Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media3D / Generated / Size3DConverter.cs / 1 / Size3DConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media.Media3D { ////// Size3DConverter - Converter class for converting instances of other types to and from Size3D instances /// public sealed class Size3DConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Size3D from the given object. /// ////// The Size3D which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Size3D. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Size3D. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Size3D.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Size3D to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Size3D, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Size3D instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Size3D) { Size3D instance = (Size3D)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.Text; using System.Windows.Markup; using System.Windows.Media.Media3D.Converters; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media.Media3D { ////// Size3DConverter - Converter class for converting instances of other types to and from Size3D instances /// public sealed class Size3DConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Size3D from the given object. /// ////// The Size3D which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Size3D. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Size3D. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Size3D.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Size3D to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Size3D, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Size3D instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Size3D) { Size3D instance = (Size3D)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
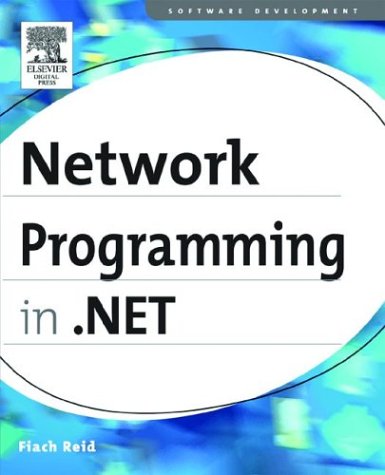
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesigntimeLicenseContext.cs
- DataGridViewUtilities.cs
- Interfaces.cs
- SafeUserTokenHandle.cs
- ConnectionStringsExpressionEditor.cs
- SessionStateModule.cs
- SerializationStore.cs
- SqlUtil.cs
- ClientProxyGenerator.cs
- DnsPermission.cs
- XmlDictionaryReader.cs
- URLBuilder.cs
- AuthenticationService.cs
- RegexMatchCollection.cs
- StringAttributeCollection.cs
- SqlCacheDependency.cs
- ProcessProtocolHandler.cs
- DropSourceBehavior.cs
- DataListDesigner.cs
- ContextQuery.cs
- SafeFileMapViewHandle.cs
- _OSSOCK.cs
- Interlocked.cs
- ResolvedKeyFrameEntry.cs
- ProgressChangedEventArgs.cs
- SslSecurityTokenParameters.cs
- _DomainName.cs
- ClientConfigPaths.cs
- TextRenderer.cs
- EventLogPermission.cs
- SQLBytesStorage.cs
- ScrollChrome.cs
- EdmRelationshipNavigationPropertyAttribute.cs
- CodeIdentifier.cs
- CancelAsyncOperationRequest.cs
- processwaithandle.cs
- PrivilegeNotHeldException.cs
- BooleanToVisibilityConverter.cs
- LinkedResource.cs
- ConfigurationException.cs
- ReflectionUtil.cs
- ProxyElement.cs
- FastEncoder.cs
- UpdateCommand.cs
- CompositeDuplexBindingElement.cs
- TransformerInfoCollection.cs
- TickBar.cs
- PolyLineSegmentFigureLogic.cs
- InkCanvasFeedbackAdorner.cs
- TableProviderWrapper.cs
- DataControlFieldCell.cs
- XmlSchemaObject.cs
- RecommendedAsConfigurableAttribute.cs
- ClientFormsIdentity.cs
- UnicastIPAddressInformationCollection.cs
- ArithmeticException.cs
- KeyGestureValueSerializer.cs
- InvalidOperationException.cs
- FlowDocumentPaginator.cs
- ExtenderControl.cs
- DbModificationCommandTree.cs
- SrgsRulesCollection.cs
- ListItemCollection.cs
- NoResizeSelectionBorderGlyph.cs
- GeometryCombineModeValidation.cs
- MenuItemCollection.cs
- BrushConverter.cs
- FieldNameLookup.cs
- OraclePermissionAttribute.cs
- SystemWebSectionGroup.cs
- BindingManagerDataErrorEventArgs.cs
- KeyValuePair.cs
- RemoteWebConfigurationHostServer.cs
- SiteMapDataSourceView.cs
- SqlCacheDependency.cs
- BufferBuilder.cs
- WebBrowser.cs
- SelectedCellsCollection.cs
- ReferenceEqualityComparer.cs
- SqlUserDefinedTypeAttribute.cs
- TreeNodeEventArgs.cs
- InvalidCastException.cs
- LineGeometry.cs
- OdbcErrorCollection.cs
- ListControlConvertEventArgs.cs
- SpecialFolderEnumConverter.cs
- XmlException.cs
- HttpClientCertificate.cs
- ScanQueryOperator.cs
- TypedElement.cs
- UnitySerializationHolder.cs
- TableCell.cs
- ComNativeDescriptor.cs
- WorkflowServiceHost.cs
- _SslSessionsCache.cs
- EnumCodeDomSerializer.cs
- RowTypePropertyElement.cs
- ThicknessAnimationBase.cs
- ListBindingHelper.cs
- WindowsGrip.cs