Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / Ink / StrokeFIndices.cs / 1 / StrokeFIndices.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Utility; using MS.Internal; using System; using System.Windows; using System.Collections.Generic; using System.Globalization; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink { #region StrokeFIndices ////// A helper struct that represents a fragment of a stroke spine. /// internal struct StrokeFIndices : IEquatable{ #region Private statics private static StrokeFIndices s_empty = new StrokeFIndices(AfterLast, BeforeFirst); private static StrokeFIndices s_full = new StrokeFIndices(BeforeFirst, AfterLast); #endregion #region Internal API /// /// BeforeFirst /// ///internal static double BeforeFirst { get { return double.MinValue; } } /// /// AfterLast /// ///internal static double AfterLast { get { return double.MaxValue; } } /// /// StrokeFIndices /// /// beginFIndex /// endFIndex internal StrokeFIndices(double beginFIndex, double endFIndex) { _beginFIndex = beginFIndex; _endFIndex = endFIndex; } ////// BeginFIndex /// ///internal double BeginFIndex { get { return _beginFIndex; } set { _beginFIndex = value; } } /// /// EndFIndex /// ///internal double EndFIndex { get { return _endFIndex; } set { _endFIndex = value;} } /// /// ToString /// public override string ToString() { return "{" + GetStringRepresentation(_beginFIndex) + "," + GetStringRepresentation(_endFIndex) + "}"; } ////// Equals /// /// ///public bool Equals(StrokeFIndices strokeFIndices) { return (strokeFIndices == this); } /// /// Equals /// /// ///public override bool Equals(Object obj) { // Check for null and compare run-time types if (obj == null || GetType() != obj.GetType()) return false; return ((StrokeFIndices)obj == this); } /// /// GetHashCode /// ///public override int GetHashCode() { return _beginFIndex.GetHashCode() ^ _endFIndex.GetHashCode(); } /// /// operator == /// /// /// ///public static bool operator ==(StrokeFIndices sfiLeft, StrokeFIndices sfiRight) { return (DoubleUtil.AreClose(sfiLeft._beginFIndex, sfiRight._beginFIndex) && DoubleUtil.AreClose(sfiLeft._endFIndex, sfiRight._endFIndex)); } /// /// operator != /// /// /// ///public static bool operator !=(StrokeFIndices sfiLeft, StrokeFIndices sfiRight) { return !(sfiLeft == sfiRight); } internal static string GetStringRepresentation(double fIndex) { if (DoubleUtil.AreClose(fIndex, StrokeFIndices.BeforeFirst)) { return "BeforeFirst"; } if (DoubleUtil.AreClose(fIndex, StrokeFIndices.AfterLast)) { return "AfterLast"; } return fIndex.ToString(CultureInfo.InvariantCulture); } /// /// /// internal static StrokeFIndices Empty { get { return s_empty; } } ////// /// internal static StrokeFIndices Full { get { return s_full; } } ////// /// internal bool IsEmpty { get { return DoubleUtil.GreaterThanOrClose(_beginFIndex, _endFIndex); } } ////// /// internal bool IsFull { get { return ((DoubleUtil.AreClose(_beginFIndex, BeforeFirst)) && (DoubleUtil.AreClose(_endFIndex,AfterLast))); } } #if DEBUG ////// /// private bool IsValid { get { return !double.IsNaN(_beginFIndex) && !double.IsNaN(_endFIndex) && _beginFIndex < _endFIndex; } } #endif ////// Compare StrokeFIndices based on the BeinFIndex /// /// ///internal int CompareTo(StrokeFIndices fIndices) { #if DEBUG