Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / SolidColorBrush.cs / 1 / SolidColorBrush.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: SolidColorBrush.cs // // Description: This file contains the implementation of SolidColorBrush. // The SolidColorBrush is the simplest of the Brushes. consisting // as it does of just a color. // // History: // 04/28/2003 : adsmith - Created it. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// SolidColorBrush /// The SolidColorBrush is the simplest of the Brushes. It can be used to /// fill an area with a solid color, which can be animate. /// public sealed partial class SolidColorBrush : Brush { #region Constructors ////// Default constructor for SolidColorBrush. /// public SolidColorBrush() { } ////// SolidColorBrush - The constructor accepts the color of the brush /// /// The color value. public SolidColorBrush(Color color) { Color = color; } #endregion Constructors #region Serialization // This enum is used to identify brush types for deserialization in the // ConvertCustomBinaryToObject method. If we support more types of brushes, // then we may have to expose this publically and add more enum values. internal enum SerializationBrushType : byte { Unknown = 0, KnownSolidColor = 1, OtherColor = 2, } ////// Serialize this object using the passed writer in compact BAML binary format. /// ////// This is called ONLY from the Parser and is not a general public method. /// ////// Thrown if "writer" is null. /// [FriendAccessAllowed] // Built into Core, also used by Framework. internal static bool SerializeOn(BinaryWriter writer, string stringValue) { // ********* VERY IMPORTANT NOTE ***************** // If this method is changed, then XamlBrushSerilaizer.SerializeOn() needs // to be correspondingly changed as well. That code is linked into PBT.dll // and duplicates the code below to avoid pulling in SCB & base classes as well. // ********* VERY IMPORTANT NOTE ***************** if (writer == null) { throw new ArgumentNullException("writer"); } KnownColor knownColor = KnownColors.ColorStringToKnownColor(stringValue); if (knownColor != KnownColor.UnknownColor) { // Serialize values of the type "Red", "Blue" and other names writer.Write((byte)SerializationBrushType.KnownSolidColor); writer.Write((uint)knownColor); return true; } else { // Serialize values of the type "#F00", "#0000FF" and other hex color values. // We don't have a good way to check if this is valid without running the // converter at this point, so just store the string if it has at least a // minimum length of 4. stringValue = stringValue.Trim(); if (stringValue.Length > 3) { writer.Write((byte)SerializationBrushType.OtherColor); writer.Write(stringValue); return true; } } return false; } ////// Deserialize this object using the passed reader. Throw an exception if /// the format is not a solid color brush. /// ////// This is called ONLY from the Parser and is not a general public method. /// ////// Thrown if "reader" is null. /// public static object DeserializeFrom(BinaryReader reader) { if (reader == null) { throw new ArgumentNullException("reader"); } return DeserializeFrom(reader, null); } internal static object DeserializeFrom(BinaryReader reader, ITypeDescriptorContext context) { SerializationBrushType brushType = (SerializationBrushType)reader.ReadByte(); if (brushType == SerializationBrushType.KnownSolidColor) { uint knownColorUint = reader.ReadUInt32(); return KnownColors.SolidColorBrushFromUint(knownColorUint); } else if (brushType == SerializationBrushType.OtherColor) { string colorValue = reader.ReadString(); BrushConverter converter = new BrushConverter(); return converter.ConvertFromInvariantString(context, colorValue); } else { throw new