Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / DbFunctionCommandTree.cs / 1 / DbFunctionCommandTree.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// Represents a function invocation expressed as a canonical command tree /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbFunctionCommandTree : DbCommandTree { private readonly EdmFunction _edmFunction; private readonly TypeUsage _resultType; ////// Constructs a new DbFunctionCommandTree that uses the specified metadata workspace, data space and function metadata /// /// The metadata workspace that the command tree should use. /// The logical 'space' that metadata in the expressions used in this command tree must belong to. /// /// ////// , or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbFunctionCommandTree(MetadataWorkspace metadata, DataSpace dataSpace, EdmFunction edmFunction, TypeUsage resultType) : base(metadata, dataSpace) { using (new EntityBid.ScopeAuto(" does not represent a valid data space or /// is a composable function %d#", this.ObjectId)) { EntityUtil.CheckArgumentNull(edmFunction, "edmFunction"); _edmFunction = edmFunction; _resultType = resultType; } } /// /// Gets the [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public EdmFunction EdmFunction { get { return _edmFunction; } } ///that represents the function to invoke /// /// Gets the result type of the function; currently constrained to be a Collection of /// RowTypes. Unlike typical RowType instance, merely indicates name/type not parameter /// order. /// public TypeUsage ResultType { get { return _resultType; } } internal override DbCommandTreeKind CommandTreeKind { get { return DbCommandTreeKind.Function; } } internal override void DumpStructure(ExpressionDumper dumper) { if (this.EdmFunction != null) { dumper.Dump(this.EdmFunction); } } internal override string PrintTree(ExpressionPrinter printer) { return printer.Print(this); } internal override void Replace(ExpressionReplacer callback) { throw EntityUtil.NotSupported(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// Represents a function invocation expressed as a canonical command tree /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbFunctionCommandTree : DbCommandTree { private readonly EdmFunction _edmFunction; private readonly TypeUsage _resultType; ////// Constructs a new DbFunctionCommandTree that uses the specified metadata workspace, data space and function metadata /// /// The metadata workspace that the command tree should use. /// The logical 'space' that metadata in the expressions used in this command tree must belong to. /// /// ////// , or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbFunctionCommandTree(MetadataWorkspace metadata, DataSpace dataSpace, EdmFunction edmFunction, TypeUsage resultType) : base(metadata, dataSpace) { using (new EntityBid.ScopeAuto(" does not represent a valid data space or /// is a composable function %d#", this.ObjectId)) { EntityUtil.CheckArgumentNull(edmFunction, "edmFunction"); _edmFunction = edmFunction; _resultType = resultType; } } /// /// Gets the [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public EdmFunction EdmFunction { get { return _edmFunction; } } ///that represents the function to invoke /// /// Gets the result type of the function; currently constrained to be a Collection of /// RowTypes. Unlike typical RowType instance, merely indicates name/type not parameter /// order. /// public TypeUsage ResultType { get { return _resultType; } } internal override DbCommandTreeKind CommandTreeKind { get { return DbCommandTreeKind.Function; } } internal override void DumpStructure(ExpressionDumper dumper) { if (this.EdmFunction != null) { dumper.Dump(this.EdmFunction); } } internal override string PrintTree(ExpressionPrinter printer) { return printer.Print(this); } internal override void Replace(ExpressionReplacer callback) { throw EntityUtil.NotSupported(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
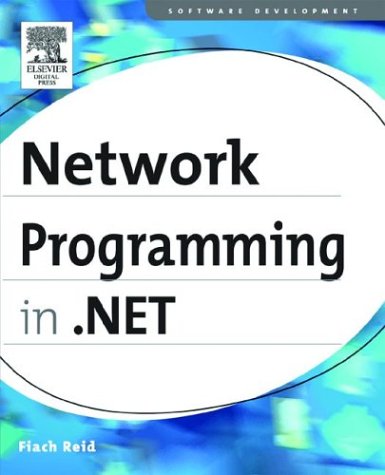
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StoreItemCollection.cs
- QilLoop.cs
- PropertyInformationCollection.cs
- ToolStripGripRenderEventArgs.cs
- CatalogZone.cs
- OleDbErrorCollection.cs
- httpserverutility.cs
- Oci.cs
- MissingSatelliteAssemblyException.cs
- ExpressionNode.cs
- StrokeNode.cs
- SecUtil.cs
- ResourceWriter.cs
- SubMenuStyle.cs
- PageCache.cs
- Mutex.cs
- BevelBitmapEffect.cs
- CommandManager.cs
- StringUtil.cs
- StylusTip.cs
- CommonGetThemePartSize.cs
- RelationshipNavigation.cs
- RedBlackList.cs
- HiddenField.cs
- UserPersonalizationStateInfo.cs
- CrossContextChannel.cs
- WebPartVerbCollection.cs
- XmlComplianceUtil.cs
- TableProviderWrapper.cs
- SerializerDescriptor.cs
- DataPagerFieldItem.cs
- TextFragmentEngine.cs
- MethodCallTranslator.cs
- ThaiBuddhistCalendar.cs
- SortQuery.cs
- StylusLogic.cs
- CodeExpressionStatement.cs
- Int64Storage.cs
- TraceSection.cs
- OleDbWrapper.cs
- MultiDataTrigger.cs
- EntityTypeBase.cs
- CreateSequence.cs
- DoubleAnimationClockResource.cs
- XPathMessageFilter.cs
- ScrollViewerAutomationPeer.cs
- SystemWebSectionGroup.cs
- BuildResult.cs
- HttpProxyCredentialType.cs
- ZipFileInfoCollection.cs
- RawStylusSystemGestureInputReport.cs
- AuthenticationService.cs
- InputManager.cs
- Container.cs
- TemplateDefinition.cs
- UnsafeCollabNativeMethods.cs
- embossbitmapeffect.cs
- COM2PictureConverter.cs
- ChangeProcessor.cs
- SiteIdentityPermission.cs
- SystemDiagnosticsSection.cs
- XsdCachingReader.cs
- ADMembershipUser.cs
- WebPartUserCapability.cs
- HtmlInputFile.cs
- Deflater.cs
- DataGridViewLayoutData.cs
- TypeDescriptionProvider.cs
- OutputScopeManager.cs
- HttpRequestContext.cs
- ContextMenuService.cs
- PerfCounterSection.cs
- FakeModelItemImpl.cs
- ObjectDataSourceFilteringEventArgs.cs
- StackBuilderSink.cs
- UITypeEditors.cs
- TemplateColumn.cs
- DataGridViewControlCollection.cs
- XmlTextReaderImpl.cs
- LinearKeyFrames.cs
- SmiEventSink.cs
- TdsParserStaticMethods.cs
- ListControlDesigner.cs
- IconConverter.cs
- SizeLimitedCache.cs
- IpcPort.cs
- ReaderWriterLock.cs
- DataObjectAttribute.cs
- SqlDuplicator.cs
- MetadataStore.cs
- ServiceNameElement.cs
- Win32KeyboardDevice.cs
- ErrorTableItemStyle.cs
- PeerPresenceInfo.cs
- ModuleBuilder.cs
- OletxTransactionManager.cs
- HtmlTable.cs
- DecimalAnimationBase.cs
- TreeNodeEventArgs.cs
- DataGridViewCheckBoxColumn.cs