Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Imaging / BitmapFrame.cs / 1 / BitmapFrame.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation // // File: BitmapFrame.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using MS.Win32; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods = MS.Win32.PresentationCore.UnsafeNativeMethods; using System.Windows.Markup; using System.Net.Cache; namespace System.Windows.Media.Imaging { #region BitmapFrame ////// BitmapFrame abstract class /// public abstract class BitmapFrame : BitmapSource, IUriContext { #region Constructors ////// Constructor /// protected BitmapFrame() { } ////// Internal Constructor /// internal BitmapFrame(bool useVirtuals) : base(useVirtuals) { } ////// Create BitmapFrame from the uri or stream /// internal static BitmapFrame CreateFromUriOrStream( Uri baseUri, Uri uri, Stream stream, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption, RequestCachePolicy uriCachePolicy ) { // Create a decoder and return the first frame if (uri != null) { Debug.Assert((stream == null), "Both stream and uri are non-null"); BitmapDecoder decoder = BitmapDecoder.CreateFromUriOrStream( baseUri, uri, null, createOptions, cacheOption, uriCachePolicy, true ); if (decoder.Frames.Count == 0) { throw new System.ArgumentException(SR.Get(SRID.Image_NoDecodeFrames), "uri"); } return decoder.Frames[0]; } else { Debug.Assert((stream != null), "Both stream and uri are null"); BitmapDecoder decoder = BitmapDecoder.Create( stream, createOptions, cacheOption ); if (decoder.Frames.Count == 0) { throw new System.ArgumentException(SR.Get(SRID.Image_NoDecodeFrames), "stream"); } return decoder.Frames[0]; } } ////// Create a BitmapFrame from a Uri using BitmapCreateOptions.None and /// BitmapCacheOption.Default /// /// Uri of the Bitmap public static BitmapFrame Create( Uri bitmapUri ) { return Create(bitmapUri, null); } ////// Create a BitmapFrame from a Uri using BitmapCreateOptions.None and /// BitmapCacheOption.Default /// /// Uri of the Bitmap /// Optional web request cache policy public static BitmapFrame Create( Uri bitmapUri, RequestCachePolicy uriCachePolicy ) { if (bitmapUri == null) { throw new ArgumentNullException("bitmapUri"); } return CreateFromUriOrStream( null, bitmapUri, null, BitmapCreateOptions.None, BitmapCacheOption.Default, uriCachePolicy ); } ////// Create a BitmapFrame from a Uri with the specified BitmapCreateOptions and /// BitmapCacheOption /// /// Uri of the Bitmap /// Creation options /// Caching option public static BitmapFrame Create( Uri bitmapUri, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption ) { return Create(bitmapUri, createOptions, cacheOption, null); } ////// Create a BitmapFrame from a Uri with the specified BitmapCreateOptions and /// BitmapCacheOption /// /// Uri of the Bitmap /// Creation options /// Caching option /// Optional web request cache policy public static BitmapFrame Create( Uri bitmapUri, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption, RequestCachePolicy uriCachePolicy ) { if (bitmapUri == null) { throw new ArgumentNullException("bitmapUri"); } return CreateFromUriOrStream( null, bitmapUri, null, createOptions, cacheOption, uriCachePolicy ); } ////// Create a BitmapFrame from a Stream using BitmapCreateOptions.None and /// BitmapCacheOption.Default /// /// Stream of the Bitmap public static BitmapFrame Create( Stream bitmapStream ) { if (bitmapStream == null) { throw new ArgumentNullException("bitmapStream"); } return CreateFromUriOrStream( null, null, bitmapStream, BitmapCreateOptions.None, BitmapCacheOption.Default, null ); } ////// Create a BitmapFrame from a Stream with the specified BitmapCreateOptions and /// BitmapCacheOption /// /// Stream of the Bitmap /// Creation options /// Caching option public static BitmapFrame Create( Stream bitmapStream, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption ) { if (bitmapStream == null) { throw new ArgumentNullException("bitmapStream"); } return CreateFromUriOrStream( null, null, bitmapStream, createOptions, cacheOption, null ); } ////// Create a BitmapFrame from a BitmapSource /// /// Source input of the Bitmap public static BitmapFrame Create( BitmapSource source ) { if (source == null) { throw new ArgumentNullException("source"); } BitmapMetadata