Exception(SR.Get(SRID.BrushUnknownBamlType)); } } #endregion Serialization #region ToString ////// CanSerializeToString - an internal helper method which determines whether this object /// can fully serialize to a string with no data loss. /// ////// bool - true if full fidelity serialization is possible, false if not. /// internal override bool CanSerializeToString() { if (HasAnimatedProperties || HasAnyExpression() || !Transform.IsIdentity || !DoubleUtil.AreClose(Opacity, Brush.c_Opacity)) { return false; } return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { return Color.ConvertToString(format, provider); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: SolidColorBrush.cs // // Description: This file contains the implementation of SolidColorBrush. // The SolidColorBrush is the simplest of the Brushes. consisting // as it does of just a color. // // History: // 04/28/2003 : adsmith - Created it. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.IO; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Markup; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// SolidColorBrush /// The SolidColorBrush is the simplest of the Brushes. It can be used to /// fill an area with a solid color, which can be animate. /// public sealed partial class SolidColorBrush : Brush { #region Constructors ////// Default constructor for SolidColorBrush. /// public SolidColorBrush() { } ////// SolidColorBrush - The constructor accepts the color of the brush /// /// The color value. public SolidColorBrush(Color color) { Color = color; } #endregion Constructors #region Serialization // This enum is used to identify brush types for deserialization in the // ConvertCustomBinaryToObject method. If we support more types of brushes, // then we may have to expose this publically and add more enum values. internal enum SerializationBrushType : byte { Unknown = 0, KnownSolidColor = 1, OtherColor = 2, } ////// Serialize this object using the passed writer in compact BAML binary format. /// ////// This is called ONLY from the Parser and is not a general public method. /// ////// Thrown if "writer" is null. /// [FriendAccessAllowed] // Built into Core, also used by Framework. internal static bool SerializeOn(BinaryWriter writer, string stringValue) { // ********* VERY IMPORTANT NOTE ***************** // If this method is changed, then XamlBrushSerilaizer.SerializeOn() needs // to be correspondingly changed as well. That code is linked into PBT.dll // and duplicates the code below to avoid pulling in SCB & base classes as well. // ********* VERY IMPORTANT NOTE ***************** if (writer == null) { throw new ArgumentNullException("writer"); } KnownColor knownColor = KnownColors.ColorStringToKnownColor(stringValue); if (knownColor != KnownColor.UnknownColor) { // Serialize values of the type "Red", "Blue" and other names writer.Write((byte)SerializationBrushType.KnownSolidColor); writer.Write((uint)knownColor); return true; } else { // Serialize values of the type "#F00", "#0000FF" and other hex color values. // We don't have a good way to check if this is valid without running the // converter at this point, so just store the string if it has at least a // minimum length of 4. stringValue = stringValue.Trim(); if (stringValue.Length > 3) { writer.Write((byte)SerializationBrushType.OtherColor); writer.Write(stringValue); return true; } } return false; } ////// Deserialize this object using the passed reader. Throw an exception if /// the format is not a solid color brush. /// ////// This is called ONLY from the Parser and is not a general public method. /// ////// Thrown if "reader" is null. /// public static object DeserializeFrom(BinaryReader reader) { if (reader == null) { throw new ArgumentNullException("reader"); } return