Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Configuration / System / Configuration / PropertyInformation.cs / 1 / PropertyInformation.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration; using System.Collections.Specialized; using System.ComponentModel; using System.Collections; using System.Runtime.Serialization; namespace System.Configuration { // PropertyInformation // // Contains information about a property // public sealed class PropertyInformation { private ConfigurationElement ThisElement = null; private string PropertyName; private ConfigurationProperty _Prop = null; private const string LockAll = "*"; private ConfigurationProperty Prop { get { if (_Prop == null) { _Prop = ThisElement.Properties[PropertyName]; } return _Prop; } } internal PropertyInformation(ConfigurationElement thisElement, string propertyName) { PropertyName = propertyName; ThisElement = thisElement; } public string Name { get { return PropertyName; } } internal string ProvidedName { get { return Prop.ProvidedName; } } public object Value { get { return ThisElement[PropertyName]; } set { ThisElement[PropertyName] = value; } } // DefaultValue // // What is the default value for this property // public object DefaultValue { get { return Prop.DefaultValue; } } // ValueOrigin // // Where was the property retrieved from // public PropertyValueOrigin ValueOrigin { get { if (ThisElement.Values[PropertyName] == null) { return PropertyValueOrigin.Default; } if (ThisElement.Values.IsInherited(PropertyName)) { return PropertyValueOrigin.Inherited; } return PropertyValueOrigin.SetHere; } } // IsModified // // Was the property Modified // public bool IsModified { get { if (ThisElement.Values[PropertyName] == null) { return false; } if (ThisElement.Values.IsModified(PropertyName)) { return true; } return false; } } // IsKey // // Is this property a key? // public bool IsKey { get { return Prop.IsKey; } } // IsRequired // // Is this property required? // public bool IsRequired { get { return Prop.IsRequired; } } // IsLocked // // Is this property locked? // public bool IsLocked { get { return ((ThisElement.LockedAllExceptAttributesList != null && !ThisElement.LockedAllExceptAttributesList.DefinedInParent(PropertyName)) || (ThisElement.LockedAttributesList != null && (ThisElement.LockedAttributesList.DefinedInParent(PropertyName) || ThisElement.LockedAttributesList.DefinedInParent(LockAll))) || (((ThisElement.ItemLocked & ConfigurationValueFlags.Locked) != 0) && ((ThisElement.ItemLocked & ConfigurationValueFlags.Inherited) != 0))); } } // Source // // What is the source file where this data came from // public string Source { get { PropertySourceInfo psi = ThisElement.Values.GetSourceInfo(PropertyName); if (psi == null) { psi = ThisElement.Values.GetSourceInfo(String.Empty); } if (psi == null) { return String.Empty; } return psi.FileName; } } // LineNumber // // What is the line number associated with the source // // Note: // 1 is the first line in the file. 0 is returned when there is no // source // public int LineNumber { get { PropertySourceInfo psi = ThisElement.Values.GetSourceInfo(PropertyName); if (psi == null) { psi = ThisElement.Values.GetSourceInfo(String.Empty); } if (psi == null) { return 0; } return psi.LineNumber; } } // Type // // What is the type for the property // public Type Type { get { return Prop.Type; } } // Validator // public ConfigurationValidatorBase Validator { get { return Prop.Validator; } } // Converter // public TypeConverter Converter { get { return Prop.Converter; } } // Property description ( comments etc ) public string Description { get { return Prop.Description; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Configuration; using System.Collections.Specialized; using System.ComponentModel; using System.Collections; using System.Runtime.Serialization; namespace System.Configuration { // PropertyInformation // // Contains information about a property // public sealed class PropertyInformation { private ConfigurationElement ThisElement = null; private string PropertyName; private ConfigurationProperty _Prop = null; private const string LockAll = "*"; private ConfigurationProperty Prop { get { if (_Prop == null) { _Prop = ThisElement.Properties[PropertyName]; } return _Prop; } } internal PropertyInformation(ConfigurationElement thisElement, string propertyName) { PropertyName = propertyName; ThisElement = thisElement; } public string Name { get { return PropertyName; } } internal string ProvidedName { get { return Prop.ProvidedName; } } public object Value { get { return ThisElement[PropertyName]; } set { ThisElement[PropertyName] = value; } } // DefaultValue // // What is the default value for this property // public object DefaultValue { get { return Prop.DefaultValue; } } // ValueOrigin // // Where was the property retrieved