ProcessRequestArgs.cs
- TemplateColumn.cs
- Normalization.cs
- TextOptions.cs
- BindingGroup.cs
- ButtonBaseAdapter.cs
- Column.cs
- ToolStripHighContrastRenderer.cs
- SqlReferenceCollection.cs
- XmlSerializationReader.cs
- RecipientInfo.cs
- SmiContext.cs
- MultiSelectRootGridEntry.cs
- AssertFilter.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- RightsManagementEncryptedStream.cs
- TextBoxAutoCompleteSourceConverter.cs
- WhileDesigner.cs
- GeneralTransform2DTo3DTo2D.cs
- CompositionTarget.cs
- ObjectManager.cs
- XmlDataCollection.cs
- objectquery_tresulttype.cs
- StringAnimationUsingKeyFrames.cs
- SystemTcpStatistics.cs
- FormatException.cs
- SqlTopReducer.cs
- UserValidatedEventArgs.cs
- SerializerWriterEventHandlers.cs
- DataGridColumnHeader.cs
- Pair.cs
- Header.cs