Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / httpapplicationstate.cs / 1 / httpapplicationstate.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Application State Dictionary class * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web { using System.Threading; using System.Runtime.InteropServices; using System.Security.Permissions; using System.Collections; using System.Collections.Specialized; using System.Web; using System.Web.Util; // // Application state collection // ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpApplicationState : NameObjectCollectionBase { // app lock with auto-unlock feature private HttpApplicationStateLock _lock = new HttpApplicationStateLock(); // static object collections private HttpStaticObjectsCollection _applicationStaticObjects; private HttpStaticObjectsCollection _sessionStaticObjects; internal HttpApplicationState() : this(null, null) { } internal HttpApplicationState(HttpStaticObjectsCollection applicationStaticObjects, HttpStaticObjectsCollection sessionStaticObjects) : base(Misc.CaseInsensitiveInvariantKeyComparer) { _applicationStaticObjects = applicationStaticObjects; if (_applicationStaticObjects == null) _applicationStaticObjects = new HttpStaticObjectsCollection(); _sessionStaticObjects = sessionStaticObjects; if (_sessionStaticObjects == null) _sessionStaticObjects = new HttpStaticObjectsCollection(); } // // Internal accessor to session static objects collection // internal HttpStaticObjectsCollection SessionStaticObjects { get { return _sessionStaticObjects;} } // // Implementation of standard collection stuff // ////// The HttpApplicationState class enables developers to /// share global information across multiple requests, sessions, and pipelines /// within an ASP.NET application. (An ASP.NET application is the sum of all files, pages, /// handlers, modules, and code /// within the scope of a virtual directory and its /// subdirectories on a single web server). /// ////// public override int Count { get { int c = 0; _lock.AcquireRead(); try { c = base.Count; } finally { _lock.ReleaseRead(); } return c; } } // modifying methods ///Gets /// the number of item objects in the application state collection. ////// public void Add(String name, Object value) { _lock.AcquireWrite(); try { BaseAdd(name, value); } finally { _lock.ReleaseWrite(); } } ////// Adds /// a new state object to the application state collection. /// ////// public void Set(String name, Object value) { _lock.AcquireWrite(); try { BaseSet(name, value); } finally { _lock.ReleaseWrite(); } } ///Updates an HttpApplicationState value within the collection. ////// public void Remove(String name) { _lock.AcquireWrite(); try { BaseRemove(name); } finally { _lock.ReleaseWrite(); } } ///Removes /// an /// object from the application state collection by name. ////// public void RemoveAt(int index) { _lock.AcquireWrite(); try { BaseRemoveAt(index); } finally { _lock.ReleaseWrite(); } } ///Removes /// an /// object from the application state collection by name. ////// public void Clear() { _lock.AcquireWrite(); try { BaseClear(); } finally { _lock.ReleaseWrite(); } } ////// Removes /// all objects from the application state collection. /// ////// public void RemoveAll() { Clear(); } // access by key ////// Removes /// all objects from the application state collection. /// ////// public Object Get(String name) { Object obj = null; _lock.AcquireRead(); try { obj = BaseGet(name); } finally { _lock.ReleaseRead(); } return obj; } ////// Enables user to retrieve application state object by name. /// ////// public Object this[String name] { get { return Get(name);} set { Set(name, value);} } // access by index ///Enables /// a user to add/remove/update a single application state object. ////// public Object Get(int index) { Object obj = null; _lock.AcquireRead(); try { obj = BaseGet(index); } finally { _lock.ReleaseRead(); } return obj; } ////// Enables user /// to retrieve a single application state object by index. /// ////// public String GetKey(int index) { String s = null; _lock.AcquireRead(); try { s = BaseGetKey(index); } finally { _lock.ReleaseRead(); } return s; } ////// Enables user to retrieve an application state object name by index. /// ////// public Object this[int index] { get { return Get(index);} } // access to keys and values as arrays ////// Enables /// user to retrieve an application state object by index. /// ////// public String[] AllKeys { get { String [] allKeys = null; _lock.AcquireRead(); try { allKeys = BaseGetAllKeys(); } finally { _lock.ReleaseRead(); } return allKeys; } } // // Public properties // ////// Enables user /// to retrieve