Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / ReferenceSchema.cs / 2 / ReferenceSchema.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Xml; using System.Data; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { ////// Summary description for UsingElement. /// internal class UsingElement : SchemaElement { #region Instance Fields private string _alias = null; private string _namespaceName = null; #endregion #region Public Methods ////// /// /// internal UsingElement(Schema parentElement) : base(parentElement) { } #endregion #region Public Properties ////// /// public virtual string Alias { get { return _alias; } private set { _alias = value; } } ////// /// public virtual string NamespaceName { get { return _namespaceName; } private set { _namespaceName = value; } } ////// /// public override string FQName { get { return null; } } #endregion #region Protected Properties ////// /// protected override bool ProhibitAttribute(string namespaceUri, string localName) { if (base.ProhibitAttribute(namespaceUri, localName)) { return true; } if (namespaceUri == null && localName == XmlConstants.Name) { return false; } return false; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.Namespace)) { HandleNamespaceAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.Alias)) { HandleAliasAttribute(reader); return true; } return false; } #endregion #region Private Methods ////// /// /// private void HandleNamespaceAttribute(XmlReader reader) { Debug.Assert(String.IsNullOrEmpty(NamespaceName), "Alias must be set only once"); ReturnValuereturnValue = HandleDottedNameAttribute(reader,NamespaceName, Strings.AlreadyDefined); if ( returnValue.Succeeded ) NamespaceName = returnValue.Value; } /// /// /// /// private void HandleAliasAttribute(XmlReader reader) { Debug.Assert(String.IsNullOrEmpty(Alias), "Alias must be set only once"); Alias = HandleUndottedNameAttribute(reader, Alias); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Xml; using System.Data; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { ////// Summary description for UsingElement. /// internal class UsingElement : SchemaElement { #region Instance Fields private string _alias = null; private string _namespaceName = null; #endregion #region Public Methods ////// /// /// internal UsingElement(Schema parentElement) : base(parentElement) { } #endregion #region Public Properties ////// /// public virtual string Alias { get { return _alias; } private set { _alias = value; } } ////// /// public virtual string NamespaceName { get { return _namespaceName; } private set { _namespaceName = value; } } ////// /// public override string FQName { get { return null; } } #endregion #region Protected Properties ////// /// protected override bool ProhibitAttribute(string namespaceUri, string localName) { if (base.ProhibitAttribute(namespaceUri, localName)) { return true; } if (namespaceUri == null && localName == XmlConstants.Name) { return false; } return false; } protected override bool HandleAttribute(XmlReader reader) { if (base.HandleAttribute(reader)) { return true; } else if (CanHandleAttribute(reader, XmlConstants.Namespace)) { HandleNamespaceAttribute(reader); return true; } else if (CanHandleAttribute(reader, XmlConstants.Alias)) { HandleAliasAttribute(reader); return true; } return false; } #endregion #region Private Methods ////// /// /// private void HandleNamespaceAttribute(XmlReader reader) { Debug.Assert(String.IsNullOrEmpty(NamespaceName), "Alias must be set only once"); ReturnValuereturnValue = HandleDottedNameAttribute(reader,NamespaceName, Strings.AlreadyDefined); if ( returnValue.Succeeded ) NamespaceName = returnValue.Value; } /// /// /// /// private void HandleAliasAttribute(XmlReader reader) { Debug.Assert(String.IsNullOrEmpty(Alias), "Alias must be set only once"); Alias = HandleUndottedNameAttribute(reader, Alias); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
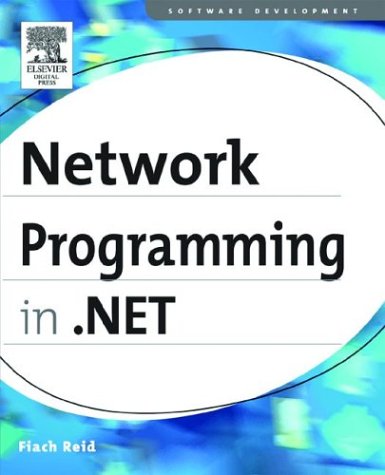
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpConfigurationContext.cs
- ContentHostHelper.cs
- SignatureGenerator.cs
- DecimalMinMaxAggregationOperator.cs
- DateTimeOffset.cs
- EventDescriptorCollection.cs
- WindowsStatusBar.cs
- Models.cs
- NullReferenceException.cs
- TablePatternIdentifiers.cs
- XmlAnyElementAttributes.cs
- LocatorPartList.cs
- NamedPermissionSet.cs
- NameTable.cs
- TextTreeInsertUndoUnit.cs
- XmlRawWriter.cs
- TablePatternIdentifiers.cs
- SymbolPair.cs
- CacheSection.cs
- ActionMessageFilterTable.cs
- NetworkInformationPermission.cs
- IDQuery.cs
- XPathPatternParser.cs
- FormViewModeEventArgs.cs
- ErrorHandler.cs
- util.cs
- SortedDictionary.cs
- ExpandCollapseProviderWrapper.cs
- MetadataUtilsSmi.cs
- AsnEncodedData.cs
- XmlTextReader.cs
- NavigationWindow.cs
- InternalRelationshipCollection.cs
- CorrelationManager.cs
- BitmapData.cs
- ItemContainerGenerator.cs
- PortCache.cs
- DummyDataSource.cs
- EpmHelper.cs
- RegexTree.cs
- XamlBuildProvider.cs
- ConfigurationValidatorBase.cs
- OrthographicCamera.cs
- CalendarDateRange.cs
- BuilderPropertyEntry.cs
- UnsafeNativeMethodsPenimc.cs
- CaseExpr.cs
- FlagsAttribute.cs
- MethodBuilder.cs
- WebDescriptionAttribute.cs
- Context.cs
- SolidColorBrush.cs
- WebSysDisplayNameAttribute.cs
- KeyValuePair.cs
- DataControlPagerLinkButton.cs
- DataGridViewTextBoxEditingControl.cs
- LocalValueEnumerator.cs
- SafeWaitHandle.cs
- ExpressionContext.cs
- ContentDisposition.cs
- InlineObject.cs
- XPathSingletonIterator.cs
- LocalizedNameDescriptionPair.cs
- ReferentialConstraintRoleElement.cs
- XmlCodeExporter.cs
- EntitySqlQueryState.cs
- ISO2022Encoding.cs
- IgnoreDeviceFilterElement.cs
- Parser.cs
- ColorDialog.cs
- DatePickerTextBox.cs
- DataGridViewCellErrorTextNeededEventArgs.cs
- StatusBarDrawItemEvent.cs
- TargetParameterCountException.cs
- XmlSchemaSimpleContentRestriction.cs
- EdmToObjectNamespaceMap.cs
- PrtTicket_Editor.cs
- ExtenderProvidedPropertyAttribute.cs
- CardSpaceSelector.cs
- DocumentProperties.cs
- SeparatorAutomationPeer.cs
- ContentPlaceHolder.cs
- DbgUtil.cs
- TogglePattern.cs
- XmlSchemaSimpleTypeList.cs
- XPathScanner.cs
- HuffmanTree.cs
- OracleConnectionString.cs
- DataBoundControl.cs
- Ops.cs
- Divide.cs
- BinaryReader.cs
- ControlCollection.cs
- Int32Animation.cs
- Vector3D.cs
- SystemDiagnosticsSection.cs
- ViewManager.cs
- SqlTransaction.cs
- StrongNameIdentityPermission.cs
- BufferedStream.cs