Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Documents / RtfControls.cs / 1 / RtfControls.cs
//---------------------------------------------------------------------------- // // File: RtfControls.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Rtf controls that specify Rtf control and control word information. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { ////// Rtf controls and control word information. /// internal static class RtfControls { // Flags used for controlwords public const uint RTK_TOGGLE = 0x00000001; public const uint RTK_FLAG = 0x00000002; public const uint RTK_VALUE = 0x00000004; public const uint RTK_DESTINATION = 0x00000008; public const uint RTK_SYMBOL = 0x00000010; public const uint RTK_PARAM = 0x00000020; public const uint RTK_TEXT = 0x00000040; public const uint RTK_ACHARPROPS = 0x00000080; public const uint RTK_CHARPROPS = 0x00000100; public const uint RTK_PARAPROPS = 0x00000200; public const uint RTK_OBJPROPS = 0x00000400; public const uint RTK_BOOKMARKPROPS = 0x00000800; public const uint RTK_SECTIONPROPS = 0x00001000; public const uint RTK_DOCPROPS = 0x00002000; public const uint RTK_FILEPROPS = 0x00004000; public const uint RTK_COMMENT = 0x00008000; public const uint RTK_STYLE = 0x00010000; public const uint RTK_FONTTABLE = 0x00020000; public const uint RTK_COLORTABLE = 0x00040000; public const uint RTK_BULLETS = 0x00080000; public const uint RTK_TABLEDEF = 0x00100000; public const uint RTK_SHAPES = 0x00200000; public const uint RTK_POSOBJ = 0x00400000; public const uint RTK_BORDER = 0x00800000; public const uint RTK_SHADING = 0x01000000; public const uint RTK_PICT = 0x02000000; public const uint RTK_FIELD = 0x04000000; public const uint RTK_FORMFIELD = 0x08000000; public const uint RTK_INDEX = 0x10000000; public const uint RTK_DRAWOBJ = 0x20000000; public const uint RTK_INFO = 0x40000000; public const uint RTK_LISTTABLE = 0x80000000; public const uint RTK_PICTPROPS = 0x80000000; public const uint RTK_CHARSET = 0x80000000; public const uint RTK_UNICODE = 0x80000000; public const uint RTK_TABS = 0x80000000; public const uint RTK_FOOTNOTES = 0x80000000; public const uint RTK_HIGHLIGHT = 0x80000000; public const uint RTK_FILETABLE = 0x80000000; public const uint RTK_HEADER = 0x80000000; public const uint RTK_OBJECTS = 0x80000000; public const uint RTK_PARATEXT = 0x80000000; public const uint RTK_SPECIAL = 0x80000000; public const uint RTK_SECTION = 0x80000000; public const uint RTK_TOC = 0x80000000; //----------------------------------------------------- // // Internal Const ControlWordInfo // //----------------------------------------------------- #region Internal Const // Helper function to reduce code size [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static RtfControlWordInfo CreateCommonControlWord(string controlName, uint flags) { return new RtfControlWordInfo(RtfControlWord.Ctrl_Unknown, controlName, flags); } // Helper function to reduce code size [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static RtfControlWordInfo CreateCommonDocPropsControlWord(string controlName) { return new RtfControlWordInfo(RtfControlWord.Ctrl_Unknown, controlName, RTK_DOCPROPS | RTK_FLAG); } // Helper function to reduce code size [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static RtfControlWordInfo CreateCommonDrawObjControlWord(string controlName) { return new RtfControlWordInfo(RtfControlWord.Ctrl_Unknown, controlName, RTK_DRAWOBJ | RTK_FLAG); } internal static RtfControlWordInfo[] ControlTable = { new RtfControlWordInfo(RtfControlWord.Ctrl_AB, "ab", RTK_ACHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ABSH, "absh", RTK_POSOBJ | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ABSLOCK, "abslock", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("absnoovrlp", RTK_POSOBJ | RTK_TOGGLE | RTK_PARAM ), CreateCommonControlWord("absw", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("acaps", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("acccomma", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("accdot", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("accnone", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("acf", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonControlWord("additive", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("adjustright", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("adn", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("aenddoc"), CreateCommonDocPropsControlWord("aendnotes"), CreateCommonControlWord("aexpnd", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonControlWord("af", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonControlWord("affixed", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("afs", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("aftnbj"), CreateCommonControlWord("aftncn", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonDocPropsControlWord("aftnnalc"), CreateCommonDocPropsControlWord("aftnnar"), CreateCommonDocPropsControlWord("aftnnauc"), CreateCommonDocPropsControlWord("aftnnchi"), CreateCommonDocPropsControlWord("aftnnchosung"), CreateCommonDocPropsControlWord("aftnncnum"), CreateCommonDocPropsControlWord("aftnndbar"), CreateCommonDocPropsControlWord("aftnndbnum"), CreateCommonDocPropsControlWord("aftnndbnumd"), CreateCommonDocPropsControlWord("aftnndbnumk"), CreateCommonDocPropsControlWord("aftnndbnumt"), CreateCommonDocPropsControlWord("aftnnganada"), CreateCommonDocPropsControlWord("aftnngbnum"), CreateCommonDocPropsControlWord("aftnngbnumd"), CreateCommonDocPropsControlWord("aftnngbnumk"), CreateCommonDocPropsControlWord("aftnngbnuml"), CreateCommonDocPropsControlWord("aftnnrlc"), CreateCommonDocPropsControlWord("aftnnruc"), CreateCommonDocPropsControlWord("aftnnzodiac"), CreateCommonDocPropsControlWord("aftnnzodiacd"), CreateCommonDocPropsControlWord("aftnnzodiacl"), CreateCommonDocPropsControlWord("aftnrestart"), CreateCommonDocPropsControlWord("aftnrstcont"), CreateCommonControlWord("aftnsep", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("aftnsepc", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("aftnstart", RTK_DOCPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("aftntj"), CreateCommonControlWord("ai", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("alang", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("allprot"), CreateCommonDocPropsControlWord("alntblind"), CreateCommonControlWord("alt", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("animtext", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("annotation", RTK_COMMENT | RTK_DESTINATION ), CreateCommonDocPropsControlWord("annotprot"), new RtfControlWordInfo(RtfControlWord.Ctrl_ANSI, "ansi", RTK_DOCPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_ANSICPG, "ansicpg", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("aoutl", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("ascaps", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("ashad", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("aspalpha", RTK_PARAPROPS | RTK_TOGGLE ), CreateCommonControlWord("aspnum", RTK_PARAPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ASTRIKE, "astrike", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("atnauthor", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atnicn", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atnid", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atnref", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atntime", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atrfend", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atrfstart", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("aul", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("auld", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("auldb", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("aulnone", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("aulw", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("aup", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonControlWord("author", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_B, "b", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("background", RTK_SHAPES | RTK_DESTINATION ), CreateCommonDocPropsControlWord("bdbfhdr"), CreateCommonDocPropsControlWord("bdrrlswsix"), CreateCommonControlWord("bgbdiag", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgcross", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdcross", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkbdiag", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkcross", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkdcross", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkfdiag", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkhoriz", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkvert", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgfdiag", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bghoriz", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgvert", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BIN, "bin", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("binfsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("binsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("bkmkcolf", RTK_BOOKMARKPROPS | RTK_VALUE ), CreateCommonControlWord("bkmkcoll", RTK_BOOKMARKPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_BKMKEND, "bkmkend", RTK_BOOKMARKPROPS | RTK_DESTINATION ), CreateCommonControlWord("bkmkpub", RTK_OBJPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BKMKSTART, "bkmkstart", RTK_BOOKMARKPROPS | RTK_DESTINATION ), CreateCommonControlWord("bliptag", RTK_PICTPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("blipuid", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("blipupi", RTK_PICTPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_BLUE, "blue", RTK_COLORTABLE | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_BOX, "box", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRART, "brdrart", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRB, "brdrb", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRBAR, "brdrbar", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRBTW, "brdrbtw", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRCF, "brdrcf", RTK_PARAPROPS | RTK_BORDER | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDASH, "brdrdash", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDASHD, "brdrdashd", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDASHDD, "brdrdashdd", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDASHDOTSTR, "brdrdashdotstr", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDASHSM, "brdrdashsm", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDB, "brdrdb", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDOT, "brdrdot", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDREMBOSS, "brdremboss", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRENGRAVE, "brdrengrave", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRFRAME, "brdrframe", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRHAIR, "brdrhair", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRINSET, "brdrinset", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRL, "brdrl", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDROUTSET, "brdroutset", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRNIL, "brdrnil", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTBL, "brdrtbl", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRR, "brdrr", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRS, "brdrs", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRSH, "brdrsh", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRT, "brdrt", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTH, "brdrth", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTHTNLG, "brdrthtnlg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTHTNMG, "brdrthtnmg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTHTNSG, "brdrthtnsg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHLG, "brdrtnthlg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHMG, "brdrtnthmg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHSG, "brdrtnthsg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHTNLG, "brdrtnthtnlg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHTNMG, "brdrtnthtnmg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHTNSG, "brdrtnthtnsg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTRIPLE, "brdrtriple", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRW, "brdrw", RTK_PARAPROPS | RTK_BORDER | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRWAVY, "brdrwavy", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRWAVYDB, "brdrwavydb", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), CreateCommonDocPropsControlWord("brkfrm"), new RtfControlWordInfo(RtfControlWord.Ctrl_BRSP, "brsp", RTK_PARAPROPS | RTK_BORDER | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_BULLET, "bullet", RTK_SYMBOL ), CreateCommonControlWord("buptim", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("bxe", RTK_INDEX | RTK_FLAG ), CreateCommonControlWord("caps", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("category", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_CB, "cb", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CBPAT, "cbpat", RTK_PARAPROPS | RTK_SHADING | RTK_VALUE ), CreateCommonControlWord("cchs", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CELL, "cell", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_CELLX, "cellx", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CF, "cf", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CFPAT, "cfpat", RTK_PARAPROPS | RTK_SHADING | RTK_VALUE ), CreateCommonControlWord("cgrid", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("charscalex", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("chatn", RTK_SYMBOL ), CreateCommonControlWord("chbgbdiag", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgcross", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdcross", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkbdiag", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkcross", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkdcross", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkfdiag", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkhoriz", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkvert", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgfdiag", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbghoriz", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgvert", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbrdr", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chcbpat", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("chcfpat", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("chdate", RTK_SYMBOL ), CreateCommonControlWord("chdpa", RTK_SYMBOL ), CreateCommonControlWord("chdpl", RTK_SYMBOL ), CreateCommonControlWord("chftn", RTK_SYMBOL ), CreateCommonControlWord("chftnsep", RTK_SYMBOL ), CreateCommonControlWord("chftnsepc", RTK_SYMBOL ), CreateCommonControlWord("chpgn", RTK_SYMBOL ), CreateCommonControlWord("chshdng", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("chtime", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGBDIAG, "clbgbdiag", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGCROSS, "clbgcross", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDCROSS, "clbgdcross", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKBDIAG, "clbgdkbdiag", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKCROSS, "clbgdkcross", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKDCROSS, "clbgdkdcross", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKFDIAG, "clbgdkfdiag", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKHOR, "clbgdkhor", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKVERT, "clbgdkvert", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGFDIAG, "clbgfdiag", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGHORIZ, "clbghoriz", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGVERT, "clbgvert", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBRDRB, "clbrdrb", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBRDRL, "clbrdrl", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBRDRR, "clbrdrr", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBRDRT, "clbrdrt", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLCBPAT, "clcbpat", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLCFPAT, "clcfpat", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLDGLL, "cldgll", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLDGLU, "cldglu", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLFITTEXT, "clfittext", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLFTSWIDTH, "clftswidth", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLMGF, "clmgf", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLMRG, "clmrg", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLOWRAP, "clowrap", RTK_TABLEDEF | RTK_FLAG | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADB, "clpadb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADFB, "clpadfb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADFL, "clpadfl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADFR, "clpadfr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADFT, "clpadft", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADL, "clpadl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADR, "clpadr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADT, "clpadt", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLSHDNG, "clshdng", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLSHDRAWNIL, "clshdrawnil", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLTXBTLR, "cltxbtlr", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLTXLRTB, "cltxlrtb", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLTXLRTBV, "cltxlrtbv", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLTXTBRL, "cltxtbrl", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLTXTBRLV, "cltxtbrlv", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLVERTALB, "clvertalb", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLVERTALC, "clvertalc", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLVERTALT, "clvertalt", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLVMGF, "clvmgf", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLVMRG, "clvmrg", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLWWIDTH, "clwwidth", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLLAPSED, "collapsed", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLNO, "colno", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLORTBL, "colortbl", RTK_COLORTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLS, "cols", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLSR, "colsr", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLSX, "colsx", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLUMN, "column", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLW, "colw", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("comment", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("company", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("cpg", RTK_CHARSET | RTK_VALUE ), CreateCommonControlWord("crauth", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("crdate", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("creatim", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("cs", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("ctrl", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("cts", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("cufi", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("culi", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("curi", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("cvmme"), CreateCommonControlWord("datafield", RTK_FIELD | RTK_DESTINATION ), CreateCommonControlWord("date", RTK_FIELD | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_DBCH, "dbch", RTK_ACHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_DEFF, "deff", RTK_FONTTABLE | RTK_VALUE ), CreateCommonDocPropsControlWord("defformat"), new RtfControlWordInfo(RtfControlWord.Ctrl_DEFLANG, "deflang", RTK_DOCPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_DEFLANGA, "deflanga", RTK_DOCPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_DEFLANGFE, "deflangfe", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("defshp", RTK_PICT | RTK_FLAG ), CreateCommonControlWord("deftab", RTK_DOCPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_DELETED, "deleted", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("dfrauth", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dfrdate", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dfrmtxtx", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("dfrmtxty", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("dfrstart", RTK_PARATEXT | RTK_VALUE ), CreateCommonControlWord("dfrstop", RTK_PARATEXT | RTK_VALUE ), CreateCommonControlWord("dfrxst", RTK_PARATEXT | RTK_VALUE ), CreateCommonControlWord("dghorigin", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dghshow", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dghspace", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("dgmargin"), CreateCommonDocPropsControlWord("dgsnap"), CreateCommonControlWord("dgvorigin", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dgvshow", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dgvspace", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dibitmap", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("dn", RTK_CHARPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("dntblnsbdb"), new RtfControlWordInfo(RtfControlWord.Ctrl_DO, "do", RTK_DRAWOBJ | RTK_DESTINATION ), CreateCommonDrawObjControlWord("dobxcolumn"), CreateCommonDrawObjControlWord("dobxmargin"), CreateCommonDrawObjControlWord("dobxpage"), CreateCommonDrawObjControlWord("dobymargin"), CreateCommonDrawObjControlWord("dobypage"), CreateCommonDrawObjControlWord("dobypara"), CreateCommonControlWord("doccomm", RTK_INFO | RTK_DESTINATION ), CreateCommonDocPropsControlWord("doctemp"), CreateCommonControlWord("doctype", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("docvar", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("dodhgt", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dolock"), CreateCommonDrawObjControlWord("dpaendhol"), CreateCommonControlWord("dpaendl", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpaendsol"), CreateCommonControlWord("dpaendw", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dparc"), CreateCommonDrawObjControlWord("dparcflipx"), CreateCommonDrawObjControlWord("dparcflipy"), CreateCommonDrawObjControlWord("dpastarthol"), CreateCommonControlWord("dpastartl", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpastartsol"), CreateCommonControlWord("dpastartw", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcallout"), CreateCommonControlWord("dpcoa", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcoaccent"), CreateCommonDrawObjControlWord("dpcobestfit"), CreateCommonDrawObjControlWord("dpcoborder"), CreateCommonControlWord("dpcodabs", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcodbottom"), CreateCommonDrawObjControlWord("dpcodcenter"), CreateCommonControlWord("dpcodescent", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcodtop"), CreateCommonControlWord("dpcolength", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcominusx"), CreateCommonDrawObjControlWord("dpcominusy"), CreateCommonControlWord("dpcooffset", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcosmarta"), CreateCommonDrawObjControlWord("dpcotdouble"), CreateCommonDrawObjControlWord("dpcotright"), CreateCommonDrawObjControlWord("dpcotsingle"), CreateCommonDrawObjControlWord("dpcottriple"), CreateCommonControlWord("dpcount", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpellipse"), CreateCommonDrawObjControlWord("dpendgroup"), CreateCommonControlWord("dpfillbgcb", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillbgcg", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillbgcr", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillbggray", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpfillbgpal"), CreateCommonControlWord("dpfillfgcb", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillfgcg", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillfgcr", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillfggray", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpfillfgpal"), CreateCommonControlWord("dpfillpat", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpgroup"), CreateCommonDrawObjControlWord("dpline"), CreateCommonControlWord("dplinecob", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dplinecog", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dplinecor", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dplinedado"), CreateCommonDrawObjControlWord("dplinedadodo"), CreateCommonDrawObjControlWord("dplinedash"), CreateCommonDrawObjControlWord("dplinedot"), CreateCommonControlWord("dplinegray", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dplinehollow"), CreateCommonDrawObjControlWord("dplinepal"), CreateCommonDrawObjControlWord("dplinesolid"), CreateCommonControlWord("dplinew", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dppolycount", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dppolygon"), CreateCommonDrawObjControlWord("dppolyline"), CreateCommonControlWord("dpptx", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dppty", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dprect"), CreateCommonDrawObjControlWord("dproundr"), CreateCommonDrawObjControlWord("dpshadow"), CreateCommonControlWord("dpshadx", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpshady", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dptxbtlr"), CreateCommonDrawObjControlWord("dptxbx"), CreateCommonControlWord("dptxbxmar", RTK_DRAWOBJ | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_DPTXBXTEXT, "dptxbxtext", RTK_DRAWOBJ | RTK_DESTINATION ), CreateCommonDrawObjControlWord("dptxlrtb"), CreateCommonDrawObjControlWord("dptxlrtbv"), CreateCommonDrawObjControlWord("dptxtbrl"), CreateCommonDrawObjControlWord("dptxtbrlv"), CreateCommonControlWord("dpx", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpxsize", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpy", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpysize", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dropcapli", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("dropcapt", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("ds", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("dxfrtext", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("dy", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("edmins", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("embo", RTK_CHARPROPS | RTK_SHADING | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_EMDASH, "emdash", RTK_SYMBOL ), CreateCommonControlWord("emfblip", RTK_PICT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_EMSPACE, "emspace", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_ENDASH, "endash", RTK_SYMBOL ), CreateCommonDocPropsControlWord("enddoc"), CreateCommonControlWord("endnhere", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("endnotes"), new RtfControlWordInfo(RtfControlWord.Ctrl_ENSPACE, "enspace", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_EXPND, "expnd", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_EXPNDTW, "expndtw", RTK_CHARPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("expshrtn"), new RtfControlWordInfo(RtfControlWord.Ctrl_F, "f", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("faauto", RTK_PARAPROPS | RTK_VALUE ), CreateCommonControlWord("facenter", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("facingp"), CreateCommonControlWord("fahang", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("falt", RTK_FONTTABLE | RTK_DESTINATION ), CreateCommonControlWord("faroman", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("favar", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("fbias", RTK_FONTTABLE | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("fbidi", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("fchars", RTK_DOCPROPS | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FCHARSET, "fcharset", RTK_FONTTABLE | RTK_VALUE ), CreateCommonControlWord("fdecor", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("fet", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("fetch", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("ffdefres", RTK_FORMFIELD | RTK_VALUE ), CreateCommonControlWord("ffdeftext", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffentrymcr", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffexitmcr", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffformat", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffhaslistbox", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffhelptext", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffhps", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffl", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffmaxlen", RTK_FORMFIELD | RTK_VALUE ), CreateCommonControlWord("ffname", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffownhelp", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffownstat", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffprot", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffrecalc", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffres", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffsize", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffstattext", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("fftype", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("fftypetxt", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_FI, "fi", RTK_PARAPROPS | RTK_VALUE ), CreateCommonControlWord("fid", RTK_FILETABLE | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_FIELD, "field", RTK_FIELD | RTK_DESTINATION ), CreateCommonControlWord("file", RTK_FILETABLE | RTK_DESTINATION ), CreateCommonControlWord("filetbl", RTK_FILETABLE | RTK_DESTINATION ), CreateCommonControlWord("fittext", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("fldalt"), CreateCommonControlWord("flddirty", RTK_FIELD | RTK_FLAG ), CreateCommonControlWord("fldedit", RTK_FIELD | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_FLDINST, "fldinst", RTK_FIELD | RTK_DESTINATION ), CreateCommonControlWord("fldlock", RTK_FIELD | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_FLDPRIV, "fldpriv", RTK_FIELD | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_FLDRSLT, "fldrslt", RTK_FIELD | RTK_DESTINATION ), CreateCommonControlWord("fldtype", RTK_FIELD | RTK_DESTINATION ), CreateCommonControlWord("fmodern", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("fn", RTK_STYLE | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_FNAME, "fname", RTK_FONTTABLE | RTK_DESTINATION ), CreateCommonControlWord("fnetwork", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("fnil", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("fontemb", RTK_FONTTABLE | RTK_DESTINATION ), CreateCommonControlWord("fontfile", RTK_FONTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FONTTBL, "fonttbl", RTK_FONTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FOOTER, "footer", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FOOTERF, "footerf", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FOOTERL, "footerl", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FOOTERR, "footerr", RTK_HEADER | RTK_DESTINATION ), CreateCommonControlWord("footery", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("footnote", RTK_FOOTNOTES | RTK_DESTINATION ), CreateCommonDocPropsControlWord("formdisp"), CreateCommonControlWord("formfield", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonDocPropsControlWord("formprot"), CreateCommonDocPropsControlWord("formshade"), CreateCommonControlWord("fosnum", RTK_FILETABLE | RTK_VALUE ), CreateCommonControlWord("fprq", RTK_FONTTABLE | RTK_VALUE ), CreateCommonDocPropsControlWord("fracwidth"), CreateCommonControlWord("frelative", RTK_FILETABLE | RTK_VALUE ), CreateCommonControlWord("frmtxbtlr", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("frmtxlrtb", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("frmtxlrtbv", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("frmtxtbrl", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("frmtxtbrlv", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("froman", RTK_FONTTABLE | RTK_FLAG ), CreateCommonDocPropsControlWord("fromhtml"), CreateCommonDocPropsControlWord("fromtext"), new RtfControlWordInfo(RtfControlWord.Ctrl_FS, "fs", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("fscript", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("fswiss", RTK_FONTTABLE | RTK_FLAG ), CreateCommonDocPropsControlWord("ftnalt"), CreateCommonDocPropsControlWord("ftnbj"), CreateCommonControlWord("ftncn", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("ftnil", RTK_FONTTABLE | RTK_FLAG ), CreateCommonDocPropsControlWord("ftnlytwnine"), CreateCommonDocPropsControlWord("ftnnalc"), CreateCommonDocPropsControlWord("ftnnar"), CreateCommonDocPropsControlWord("ftnnauc"), CreateCommonDocPropsControlWord("ftnnchi"), CreateCommonDocPropsControlWord("ftnnchosung"), CreateCommonDocPropsControlWord("ftnncnum"), CreateCommonDocPropsControlWord("ftnndbar"), CreateCommonDocPropsControlWord("ftnndbnum"), CreateCommonDocPropsControlWord("ftnndbnumd"), CreateCommonDocPropsControlWord("ftnndbnumk"), CreateCommonDocPropsControlWord("ftnndbnumt"), CreateCommonDocPropsControlWord("ftnnganada"), CreateCommonDocPropsControlWord("ftnngbnum"), CreateCommonDocPropsControlWord("ftnngbnumd"), CreateCommonDocPropsControlWord("ftnngbnumk"), CreateCommonDocPropsControlWord("ftnngbnuml"), CreateCommonDocPropsControlWord("ftnnrlc"), CreateCommonDocPropsControlWord("ftnnruc"), CreateCommonDocPropsControlWord("ftnnzodiac"), CreateCommonDocPropsControlWord("ftnnzodiacd"), CreateCommonDocPropsControlWord("ftnnzodiacl"), CreateCommonDocPropsControlWord("ftnrestart"), CreateCommonDocPropsControlWord("ftnrstcont"), CreateCommonDocPropsControlWord("ftnrstpg"), CreateCommonControlWord("ftnsep", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("ftnsepc", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("ftnstart", RTK_DOCPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("ftntj"), CreateCommonControlWord("fttruetype", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("fvaliddos", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("fvalidhpfs", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("fvalidmac", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("fvalidntfs", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("g", RTK_CHARPROPS | RTK_DESTINATION ), CreateCommonControlWord("gcw", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_GREEN, "green", RTK_COLORTABLE | RTK_VALUE ), CreateCommonControlWord("gridtbl", RTK_CHARPROPS | RTK_DESTINATION ), CreateCommonControlWord("gutter", RTK_DOCPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("gutterprl"), CreateCommonControlWord("guttersxn", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_HEADER, "header", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_HEADERF, "headerf", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_HEADERL, "headerl", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_HEADERR, "headerr", RTK_HEADER | RTK_DESTINATION ), CreateCommonControlWord("headery", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_HICH, "hich", RTK_ACHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_HIGHLIGHT, "highlight", RTK_HIGHLIGHT | RTK_VALUE ), CreateCommonControlWord("hlfr", RTK_SHAPES | RTK_VALUE ), CreateCommonControlWord("hlinkbase", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("hlloc", RTK_SHAPES | RTK_VALUE ), CreateCommonControlWord("hlsrc", RTK_SHAPES | RTK_VALUE ), CreateCommonDocPropsControlWord("horzdoc"), CreateCommonControlWord("horzsect", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("hr", RTK_INFO | RTK_VALUE ), CreateCommonDocPropsControlWord("htmautsp"), CreateCommonDocPropsControlWord("htmlbase"), CreateCommonControlWord("htmlrtf", RTK_DOCPROPS | RTK_TOGGLE ), CreateCommonControlWord("htmltag", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("hyphauto", RTK_DOCPROPS | RTK_TOGGLE ), CreateCommonControlWord("hyphcaps", RTK_DOCPROPS | RTK_TOGGLE ), CreateCommonControlWord("hyphconsec", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("hyphhotz", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("hyphpar", RTK_PARAPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_I, "i", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("id", RTK_INFO | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ILVL, "ilvl", RTK_PARATEXT | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_IMPR, "impr", RTK_CHARPROPS | RTK_SHADING | RTK_TOGGLE ), CreateCommonControlWord("info", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_INTBL, "intbl", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_ITAP, "itap", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ixe", RTK_INDEX | RTK_FLAG ), CreateCommonDocPropsControlWord("jcompress"), CreateCommonDocPropsControlWord("jexpand"), new RtfControlWordInfo(RtfControlWord.Ctrl_JPEGBLIP, "jpegblip", RTK_PICT | RTK_FLAG ), CreateCommonDocPropsControlWord("jsksu"), CreateCommonControlWord("keep", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("keepn", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("kerning", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("keycode", RTK_STYLE | RTK_DESTINATION ), CreateCommonControlWord("keywords", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("ksulang", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("landscape"), new RtfControlWordInfo(RtfControlWord.Ctrl_LANG, "lang", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LANGFE, "langfe", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LANGFENP, "langfenp", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LANGNP, "langnp", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("lbr", RTK_SYMBOL | RTK_PARAM ), CreateCommonControlWord("lchars", RTK_DOCPROPS | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LDBLQUOTE, "ldblquote", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVEL, "level", RTK_PARAPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELFOLLOW, "levelfollow", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELINDENT, "levelindent", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELJC, "leveljc", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELJCN, "leveljcn", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELLEGAL, "levellegal", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELNFC, "levelnfc", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELNFCN, "levelnfcn", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELNORESTART, "levelnorestart", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELNUMBERS, "levelnumbers", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELOLD, "levelold", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELPREV, "levelprev", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELPREVSPACE, "levelprevspace", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELSPACE, "levelspace", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELSTARTAT, "levelstartat", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELTEMPLATEID, "leveltemplateid", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELTEXT, "leveltext", RTK_LISTTABLE | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LFOLEVEL, "lfolevel", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LI, "li", RTK_PARAPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LINE, "line", RTK_SYMBOL ), CreateCommonControlWord("linebetcol", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("linecont", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("linemod", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("lineppage", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("linerestart", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("linestart", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("linestarts", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("linex", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("linkself", RTK_OBJECTS | RTK_FLAG ), CreateCommonDocPropsControlWord("linkstyles"), CreateCommonControlWord("linkval", RTK_INFO | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LIN, "lin", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISA, "lisa", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISB, "lisb", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LIST, "list", RTK_LISTTABLE | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTHYBRID, "listhybrid", RTK_LISTTABLE | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTID, "listid", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTLEVEL, "listlevel", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTNAME, "listname", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTOVERRIDE, "listoverride", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTOVERRIDECOUNT, "listoverridecount", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTOVERRIDEFORMAT, "listoverrideformat", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTOVERRIDESTART, "listoverridestart", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTPICTURE, "listpicture", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTRESTARTHDN, "listrestarthdn", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTSIMPLE, "listsimple", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTTABLE, "listtable", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTOVERRIDETABLE, "listoverridetable", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTTEMPLATEID, "listtemplateid", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTTEXT, "listtext", RTK_PARATEXT | RTK_DESTINATION ), CreateCommonDocPropsControlWord("lnbrkrule"), CreateCommonControlWord("lndscpsxn", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("lnongrid"), new RtfControlWordInfo(RtfControlWord.Ctrl_LOCH, "loch", RTK_ACHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LQUOTE, "lquote", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_LS, "ls", RTK_LISTTABLE | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRCH, "ltrch", RTK_CHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRDOC, "ltrdoc", RTK_DOCPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRMARK, "ltrmark", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRPAR, "ltrpar", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRROW, "ltrrow", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRSECT, "ltrsect", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("lytcalctblwd"), CreateCommonDocPropsControlWord("lytexcttp"), CreateCommonDocPropsControlWord("lytprtmet"), CreateCommonDocPropsControlWord("lyttblrtgr"), new RtfControlWordInfo(RtfControlWord.Ctrl_MAC, "mac", RTK_CHARSET | RTK_FLAG ), CreateCommonControlWord("macpict", RTK_PICT | RTK_FLAG ), CreateCommonDocPropsControlWord("makebackup"), CreateCommonControlWord("manager", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("margb", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("margbsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("margl", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("marglsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("margmirror"), CreateCommonControlWord("margr", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("margrsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("margt", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("margtsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("mhtmltag", RTK_DESTINATION ), CreateCommonControlWord("min", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("mo", RTK_INFO | RTK_VALUE ), CreateCommonDocPropsControlWord("msmcap"), new RtfControlWordInfo(RtfControlWord.Ctrl_NESTCELL, "nestcell", RTK_TABLEDEF | RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_NESTROW, "nestrow", RTK_TABLEDEF | RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_NESTTABLEPROPS, "nesttableprops", RTK_TABLEDEF | RTK_DESTINATION ), CreateCommonControlWord("nextfile", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonDocPropsControlWord("nocolbal"), CreateCommonControlWord("nocwrap", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("noextrasprl"), CreateCommonControlWord("nofchars", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("nofcharsws", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("nofpages", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("nofwords", RTK_INFO | RTK_VALUE ), CreateCommonDocPropsControlWord("nolead"), CreateCommonControlWord("noline", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("nolnhtadjtbl"), CreateCommonControlWord("nonesttables", RTK_TABLEDEF | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_NONSHPPICT, "nonshppict", RTK_PICT | RTK_FLAG ), CreateCommonControlWord("nooverflow", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("noproof", RTK_CHARPROPS | RTK_FLAG ), CreateCommonControlWord("nosectexpand", RTK_CHARPROPS | RTK_FLAG ), CreateCommonControlWord("nosnaplinegrid", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("nospaceforul"), new RtfControlWordInfo(RtfControlWord.Ctrl_NOSUPERSUB, "nosupersub", RTK_CHARPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("notabind"), CreateCommonDocPropsControlWord("noultrlspc"), CreateCommonControlWord("nowidctlpar", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("nowrap", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("nowwrap", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("noxlattoyen"), CreateCommonControlWord("objalias", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objalign", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objattph", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objautlink", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objclass", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objcropb", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objcropl", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objcropr", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objcropt", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objdata", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("object", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objemb", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objh", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objhtml", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objicemb", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objlink", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objlock", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objname", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objocx", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objpub", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objscalex", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objscaley", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objsect", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objsetsize", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objsub", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objtime", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objtransy", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objupdate", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objw", RTK_OBJECTS | RTK_VALUE ), CreateCommonDocPropsControlWord("oldas"), CreateCommonDocPropsControlWord("oldlinewrap"), CreateCommonControlWord("operator", RTK_INFO | RTK_DESTINATION ), CreateCommonDocPropsControlWord("otblrul"), new RtfControlWordInfo(RtfControlWord.Ctrl_OUTL, "outl", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_OUTLINELEVEL, "outlinelevel", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("overlay", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PAGE, "page", RTK_SYMBOL ), CreateCommonControlWord("pagebb", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("panose", RTK_FONTTABLE | RTK_DESTINATION ), CreateCommonControlWord("paperh", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("paperw", RTK_DOCPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PAR, "par", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_PARD, "pard", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PC, "pc", RTK_CHARSET | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PCA, "pca", RTK_CHARSET | RTK_FLAG ), CreateCommonDocPropsControlWord("pgbrdrb"), CreateCommonDocPropsControlWord("pgbrdrfoot"), CreateCommonDocPropsControlWord("pgbrdrhead"), CreateCommonDocPropsControlWord("pgbrdrl"), CreateCommonControlWord("pgbrdropt", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("pgbrdrr"), CreateCommonDocPropsControlWord("pgbrdrsnap"), CreateCommonDocPropsControlWord("pgbrdrt"), CreateCommonControlWord("pghsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("pgnbidia", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnbidib", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnchosung", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgncnum", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgncont", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgndbnum", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgndbnumd", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgndbnumk", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgndbnumt", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgndec", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgndecd", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnganada", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgngbnum", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgngbnumd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgngbnumk", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgngbnuml", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgnhn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("pgnhnsc", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnhnsh", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnhnsm", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnhnsn", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnhnsp", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnlcltr", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnlcrm", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnrestart", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnstart", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("pgnstarts", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("pgnucltr", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnucrm", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnx", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("pgny", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("pgnzodiac", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgnzodiacd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgnzodiacl", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgwsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("phcol", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("phmrg", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("phpg", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("picbmp", RTK_PICT | RTK_FLAG ), CreateCommonControlWord("picbpp", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("piccropb", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("piccropl", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("piccropr", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("piccropt", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("pich", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PICHGOAL, "pichgoal", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("picprop", RTK_PICT | RTK_DESTINATION ), CreateCommonControlWord("picscaled", RTK_PICT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PICSCALEX, "picscalex", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PICSCALEY, "picscaley", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PICT, "pict", RTK_PICT | RTK_DESTINATION ), CreateCommonControlWord("picw", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PICWGOAL, "picwgoal", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PLAIN, "plain", RTK_CHARPROPS | RTK_FLAG ), CreateCommonControlWord("pmmetafile", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PN, "pn", RTK_BULLETS | RTK_DESTINATION ), CreateCommonControlWord("pnacross", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnaiu", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnaiud", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnaiueo", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnaiueod", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnb", RTK_BULLETS | RTK_TOGGLE ), CreateCommonControlWord("pnbidia", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNBIDIB, "pnbidib", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pncaps", RTK_BULLETS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNCARD, "pncard", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pncf", RTK_BULLETS | RTK_VALUE ), CreateCommonControlWord("pnchosung", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pncnum", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pndbnum", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pndbnumd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pndbnumk", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pndbnuml", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pndbnumt", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNDEC, "pndec", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNDECD, "pndecd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnf", RTK_BULLETS | RTK_VALUE ), CreateCommonControlWord("pnfs", RTK_BULLETS | RTK_VALUE ), CreateCommonControlWord("pnganada", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNGBLIP, "pngblip", RTK_PICT | RTK_FLAG ), CreateCommonControlWord("pngbnum", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pngbnumd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pngbnumk", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pngbnuml", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNHANG, "pnhang", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNI, "pni", RTK_BULLETS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNINDENT, "pnindent", RTK_BULLETS | RTK_VALUE ), CreateCommonControlWord("pniroha", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnirohad", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLCLTR, "pnlcltr", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLCRM, "pnlcrm", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLVL, "pnlvl", RTK_BULLETS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLVLBLT, "pnlvlblt", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLVLBODY, "pnlvlbody", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLVLCONT, "pnlvlcont", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnnumonce", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNORD, "pnord", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNORDT, "pnordt", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnprev", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNQC, "pnqc", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNQL, "pnql", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNQR, "pnqr", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnrauth", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrdate", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNRESTART, "pnrestart", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnrnfc", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrnot", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), CreateCommonControlWord("pnrpnbr", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrrgb", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrstart", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrstop", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrxst", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnscaps", RTK_BULLETS | RTK_TOGGLE ), CreateCommonControlWord("pnseclvl", RTK_BULLETS | RTK_DESTINATION ), CreateCommonControlWord("pnsp", RTK_BULLETS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNSTART, "pnstart", RTK_BULLETS | RTK_VALUE ), CreateCommonControlWord("pnstrike", RTK_BULLETS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNTEXT, "pntext", RTK_BULLETS | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNTXTA, "pntxta", RTK_BULLETS | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNTXTB, "pntxtb", RTK_BULLETS | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNUCLTR, "pnucltr", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNUCRM, "pnucrm", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnul", RTK_BULLETS | RTK_TOGGLE ), CreateCommonControlWord("pnuld", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnuldash", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnuldashd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnuldashdd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnuldb", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnulhair", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnulnone", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnulth", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnulw", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnulwave", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnzodiac", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnzodiacd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnzodiacl", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("posnegx", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("posnegy", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("posx", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("posxc", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posxi", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posxl", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posxo", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posxr", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posy", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("posyb", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posyc", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posyil", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posyin", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), CreateCommonControlWord("posyout", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), CreateCommonControlWord("posyt", RTK_POSOBJ | RTK_FLAG ), CreateCommonDocPropsControlWord("prcolbl"), CreateCommonDocPropsControlWord("printdata"), CreateCommonControlWord("printim", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("private", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("propname", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("proptype", RTK_INFO | RTK_VALUE ), CreateCommonDocPropsControlWord("psover"), CreateCommonControlWord("psz", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("pubauto", RTK_OBJPROPS | RTK_FLAG ), CreateCommonControlWord("pvmrg", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("pvpara", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("pvpg", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("pwd", RTK_DESTINATION | RTK_PARAM ), CreateCommonControlWord("pxe", RTK_INDEX | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_QC, "qc", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_QD, "qd", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_QJ, "qj", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_QL, "ql", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_QMSPACE, "qmspace", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_QR, "qr", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_RDBLQUOTE, "rdblquote", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_RED, "red", RTK_COLORTABLE | RTK_VALUE ), CreateCommonControlWord("result", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("revauth", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("revauthdel", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("revbar", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("revdttm", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("revdttmdel", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("revised", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonDocPropsControlWord("revisions"), CreateCommonControlWord("revprop", RTK_DOCPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("revprot"), CreateCommonControlWord("revtbl", RTK_DESTINATION ), CreateCommonControlWord("revtim", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_RI, "ri", RTK_PARAPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_RIN, "rin", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_ROW, "row", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_RQUOTE, "rquote", RTK_SYMBOL ), CreateCommonControlWord("rsltbmp", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("rslthtml", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("rsltmerge", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("rsltpict", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("rsltrtf", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("rslttxt", RTK_OBJECTS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_RTF, "rtf", RTK_FILEPROPS ), new RtfControlWordInfo(RtfControlWord.Ctrl_RTLCH, "rtlch", RTK_CHARPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("rtldoc"), CreateCommonDocPropsControlWord("rtlgutter"), new RtfControlWordInfo(RtfControlWord.Ctrl_RTLMARK, "rtlmark", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_RTLPAR, "rtlpar", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_RTLROW, "rtlrow", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_RTLSECT, "rtlsect", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("rxe", RTK_INDEX | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_S, "s", RTK_PARAPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_SA, "sa", RTK_PARAPROPS | RTK_VALUE ), CreateCommonControlWord("saauto", RTK_PARAPROPS | RTK_TOGGLE | RTK_PARAM ), CreateCommonControlWord("sautoupd", RTK_STYLE | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_SB, "sb", RTK_PARAPROPS | RTK_VALUE ), CreateCommonControlWord("sbasedon", RTK_STYLE | RTK_VALUE ), CreateCommonControlWord("sbauto", RTK_PARAPROPS | RTK_TOGGLE | RTK_PARAM ), CreateCommonControlWord("sbkcol", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sbkeven", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sbknone", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sbkodd", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sbkpage", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sbys", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_SCAPS, "scaps", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("scompose", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("sec", RTK_INFO | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_SECT, "sect", RTK_SYMBOL ), CreateCommonControlWord("sectd", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sectdefaultcl", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("sectexpand", RTK_SECTIONPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("sectlinegrid", RTK_SECTIONPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("sectnum", RTK_SYMBOL ), CreateCommonControlWord("sectspecifycl", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("sectspecifygen", RTK_SECTIONPROPS | RTK_FLAG | RTK_PARAM ), CreateCommonControlWord("sectspecifyl", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("sectunlocked", RTK_SECTIONPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_SHAD, "shad", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_SHADING, "shading", RTK_PARAPROPS | RTK_SHADING | RTK_VALUE ), CreateCommonControlWord("shidden", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("shift", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("shpbottom", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shpbxcolumn", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbxignore", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbxmargin", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbxpage", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbyignore", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbymargin", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbypage", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbypara", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpfblwtxt", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shpfhdr", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shpgrp", RTK_SHAPES | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_SHPINST, "shpinst", RTK_SHAPES | RTK_DESTINATION ), CreateCommonControlWord("shpleft", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shplid", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shplockanchor", RTK_SHAPES | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_SHPPICT, "shppict", RTK_PICT | RTK_DESTINATION ), CreateCommonControlWord("shpright", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_SHPRSLT, "shprslt", RTK_SHAPES | RTK_VALUE ), CreateCommonControlWord("shptop", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shptxt", RTK_SHAPES | RTK_VALUE ), CreateCommonControlWord("shpwrk", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shpwr", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shpz", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_SL, "sl", RTK_PARAPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_SLMULT, "slmult", RTK_PARAPROPS | RTK_VALUE ), CreateCommonControlWord("snext", RTK_STYLE | RTK_VALUE ), CreateCommonControlWord("softcol", RTK_SPECIAL | RTK_FLAG ), CreateCommonControlWord("softlheight", RTK_SPECIAL | RTK_VALUE ), CreateCommonControlWord("softline", RTK_SPECIAL | RTK_FLAG ), CreateCommonControlWord("softpage", RTK_SPECIAL | RTK_FLAG ), CreateCommonControlWord("spersonal", RTK_STYLE | RTK_FLAG ), CreateCommonDocPropsControlWord("splytwnine"), CreateCommonDocPropsControlWord("sprsbsp"), CreateCommonDocPropsControlWord("sprslnsp"), CreateCommonDocPropsControlWord("sprsspbf"), CreateCommonDocPropsControlWord("sprstsm"), CreateCommonDocPropsControlWord("sprstsp"), CreateCommonControlWord("sreply", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("staticval", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("stextflow", RTK_SECTION | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_STRIKE, "strike", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_STRIKED, "striked", RTK_CHARPROPS | RTK_SHADING | RTK_TOGGLE ), CreateCommonControlWord("stylesheet", RTK_STYLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_SUB, "sub", RTK_CHARPROPS | RTK_FLAG ), CreateCommonControlWord("subdocument", RTK_PARAPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("subfontbysize"), CreateCommonControlWord("subject", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_SUPER, "super", RTK_CHARPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("swpbdr"), new RtfControlWordInfo(RtfControlWord.Ctrl_TAB, "tab", RTK_SYMBOL ), CreateCommonControlWord("tabsnoovrlp", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("taprtl", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tb", RTK_TABS | RTK_VALUE ), CreateCommonControlWord("tc", RTK_TOC | RTK_DESTINATION ), CreateCommonControlWord("tcelld", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tcf", RTK_TOC | RTK_VALUE ), CreateCommonControlWord("tcl", RTK_TOC | RTK_VALUE ), CreateCommonControlWord("tcn", RTK_TOC | RTK_FLAG ), CreateCommonControlWord("tdfrmtxtbottom", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tdfrmtxtleft", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tdfrmtxtright", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tdfrmtxttop", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("template", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("time", RTK_FIELD | RTK_FLAG ), CreateCommonControlWord("title", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("titlepg", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("tldot", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tleq", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tlhyph", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tlmdot", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tlth", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tlul", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tphcol", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tphmrg", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tphpg", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposnegx", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tposnegy", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tposxc", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposxi", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposxl", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposx", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tposxo", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposxr", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposy", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyb", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyc", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyil", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyin", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyoutv", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyt", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tpvmrg", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tpvpara", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tpvpg", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tqc", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tqdec", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tqr", RTK_TABS | RTK_FLAG ), CreateCommonDocPropsControlWord("transmf"), new RtfControlWordInfo(RtfControlWord.Ctrl_TRAUTOFIT, "trautofit", RTK_TABLEDEF | RTK_TOGGLE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRB, "trbrdrb", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRH, "trbrdrh", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRL, "trbrdrl", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRR, "trbrdrr", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRT, "trbrdrt", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRV, "trbrdrv", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRFTSWIDTHA, "trftswidtha", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRFTSWIDTHB, "trftswidthb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRFTSWIDTH, "trftswidth", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRGAPH, "trgaph", RTK_TABLEDEF | RTK_VALUE ), CreateCommonControlWord("trhdr", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("trkeep", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRLEFT, "trleft", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_TROWD, "trowd", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDB, "trpaddb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDFB, "trpaddfb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDFL, "trpaddfl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDFR, "trpaddfr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDFT, "trpaddft", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDL, "trpaddl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDR, "trpaddr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDT, "trpaddt", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRQC, "trqc", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRQL, "trql", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRQR, "trqr", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRRH, "trrh", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDB, "trspdb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDFB, "trspdfb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDFL, "trspdfl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDFR, "trspdfr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDFT, "trspdft", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDL, "trspdl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDR, "trspdr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDT, "trspdt", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("truncatefontheight"), new RtfControlWordInfo(RtfControlWord.Ctrl_TRWWIDTHA, "trwwidtha", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRWWIDTHB, "trwwidthb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRWWIDTH, "trwwidth", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("twoonone"), CreateCommonControlWord("tx", RTK_TABS | RTK_VALUE ), CreateCommonControlWord("txe", RTK_INDEX | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_UC, "uc", RTK_UNICODE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_UD, "ud", RTK_UNICODE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_UL, "ul", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULC, "ulc", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULD, "uld", RTK_CHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULDASH, "uldash", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULDASHD, "uldashd", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULDASHDD, "uldashdd", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULDB, "uldb", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULHAIR, "ulhair", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULHWAVE, "ulhwave", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULLDASH, "ulldash", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULNONE, "ulnone", RTK_CHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTH, "ulth", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTHD, "ulthd", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTHDASH, "ulthdash", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTHDASHD, "ulthdashd", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTHDASHDD, "ulthdashdd", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTHLDASH, "ulthldash", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULULDBWAVE, "ululdbwave", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULW, "ulw", RTK_CHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULWAVE, "ulwave", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_U, "u", RTK_UNICODE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_UP, "up", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_UPR, "upr", RTK_UNICODE | RTK_DESTINATION ), CreateCommonControlWord("urtf", RTK_DESTINATION | RTK_PARAM ), CreateCommonDocPropsControlWord("useltbaln"), CreateCommonControlWord("userprops", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_V, "v", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("vern", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("version", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("vertalb", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("vertalc", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("vertalj", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("vertalt", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("vertdoc"), CreateCommonControlWord("vertsect", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("viewkind", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("viewscale", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("viewzk", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("wbitmap", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("wbmbitspixel", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("wbmplanes", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("wbmwidthbytes", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("webhidden", RTK_CHARPROPS | RTK_FLAG ), CreateCommonControlWord("widctlpar", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("widowctrl"), CreateCommonControlWord("windowcaption", RTK_DOCPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_WMETAFILE, "wmetafile", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("wpeqn", RTK_FIELD | RTK_FLAG ), CreateCommonDocPropsControlWord("wpjst"), CreateCommonDocPropsControlWord("wpsp"), CreateCommonDocPropsControlWord("wraptrsp"), CreateCommonControlWord("xe", RTK_INDEX | RTK_DESTINATION ), CreateCommonControlWord("xef", RTK_INDEX | RTK_VALUE ), CreateCommonControlWord("yr", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("yxe", RTK_INDEX | RTK_FLAG ), CreateCommonControlWord("zwbo", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_ZWJ, "zwj", RTK_SYMBOL ), CreateCommonControlWord("zwnbo", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_ZWNJ, "zwnj", RTK_SYMBOL) }; #endregion Internal Const } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: RtfControls.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Rtf controls that specify Rtf control and control word information. