Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / FunctionParameter.cs / 1 / FunctionParameter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Text; namespace System.Data.Metadata.Edm { ////// Class representing a function parameter /// public sealed class FunctionParameter : MetadataItem { internal static Func> DeclaringFunctionLinker = fp => fp._declaringFunction; #region Constructors /// /// The constructor for FunctionParameter taking in a name and a TypeUsage object /// /// The name of this FunctionParameter /// The TypeUsage describing the type of this FunctionParameter /// Mode of the parameter ///Thrown if name or typeUsage arguments are null ///Thrown if name argument is empty string internal FunctionParameter(string name, TypeUsage typeUsage, ParameterMode parameterMode) { EntityUtil.CheckStringArgument(name, "name"); EntityUtil.GenericCheckArgumentNull(typeUsage, "typeUsage"); _name = name; _typeUsage = typeUsage; SetParameterMode(parameterMode); } #endregion #region Fields private readonly TypeUsage _typeUsage; private readonly string _name; private readonly SafeLink_declaringFunction = new SafeLink (); #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.FunctionParameter; } } ////// Gets/Sets the mode of this parameter /// ///Thrown if value passed into setter is null ///Thrown if the FunctionParameter instance is in ReadOnly state [MetadataProperty(BuiltInTypeKind.ParameterMode, false)] public ParameterMode Mode { get { return GetParameterMode(); } } ////// Returns the identity of the member /// internal override string Identity { get { return _name; } } ////// Returns the name of the member /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _name; } } ////// Returns the TypeUsage object containing the type information and facets /// about the type /// [MetadataProperty(BuiltInTypeKind.TypeUsage, false)] public TypeUsage TypeUsage { get { return _typeUsage; } } ////// Returns the declaring function of this parameter /// public EdmFunction DeclaringFunction { get { return _declaringFunction.Value; } } #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return Name; } ////// Sets the member to read only mode. Once this is done, there are no changes /// that can be done to this class /// internal override void SetReadOnly() { if (!IsReadOnly) { base.SetReadOnly(); // TypeUsage is always readonly, no reason to set it } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Text; namespace System.Data.Metadata.Edm { ////// Class representing a function parameter /// public sealed class FunctionParameter : MetadataItem { internal static Func> DeclaringFunctionLinker = fp => fp._declaringFunction; #region Constructors /// /// The constructor for FunctionParameter taking in a name and a TypeUsage object /// /// The name of this FunctionParameter /// The TypeUsage describing the type of this FunctionParameter /// Mode of the parameter ///Thrown if name or typeUsage arguments are null ///Thrown if name argument is empty string internal FunctionParameter(string name, TypeUsage typeUsage, ParameterMode parameterMode) { EntityUtil.CheckStringArgument(name, "name"); EntityUtil.GenericCheckArgumentNull(typeUsage, "typeUsage"); _name = name; _typeUsage = typeUsage; SetParameterMode(parameterMode); } #endregion #region Fields private readonly TypeUsage _typeUsage; private readonly string _name; private readonly SafeLink_declaringFunction = new SafeLink (); #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.FunctionParameter; } } ////// Gets/Sets the mode of this parameter /// ///Thrown if value passed into setter is null ///Thrown if the FunctionParameter instance is in ReadOnly state [MetadataProperty(BuiltInTypeKind.ParameterMode, false)] public ParameterMode Mode { get { return GetParameterMode(); } } ////// Returns the identity of the member /// internal override string Identity { get { return _name; } } ////// Returns the name of the member /// [MetadataProperty(PrimitiveTypeKind.String, false)] public String Name { get { return _name; } } ////// Returns the TypeUsage object containing the type information and facets /// about the type /// [MetadataProperty(BuiltInTypeKind.TypeUsage, false)] public TypeUsage TypeUsage { get { return _typeUsage; } } ////// Returns the declaring function of this parameter /// public EdmFunction DeclaringFunction { get { return _declaringFunction.Value; } } #endregion #region Methods ////// Overriding System.Object.ToString to provide better String representation /// for this type. /// public override string ToString() { return Name; } ////// Sets the member to read only mode. Once this is done, there are no changes /// that can be done to this class /// internal override void SetReadOnly() { if (!IsReadOnly) { base.SetReadOnly(); // TypeUsage is always readonly, no reason to set it } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
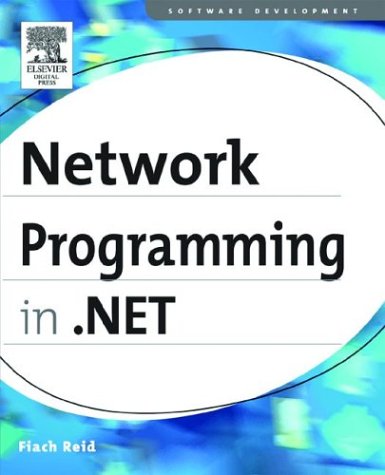
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XMLSchema.cs
- ZoneMembershipCondition.cs
- ActivityWithResultWrapper.cs
- ValidationRule.cs
- Rotation3DKeyFrameCollection.cs
- DataGridColumnStyleMappingNameEditor.cs
- RichTextBoxAutomationPeer.cs
- Label.cs
- Process.cs
- WebContext.cs
- DocumentViewer.cs
- FormsAuthenticationConfiguration.cs
- Barrier.cs
- DataGridPagerStyle.cs
- ObjectAnimationUsingKeyFrames.cs
- XmlSchemaProviderAttribute.cs
- SqlDataSourceCustomCommandPanel.cs
- EraserBehavior.cs
- WindowsScrollBarBits.cs
- HWStack.cs
- PersonalizationProviderCollection.cs
- RichTextBox.cs
- UrlPath.cs
- TextDecorationCollectionConverter.cs
- CompositeFontParser.cs
- TextBox.cs
- Bits.cs
- Environment.cs
- DoubleLinkListEnumerator.cs
- WpfXamlType.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- WebPartHelpVerb.cs
- HwndHost.cs
- TextCompositionManager.cs
- HashCodeCombiner.cs
- NativeObjectSecurity.cs
- TypeConverterHelper.cs
- EventDescriptorCollection.cs
- CompositeDataBoundControl.cs
- SubstitutionDesigner.cs
- NoPersistHandle.cs
- Clock.cs
- TemplatePagerField.cs
- TemplateControlParser.cs
- TargetException.cs
- UIElementIsland.cs
- ChannelTokenTypeConverter.cs
- TypeDescriptionProvider.cs
- FlowLayoutSettings.cs
- UrlPropertyAttribute.cs
- WindowsListViewSubItem.cs
- ContainerAction.cs
- MenuItemCollectionEditorDialog.cs
- SystemWebCachingSectionGroup.cs
- SessionStateModule.cs
- DNS.cs
- WebPart.cs
- TextDecorationCollection.cs
- MsmqIntegrationMessageProperty.cs
- RegexCapture.cs
- SpecialNameAttribute.cs
- SplitterEvent.cs
- _DynamicWinsockMethods.cs
- DesignerTransactionCloseEvent.cs
- ExceptionUtil.cs
- CompilerScope.Storage.cs
- RegistrySecurity.cs
- CompoundFileReference.cs
- SetterBase.cs
- Console.cs
- TableLayoutPanelCodeDomSerializer.cs
- MsmqTransportBindingElement.cs
- SafeCertificateStore.cs
- EventLogPermissionEntryCollection.cs
- SplashScreenNativeMethods.cs
- SystemIcmpV6Statistics.cs
- FlowDocumentReaderAutomationPeer.cs
- IriParsingElement.cs
- EpmContentSerializer.cs
- ConnectionPoint.cs
- DrawingContextWalker.cs
- VectorAnimationUsingKeyFrames.cs
- AttributeEmitter.cs
- FacetValues.cs
- ECDiffieHellman.cs
- NativeMethods.cs
- ThaiBuddhistCalendar.cs
- EvidenceBase.cs
- CryptoHelper.cs
- DetailsView.cs
- RequiredAttributeAttribute.cs
- CharEntityEncoderFallback.cs
- DataControlFieldHeaderCell.cs
- SingleKeyFrameCollection.cs
- ImagingCache.cs
- Trace.cs
- isolationinterop.cs
- BasicExpressionVisitor.cs
- ThousandthOfEmRealPoints.cs
- ButtonAutomationPeer.cs