from // public PropertyValueOrigin ValueOrigin { get { if (ThisElement.Values[PropertyName] == null) { return PropertyValueOrigin.Default; } if (ThisElement.Values.IsInherited(PropertyName)) { return PropertyValueOrigin.Inherited; } return PropertyValueOrigin.SetHere; } } // IsModified // // Was the property Modified // public bool IsModified { get { if (ThisElement.Values[PropertyName] == null) { return false; } if (ThisElement.Values.IsModified(PropertyName)) { return true; } return false; } } // IsKey // // Is this property a key? // public bool IsKey { get { return Prop.IsKey; } } // IsRequired // // Is this property required? // public bool IsRequired { get { return Prop.IsRequired; } } // IsLocked // // Is this property locked? // public bool IsLocked { get { return ((ThisElement.LockedAllExceptAttributesList != null && !ThisElement.LockedAllExceptAttributesList.DefinedInParent(PropertyName)) || (ThisElement.LockedAttributesList != null && (ThisElement.LockedAttributesList.DefinedInParent(PropertyName) || ThisElement.LockedAttributesList.DefinedInParent(LockAll))) || (((ThisElement.ItemLocked & ConfigurationValueFlags.Locked) != 0) && ((ThisElement.ItemLocked & ConfigurationValueFlags.Inherited) != 0))); } } // Source // // What is the source file where this data came from // public string Source { get { PropertySourceInfo psi = ThisElement.Values.GetSourceInfo(PropertyName); if (psi == null) { psi = ThisElement.Values.GetSourceInfo(String.Empty); } if (psi == null) { return String.Empty; } return psi.FileName; } } // LineNumber // // What is the line number associated with the source // // Note: // 1 is the first line in the file. 0 is returned when there is no // source // public int LineNumber { get { PropertySourceInfo psi = ThisElement.Values.GetSourceInfo(PropertyName); if (psi == null) { psi = ThisElement.Values.GetSourceInfo(String.Empty); } if (psi == null) { return 0; } return psi.LineNumber; } } // Type // // What is the type for the property // public Type Type { get { return Prop.Type; } } // Validator // public ConfigurationValidatorBase Validator { get { return Prop.Validator; } } // Converter // public TypeConverter Converter { get { return Prop.Converter; } } // Property description ( comments etc ) public string Description { get { return Prop.Description; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
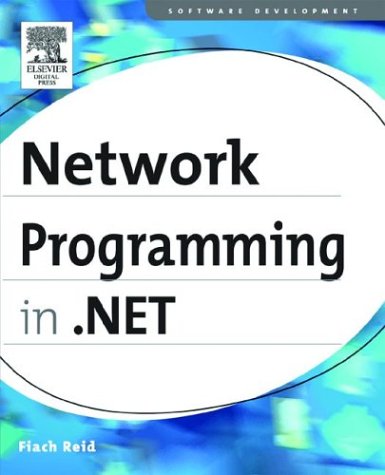
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewColumnConverter.cs
- Subordinate.cs
- PopupEventArgs.cs
- CdpEqualityComparer.cs
- ActivationArguments.cs
- SerialErrors.cs
- SmtpNetworkElement.cs
- NonBatchDirectoryCompiler.cs
- Zone.cs
- PrintPreviewGraphics.cs
- SingleStorage.cs
- ApplicationFileParser.cs
- IncrementalCompileAnalyzer.cs
- DrawingContextWalker.cs
- DataGridViewTopLeftHeaderCell.cs
- VirtualDirectoryMapping.cs
- CustomExpression.cs
- TextParaLineResult.cs
- Filter.cs
- ObjectListField.cs
- GenerateScriptTypeAttribute.cs
- EventLogPermissionEntry.cs
- CodeMethodMap.cs
- FixUp.cs
- DPCustomTypeDescriptor.cs
- DiscardableAttribute.cs
- OracleLob.cs
- TdsParameterSetter.cs
- NativeActivityTransactionContext.cs
- ObjectSet.cs
- ToolStripPanelCell.cs
- _ProxyRegBlob.cs
- HtmlInputRadioButton.cs
- SoundPlayer.cs
- BinaryFormatterSinks.cs
- MenuItemStyleCollection.cs
- TextBoxRenderer.cs
- DataSetMappper.cs
- FixedSOMLineCollection.cs
- SByte.cs
- Compilation.cs
- RelationshipEntry.cs
- QilXmlReader.cs
- FamilyTypeface.cs
- MenuItem.cs
- DataBoundControl.cs
- ICspAsymmetricAlgorithm.cs
- DbBuffer.cs
- TreeView.cs
- ClientOptions.cs
- TextServicesCompartmentEventSink.cs
- PropertyItem.cs
- RenderingBiasValidation.cs
- PropertyDescriptorCollection.cs
- DataGridViewCellStyle.cs
- SQLInt16.cs
- DesignerSerializerAttribute.cs
- OperationContext.cs
- ButtonDesigner.cs
- OdbcRowUpdatingEvent.cs
- ConfigurationStrings.cs
- SerializationFieldInfo.cs
- SqlUserDefinedAggregateAttribute.cs
- AffineTransform3D.cs
- Facet.cs
- LiteralTextContainerControlBuilder.cs
- WebPartVerbCollection.cs
- CacheHelper.cs
- SqlRewriteScalarSubqueries.cs
- SolidColorBrush.cs
- Object.cs
- InnerItemCollectionView.cs
- IdentityHolder.cs
- DataBindingCollectionEditor.cs
- BinHexEncoder.cs
- ControlAdapter.cs
- XPathArrayIterator.cs
- LockRecursionException.cs
- GridPatternIdentifiers.cs
- CounterCreationDataCollection.cs
- FormViewModeEventArgs.cs
- RuleSettings.cs
- PackageStore.cs
- AddInDeploymentState.cs
- SkipStoryboardToFill.cs
- RefreshPropertiesAttribute.cs
- DataBindingsDialog.cs
- NavigatorOutput.cs
- safemediahandle.cs
- CachedBitmap.cs
- LocatorGroup.cs
- CodeTypeMemberCollection.cs
- ValidationRuleCollection.cs
- SmtpLoginAuthenticationModule.cs
- XmlSchemaParticle.cs
- DebugHandleTracker.cs
- SpeechRecognizer.cs
- CodeObject.cs
- InfiniteTimeSpanConverter.cs
- MergePropertyDescriptor.cs