metadata = null; try { metadata = source.Metadata as BitmapMetadata; } catch (System.NotSupportedException) { //do not throw not support exception //just pass null } if (metadata != null) { metadata = metadata.Clone(); } return new BitmapFrameEncode(source, null, metadata, null); } ////// Create a BitmapFrame from a BitmapSource with the specified Thumbnail /// /// Source input of the Bitmap /// Thumbnail of the resulting Bitmap public static BitmapFrame Create( BitmapSource source, BitmapSource thumbnail ) { if (source == null) { throw new ArgumentNullException("source"); } BitmapMetadata metadata = null; try { metadata = source.Metadata as BitmapMetadata; } catch (System.NotSupportedException) { //do not throw not support exception //just pass null } if (metadata != null) { metadata = metadata.Clone(); } return BitmapFrame.Create(source, thumbnail, metadata, null); } ////// Create a BitmapFrame from a BitmapSource with the specified Thumbnail and metadata /// /// Source input of the Bitmap /// Thumbnail of the resulting Bitmap /// BitmapMetadata of the resulting Bitmap /// The ColorContexts for the resulting Bitmap public static BitmapFrame Create( BitmapSource source, BitmapSource thumbnail, BitmapMetadata metadata, ReadOnlyCollectioncolorContexts ) { if (source == null) { throw new ArgumentNullException("source"); } return new BitmapFrameEncode(source, thumbnail, metadata, colorContexts); } #endregion #region IUriContext /// /// Provides the base uri of the current context. /// public abstract Uri BaseUri { get; set; } #endregion #region Public Properties ////// Accesses the Thumbnail property for this BitmapFrame /// public abstract BitmapSource Thumbnail { get; } ////// Accesses the Decoder property for this BitmapFrame /// public abstract BitmapDecoder Decoder { get; } ////// Accesses the Decoder property for this BitmapFrame /// public abstract ReadOnlyCollectionColorContexts { get; } #endregion #region Public Properties /// /// Create an in-place bitmap metadata writer. /// public abstract InPlaceBitmapMetadataWriter CreateInPlaceBitmapMetadataWriter(); #endregion #region Internal Properties ////// Returns cached metadata and creates BitmapMetadata if it does not exist. /// This code will demand site of origin permissions. /// internal virtual BitmapMetadata InternalMetadata { get { return null; } set { throw new NotImplementedException(); } } #endregion #region Internal Abstract /// "Seals" the object internal abstract void SealObject(); #endregion #region Data Members /// IWICBitmapFrameDecode source internal BitmapSourceSafeMILHandle _frameSource = null; /// Thumbnail internal BitmapSource _thumbnail = null; ////// Metadata /// ////// Critical - Access only granted if SiteOfOrigin demanded. /// [SecurityCritical] internal BitmapMetadata _metadata; /// Decoder internal BitmapDecoder _decoder; /// ColorContexts collection internal ReadOnlyCollection_readOnlycolorContexts; #endregion } #endregion // BitmapFrame } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation // // File: BitmapFrame.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Collections.ObjectModel; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using MS.Win32; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods = MS.Win32.PresentationCore.UnsafeNativeMethods; using System.Windows.Markup; using System.Net.Cache; namespace System.Windows.Media.Imaging { #region BitmapFrame /// /// BitmapFrame abstract class /// public abstract class BitmapFrame : BitmapSource, IUriContext { #region Constructors ////// Constructor /// protected BitmapFrame() { } ////// Internal Constructor /// internal BitmapFrame(bool useVirtuals) : base(useVirtuals) { } ////// Create BitmapFrame from the uri or stream /// internal static BitmapFrame CreateFromUriOrStream( Uri baseUri, Uri uri, Stream stream, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption, RequestCachePolicy uriCachePolicy ) { // Create a decoder and return the first frame if (uri != null) { Debug.Assert((stream == null), "Both stream and uri are non-null"); BitmapDecoder decoder = BitmapDecoder.CreateFromUriOrStream( baseUri, uri, null, createOptions, cacheOption, uriCachePolicy, true ); if (decoder.Frames.Count == 0) { throw new System.ArgumentException(SR.Get(SRID.Image_NoDecodeFrames), "uri"); } return