Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebParts / ConsumerConnectionPointCollection.cs / 1 / ConsumerConnectionPointCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class ConsumerConnectionPointCollection : ReadOnlyCollectionBase { private HybridDictionary _ids; public ConsumerConnectionPointCollection() { } public ConsumerConnectionPointCollection(ICollection connectionPoints) { if (connectionPoints == null) { throw new ArgumentNullException("connectionPoints"); } _ids = new HybridDictionary(connectionPoints.Count, true /* caseInsensitive */); foreach (object obj in connectionPoints) { if (obj == null) { throw new ArgumentException(SR.GetString(SR.Collection_CantAddNull), "connectionPoints"); } ConsumerConnectionPoint point = obj as ConsumerConnectionPoint; if (point == null) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "ConsumerConnectionPoint"), "connectionPoints"); } string id = point.ID; if (!_ids.Contains(id)) { InnerList.Add(point); _ids.Add(id, point); } else { throw new ArgumentException(SR.GetString( SR.WebPart_Collection_DuplicateID, "ConsumerConnectionPoint", id), "connectionPoints"); } } } public ConsumerConnectionPoint Default { get { return this[ConnectionPoint.DefaultID]; } } public ConsumerConnectionPoint this[int index] { get { return (ConsumerConnectionPoint)InnerList[index]; } } public ConsumerConnectionPoint this[string id] { get { return ((_ids != null) ? (ConsumerConnectionPoint)_ids[id] : null); } } public bool Contains(ConsumerConnectionPoint connectionPoint) { return InnerList.Contains(connectionPoint); } public int IndexOf(ConsumerConnectionPoint connectionPoint) { return InnerList.IndexOf(connectionPoint); } public void CopyTo(ConsumerConnectionPoint[] array, int index) { InnerList.CopyTo(array, index); } } }
Link Menu
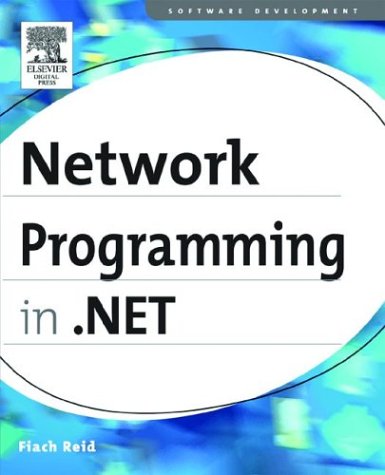
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeLoadException.cs
- DiscoveryOperationContextExtension.cs
- MarkupCompilePass2.cs
- CallbackHandler.cs
- Button.cs
- DurableTimerExtension.cs
- ToolStripContentPanel.cs
- RegionInfo.cs
- ParallelTimeline.cs
- HttpContextWrapper.cs
- AppSettingsExpressionBuilder.cs
- DbMetaDataColumnNames.cs
- FastEncoderWindow.cs
- CheckBoxList.cs
- TemplateNameScope.cs
- SystemColorTracker.cs
- WinInet.cs
- FrugalMap.cs
- ByteKeyFrameCollection.cs
- LoginView.cs
- InvalidOleVariantTypeException.cs
- PropertyReferenceExtension.cs
- HandleRef.cs
- InputLangChangeRequestEvent.cs
- ColumnWidthChangingEvent.cs
- TabItemWrapperAutomationPeer.cs
- listitem.cs
- ResourceExpressionEditor.cs
- ContractAdapter.cs
- CodeAccessSecurityEngine.cs
- TableLayoutSettings.cs
- TypeValidationEventArgs.cs
- PointCollection.cs
- XmlSchemaType.cs
- StateBag.cs
- ControlAdapter.cs
- TypeSystem.cs
- Descriptor.cs
- DPCustomTypeDescriptor.cs
- LicenseException.cs
- Message.cs
- DataGridViewTextBoxCell.cs
- XPathParser.cs
- RectKeyFrameCollection.cs
- ContentType.cs
- UrlAuthorizationModule.cs
- SlotInfo.cs
- TextDocumentView.cs
- EntityModelBuildProvider.cs
- processwaithandle.cs
- AssemblyResourceLoader.cs
- SHA512Managed.cs
- AttributeTableBuilder.cs
- ClientSideQueueItem.cs
- X509SecurityTokenProvider.cs
- ProgressBarAutomationPeer.cs
- GridViewUpdateEventArgs.cs
- AppSecurityManager.cs
- SignedXml.cs
- HtmlGenericControl.cs
- Boolean.cs
- XmlWriter.cs
- OleDbEnumerator.cs
- CodeTypeReferenceSerializer.cs
- Manipulation.cs
- XmlTextReaderImplHelpers.cs
- ConnectionPointGlyph.cs
- SurrogateEncoder.cs
- SrgsOneOf.cs
- AuthorizationSection.cs
- SerializationInfo.cs
- PropertyEmitterBase.cs
- SeekStoryboard.cs
- SecureEnvironment.cs
- SamlAction.cs
- relpropertyhelper.cs
- TypeSystemHelpers.cs
- RequestCacheManager.cs
- IDataContractSurrogate.cs
- FontInfo.cs
- XsltConvert.cs
- Button.cs
- Int32Animation.cs
- GeneralTransformCollection.cs
- updateconfighost.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- TypeReference.cs
- CompiledIdentityConstraint.cs
- EventItfInfo.cs
- ChannelSinkStacks.cs
- ListCommandEventArgs.cs
- ParagraphResult.cs
- BinaryConverter.cs
- XmlChildNodes.cs
- Content.cs
- StringSource.cs
- OpenTypeLayout.cs
- WebPartCloseVerb.cs
- MenuItemStyle.cs
- HttpWebRequest.cs