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { ////// Rtf controls and control word information. /// internal static class RtfControls { // Flags used for controlwords public const uint RTK_TOGGLE = 0x00000001; public const uint RTK_FLAG = 0x00000002; public const uint RTK_VALUE = 0x00000004; public const uint RTK_DESTINATION = 0x00000008; public const uint RTK_SYMBOL = 0x00000010; public const uint RTK_PARAM = 0x00000020; public const uint RTK_TEXT = 0x00000040; public const uint RTK_ACHARPROPS = 0x00000080; public const uint RTK_CHARPROPS = 0x00000100; public const uint RTK_PARAPROPS = 0x00000200; public const uint RTK_OBJPROPS = 0x00000400; public const uint RTK_BOOKMARKPROPS = 0x00000800; public const uint RTK_SECTIONPROPS = 0x00001000; public const uint RTK_DOCPROPS = 0x00002000; public const uint RTK_FILEPROPS = 0x00004000; public const uint RTK_COMMENT = 0x00008000; public const uint RTK_STYLE = 0x00010000; public const uint RTK_FONTTABLE = 0x00020000; public const uint RTK_COLORTABLE = 0x00040000; public const uint RTK_BULLETS = 0x00080000; public const uint RTK_TABLEDEF = 0x00100000; public const uint RTK_SHAPES = 0x00200000; public const uint RTK_POSOBJ = 0x00400000; public const uint RTK_BORDER = 0x00800000; public const uint RTK_SHADING = 0x01000000; public const uint RTK_PICT = 0x02000000; public const uint RTK_FIELD = 0x04000000; public const uint RTK_FORMFIELD = 0x08000000; public const uint RTK_INDEX = 0x10000000; public const uint RTK_DRAWOBJ = 0x20000000; public const uint RTK_INFO = 0x40000000; public const uint RTK_LISTTABLE = 0x80000000; public const uint RTK_PICTPROPS = 0x80000000; public const uint RTK_CHARSET = 0x80000000; public const uint RTK_UNICODE = 0x80000000; public const uint RTK_TABS = 0x80000000; public const uint RTK_FOOTNOTES = 0x80000000; public const uint RTK_HIGHLIGHT = 0x80000000; public const uint RTK_FILETABLE = 0x80000000; public const uint RTK_HEADER = 0x80000000; public const uint RTK_OBJECTS = 0x80000000; public const uint RTK_PARATEXT = 0x80000000; public const uint RTK_SPECIAL = 0x80000000; public const uint RTK_SECTION = 0x80000000; public const uint RTK_TOC = 0x80000000; //----------------------------------------------------- // // Internal Const ControlWordInfo // //----------------------------------------------------- #region Internal Const // Helper function to reduce code size [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static RtfControlWordInfo CreateCommonControlWord(string controlName, uint flags) { return new RtfControlWordInfo(RtfControlWord.Ctrl_Unknown, controlName, flags); } // Helper function to reduce code size [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static RtfControlWordInfo CreateCommonDocPropsControlWord(string controlName) { return new RtfControlWordInfo(RtfControlWord.Ctrl_Unknown, controlName, RTK_DOCPROPS | RTK_FLAG); } // Helper function to reduce code size [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] private static RtfControlWordInfo CreateCommonDrawObjControlWord(string controlName) { return new RtfControlWordInfo(RtfControlWord.Ctrl_Unknown, controlName, RTK_DRAWOBJ | RTK_FLAG); } internal static RtfControlWordInfo[] ControlTable = { new RtfControlWordInfo(RtfControlWord.Ctrl_AB, "ab", RTK_ACHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ABSH, "absh", RTK_POSOBJ | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ABSLOCK, "abslock", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("absnoovrlp", RTK_POSOBJ | RTK_TOGGLE | RTK_PARAM ), CreateCommonControlWord("absw", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("acaps", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("acccomma", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("accdot", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("accnone", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("acf", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonControlWord("additive", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("adjustright", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("adn", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("aenddoc"), CreateCommonDocPropsControlWord("aendnotes"), CreateCommonControlWord("aexpnd", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonControlWord("af", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonControlWord("affixed", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("afs", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("aftnbj"), CreateCommonControlWord("aftncn", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonDocPropsControlWord("aftnnalc"), CreateCommonDocPropsControlWord("aftnnar"), CreateCommonDocPropsControlWord("aftnnauc"), CreateCommonDocPropsControlWord("aftnnchi"), CreateCommonDocPropsControlWord("aftnnchosung"), CreateCommonDocPropsControlWord("aftnncnum"), CreateCommonDocPropsControlWord("aftnndbar"), CreateCommonDocPropsControlWord("aftnndbnum"), CreateCommonDocPropsControlWord("aftnndbnumd"), CreateCommonDocPropsControlWord("aftnndbnumk"), CreateCommonDocPropsControlWord("aftnndbnumt"), CreateCommonDocPropsControlWord("aftnnganada"), CreateCommonDocPropsControlWord("aftnngbnum"), CreateCommonDocPropsControlWord("aftnngbnumd"), CreateCommonDocPropsControlWord("aftnngbnumk"), CreateCommonDocPropsControlWord("aftnngbnuml"), CreateCommonDocPropsControlWord("aftnnrlc"), CreateCommonDocPropsControlWord("aftnnruc"), CreateCommonDocPropsControlWord("aftnnzodiac"), CreateCommonDocPropsControlWord("aftnnzodiacd"), CreateCommonDocPropsControlWord("aftnnzodiacl"), CreateCommonDocPropsControlWord("aftnrestart"), CreateCommonDocPropsControlWord("aftnrstcont"), CreateCommonControlWord("aftnsep", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("aftnsepc", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("aftnstart", RTK_DOCPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("aftntj"), CreateCommonControlWord("ai", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("alang", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("allprot"), CreateCommonDocPropsControlWord("alntblind"), CreateCommonControlWord("alt", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("animtext", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("annotation", RTK_COMMENT | RTK_DESTINATION ), CreateCommonDocPropsControlWord("annotprot"), new RtfControlWordInfo(RtfControlWord.Ctrl_ANSI, "ansi", RTK_DOCPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_ANSICPG, "ansicpg", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("aoutl", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("ascaps", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("ashad", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("aspalpha", RTK_PARAPROPS | RTK_TOGGLE ), CreateCommonControlWord("aspnum", RTK_PARAPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ASTRIKE, "astrike", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("atnauthor", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atnicn", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atnid", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atnref", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atntime", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atrfend", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("atrfstart", RTK_COMMENT | RTK_DESTINATION ), CreateCommonControlWord("aul", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("auld", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("auldb", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("aulnone", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("aulw", RTK_ACHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("aup", RTK_ACHARPROPS | RTK_VALUE ), CreateCommonControlWord("author", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_B, "b", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("background", RTK_SHAPES | RTK_DESTINATION ), CreateCommonDocPropsControlWord("bdbfhdr"), CreateCommonDocPropsControlWord("bdrrlswsix"), CreateCommonControlWord("bgbdiag", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgcross", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdcross", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkbdiag", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkcross", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkdcross", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkfdiag", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkhoriz", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgdkvert", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgfdiag", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bghoriz", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("bgvert", RTK_PARAPROPS | RTK_SHADING | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BIN, "bin", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("binfsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("binsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("bkmkcolf", RTK_BOOKMARKPROPS | RTK_VALUE ), CreateCommonControlWord("bkmkcoll", RTK_BOOKMARKPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_BKMKEND, "bkmkend", RTK_BOOKMARKPROPS | RTK_DESTINATION ), CreateCommonControlWord("bkmkpub", RTK_OBJPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BKMKSTART, "bkmkstart", RTK_BOOKMARKPROPS | RTK_DESTINATION ), CreateCommonControlWord("bliptag", RTK_PICTPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("blipuid", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("blipupi", RTK_PICTPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_BLUE, "blue", RTK_COLORTABLE | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_BOX, "box", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRART, "brdrart", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRB, "brdrb", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRBAR, "brdrbar", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRBTW, "brdrbtw", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRCF, "brdrcf", RTK_PARAPROPS | RTK_BORDER | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDASH, "brdrdash", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDASHD, "brdrdashd", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDASHDD, "brdrdashdd", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDASHDOTSTR, "brdrdashdotstr", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDASHSM, "brdrdashsm", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDB, "brdrdb", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRDOT, "brdrdot", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDREMBOSS, "brdremboss", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRENGRAVE, "brdrengrave", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRFRAME, "brdrframe", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRHAIR, "brdrhair", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRINSET, "brdrinset", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRL, "brdrl", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDROUTSET, "brdroutset", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRNIL, "brdrnil", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTBL, "brdrtbl", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRR, "brdrr", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRS, "brdrs", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRSH, "brdrsh", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRT, "brdrt", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTH, "brdrth", RTK_PARAPROPS | RTK_BORDER | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTHTNLG, "brdrthtnlg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTHTNMG, "brdrthtnmg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTHTNSG, "brdrthtnsg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHLG, "brdrtnthlg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHMG, "brdrtnthmg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHSG, "brdrtnthsg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHTNLG, "brdrtnthtnlg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHTNMG, "brdrtnthtnmg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTNTHTNSG, "brdrtnthtnsg", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRTRIPLE, "brdrtriple", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRW, "brdrw", RTK_PARAPROPS | RTK_BORDER | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRWAVY, "brdrwavy", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_BRDRWAVYDB, "brdrwavydb", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), CreateCommonDocPropsControlWord("brkfrm"), new RtfControlWordInfo(RtfControlWord.Ctrl_BRSP, "brsp", RTK_PARAPROPS | RTK_BORDER | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_BULLET, "bullet", RTK_SYMBOL ), CreateCommonControlWord("buptim", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("bxe", RTK_INDEX | RTK_FLAG ), CreateCommonControlWord("caps", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("category", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_CB, "cb", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CBPAT, "cbpat", RTK_PARAPROPS | RTK_SHADING | RTK_VALUE ), CreateCommonControlWord("cchs", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CELL, "cell", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_CELLX, "cellx", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CF, "cf", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CFPAT, "cfpat", RTK_PARAPROPS | RTK_SHADING | RTK_VALUE ), CreateCommonControlWord("cgrid", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("charscalex", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("chatn", RTK_SYMBOL ), CreateCommonControlWord("chbgbdiag", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgcross", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdcross", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkbdiag", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkcross", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkdcross", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkfdiag", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkhoriz", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgdkvert", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgfdiag", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbghoriz", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbgvert", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chbrdr", RTK_CHARPROPS | RTK_SHADING | RTK_FLAG ), CreateCommonControlWord("chcbpat", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("chcfpat", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("chdate", RTK_SYMBOL ), CreateCommonControlWord("chdpa", RTK_SYMBOL ), CreateCommonControlWord("chdpl", RTK_SYMBOL ), CreateCommonControlWord("chftn", RTK_SYMBOL ), CreateCommonControlWord("chftnsep", RTK_SYMBOL ), CreateCommonControlWord("chftnsepc", RTK_SYMBOL ), CreateCommonControlWord("chpgn", RTK_SYMBOL ), CreateCommonControlWord("chshdng", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("chtime", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGBDIAG, "clbgbdiag", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGCROSS, "clbgcross", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDCROSS, "clbgdcross", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKBDIAG, "clbgdkbdiag", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKCROSS, "clbgdkcross", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKDCROSS, "clbgdkdcross", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKFDIAG, "clbgdkfdiag", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKHOR, "clbgdkhor", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGDKVERT, "clbgdkvert", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGFDIAG, "clbgfdiag", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGHORIZ, "clbghoriz", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBGVERT, "clbgvert", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBRDRB, "clbrdrb", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBRDRL, "clbrdrl", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBRDRR, "clbrdrr", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLBRDRT, "clbrdrt", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLCBPAT, "clcbpat", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLCFPAT, "clcfpat", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLDGLL, "cldgll", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLDGLU, "cldglu", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLFITTEXT, "clfittext", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLFTSWIDTH, "clftswidth", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLMGF, "clmgf", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLMRG, "clmrg", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLOWRAP, "clowrap", RTK_TABLEDEF | RTK_FLAG | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADB, "clpadb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADFB, "clpadfb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADFL, "clpadfl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADFR, "clpadfr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADFT, "clpadft", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADL, "clpadl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADR, "clpadr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLPADT, "clpadt", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLSHDNG, "clshdng", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLSHDRAWNIL, "clshdrawnil", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLTXBTLR, "cltxbtlr", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLTXLRTB, "cltxlrtb", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLTXLRTBV, "cltxlrtbv", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLTXTBRL, "cltxtbrl", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLTXTBRLV, "cltxtbrlv", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLVERTALB, "clvertalb", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLVERTALC, "clvertalc", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLVERTALT, "clvertalt", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLVMGF, "clvmgf", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLVMRG, "clvmrg", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_CLWWIDTH, "clwwidth", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLLAPSED, "collapsed", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLNO, "colno", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLORTBL, "colortbl", RTK_COLORTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLS, "cols", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLSR, "colsr", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLSX, "colsx", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLUMN, "column", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_COLW, "colw", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("comment", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("company", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("cpg", RTK_CHARSET | RTK_VALUE ), CreateCommonControlWord("crauth", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("crdate", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("creatim", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("cs", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("ctrl", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("cts", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("cufi", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("culi", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("curi", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("cvmme"), CreateCommonControlWord("datafield", RTK_FIELD | RTK_DESTINATION ), CreateCommonControlWord("date", RTK_FIELD | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_DBCH, "dbch", RTK_ACHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_DEFF, "deff", RTK_FONTTABLE | RTK_VALUE ), CreateCommonDocPropsControlWord("defformat"), new RtfControlWordInfo(RtfControlWord.Ctrl_DEFLANG, "deflang", RTK_DOCPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_DEFLANGA, "deflanga", RTK_DOCPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_DEFLANGFE, "deflangfe", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("defshp", RTK_PICT | RTK_FLAG ), CreateCommonControlWord("deftab", RTK_DOCPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_DELETED, "deleted", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("dfrauth", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dfrdate", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dfrmtxtx", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("dfrmtxty", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("dfrstart", RTK_PARATEXT | RTK_VALUE ), CreateCommonControlWord("dfrstop", RTK_PARATEXT | RTK_VALUE ), CreateCommonControlWord("dfrxst", RTK_PARATEXT | RTK_VALUE ), CreateCommonControlWord("dghorigin", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dghshow", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dghspace", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("dgmargin"), CreateCommonDocPropsControlWord("dgsnap"), CreateCommonControlWord("dgvorigin", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dgvshow", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dgvspace", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("dibitmap", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("dn", RTK_CHARPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("dntblnsbdb"), new RtfControlWordInfo(RtfControlWord.Ctrl_DO, "do", RTK_DRAWOBJ | RTK_DESTINATION ), CreateCommonDrawObjControlWord("dobxcolumn"), CreateCommonDrawObjControlWord("dobxmargin"), CreateCommonDrawObjControlWord("dobxpage"), CreateCommonDrawObjControlWord("dobymargin"), CreateCommonDrawObjControlWord("dobypage"), CreateCommonDrawObjControlWord("dobypara"), CreateCommonControlWord("doccomm", RTK_INFO | RTK_DESTINATION ), CreateCommonDocPropsControlWord("doctemp"), CreateCommonControlWord("doctype", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("docvar", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("dodhgt", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dolock"), CreateCommonDrawObjControlWord("dpaendhol"), CreateCommonControlWord("dpaendl", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpaendsol"), CreateCommonControlWord("dpaendw", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dparc"), CreateCommonDrawObjControlWord("dparcflipx"), CreateCommonDrawObjControlWord("dparcflipy"), CreateCommonDrawObjControlWord("dpastarthol"), CreateCommonControlWord("dpastartl", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpastartsol"), CreateCommonControlWord("dpastartw", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcallout"), CreateCommonControlWord("dpcoa", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcoaccent"), CreateCommonDrawObjControlWord("dpcobestfit"), CreateCommonDrawObjControlWord("dpcoborder"), CreateCommonControlWord("dpcodabs", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcodbottom"), CreateCommonDrawObjControlWord("dpcodcenter"), CreateCommonControlWord("dpcodescent", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcodtop"), CreateCommonControlWord("dpcolength", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcominusx"), CreateCommonDrawObjControlWord("dpcominusy"), CreateCommonControlWord("dpcooffset", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpcosmarta"), CreateCommonDrawObjControlWord("dpcotdouble"), CreateCommonDrawObjControlWord("dpcotright"), CreateCommonDrawObjControlWord("dpcotsingle"), CreateCommonDrawObjControlWord("dpcottriple"), CreateCommonControlWord("dpcount", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpellipse"), CreateCommonDrawObjControlWord("dpendgroup"), CreateCommonControlWord("dpfillbgcb", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillbgcg", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillbgcr", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillbggray", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpfillbgpal"), CreateCommonControlWord("dpfillfgcb", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillfgcg", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillfgcr", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpfillfggray", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpfillfgpal"), CreateCommonControlWord("dpfillpat", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dpgroup"), CreateCommonDrawObjControlWord("dpline"), CreateCommonControlWord("dplinecob", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dplinecog", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dplinecor", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dplinedado"), CreateCommonDrawObjControlWord("dplinedadodo"), CreateCommonDrawObjControlWord("dplinedash"), CreateCommonDrawObjControlWord("dplinedot"), CreateCommonControlWord("dplinegray", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dplinehollow"), CreateCommonDrawObjControlWord("dplinepal"), CreateCommonDrawObjControlWord("dplinesolid"), CreateCommonControlWord("dplinew", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dppolycount", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dppolygon"), CreateCommonDrawObjControlWord("dppolyline"), CreateCommonControlWord("dpptx", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dppty", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dprect"), CreateCommonDrawObjControlWord("dproundr"), CreateCommonDrawObjControlWord("dpshadow"), CreateCommonControlWord("dpshadx", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpshady", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonDrawObjControlWord("dptxbtlr"), CreateCommonDrawObjControlWord("dptxbx"), CreateCommonControlWord("dptxbxmar", RTK_DRAWOBJ | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_DPTXBXTEXT, "dptxbxtext", RTK_DRAWOBJ | RTK_DESTINATION ), CreateCommonDrawObjControlWord("dptxlrtb"), CreateCommonDrawObjControlWord("dptxlrtbv"), CreateCommonDrawObjControlWord("dptxtbrl"), CreateCommonDrawObjControlWord("dptxtbrlv"), CreateCommonControlWord("dpx", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpxsize", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpy", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dpysize", RTK_DRAWOBJ | RTK_VALUE ), CreateCommonControlWord("dropcapli", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("dropcapt", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("ds", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("dxfrtext", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("dy", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("edmins", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("embo", RTK_CHARPROPS | RTK_SHADING | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_EMDASH, "emdash", RTK_SYMBOL ), CreateCommonControlWord("emfblip", RTK_PICT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_EMSPACE, "emspace", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_ENDASH, "endash", RTK_SYMBOL ), CreateCommonDocPropsControlWord("enddoc"), CreateCommonControlWord("endnhere", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("endnotes"), new RtfControlWordInfo(RtfControlWord.Ctrl_ENSPACE, "enspace", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_EXPND, "expnd", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_EXPNDTW, "expndtw", RTK_CHARPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("expshrtn"), new RtfControlWordInfo(RtfControlWord.Ctrl_F, "f", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("faauto", RTK_PARAPROPS | RTK_VALUE ), CreateCommonControlWord("facenter", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("facingp"), CreateCommonControlWord("fahang", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("falt", RTK_FONTTABLE | RTK_DESTINATION ), CreateCommonControlWord("faroman", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("favar", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("fbias", RTK_FONTTABLE | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("fbidi", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("fchars", RTK_DOCPROPS | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FCHARSET, "fcharset", RTK_FONTTABLE | RTK_VALUE ), CreateCommonControlWord("fdecor", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("fet", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("fetch", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("ffdefres", RTK_FORMFIELD | RTK_VALUE ), CreateCommonControlWord("ffdeftext", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffentrymcr", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffexitmcr", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffformat", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffhaslistbox", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffhelptext", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffhps", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffl", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffmaxlen", RTK_FORMFIELD | RTK_VALUE ), CreateCommonControlWord("ffname", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("ffownhelp", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffownstat", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffprot", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffrecalc", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffres", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffsize", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ffstattext", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonControlWord("fftype", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("fftypetxt", RTK_FORMFIELD | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_FI, "fi", RTK_PARAPROPS | RTK_VALUE ), CreateCommonControlWord("fid", RTK_FILETABLE | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_FIELD, "field", RTK_FIELD | RTK_DESTINATION ), CreateCommonControlWord("file", RTK_FILETABLE | RTK_DESTINATION ), CreateCommonControlWord("filetbl", RTK_FILETABLE | RTK_DESTINATION ), CreateCommonControlWord("fittext", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("fldalt"), CreateCommonControlWord("flddirty", RTK_FIELD | RTK_FLAG ), CreateCommonControlWord("fldedit", RTK_FIELD | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_FLDINST, "fldinst", RTK_FIELD | RTK_DESTINATION ), CreateCommonControlWord("fldlock", RTK_FIELD | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_FLDPRIV, "fldpriv", RTK_FIELD | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_FLDRSLT, "fldrslt", RTK_FIELD | RTK_DESTINATION ), CreateCommonControlWord("fldtype", RTK_FIELD | RTK_DESTINATION ), CreateCommonControlWord("fmodern", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("fn", RTK_STYLE | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_FNAME, "fname", RTK_FONTTABLE | RTK_DESTINATION ), CreateCommonControlWord("fnetwork", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("fnil", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("fontemb", RTK_FONTTABLE | RTK_DESTINATION ), CreateCommonControlWord("fontfile", RTK_FONTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FONTTBL, "fonttbl", RTK_FONTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FOOTER, "footer", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FOOTERF, "footerf", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FOOTERL, "footerl", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_FOOTERR, "footerr", RTK_HEADER | RTK_DESTINATION ), CreateCommonControlWord("footery", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("footnote", RTK_FOOTNOTES | RTK_DESTINATION ), CreateCommonDocPropsControlWord("formdisp"), CreateCommonControlWord("formfield", RTK_FORMFIELD | RTK_DESTINATION ), CreateCommonDocPropsControlWord("formprot"), CreateCommonDocPropsControlWord("formshade"), CreateCommonControlWord("fosnum", RTK_FILETABLE | RTK_VALUE ), CreateCommonControlWord("fprq", RTK_FONTTABLE | RTK_VALUE ), CreateCommonDocPropsControlWord("fracwidth"), CreateCommonControlWord("frelative", RTK_FILETABLE | RTK_VALUE ), CreateCommonControlWord("frmtxbtlr", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("frmtxlrtb", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("frmtxlrtbv", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("frmtxtbrl", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("frmtxtbrlv", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("froman", RTK_FONTTABLE | RTK_FLAG ), CreateCommonDocPropsControlWord("fromhtml"), CreateCommonDocPropsControlWord("fromtext"), new RtfControlWordInfo(RtfControlWord.Ctrl_FS, "fs", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("fscript", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("fswiss", RTK_FONTTABLE | RTK_FLAG ), CreateCommonDocPropsControlWord("ftnalt"), CreateCommonDocPropsControlWord("ftnbj"), CreateCommonControlWord("ftncn", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("ftnil", RTK_FONTTABLE | RTK_FLAG ), CreateCommonDocPropsControlWord("ftnlytwnine"), CreateCommonDocPropsControlWord("ftnnalc"), CreateCommonDocPropsControlWord("ftnnar"), CreateCommonDocPropsControlWord("ftnnauc"), CreateCommonDocPropsControlWord("ftnnchi"), CreateCommonDocPropsControlWord("ftnnchosung"), CreateCommonDocPropsControlWord("ftnncnum"), CreateCommonDocPropsControlWord("ftnndbar"), CreateCommonDocPropsControlWord("ftnndbnum"), CreateCommonDocPropsControlWord("ftnndbnumd"), CreateCommonDocPropsControlWord("ftnndbnumk"), CreateCommonDocPropsControlWord("ftnndbnumt"), CreateCommonDocPropsControlWord("ftnnganada"), CreateCommonDocPropsControlWord("ftnngbnum"), CreateCommonDocPropsControlWord("ftnngbnumd"), CreateCommonDocPropsControlWord("ftnngbnumk"), CreateCommonDocPropsControlWord("ftnngbnuml"), CreateCommonDocPropsControlWord("ftnnrlc"), CreateCommonDocPropsControlWord("ftnnruc"), CreateCommonDocPropsControlWord("ftnnzodiac"), CreateCommonDocPropsControlWord("ftnnzodiacd"), CreateCommonDocPropsControlWord("ftnnzodiacl"), CreateCommonDocPropsControlWord("ftnrestart"), CreateCommonDocPropsControlWord("ftnrstcont"), CreateCommonDocPropsControlWord("ftnrstpg"), CreateCommonControlWord("ftnsep", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("ftnsepc", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("ftnstart", RTK_DOCPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("ftntj"), CreateCommonControlWord("fttruetype", RTK_FONTTABLE | RTK_FLAG ), CreateCommonControlWord("fvaliddos", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("fvalidhpfs", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("fvalidmac", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("fvalidntfs", RTK_FILETABLE | RTK_FLAG ), CreateCommonControlWord("g", RTK_CHARPROPS | RTK_DESTINATION ), CreateCommonControlWord("gcw", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_GREEN, "green", RTK_COLORTABLE | RTK_VALUE ), CreateCommonControlWord("gridtbl", RTK_CHARPROPS | RTK_DESTINATION ), CreateCommonControlWord("gutter", RTK_DOCPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("gutterprl"), CreateCommonControlWord("guttersxn", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_HEADER, "header", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_HEADERF, "headerf", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_HEADERL, "headerl", RTK_HEADER | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_HEADERR, "headerr", RTK_HEADER | RTK_DESTINATION ), CreateCommonControlWord("headery", RTK_SECTIONPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_HICH, "hich", RTK_ACHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_HIGHLIGHT, "highlight", RTK_HIGHLIGHT | RTK_VALUE ), CreateCommonControlWord("hlfr", RTK_SHAPES | RTK_VALUE ), CreateCommonControlWord("hlinkbase", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("hlloc", RTK_SHAPES | RTK_VALUE ), CreateCommonControlWord("hlsrc", RTK_SHAPES | RTK_VALUE ), CreateCommonDocPropsControlWord("horzdoc"), CreateCommonControlWord("horzsect", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("hr", RTK_INFO | RTK_VALUE ), CreateCommonDocPropsControlWord("htmautsp"), CreateCommonDocPropsControlWord("htmlbase"), CreateCommonControlWord("htmlrtf", RTK_DOCPROPS | RTK_TOGGLE ), CreateCommonControlWord("htmltag", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("hyphauto", RTK_DOCPROPS | RTK_TOGGLE ), CreateCommonControlWord("hyphcaps", RTK_DOCPROPS | RTK_TOGGLE ), CreateCommonControlWord("hyphconsec", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("hyphhotz", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("hyphpar", RTK_PARAPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_I, "i", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("id", RTK_INFO | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ILVL, "ilvl", RTK_PARATEXT | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_IMPR, "impr", RTK_CHARPROPS | RTK_SHADING | RTK_TOGGLE ), CreateCommonControlWord("info", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_INTBL, "intbl", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_ITAP, "itap", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("ixe", RTK_INDEX | RTK_FLAG ), CreateCommonDocPropsControlWord("jcompress"), CreateCommonDocPropsControlWord("jexpand"), new RtfControlWordInfo(RtfControlWord.Ctrl_JPEGBLIP, "jpegblip", RTK_PICT | RTK_FLAG ), CreateCommonDocPropsControlWord("jsksu"), CreateCommonControlWord("keep", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("keepn", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("kerning", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("keycode", RTK_STYLE | RTK_DESTINATION ), CreateCommonControlWord("keywords", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("ksulang", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("landscape"), new RtfControlWordInfo(RtfControlWord.Ctrl_LANG, "lang", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LANGFE, "langfe", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LANGFENP, "langfenp", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LANGNP, "langnp", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("lbr", RTK_SYMBOL | RTK_PARAM ), CreateCommonControlWord("lchars", RTK_DOCPROPS | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LDBLQUOTE, "ldblquote", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVEL, "level", RTK_PARAPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELFOLLOW, "levelfollow", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELINDENT, "levelindent", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELJC, "leveljc", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELJCN, "leveljcn", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELLEGAL, "levellegal", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELNFC, "levelnfc", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELNFCN, "levelnfcn", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELNORESTART, "levelnorestart", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELNUMBERS, "levelnumbers", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELOLD, "levelold", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELPREV, "levelprev", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELPREVSPACE, "levelprevspace", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELSPACE, "levelspace", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELSTARTAT, "levelstartat", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELTEMPLATEID, "leveltemplateid", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LEVELTEXT, "leveltext", RTK_LISTTABLE | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LFOLEVEL, "lfolevel", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LI, "li", RTK_PARAPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LINE, "line", RTK_SYMBOL ), CreateCommonControlWord("linebetcol", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("linecont", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("linemod", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("lineppage", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("linerestart", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("linestart", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("linestarts", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("linex", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("linkself", RTK_OBJECTS | RTK_FLAG ), CreateCommonDocPropsControlWord("linkstyles"), CreateCommonControlWord("linkval", RTK_INFO | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LIN, "lin", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISA, "lisa", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISB, "lisb", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LIST, "list", RTK_LISTTABLE | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTHYBRID, "listhybrid", RTK_LISTTABLE | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTID, "listid", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTLEVEL, "listlevel", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTNAME, "listname", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTOVERRIDE, "listoverride", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTOVERRIDECOUNT, "listoverridecount", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTOVERRIDEFORMAT, "listoverrideformat", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTOVERRIDESTART, "listoverridestart", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTPICTURE, "listpicture", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTRESTARTHDN, "listrestarthdn", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTSIMPLE, "listsimple", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTTABLE, "listtable", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTOVERRIDETABLE, "listoverridetable", RTK_LISTTABLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTTEMPLATEID, "listtemplateid", RTK_LISTTABLE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_LISTTEXT, "listtext", RTK_PARATEXT | RTK_DESTINATION ), CreateCommonDocPropsControlWord("lnbrkrule"), CreateCommonControlWord("lndscpsxn", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("lnongrid"), new RtfControlWordInfo(RtfControlWord.Ctrl_LOCH, "loch", RTK_ACHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LQUOTE, "lquote", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_LS, "ls", RTK_LISTTABLE | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRCH, "ltrch", RTK_CHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRDOC, "ltrdoc", RTK_DOCPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRMARK, "ltrmark", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRPAR, "ltrpar", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRROW, "ltrrow", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_LTRSECT, "ltrsect", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("lytcalctblwd"), CreateCommonDocPropsControlWord("lytexcttp"), CreateCommonDocPropsControlWord("lytprtmet"), CreateCommonDocPropsControlWord("lyttblrtgr"), new RtfControlWordInfo(RtfControlWord.Ctrl_MAC, "mac", RTK_CHARSET | RTK_FLAG ), CreateCommonControlWord("macpict", RTK_PICT | RTK_FLAG ), CreateCommonDocPropsControlWord("makebackup"), CreateCommonControlWord("manager", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("margb", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("margbsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("margl", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("marglsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("margmirror"), CreateCommonControlWord("margr", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("margrsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("margt", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("margtsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("mhtmltag", RTK_DESTINATION ), CreateCommonControlWord("min", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("mo", RTK_INFO | RTK_VALUE ), CreateCommonDocPropsControlWord("msmcap"), new RtfControlWordInfo(RtfControlWord.Ctrl_NESTCELL, "nestcell", RTK_TABLEDEF | RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_NESTROW, "nestrow", RTK_TABLEDEF | RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_NESTTABLEPROPS, "nesttableprops", RTK_TABLEDEF | RTK_DESTINATION ), CreateCommonControlWord("nextfile", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonDocPropsControlWord("nocolbal"), CreateCommonControlWord("nocwrap", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("noextrasprl"), CreateCommonControlWord("nofchars", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("nofcharsws", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("nofpages", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("nofwords", RTK_INFO | RTK_VALUE ), CreateCommonDocPropsControlWord("nolead"), CreateCommonControlWord("noline", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("nolnhtadjtbl"), CreateCommonControlWord("nonesttables", RTK_TABLEDEF | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_NONSHPPICT, "nonshppict", RTK_PICT | RTK_FLAG ), CreateCommonControlWord("nooverflow", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("noproof", RTK_CHARPROPS | RTK_FLAG ), CreateCommonControlWord("nosectexpand", RTK_CHARPROPS | RTK_FLAG ), CreateCommonControlWord("nosnaplinegrid", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("nospaceforul"), new RtfControlWordInfo(RtfControlWord.Ctrl_NOSUPERSUB, "nosupersub", RTK_CHARPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("notabind"), CreateCommonDocPropsControlWord("noultrlspc"), CreateCommonControlWord("nowidctlpar", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("nowrap", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("nowwrap", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("noxlattoyen"), CreateCommonControlWord("objalias", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objalign", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objattph", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objautlink", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objclass", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objcropb", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objcropl", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objcropr", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objcropt", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objdata", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("object", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objemb", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objh", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objhtml", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objicemb", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objlink", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objlock", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objname", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objocx", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objpub", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objscalex", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objscaley", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objsect", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objsetsize", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objsub", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objtime", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("objtransy", RTK_OBJECTS | RTK_VALUE ), CreateCommonControlWord("objupdate", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("objw", RTK_OBJECTS | RTK_VALUE ), CreateCommonDocPropsControlWord("oldas"), CreateCommonDocPropsControlWord("oldlinewrap"), CreateCommonControlWord("operator", RTK_INFO | RTK_DESTINATION ), CreateCommonDocPropsControlWord("otblrul"), new RtfControlWordInfo(RtfControlWord.Ctrl_OUTL, "outl", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_OUTLINELEVEL, "outlinelevel", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("overlay", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PAGE, "page", RTK_SYMBOL ), CreateCommonControlWord("pagebb", RTK_PARAPROPS | RTK_FLAG ), CreateCommonControlWord("panose", RTK_FONTTABLE | RTK_DESTINATION ), CreateCommonControlWord("paperh", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("paperw", RTK_DOCPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PAR, "par", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_PARD, "pard", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PC, "pc", RTK_CHARSET | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PCA, "pca", RTK_CHARSET | RTK_FLAG ), CreateCommonDocPropsControlWord("pgbrdrb"), CreateCommonDocPropsControlWord("pgbrdrfoot"), CreateCommonDocPropsControlWord("pgbrdrhead"), CreateCommonDocPropsControlWord("pgbrdrl"), CreateCommonControlWord("pgbrdropt", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("pgbrdrr"), CreateCommonDocPropsControlWord("pgbrdrsnap"), CreateCommonDocPropsControlWord("pgbrdrt"), CreateCommonControlWord("pghsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("pgnbidia", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnbidib", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnchosung", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgncnum", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgncont", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgndbnum", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgndbnumd", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgndbnumk", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgndbnumt", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgndec", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgndecd", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnganada", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgngbnum", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgngbnumd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgngbnumk", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgngbnuml", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgnhn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("pgnhnsc", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnhnsh", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnhnsm", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnhnsn", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnhnsp", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnlcltr", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnlcrm", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnrestart", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnstart", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("pgnstarts", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("pgnucltr", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnucrm", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("pgnx", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("pgny", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("pgnzodiac", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgnzodiacd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgnzodiacl", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pgwsxn", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("phcol", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("phmrg", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("phpg", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("picbmp", RTK_PICT | RTK_FLAG ), CreateCommonControlWord("picbpp", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("piccropb", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("piccropl", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("piccropr", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("piccropt", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("pich", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PICHGOAL, "pichgoal", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("picprop", RTK_PICT | RTK_DESTINATION ), CreateCommonControlWord("picscaled", RTK_PICT | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PICSCALEX, "picscalex", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PICSCALEY, "picscaley", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PICT, "pict", RTK_PICT | RTK_DESTINATION ), CreateCommonControlWord("picw", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PICWGOAL, "picwgoal", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PLAIN, "plain", RTK_CHARPROPS | RTK_FLAG ), CreateCommonControlWord("pmmetafile", RTK_PICTPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PN, "pn", RTK_BULLETS | RTK_DESTINATION ), CreateCommonControlWord("pnacross", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnaiu", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnaiud", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnaiueo", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnaiueod", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnb", RTK_BULLETS | RTK_TOGGLE ), CreateCommonControlWord("pnbidia", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNBIDIB, "pnbidib", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pncaps", RTK_BULLETS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNCARD, "pncard", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pncf", RTK_BULLETS | RTK_VALUE ), CreateCommonControlWord("pnchosung", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pncnum", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pndbnum", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pndbnumd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pndbnumk", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pndbnuml", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pndbnumt", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNDEC, "pndec", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNDECD, "pndecd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnf", RTK_BULLETS | RTK_VALUE ), CreateCommonControlWord("pnfs", RTK_BULLETS | RTK_VALUE ), CreateCommonControlWord("pnganada", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNGBLIP, "pngblip", RTK_PICT | RTK_FLAG ), CreateCommonControlWord("pngbnum", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pngbnumd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pngbnumk", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pngbnuml", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNHANG, "pnhang", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNI, "pni", RTK_BULLETS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNINDENT, "pnindent", RTK_BULLETS | RTK_VALUE ), CreateCommonControlWord("pniroha", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnirohad", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLCLTR, "pnlcltr", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLCRM, "pnlcrm", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLVL, "pnlvl", RTK_BULLETS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLVLBLT, "pnlvlblt", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLVLBODY, "pnlvlbody", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNLVLCONT, "pnlvlcont", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnnumonce", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNORD, "pnord", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNORDT, "pnordt", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnprev", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNQC, "pnqc", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNQL, "pnql", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNQR, "pnqr", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnrauth", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrdate", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNRESTART, "pnrestart", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnrnfc", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrnot", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), CreateCommonControlWord("pnrpnbr", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrrgb", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrstart", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrstop", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnrxst", RTK_PARATEXT | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("pnscaps", RTK_BULLETS | RTK_TOGGLE ), CreateCommonControlWord("pnseclvl", RTK_BULLETS | RTK_DESTINATION ), CreateCommonControlWord("pnsp", RTK_BULLETS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNSTART, "pnstart", RTK_BULLETS | RTK_VALUE ), CreateCommonControlWord("pnstrike", RTK_BULLETS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNTEXT, "pntext", RTK_BULLETS | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNTXTA, "pntxta", RTK_BULLETS | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNTXTB, "pntxtb", RTK_BULLETS | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNUCLTR, "pnucltr", RTK_BULLETS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_PNUCRM, "pnucrm", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnul", RTK_BULLETS | RTK_TOGGLE ), CreateCommonControlWord("pnuld", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnuldash", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnuldashd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnuldashdd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnuldb", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnulhair", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnulnone", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnulth", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnulw", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnulwave", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnzodiac", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnzodiacd", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("pnzodiacl", RTK_BULLETS | RTK_FLAG ), CreateCommonControlWord("posnegx", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("posnegy", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("posx", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("posxc", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posxi", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posxl", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posxo", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posxr", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posy", RTK_POSOBJ | RTK_VALUE ), CreateCommonControlWord("posyb", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posyc", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posyil", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("posyin", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), CreateCommonControlWord("posyout", RTK_PARAPROPS | RTK_TEXT | RTK_FLAG ), CreateCommonControlWord("posyt", RTK_POSOBJ | RTK_FLAG ), CreateCommonDocPropsControlWord("prcolbl"), CreateCommonDocPropsControlWord("printdata"), CreateCommonControlWord("printim", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("private", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("propname", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("proptype", RTK_INFO | RTK_VALUE ), CreateCommonDocPropsControlWord("psover"), CreateCommonControlWord("psz", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("pubauto", RTK_OBJPROPS | RTK_FLAG ), CreateCommonControlWord("pvmrg", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("pvpara", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("pvpg", RTK_POSOBJ | RTK_FLAG ), CreateCommonControlWord("pwd", RTK_DESTINATION | RTK_PARAM ), CreateCommonControlWord("pxe", RTK_INDEX | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_QC, "qc", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_QD, "qd", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_QJ, "qj", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_QL, "ql", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_QMSPACE, "qmspace", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_QR, "qr", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_RDBLQUOTE, "rdblquote", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_RED, "red", RTK_COLORTABLE | RTK_VALUE ), CreateCommonControlWord("result", RTK_OBJECTS | RTK_DESTINATION ), CreateCommonControlWord("revauth", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("revauthdel", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("revbar", RTK_DOCPROPS | RTK_VALUE ), CreateCommonControlWord("revdttm", RTK_CHARPROPS | RTK_VALUE ), CreateCommonControlWord("revdttmdel", RTK_CHARPROPS | RTK_SHADING | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("revised", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonDocPropsControlWord("revisions"), CreateCommonControlWord("revprop", RTK_DOCPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("revprot"), CreateCommonControlWord("revtbl", RTK_DESTINATION ), CreateCommonControlWord("revtim", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_RI, "ri", RTK_PARAPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_RIN, "rin", RTK_PARAPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_ROW, "row", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_RQUOTE, "rquote", RTK_SYMBOL ), CreateCommonControlWord("rsltbmp", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("rslthtml", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("rsltmerge", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("rsltpict", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("rsltrtf", RTK_OBJECTS | RTK_FLAG ), CreateCommonControlWord("rslttxt", RTK_OBJECTS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_RTF, "rtf", RTK_FILEPROPS ), new RtfControlWordInfo(RtfControlWord.Ctrl_RTLCH, "rtlch", RTK_CHARPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("rtldoc"), CreateCommonDocPropsControlWord("rtlgutter"), new RtfControlWordInfo(RtfControlWord.Ctrl_RTLMARK, "rtlmark", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_RTLPAR, "rtlpar", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_RTLROW, "rtlrow", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_RTLSECT, "rtlsect", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("rxe", RTK_INDEX | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_S, "s", RTK_PARAPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_SA, "sa", RTK_PARAPROPS | RTK_VALUE ), CreateCommonControlWord("saauto", RTK_PARAPROPS | RTK_TOGGLE | RTK_PARAM ), CreateCommonControlWord("sautoupd", RTK_STYLE | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_SB, "sb", RTK_PARAPROPS | RTK_VALUE ), CreateCommonControlWord("sbasedon", RTK_STYLE | RTK_VALUE ), CreateCommonControlWord("sbauto", RTK_PARAPROPS | RTK_TOGGLE | RTK_PARAM ), CreateCommonControlWord("sbkcol", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sbkeven", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sbknone", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sbkodd", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sbkpage", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sbys", RTK_PARAPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_SCAPS, "scaps", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("scompose", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("sec", RTK_INFO | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_SECT, "sect", RTK_SYMBOL ), CreateCommonControlWord("sectd", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("sectdefaultcl", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("sectexpand", RTK_SECTIONPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("sectlinegrid", RTK_SECTIONPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("sectnum", RTK_SYMBOL ), CreateCommonControlWord("sectspecifycl", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("sectspecifygen", RTK_SECTIONPROPS | RTK_FLAG | RTK_PARAM ), CreateCommonControlWord("sectspecifyl", RTK_SECTIONPROPS | RTK_VALUE ), CreateCommonControlWord("sectunlocked", RTK_SECTIONPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_SHAD, "shad", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_SHADING, "shading", RTK_PARAPROPS | RTK_SHADING | RTK_VALUE ), CreateCommonControlWord("shidden", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("shift", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("shpbottom", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shpbxcolumn", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbxignore", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbxmargin", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbxpage", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbyignore", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbymargin", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbypage", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpbypara", RTK_SHAPES | RTK_FLAG ), CreateCommonControlWord("shpfblwtxt", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shpfhdr", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shpgrp", RTK_SHAPES | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_SHPINST, "shpinst", RTK_SHAPES | RTK_DESTINATION ), CreateCommonControlWord("shpleft", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shplid", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shplockanchor", RTK_SHAPES | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_SHPPICT, "shppict", RTK_PICT | RTK_DESTINATION ), CreateCommonControlWord("shpright", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_SHPRSLT, "shprslt", RTK_SHAPES | RTK_VALUE ), CreateCommonControlWord("shptop", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shptxt", RTK_SHAPES | RTK_VALUE ), CreateCommonControlWord("shpwrk", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shpwr", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("shpz", RTK_SHAPES | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_SL, "sl", RTK_PARAPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_SLMULT, "slmult", RTK_PARAPROPS | RTK_VALUE ), CreateCommonControlWord("snext", RTK_STYLE | RTK_VALUE ), CreateCommonControlWord("softcol", RTK_SPECIAL | RTK_FLAG ), CreateCommonControlWord("softlheight", RTK_SPECIAL | RTK_VALUE ), CreateCommonControlWord("softline", RTK_SPECIAL | RTK_FLAG ), CreateCommonControlWord("softpage", RTK_SPECIAL | RTK_FLAG ), CreateCommonControlWord("spersonal", RTK_STYLE | RTK_FLAG ), CreateCommonDocPropsControlWord("splytwnine"), CreateCommonDocPropsControlWord("sprsbsp"), CreateCommonDocPropsControlWord("sprslnsp"), CreateCommonDocPropsControlWord("sprsspbf"), CreateCommonDocPropsControlWord("sprstsm"), CreateCommonDocPropsControlWord("sprstsp"), CreateCommonControlWord("sreply", RTK_STYLE | RTK_FLAG ), CreateCommonControlWord("staticval", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("stextflow", RTK_SECTION | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_STRIKE, "strike", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_STRIKED, "striked", RTK_CHARPROPS | RTK_SHADING | RTK_TOGGLE ), CreateCommonControlWord("stylesheet", RTK_STYLE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_SUB, "sub", RTK_CHARPROPS | RTK_FLAG ), CreateCommonControlWord("subdocument", RTK_PARAPROPS | RTK_VALUE ), CreateCommonDocPropsControlWord("subfontbysize"), CreateCommonControlWord("subject", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_SUPER, "super", RTK_CHARPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("swpbdr"), new RtfControlWordInfo(RtfControlWord.Ctrl_TAB, "tab", RTK_SYMBOL ), CreateCommonControlWord("tabsnoovrlp", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("taprtl", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tb", RTK_TABS | RTK_VALUE ), CreateCommonControlWord("tc", RTK_TOC | RTK_DESTINATION ), CreateCommonControlWord("tcelld", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tcf", RTK_TOC | RTK_VALUE ), CreateCommonControlWord("tcl", RTK_TOC | RTK_VALUE ), CreateCommonControlWord("tcn", RTK_TOC | RTK_FLAG ), CreateCommonControlWord("tdfrmtxtbottom", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tdfrmtxtleft", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tdfrmtxtright", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tdfrmtxttop", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("template", RTK_DOCPROPS | RTK_DESTINATION ), CreateCommonControlWord("time", RTK_FIELD | RTK_FLAG ), CreateCommonControlWord("title", RTK_INFO | RTK_DESTINATION ), CreateCommonControlWord("titlepg", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("tldot", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tleq", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tlhyph", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tlmdot", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tlth", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tlul", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tphcol", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tphmrg", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tphpg", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposnegx", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tposnegy", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tposxc", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposxi", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposxl", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposx", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("tposxo", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposxr", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposy", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyb", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyc", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyil", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyin", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyoutv", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tposyt", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tpvmrg", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tpvpara", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tpvpg", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("tqc", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tqdec", RTK_TABS | RTK_FLAG ), CreateCommonControlWord("tqr", RTK_TABS | RTK_FLAG ), CreateCommonDocPropsControlWord("transmf"), new RtfControlWordInfo(RtfControlWord.Ctrl_TRAUTOFIT, "trautofit", RTK_TABLEDEF | RTK_TOGGLE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRB, "trbrdrb", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRH, "trbrdrh", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRL, "trbrdrl", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRR, "trbrdrr", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRT, "trbrdrt", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRBRDRV, "trbrdrv", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRFTSWIDTHA, "trftswidtha", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRFTSWIDTHB, "trftswidthb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRFTSWIDTH, "trftswidth", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRGAPH, "trgaph", RTK_TABLEDEF | RTK_VALUE ), CreateCommonControlWord("trhdr", RTK_TABLEDEF | RTK_FLAG ), CreateCommonControlWord("trkeep", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRLEFT, "trleft", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_TROWD, "trowd", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDB, "trpaddb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDFB, "trpaddfb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDFL, "trpaddfl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDFR, "trpaddfr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDFT, "trpaddft", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDL, "trpaddl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDR, "trpaddr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRPADDT, "trpaddt", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRQC, "trqc", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRQL, "trql", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRQR, "trqr", RTK_TABLEDEF | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRRH, "trrh", RTK_TABLEDEF | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDB, "trspdb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDFB, "trspdfb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDFL, "trspdfl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDFR, "trspdfr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDFT, "trspdft", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDL, "trspdl", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDR, "trspdr", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRSPDT, "trspdt", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("truncatefontheight"), new RtfControlWordInfo(RtfControlWord.Ctrl_TRWWIDTHA, "trwwidtha", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRWWIDTHB, "trwwidthb", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_TRWWIDTH, "trwwidth", RTK_TABLEDEF | RTK_VALUE | RTK_PARAM ), CreateCommonDocPropsControlWord("twoonone"), CreateCommonControlWord("tx", RTK_TABS | RTK_VALUE ), CreateCommonControlWord("txe", RTK_INDEX | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_UC, "uc", RTK_UNICODE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_UD, "ud", RTK_UNICODE | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_UL, "ul", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULC, "ulc", RTK_CHARPROPS | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULD, "uld", RTK_CHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULDASH, "uldash", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULDASHD, "uldashd", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULDASHDD, "uldashdd", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULDB, "uldb", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULHAIR, "ulhair", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULHWAVE, "ulhwave", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULLDASH, "ulldash", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULNONE, "ulnone", RTK_CHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTH, "ulth", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTHD, "ulthd", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTHDASH, "ulthdash", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTHDASHD, "ulthdashd", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTHDASHDD, "ulthdashdd", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULTHLDASH, "ulthldash", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULULDBWAVE, "ululdbwave", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULW, "ulw", RTK_CHARPROPS | RTK_FLAG ), new RtfControlWordInfo(RtfControlWord.Ctrl_ULWAVE, "ulwave", RTK_CHARPROPS | RTK_TOGGLE ), new RtfControlWordInfo(RtfControlWord.Ctrl_U, "u", RTK_UNICODE | RTK_VALUE | RTK_PARAM ), new RtfControlWordInfo(RtfControlWord.Ctrl_UP, "up", RTK_CHARPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_UPR, "upr", RTK_UNICODE | RTK_DESTINATION ), CreateCommonControlWord("urtf", RTK_DESTINATION | RTK_PARAM ), CreateCommonDocPropsControlWord("useltbaln"), CreateCommonControlWord("userprops", RTK_INFO | RTK_DESTINATION ), new RtfControlWordInfo(RtfControlWord.Ctrl_V, "v", RTK_CHARPROPS | RTK_TOGGLE ), CreateCommonControlWord("vern", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("version", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("vertalb", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("vertalc", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("vertalj", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("vertalt", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("vertdoc"), CreateCommonControlWord("vertsect", RTK_SECTIONPROPS | RTK_FLAG ), CreateCommonControlWord("viewkind", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("viewscale", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("viewzk", RTK_DOCPROPS | RTK_VALUE | RTK_PARAM ), CreateCommonControlWord("wbitmap", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("wbmbitspixel", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("wbmplanes", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("wbmwidthbytes", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("webhidden", RTK_CHARPROPS | RTK_FLAG ), CreateCommonControlWord("widctlpar", RTK_PARAPROPS | RTK_FLAG ), CreateCommonDocPropsControlWord("widowctrl"), CreateCommonControlWord("windowcaption", RTK_DOCPROPS | RTK_VALUE ), new RtfControlWordInfo(RtfControlWord.Ctrl_WMETAFILE, "wmetafile", RTK_PICTPROPS | RTK_VALUE ), CreateCommonControlWord("wpeqn", RTK_FIELD | RTK_FLAG ), CreateCommonDocPropsControlWord("wpjst"), CreateCommonDocPropsControlWord("wpsp"), CreateCommonDocPropsControlWord("wraptrsp"), CreateCommonControlWord("xe", RTK_INDEX | RTK_DESTINATION ), CreateCommonControlWord("xef", RTK_INDEX | RTK_VALUE ), CreateCommonControlWord("yr", RTK_INFO | RTK_VALUE ), CreateCommonControlWord("yxe", RTK_INDEX | RTK_FLAG ), CreateCommonControlWord("zwbo", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_ZWJ, "zwj", RTK_SYMBOL ), CreateCommonControlWord("zwnbo", RTK_SYMBOL ), new RtfControlWordInfo(RtfControlWord.Ctrl_ZWNJ, "zwnj", RTK_SYMBOL) }; #endregion Internal Const } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
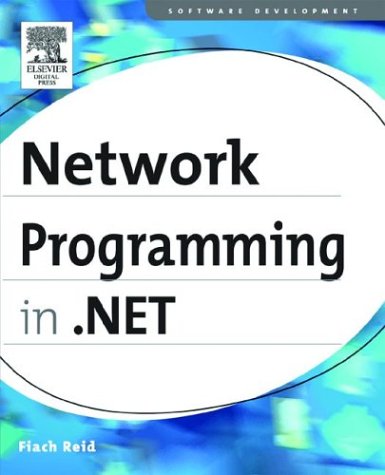
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartEditVerb.cs
- LazyTextWriterCreator.cs
- XmlSchemaAppInfo.cs
- StylusPointPropertyInfo.cs
- ObjectSerializerFactory.cs
- DataGridViewTextBoxEditingControl.cs
- HtmlString.cs
- SchemaDeclBase.cs
- ToolStripItemCollection.cs
- ContainerSelectorBehavior.cs
- XmlSchemaSimpleContent.cs
- X509PeerCertificateAuthenticationElement.cs
- ErrorStyle.cs
- CodeParameterDeclarationExpressionCollection.cs
- NegationPusher.cs
- TouchEventArgs.cs
- TypeConverterHelper.cs
- RegionInfo.cs
- SettingsContext.cs
- DashStyles.cs
- ResolveNameEventArgs.cs
- ZipFileInfo.cs
- MenuRendererClassic.cs
- DocumentGrid.cs
- Activator.cs
- AsyncPostBackTrigger.cs
- SafeFindHandle.cs
- DateTimePickerDesigner.cs
- ExpressionConverter.cs
- Screen.cs
- RelAssertionDirectKeyIdentifierClause.cs
- ProjectionCamera.cs
- _HTTPDateParse.cs
- ByteAnimationUsingKeyFrames.cs
- ImageCodecInfoPrivate.cs
- SqlDependencyListener.cs
- SafeBitVector32.cs
- rsa.cs
- XmlBufferReader.cs
- GeometryGroup.cs
- SqlProfileProvider.cs
- OleDbConnection.cs
- LineBreak.cs
- ProjectionPlan.cs
- RectangleF.cs
- TabPage.cs
- Part.cs
- RichTextBox.cs
- RelationshipManager.cs
- XmlSchemaAttributeGroupRef.cs
- ContourSegment.cs
- Models.cs
- VerticalAlignConverter.cs
- UnsafePeerToPeerMethods.cs
- DataConnectionHelper.cs
- SoapAttributeOverrides.cs
- tooltip.cs
- RowUpdatedEventArgs.cs
- SerializationInfo.cs
- FontConverter.cs
- CommandEventArgs.cs
- TextBoxView.cs
- TraceHandler.cs
- UnwrappedTypesXmlSerializerManager.cs
- ReflectionTypeLoadException.cs
- CryptoConfig.cs
- Int32CollectionConverter.cs
- CustomLineCap.cs
- MostlySingletonList.cs
- StructuredTypeInfo.cs
- FontClient.cs
- ParseChildrenAsPropertiesAttribute.cs
- Vector3DKeyFrameCollection.cs
- SortKey.cs
- AggregateNode.cs
- EmptyEnumerator.cs
- CodeNamespaceImportCollection.cs
- FieldAccessException.cs
- TemplatePagerField.cs
- HtmlInputFile.cs
- RestClientProxyHandler.cs
- EncodingNLS.cs
- SpanIndex.cs
- HMACSHA256.cs
- IntegerValidator.cs
- DataPointer.cs
- HandlerFactoryWrapper.cs
- BufferModeSettings.cs
- SymbolEqualComparer.cs
- DebuggerService.cs
- ExceptionHandlers.cs
- Hashtable.cs
- CLRBindingWorker.cs
- RadioButton.cs
- BuildProvider.cs
- DrawingGroupDrawingContext.cs
- InternalTypeHelper.cs
- Bold.cs
- SecUtil.cs
- LoginCancelEventArgs.cs