DeserializeFrom(reader, null); } internal static object DeserializeFrom(BinaryReader reader, ITypeDescriptorContext context) { SerializationBrushType brushType = (SerializationBrushType)reader.ReadByte(); if (brushType == SerializationBrushType.KnownSolidColor) { uint knownColorUint = reader.ReadUInt32(); return KnownColors.SolidColorBrushFromUint(knownColorUint); } else if (brushType == SerializationBrushType.OtherColor) { string colorValue = reader.ReadString(); BrushConverter converter = new BrushConverter(); return converter.ConvertFromInvariantString(context, colorValue); } else { throw new Exception(SR.Get(SRID.BrushUnknownBamlType)); } } #endregion Serialization #region ToString ////// CanSerializeToString - an internal helper method which determines whether this object /// can fully serialize to a string with no data loss. /// ////// bool - true if full fidelity serialization is possible, false if not. /// internal override bool CanSerializeToString() { if (HasAnimatedProperties || HasAnyExpression() || !Transform.IsIdentity || !DoubleUtil.AreClose(Opacity, Brush.c_Opacity)) { return false; } return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { return Color.ConvertToString(format, provider); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
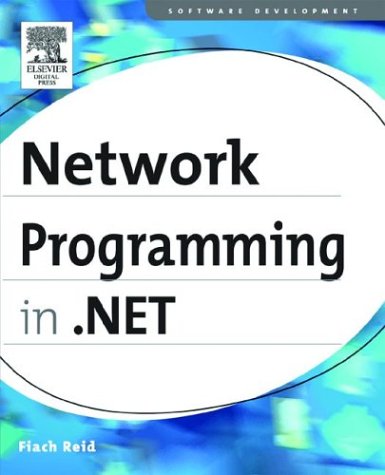
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmtpDigestAuthenticationModule.cs
- PageAsyncTaskManager.cs
- SafeCryptHandles.cs
- Emitter.cs
- mactripleDES.cs
- _ConnectionGroup.cs
- DeclaredTypeValidator.cs
- NonVisualControlAttribute.cs
- FtpWebResponse.cs
- XPathChildIterator.cs
- PackagePartCollection.cs
- DataGridViewLinkCell.cs
- DesignerTextWriter.cs
- ProjectionNode.cs
- ConnectionStringSettings.cs
- UIElement3D.cs
- FormatSettings.cs
- UInt64.cs
- StringStorage.cs
- IProvider.cs
- DrawingContext.cs
- WebPartDescription.cs
- OutOfProcStateClientManager.cs
- TextBoxRenderer.cs
- DemultiplexingClientMessageFormatter.cs
- DelegatedStream.cs
- XmlValidatingReader.cs
- HasCopySemanticsAttribute.cs
- NameScopePropertyAttribute.cs
- HttpListenerContext.cs
- HiddenFieldDesigner.cs
- SystemIPInterfaceStatistics.cs
- FlowLayoutPanel.cs
- XmlnsDictionary.cs
- HierarchicalDataSourceControl.cs
- DataGridViewDataConnection.cs
- EventProxy.cs
- APCustomTypeDescriptor.cs
- mediaclock.cs
- CodeMethodReturnStatement.cs
- PrePrepareMethodAttribute.cs
- ImageConverter.cs
- BindingMAnagerBase.cs
- DashStyle.cs
- QilTargetType.cs
- EntityCommand.cs
- ClientTarget.cs
- PropertyCollection.cs
- MessageSecurityException.cs
- ToolboxItemCollection.cs
- ToolStripEditorManager.cs
- ConfigurationValidatorBase.cs
- DesignerLoader.cs
- WorkflowShape.cs
- AvTraceFormat.cs
- DBAsyncResult.cs
- SerialPort.cs
- XamlParser.cs
- ScrollableControl.cs
- NonParentingControl.cs
- ProxyGenerator.cs
- PixelShader.cs
- KnownAssembliesSet.cs
- FormViewPagerRow.cs
- BaseTemplateBuildProvider.cs
- ManagedWndProcTracker.cs
- RangeValidator.cs
- StylusCaptureWithinProperty.cs
- CharUnicodeInfo.cs
- CurrentChangingEventManager.cs
- SchemaEntity.cs
- RemoteCryptoTokenProvider.cs
- StyleSelector.cs
- MsmqInputMessagePool.cs
- SafeFileMapViewHandle.cs
- DesignTimeDataBinding.cs
- SchemaElementDecl.cs
- XsdCachingReader.cs
- PropertyMapper.cs
- IconConverter.cs
- XmlEnumAttribute.cs
- FieldTemplateFactory.cs
- VisualTreeHelper.cs
- MergablePropertyAttribute.cs
- documentation.cs
- HyperLink.cs
- EventLogPermissionHolder.cs
- propertyentry.cs
- MetabaseSettingsIis7.cs
- ToolStripOverflowButton.cs
- SqlBulkCopyColumnMapping.cs
- HashStream.cs
- OdbcConnectionPoolProviderInfo.cs
- ApplyImportsAction.cs
- BoundColumn.cs
- DbTransaction.cs
- mediaeventshelper.cs
- SafeViewOfFileHandle.cs
- NameTable.cs
- ToolStripTextBox.cs