decoder.Frames[0]; } else { Debug.Assert((stream != null), "Both stream and uri are null"); BitmapDecoder decoder = BitmapDecoder.Create( stream, createOptions, cacheOption ); if (decoder.Frames.Count == 0) { throw new System.ArgumentException(SR.Get(SRID.Image_NoDecodeFrames), "stream"); } return decoder.Frames[0]; } } ////// Create a BitmapFrame from a Uri using BitmapCreateOptions.None and /// BitmapCacheOption.Default /// /// Uri of the Bitmap public static BitmapFrame Create( Uri bitmapUri ) { return Create(bitmapUri, null); } ////// Create a BitmapFrame from a Uri using BitmapCreateOptions.None and /// BitmapCacheOption.Default /// /// Uri of the Bitmap /// Optional web request cache policy public static BitmapFrame Create( Uri bitmapUri, RequestCachePolicy uriCachePolicy ) { if (bitmapUri == null) { throw new ArgumentNullException("bitmapUri"); } return CreateFromUriOrStream( null, bitmapUri, null, BitmapCreateOptions.None, BitmapCacheOption.Default, uriCachePolicy ); } ////// Create a BitmapFrame from a Uri with the specified BitmapCreateOptions and /// BitmapCacheOption /// /// Uri of the Bitmap /// Creation options /// Caching option public static BitmapFrame Create( Uri bitmapUri, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption ) { return Create(bitmapUri, createOptions, cacheOption, null); } ////// Create a BitmapFrame from a Uri with the specified BitmapCreateOptions and /// BitmapCacheOption /// /// Uri of the Bitmap /// Creation options /// Caching option /// Optional web request cache policy public static BitmapFrame Create( Uri bitmapUri, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption, RequestCachePolicy uriCachePolicy ) { if (bitmapUri == null) { throw new ArgumentNullException("bitmapUri"); } return CreateFromUriOrStream( null, bitmapUri, null, createOptions, cacheOption, uriCachePolicy ); } ////// Create a BitmapFrame from a Stream using BitmapCreateOptions.None and /// BitmapCacheOption.Default /// /// Stream of the Bitmap public static BitmapFrame Create( Stream bitmapStream ) { if (bitmapStream == null) { throw new ArgumentNullException("bitmapStream"); } return CreateFromUriOrStream( null, null, bitmapStream, BitmapCreateOptions.None, BitmapCacheOption.Default, null ); } ////// Create a BitmapFrame from a Stream with the specified BitmapCreateOptions and /// BitmapCacheOption /// /// Stream of the Bitmap /// Creation options /// Caching option public static BitmapFrame Create( Stream bitmapStream, BitmapCreateOptions createOptions, BitmapCacheOption cacheOption ) { if (bitmapStream == null) { throw new ArgumentNullException("bitmapStream"); } return CreateFromUriOrStream( null, null, bitmapStream, createOptions, cacheOption, null ); } ////// Create a BitmapFrame from a BitmapSource /// /// Source input of the Bitmap public static BitmapFrame Create( BitmapSource source ) { if (source == null) { throw new ArgumentNullException("source"); } BitmapMetadata metadata = null; try { metadata = source.Metadata as BitmapMetadata; } catch (System.NotSupportedException) { //do not throw not support exception //just pass null } if (metadata != null) { metadata = metadata.Clone(); } return new BitmapFrameEncode(source, null, metadata, null); } ////// Create a BitmapFrame from a BitmapSource with the specified Thumbnail /// /// Source input of the Bitmap /// Thumbnail of the resulting Bitmap public static BitmapFrame Create( BitmapSource source, BitmapSource thumbnail ) { if (source == null) { throw new ArgumentNullException("source"); } BitmapMetadata metadata = null; try { metadata = source.Metadata as BitmapMetadata; } catch (System.NotSupportedException) { //do not throw not support exception //just pass null } if (metadata != null) { metadata = metadata.Clone(); } return BitmapFrame.Create(source, thumbnail, metadata, null); } ////// Create a BitmapFrame from a BitmapSource with the specified Thumbnail and metadata /// /// Source input of the Bitmap /// Thumbnail of the resulting Bitmap /// BitmapMetadata of the resulting Bitmap /// The ColorContexts for the resulting Bitmap public static BitmapFrame Create( BitmapSource source, BitmapSource thumbnail, BitmapMetadata metadata, ReadOnlyCollectioncolorContexts ) { if (source == null) { throw new