System.Diagnostics.Debug.Assert(!double.IsNaN(_beginFIndex) && !double.IsNaN(_endFIndex) && DoubleUtil.LessThan(_beginFIndex, _endFIndex)); #endif if (DoubleUtil.AreClose(BeginFIndex, fIndices.BeginFIndex)) { return 0; } else if (DoubleUtil.GreaterThan(BeginFIndex, fIndices.BeginFIndex)) { return 1; } else { return -1; } } #endregion #region Fields private double _beginFIndex; private double _endFIndex; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Utility; using MS.Internal; using System; using System.Windows; using System.Collections.Generic; using System.Globalization; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink { #region StrokeFIndices ////// A helper struct that represents a fragment of a stroke spine. /// internal struct StrokeFIndices : IEquatable{ #region Private statics private static StrokeFIndices s_empty = new StrokeFIndices(AfterLast, BeforeFirst); private static StrokeFIndices s_full = new StrokeFIndices(BeforeFirst, AfterLast); #endregion #region Internal API /// /// BeforeFirst /// ///internal static double BeforeFirst { get { return double.MinValue; } } /// /// AfterLast /// ///internal static double AfterLast { get { return double.MaxValue; } } /// /// StrokeFIndices /// /// beginFIndex /// endFIndex internal StrokeFIndices(double beginFIndex, double endFIndex) { _beginFIndex = beginFIndex; _endFIndex = endFIndex; } ////// BeginFIndex /// ///internal double BeginFIndex { get { return _beginFIndex; } set { _beginFIndex = value; } } /// /// EndFIndex /// ///internal double EndFIndex { get { return _endFIndex; } set { _endFIndex = value;} } /// /// ToString /// public override string ToString() { return "{" + GetStringRepresentation(_beginFIndex) + "," + GetStringRepresentation(_endFIndex) + "}"; } ////// Equals /// /// ///public bool Equals(StrokeFIndices strokeFIndices) { return (strokeFIndices == this); } /// /// Equals /// /// ///public override bool Equals(Object obj) { // Check for null and compare run-time types if (obj == null || GetType() != obj.GetType()) return false; return ((StrokeFIndices)obj == this); } /// /// GetHashCode /// ///public override int GetHashCode() { return _beginFIndex.GetHashCode() ^ _endFIndex.GetHashCode(); } /// /// operator == /// /// /// ///public static bool operator ==(StrokeFIndices sfiLeft, StrokeFIndices sfiRight) { return (DoubleUtil.AreClose(sfiLeft._beginFIndex, sfiRight._beginFIndex) && DoubleUtil.AreClose(sfiLeft._endFIndex, sfiRight._endFIndex)); } /// /// operator != /// /// /// ///public static bool operator !=(StrokeFIndices sfiLeft, StrokeFIndices sfiRight) { return !(sfiLeft == sfiRight); } internal static string GetStringRepresentation(double fIndex) { if (DoubleUtil.AreClose(fIndex, StrokeFIndices.BeforeFirst)) { return "BeforeFirst"; } if (DoubleUtil.AreClose(fIndex, StrokeFIndices.AfterLast)) { return "AfterLast"; } return fIndex.ToString(CultureInfo.InvariantCulture); } /// /// /// internal static StrokeFIndices Empty { get { return s_empty; } } ////// /// internal static StrokeFIndices Full { get { return s_full; } } ////// /// internal bool IsEmpty { get { return DoubleUtil.GreaterThanOrClose(_beginFIndex, _endFIndex); } } ////// /// internal bool IsFull { get { return ((DoubleUtil.AreClose(_beginFIndex, BeforeFirst)) && (DoubleUtil.AreClose(_endFIndex,AfterLast))); } } #if