all application state object names in collection. /// ////// public HttpApplicationState Contents { get { return this;} } ////// Returns "this". Provided for legacy ASP compatibility. /// ////// public HttpStaticObjectsCollection StaticObjects { get { return _applicationStaticObjects;} } // // Locking support // ////// Exposes all objects declared via an <object /// runat=server></object> tag within the ASP.NET application file. /// ////// public void Lock() { _lock.AcquireWrite(); } ////// Locks /// access to all application state variables. Facilitates access /// synchronization. /// ////// public void UnLock() { _lock.ReleaseWrite(); } internal void EnsureUnLock() { _lock.EnsureReleaseWrite(); } } // // Recursive read-write lock that allows removing of all // outstanding write locks from the current thread at once // internal class HttpApplicationStateLock : ReadWriteObjectLock { private int _recursionCount; private int _threadId; internal HttpApplicationStateLock() { } internal override void AcquireRead() { int currentThreadId = SafeNativeMethods.GetCurrentThreadId(); if (_threadId != currentThreadId) base.AcquireRead(); // only if no write lock } internal override void ReleaseRead() { int currentThreadId = SafeNativeMethods.GetCurrentThreadId(); if (_threadId != currentThreadId) base.ReleaseRead(); // only if no write lock } internal override void AcquireWrite() { int currentThreadId = SafeNativeMethods.GetCurrentThreadId(); if (_threadId == currentThreadId) { _recursionCount++; } else { base.AcquireWrite(); _threadId = currentThreadId; _recursionCount = 1; } } internal override void ReleaseWrite() { int currentThreadId = SafeNativeMethods.GetCurrentThreadId(); if (_threadId == currentThreadId) { if (--_recursionCount == 0) { _threadId = 0; base.ReleaseWrite(); } } } // // release all write locks held by the current thread // internal void EnsureReleaseWrite() { int currentThreadId = SafeNativeMethods.GetCurrentThreadId(); if (_threadId == currentThreadId) { _threadId = 0; _recursionCount = 0; base.ReleaseWrite(); } } } }/// Unocks access to all application state variables. Facilitates access /// synchronization. /// ///
Link Menu
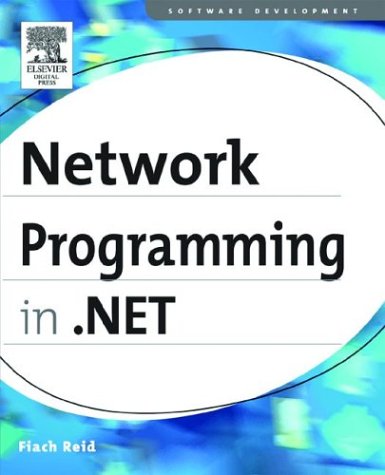
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThemeConfigurationDialog.cs
- MaskedTextProvider.cs
- RegexGroupCollection.cs
- HtmlContainerControl.cs
- DetailsViewRowCollection.cs
- LostFocusEventManager.cs
- XmlWrappingWriter.cs
- ConfigXmlText.cs
- AttributeUsageAttribute.cs
- RequiredFieldValidator.cs
- AutomationIdentifier.cs
- SchemaTableOptionalColumn.cs
- WizardForm.cs
- InitializationEventAttribute.cs
- SqlDuplicator.cs
- GotoExpression.cs
- RSAOAEPKeyExchangeFormatter.cs
- ListSourceHelper.cs
- base64Transforms.cs
- MetafileHeader.cs
- BlurEffect.cs
- ImmutableAssemblyCacheEntry.cs
- XmlNamedNodeMap.cs
- CompensatableSequenceActivity.cs
- HttpConfigurationContext.cs
- SR.cs
- Brush.cs
- DeadCharTextComposition.cs
- DataBoundControlAdapter.cs
- SerTrace.cs
- CodeAccessSecurityEngine.cs
- WinInetCache.cs
- AutomationEvent.cs
- ArrayElementGridEntry.cs
- ConstraintConverter.cs
- Task.cs
- XsdSchemaFileEditor.cs
- RenderData.cs
- DesignTimeDataBinding.cs
- TransformerInfoCollection.cs
- RemotingAttributes.cs
- EpmCustomContentSerializer.cs
- dsa.cs
- SplitterPanel.cs
- IsolatedStorageFilePermission.cs
- BitmapVisualManager.cs
- WebPartDisplayModeEventArgs.cs
- HtmlHistory.cs
- DrawToolTipEventArgs.cs
- HostingEnvironmentSection.cs
- ColorTranslator.cs
- LineServicesRun.cs
- Int16KeyFrameCollection.cs
- GZipStream.cs
- SmtpTransport.cs
- SqlTriggerContext.cs
- Helpers.cs
- ProfileParameter.cs
- X509RecipientCertificateClientElement.cs
- UInt16Converter.cs
- TemplateInstanceAttribute.cs
- PointHitTestParameters.cs
- EmptyReadOnlyDictionaryInternal.cs
- ReadWriteSpinLock.cs
- DeclarationUpdate.cs
- CodeArgumentReferenceExpression.cs
- AssemblyInfo.cs
- AsyncDataRequest.cs
- KeyMatchBuilder.cs
- ComponentChangingEvent.cs
- Permission.cs
- WebPartDisplayMode.cs
- DayRenderEvent.cs
- ConfigurationSection.cs
- BamlRecordReader.cs
- OrCondition.cs
- GenericFlowSwitchHelper.cs
- SiteMapNodeItem.cs
- FilteredXmlReader.cs
- PointAnimationUsingPath.cs
- NameValuePermission.cs
- ReferenceSchema.cs
- DBBindings.cs
- PolicyFactory.cs
- ValidationErrorCollection.cs
- _CommandStream.cs
- ConfigXmlCDataSection.cs
- TextEffectResolver.cs
- PageFunction.cs
- BinHexDecoder.cs
- UnsafeNativeMethods.cs
- RunClient.cs
- Deserializer.cs
- XmlDictionaryWriter.cs
- EventMemberCodeDomSerializer.cs
- GridViewCommandEventArgs.cs
- DataBindEngine.cs
- TreeNodeConverter.cs
- DebugController.cs
- MetadataArtifactLoaderResource.cs