ArgumentNullException("source"); } return new BitmapFrameEncode(source, thumbnail, metadata, colorContexts); } #endregion #region IUriContext /// /// Provides the base uri of the current context. /// public abstract Uri BaseUri { get; set; } #endregion #region Public Properties ////// Accesses the Thumbnail property for this BitmapFrame /// public abstract BitmapSource Thumbnail { get; } ////// Accesses the Decoder property for this BitmapFrame /// public abstract BitmapDecoder Decoder { get; } ////// Accesses the Decoder property for this BitmapFrame /// public abstract ReadOnlyCollectionColorContexts { get; } #endregion #region Public Properties /// /// Create an in-place bitmap metadata writer. /// public abstract InPlaceBitmapMetadataWriter CreateInPlaceBitmapMetadataWriter(); #endregion #region Internal Properties ////// Returns cached metadata and creates BitmapMetadata if it does not exist. /// This code will demand site of origin permissions. /// internal virtual BitmapMetadata InternalMetadata { get { return null; } set { throw new NotImplementedException(); } } #endregion #region Internal Abstract /// "Seals" the object internal abstract void SealObject(); #endregion #region Data Members /// IWICBitmapFrameDecode source internal BitmapSourceSafeMILHandle _frameSource = null; /// Thumbnail internal BitmapSource _thumbnail = null; ////// Metadata /// ////// Critical - Access only granted if SiteOfOrigin demanded. /// [SecurityCritical] internal BitmapMetadata _metadata; /// Decoder internal BitmapDecoder _decoder; /// ColorContexts collection internal ReadOnlyCollection_readOnlycolorContexts; #endregion } #endregion // BitmapFrame } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
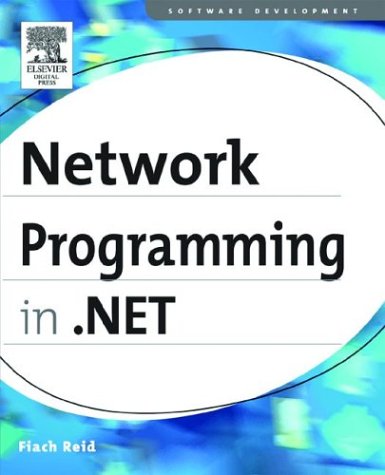
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerializationInfo.cs
- GroupByExpressionRewriter.cs
- HighContrastHelper.cs
- PersonalizationStateInfoCollection.cs
- StyleHelper.cs
- ArglessEventHandlerProxy.cs
- SiteMapDataSource.cs
- CodeEventReferenceExpression.cs
- ACE.cs
- AssignDesigner.xaml.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- BuildResult.cs
- DataGridViewCellConverter.cs
- ContentElement.cs
- CopyAction.cs
- XmlTextEncoder.cs
- UntrustedRecipientException.cs
- SchemaElementLookUpTableEnumerator.cs
- XhtmlBasicImageAdapter.cs
- XmlSchemaAppInfo.cs
- PropertyGeneratedEventArgs.cs
- XmlSchemaDatatype.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- cookiecollection.cs
- NamespaceQuery.cs
- ScrollPattern.cs
- AdornerDecorator.cs
- ToolStripContainer.cs
- ServiceContractAttribute.cs
- RenderContext.cs
- ThreadSafeList.cs
- AdPostCacheSubstitution.cs
- NonVisualControlAttribute.cs
- StrokeNode.cs
- Base64WriteStateInfo.cs
- CodeTypeMember.cs
- PrefixQName.cs
- DummyDataSource.cs
- FolderBrowserDialog.cs
- StrokeNodeOperations.cs
- FunctionImportElement.cs
- TimersDescriptionAttribute.cs
- RoleGroupCollection.cs
- Win32.cs
- Axis.cs
- Permission.cs
- FileEnumerator.cs
- HttpSocketManager.cs
- DispatchWrapper.cs
- ObjectNavigationPropertyMapping.cs
- Accessible.cs
- ObjectHandle.cs
- FactoryRecord.cs
- HttpServerUtilityWrapper.cs
- RoleManagerSection.cs
- NamespaceList.cs
- TextAutomationPeer.cs
- ConnectionsZone.cs
- QilInvokeEarlyBound.cs
- WebPartConnectionsCancelEventArgs.cs
- SQLByteStorage.cs
- Int64AnimationUsingKeyFrames.cs
- DataGridRowsPresenter.cs
- PropertyTabAttribute.cs
- EncoderNLS.cs
- MinimizableAttributeTypeConverter.cs
- ThemeableAttribute.cs
- Transform.cs
- AliasExpr.cs
- BaseValidator.cs
- MailMessageEventArgs.cs
- _ConnectStream.cs
- BulletedList.cs
- DataAdapter.cs
- InfoCardArgumentException.cs
- TdsValueSetter.cs
- Opcode.cs
- XmlSerializerOperationBehavior.cs
- XmlBinaryReader.cs
- HtmlObjectListAdapter.cs
- DataGridViewDataConnection.cs
- HtmlShimManager.cs
- OperationCanceledException.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- TextEditorContextMenu.cs
- ListSourceHelper.cs
- WmlControlAdapter.cs
- DataGridViewRowEventArgs.cs
- TextServicesCompartmentEventSink.cs
- WebPageTraceListener.cs
- XmlSchemaCollection.cs
- EmissiveMaterial.cs
- EncodingNLS.cs
- EntityClassGenerator.cs
- LambdaCompiler.Lambda.cs
- MediaScriptCommandRoutedEventArgs.cs
- ResourceReferenceKeyNotFoundException.cs
- ContentOperations.cs
- Debug.cs
- DateTime.cs