DEBUG ////// /// private bool IsValid { get { return !double.IsNaN(_beginFIndex) && !double.IsNaN(_endFIndex) && _beginFIndex < _endFIndex; } } #endif ////// Compare StrokeFIndices based on the BeinFIndex /// /// ///internal int CompareTo(StrokeFIndices fIndices) { #if DEBUG System.Diagnostics.Debug.Assert(!double.IsNaN(_beginFIndex) && !double.IsNaN(_endFIndex) && DoubleUtil.LessThan(_beginFIndex, _endFIndex)); #endif if (DoubleUtil.AreClose(BeginFIndex, fIndices.BeginFIndex)) { return 0; } else if (DoubleUtil.GreaterThan(BeginFIndex, fIndices.BeginFIndex)) { return 1; } else { return -1; } } #endregion #region Fields private double _beginFIndex; private double _endFIndex; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
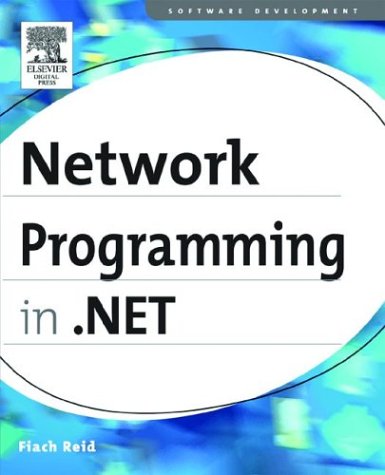
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HandlerFactoryWrapper.cs
- BinaryFormatter.cs
- unitconverter.cs
- CacheSection.cs
- DataObjectFieldAttribute.cs
- DocumentGrid.cs
- PersonalizationProviderHelper.cs
- ServiceKnownTypeAttribute.cs
- ObjectReaderCompiler.cs
- BooleanConverter.cs
- HasRunnableWorkflowEvent.cs
- DataGridRowsPresenter.cs
- Queue.cs
- GeometryModel3D.cs
- ResXFileRef.cs
- diagnosticsswitches.cs
- PropertyKey.cs
- Authorization.cs
- SemaphoreFullException.cs
- DurableOperationAttribute.cs
- UriTemplateTable.cs
- GridViewHeaderRowPresenter.cs
- ImageField.cs
- TileBrush.cs
- NonVisualControlAttribute.cs
- CheckBoxBaseAdapter.cs
- SystemBrushes.cs
- ConnectionStringsSection.cs
- TagMapInfo.cs
- UrlAuthFailedErrorFormatter.cs
- CompositeScriptReferenceEventArgs.cs
- WeakRefEnumerator.cs
- QilLoop.cs
- SqlStream.cs
- SystemUdpStatistics.cs
- DayRenderEvent.cs
- RoutingSection.cs
- JumpPath.cs
- ResourceReader.cs
- RelationshipFixer.cs
- XmlSchemaSubstitutionGroup.cs
- DerivedKeySecurityToken.cs
- sqlcontext.cs
- GeneralTransformCollection.cs
- XmlAutoDetectWriter.cs
- TypeExtensionSerializer.cs
- KeyFrames.cs
- SecurityContext.cs
- ImageDrawing.cs
- EmptyEnumerator.cs
- InkCanvasSelectionAdorner.cs
- CellPartitioner.cs
- GlobalizationSection.cs
- XmlSchemaComplexType.cs
- SerializationAttributes.cs
- UserControlAutomationPeer.cs
- XmlSchemaInferenceException.cs
- Win32.cs
- UInt32Storage.cs
- TimeEnumHelper.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- CollectionViewGroup.cs
- GetPageNumberCompletedEventArgs.cs
- HwndSourceParameters.cs
- WindowsProgressbar.cs
- MyContact.cs
- NameValuePermission.cs
- DataServiceQueryProvider.cs
- ExpandedWrapper.cs
- ArrayElementGridEntry.cs
- Enum.cs
- PLINQETWProvider.cs
- BindingEntityInfo.cs
- LassoHelper.cs
- PageSetupDialog.cs
- ScrollEvent.cs
- TextTrailingCharacterEllipsis.cs
- SourceChangedEventArgs.cs
- FixedDocument.cs
- EntityClassGenerator.cs
- BuildProvidersCompiler.cs
- MetadataItem.cs
- InputMethodStateTypeInfo.cs
- MulticastIPAddressInformationCollection.cs
- CodeAttributeDeclaration.cs
- TextTreeFixupNode.cs
- XmlBaseWriter.cs
- StructuredType.cs
- ResourceReferenceKeyNotFoundException.cs
- BamlRecordReader.cs
- MsmqNonTransactedPoisonHandler.cs
- DataSpaceManager.cs
- MimeMapping.cs
- TreeNodeStyleCollectionEditor.cs
- UIElement.cs
- SelectionPattern.cs
- FormsAuthenticationCredentials.cs
- TreeViewHitTestInfo.cs
- MulticastOption.cs
- shaperfactory.cs