Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / Diagnostics / TraceSource.cs / 1 / TraceSource.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Threading; using System.Configuration; using System.Security; using System.Security.Permissions; namespace System.Diagnostics { public class TraceSource { private static Listtracesources = new List (); private readonly TraceEventCache manager = new TraceEventCache(); private SourceSwitch internalSwitch; private TraceListenerCollection listeners; private StringDictionary attributes; private SourceLevels switchLevel; private string sourceName; internal bool _initCalled = false; // Whether we've called Initialize already. public TraceSource(string name) : this(name, SourceLevels.Off) { } public TraceSource(string name, SourceLevels defaultLevel) { if (name == null) throw new ArgumentNullException("name"); if (name.Length == 0) throw new ArgumentException("name"); sourceName = name; switchLevel = defaultLevel; // Delay load config to avoid perf (and working set) issues in retail // Add a weakreference to this source lock(tracesources) { tracesources.Add(new WeakReference(this)); } } private void Initialize() { if (!_initCalled) { lock(this) { if (_initCalled) return; SourceElementsCollection sourceElements = DiagnosticsConfiguration.Sources; if (sourceElements != null) { SourceElement sourceElement = sourceElements[sourceName]; if (sourceElement != null) { if (!String.IsNullOrEmpty(sourceElement.SwitchName)) { CreateSwitch(sourceElement.SwitchType, sourceElement.SwitchName); } else { CreateSwitch(sourceElement.SwitchType, sourceName); if (!String.IsNullOrEmpty(sourceElement.SwitchValue)) internalSwitch.Level = (SourceLevels) Enum.Parse(typeof(SourceLevels), sourceElement.SwitchValue); } listeners = sourceElement.Listeners.GetRuntimeObject(); attributes = new StringDictionary(); TraceUtils.VerifyAttributes(sourceElement.Attributes, GetSupportedAttributes(), this); attributes.contents = sourceElement.Attributes; } else NoConfigInit(); } else NoConfigInit(); _initCalled = true; } } } private void NoConfigInit() { internalSwitch = new SourceSwitch(sourceName, switchLevel.ToString()); listeners = new TraceListenerCollection(); listeners.Add(new DefaultTraceListener()); attributes = null; } [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.UnmanagedCode)] public void Close() { // No need to call Initialize() if (listeners != null) { // Use global lock lock (TraceInternal.critSec) { foreach (TraceListener listener in listeners) { listener.Close(); } } } } public void Flush() { // No need to call Initialize() if (listeners != null) { if (TraceInternal.UseGlobalLock) { lock (TraceInternal.critSec) { foreach (TraceListener listener in listeners) { listener.Flush(); } } } else { foreach (TraceListener listener in listeners) { if (!listener.IsThreadSafe) { lock (listener) { listener.Flush(); } } else { listener.Flush(); } } } } } virtual protected internal string[] GetSupportedAttributes() { return null; } internal static void RefreshAll() { lock (tracesources) { for (int i=0; i
Link Menu
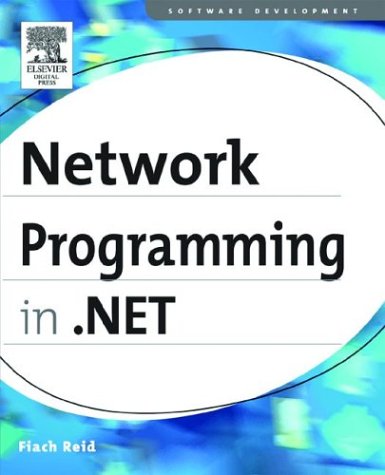
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlCachedBuffer.cs
- ContextDataSource.cs
- CssTextWriter.cs
- TaskFormBase.cs
- ListViewItem.cs
- PropertyInformation.cs
- ContainerParaClient.cs
- DataTable.cs
- QuaternionIndependentAnimationStorage.cs
- StreamGeometry.cs
- SQLUtility.cs
- CodeSubDirectoriesCollection.cs
- ParallelTimeline.cs
- XmlQueryRuntime.cs
- StateWorkerRequest.cs
- DiscreteKeyFrames.cs
- ProxyElement.cs
- TrackPoint.cs
- Part.cs
- CharEnumerator.cs
- HelpKeywordAttribute.cs
- EmptyCollection.cs
- SmtpCommands.cs
- FormattedTextSymbols.cs
- Form.cs
- ToolStripDropDown.cs
- Propagator.Evaluator.cs
- Item.cs
- BitmapEffectDrawingContextWalker.cs
- WindowShowOrOpenTracker.cs
- Version.cs
- DataExpression.cs
- HttpCapabilitiesBase.cs
- BaseTypeViewSchema.cs
- ResponseBodyWriter.cs
- DiscoveryMessageSequenceGenerator.cs
- Separator.cs
- Visual.cs
- ListManagerBindingsCollection.cs
- InvalidOleVariantTypeException.cs
- SelectionRangeConverter.cs
- LinkUtilities.cs
- DataGridViewAutoSizeModeEventArgs.cs
- SchemaTableColumn.cs
- BindingBase.cs
- TaiwanLunisolarCalendar.cs
- StringAnimationBase.cs
- DockingAttribute.cs
- HandleCollector.cs
- DbConnectionStringBuilder.cs
- AndMessageFilter.cs
- SoapMessage.cs
- UserControl.cs
- CategoryNameCollection.cs
- TagPrefixAttribute.cs
- PointAnimationUsingPath.cs
- Model3DCollection.cs
- NavigateEvent.cs
- NgenServicingAttributes.cs
- ArraySubsetEnumerator.cs
- UrlMapping.cs
- ManifestSignedXml.cs
- Form.cs
- SystemWebSectionGroup.cs
- EventData.cs
- SRef.cs
- externdll.cs
- RSAPKCS1SignatureFormatter.cs
- SystemFonts.cs
- StylusPointProperty.cs
- GridView.cs
- WindowsFormsLinkLabel.cs
- PartialTrustVisibleAssemblyCollection.cs
- URLString.cs
- DesignerTextViewAdapter.cs
- ArrayExtension.cs
- SqlHelper.cs
- EventDescriptorCollection.cs
- ManipulationCompletedEventArgs.cs
- AssociatedControlConverter.cs
- TypeUnloadedException.cs
- AxParameterData.cs
- ReservationNotFoundException.cs
- CompoundFileStorageReference.cs
- ToolStripRendererSwitcher.cs
- Container.cs
- ApplicationInfo.cs
- TextTreeExtractElementUndoUnit.cs
- ConnectionStringSettings.cs
- CorrelationService.cs
- UnicodeEncoding.cs
- FixedBufferAttribute.cs
- ConfigXmlComment.cs
- UnsafeNativeMethodsTablet.cs
- NamespaceCollection.cs
- SqlServices.cs
- FormsAuthenticationModule.cs
- ClientBuildManagerCallback.cs
- RequestQueue.cs
- DelimitedListTraceListener.cs