Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / Configuration / TagMapCollection.cs / 2 / TagMapCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Web.UI; using System.Web.Compilation; using System.Threading; using System.Web.Configuration; using System.Security.Permissions; [ConfigurationCollection(typeof(TagMapInfo))] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class TagMapCollection : ConfigurationElementCollection { private static ConfigurationPropertyCollection _properties; private Hashtable _tagMappings; static TagMapCollection() { // Property initialization _properties = new ConfigurationPropertyCollection(); } public TagMapCollection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } public TagMapInfo this[int index] { get { return (TagMapInfo)BaseGet(index); } set { if (BaseGet(index) != null) { BaseRemoveAt(index); } BaseAdd(index, value); } } public void Add(TagMapInfo tagMapInformation) { BaseAdd(tagMapInformation); } public void Remove(TagMapInfo tagMapInformation) { BaseRemove(GetElementKey(tagMapInformation)); } public void Clear() { BaseClear(); } protected override ConfigurationElement CreateNewElement() { return new TagMapInfo(); } protected override Object GetElementKey(ConfigurationElement element) { return ((TagMapInfo)element).TagType; } internal Hashtable TagTypeMappingInternal { get { if (_tagMappings == null) { lock (this) { if (_tagMappings == null) { Hashtable tagMappings = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach (TagMapInfo tmi in this) { Type tagType = ConfigUtil.GetType(tmi.TagType, "tagType", tmi); Type mappedTagType = ConfigUtil.GetType(tmi.MappedTagType, "mappedTagType", tmi); if (tagType.IsAssignableFrom(mappedTagType) == false) { throw new ConfigurationErrorsException( SR.GetString( SR.Mapped_type_must_inherit, tmi.MappedTagType, tmi.TagType), tmi.ElementInformation.Properties["mappedTagType"].Source, tmi.ElementInformation.Properties["mappedTagType"].LineNumber); } tagMappings[tagType] = mappedTagType; } _tagMappings = tagMappings; } } } return _tagMappings; } } } }
Link Menu
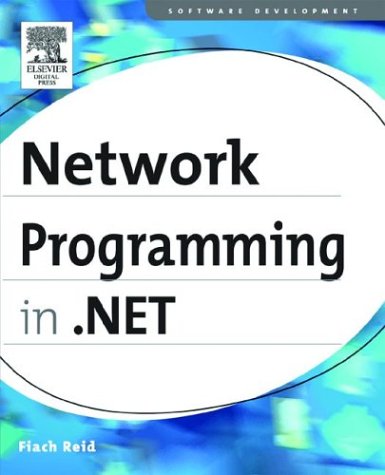
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MatrixAnimationUsingKeyFrames.cs
- NavigationPropertyEmitter.cs
- SmiTypedGetterSetter.cs
- RefreshEventArgs.cs
- X509ChainPolicy.cs
- DataControlPagerLinkButton.cs
- XmlSchemaInferenceException.cs
- TextRunTypographyProperties.cs
- XmlSchemaSearchPattern.cs
- DbConnectionFactory.cs
- DefaultEventAttribute.cs
- ByteAnimation.cs
- CapabilitiesState.cs
- ReliableInputConnection.cs
- CustomAssemblyResolver.cs
- DataServiceHostFactory.cs
- WindowPattern.cs
- TailCallAnalyzer.cs
- PngBitmapDecoder.cs
- Pts.cs
- BufferedGraphics.cs
- VolatileResourceManager.cs
- DataTableMapping.cs
- SharedTcpTransportManager.cs
- CachedPathData.cs
- HtmlButton.cs
- UnsignedPublishLicense.cs
- RelationalExpressions.cs
- DragStartedEventArgs.cs
- SessionPageStateSection.cs
- UserPreferenceChangingEventArgs.cs
- MetadataItem_Static.cs
- XmlSchemaObject.cs
- OperationAbortedException.cs
- BindingBase.cs
- PersonalizablePropertyEntry.cs
- BulletChrome.cs
- DynamicValueConverter.cs
- validation.cs
- GridViewDeleteEventArgs.cs
- PipeSecurity.cs
- ControlValuePropertyAttribute.cs
- EncodingInfo.cs
- StringToken.cs
- JpegBitmapEncoder.cs
- ListSortDescription.cs
- Int16AnimationUsingKeyFrames.cs
- HiddenFieldDesigner.cs
- UpdateCommand.cs
- PersonalizationProviderHelper.cs
- CssClassPropertyAttribute.cs
- InstanceBehavior.cs
- safelinkcollection.cs
- BitmapImage.cs
- TransformGroup.cs
- ZoneIdentityPermission.cs
- BitmapEncoder.cs
- RealProxy.cs
- ProfileGroupSettings.cs
- InputElement.cs
- Timer.cs
- CommonGetThemePartSize.cs
- log.cs
- SourceElementsCollection.cs
- WebScriptMetadataFormatter.cs
- GZipStream.cs
- DataConnectionHelper.cs
- StylusPointProperties.cs
- DeclarativeCatalogPart.cs
- QueueException.cs
- VirtualDirectoryMapping.cs
- wpf-etw.cs
- KeyValuePair.cs
- AsmxEndpointPickerExtension.cs
- LogLogRecordHeader.cs
- DetailsViewDeletedEventArgs.cs
- ISAPIRuntime.cs
- HttpModuleActionCollection.cs
- QuaternionKeyFrameCollection.cs
- NullRuntimeConfig.cs
- FileClassifier.cs
- HtmlValidationSummaryAdapter.cs
- StackBuilderSink.cs
- ACL.cs
- StylusCollection.cs
- Table.cs
- PrinterUnitConvert.cs
- MenuCommand.cs
- XmlSchemaRedefine.cs
- WebPartEditVerb.cs
- ITreeGenerator.cs
- SessionStateModule.cs
- DataGridColumnEventArgs.cs
- AnimationLayer.cs
- FatalException.cs
- OwnerDrawPropertyBag.cs
- WindowShowOrOpenTracker.cs
- SoapIncludeAttribute.cs
- SvcMapFile.cs
- ControlCollection.cs