Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / Annotations / ObservableDictionary.cs / 1 / ObservableDictionary.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // ContentLocatorPart represents a set of name/value pairs that identify a // piece of data within a certain context. The names and values are // strings. // // Spec: http://team/sites/ag/Specifications/Simplifying%20Store%20Cache%20Model.doc // // History: // 05/06/2004: ssimova: Created // 06/30/2004: rruiz: Added change notifications to parent, clean-up //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Xml; namespace MS.Internal.Annotations { ////// ContentLocatorPart represents a set of name/value pairs that identify a /// piece of data within a certain context. The names and values are /// all strings. /// internal class ObservableDictionary : IDictionary, INotifyPropertyChanged { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors /// /// Creates a ContentLocatorPart with the specified type name and namespace. /// public ObservableDictionary() { _nameValues = new Dictionary(); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods /// /// Adds a key/value pair to the ContentLocatorPart. If a value for the key already /// exists, the old value is overwritten by the new value. /// /// key /// value ///key or val is null ///a value for key is already present in the locator part public void Add(string key, string val) { if (key == null || val == null) { throw new ArgumentNullException(key == null ? "key" : "val"); } _nameValues.Add(key, val); FireDictionaryChanged(); } ////// Removes all name/value pairs from the ContentLocatorPart. /// public void Clear() { int count = _nameValues.Count; if (count > 0) { _nameValues.Clear(); // Only fire changed event if the dictionary actually changed FireDictionaryChanged(); } } ////// Returns whether or not a value of the key exists in this ContentLocatorPart. /// /// the key to check for ///true - yes, false - no public bool ContainsKey(string key) { return _nameValues.ContainsKey(key); } ////// Removes the key and its value from the ContentLocatorPart. /// /// key to be removed ///true - the key was found in the ContentLocatorPart, false o- it wasn't public bool Remove(string key) { bool exists = _nameValues.Remove(key); // Only fire changed event if the key was actually removed if (exists) { FireDictionaryChanged(); } return exists; } ////// Returns an enumerator for the key/value pairs in this ContentLocatorPart. /// ///an enumerator for the key/value pairs; never returns null IEnumerator IEnumerable.GetEnumerator() { return _nameValues.GetEnumerator(); } ////// Returns an enumerator forthe key/value pairs in this ContentLocatorPart. /// ///an enumerator for the key/value pairs; never returns null public IEnumerator> GetEnumerator() { return ((IEnumerable >)_nameValues).GetEnumerator(); } /// /// /// /// /// ////// key is null public bool TryGetValue(string key, out string value) { if (key == null) throw new ArgumentNullException("key"); return _nameValues.TryGetValue(key, out value); } ////// /// /// ///pair is null void ICollection>.Add(KeyValuePair pair) { ((ICollection >)_nameValues).Add(pair); } /// /// /// /// ////// pair is null bool ICollection>.Contains(KeyValuePair pair) { return ((ICollection >)_nameValues).Contains(pair); } /// /// /// /// ////// pair is null bool ICollection>.Remove(KeyValuePair pair) { return ((ICollection >)_nameValues).Remove(pair); } /// /// /// /// /// ///target is null ///startIndex is less than zero or greater than the lenght of target void ICollection>.CopyTo(KeyValuePair [] target, int startIndex) { if (target == null) throw new ArgumentNullException("target"); if (startIndex < 0 || startIndex > target.Length) throw new ArgumentOutOfRangeException("startIndex"); ((ICollection >)_nameValues).CopyTo(target, startIndex); } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties /// /// The number of name/value pairs in this ContentLocatorPart. /// ///count of name/value pairs public int Count { get { return _nameValues.Count; } } ////// Indexer provides lookup of values by key. Gets or sets the value /// in the ContentLocatorPart for the specified key. If the key does not exist /// in the ContentLocatorPart, /// /// key ///the value stored in this locator part for key public string this[string key] { get { if (key == null) { throw new ArgumentNullException("key"); } string value = null; _nameValues.TryGetValue(key, out value); return value; } set { if (key == null) { throw new ArgumentNullException("key"); } if (value == null) { throw new ArgumentNullException("value"); } string oldValue = null; _nameValues.TryGetValue(key, out oldValue); // If the new value is actually different, then we add it and fire // a change notification if ((oldValue == null) || (oldValue != value)) { _nameValues[key] = value; FireDictionaryChanged(); } } } ////// /// public bool IsReadOnly { get { return false; } } ////// Returns a collection of all the keys in this ContentLocatorPart. /// ///keys public ICollectionKeys { get { return _nameValues.Keys; } } /// /// Returns a collection of all the values in this ContentLocatorPart. /// ///values public ICollectionValues { get { return _nameValues.Values; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ //------------------------------------------------------ // // Internal Operators // //----------------------------------------------------- //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- #region Public Events /// /// /// public event PropertyChangedEventHandler PropertyChanged; #endregion Public Events //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods ////// Notify the owner this ContentLocatorPart has changed. /// private void FireDictionaryChanged() { if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(null)); } } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields ////// The internal data structure. /// private Dictionary_nameValues; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // ContentLocatorPart represents a set of name/value pairs that identify a // piece of data within a certain context. The names and values are // strings. // // Spec: http://team/sites/ag/Specifications/Simplifying%20Store%20Cache%20Model.doc // // History: // 05/06/2004: ssimova: Created // 06/30/2004: rruiz: Added change notifications to parent, clean-up //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Xml; namespace MS.Internal.Annotations { ////// ContentLocatorPart represents a set of name/value pairs that identify a /// piece of data within a certain context. The names and values are /// all strings. /// internal class ObservableDictionary : IDictionary, INotifyPropertyChanged { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors /// /// Creates a ContentLocatorPart with the specified type name and namespace. /// public ObservableDictionary() { _nameValues = new Dictionary(); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods /// /// Adds a key/value pair to the ContentLocatorPart. If a value for the key already /// exists, the old value is overwritten by the new value. /// /// key /// value ///key or val is null ///a value for key is already present in the locator part public void Add(string key, string val) { if (key == null || val == null) { throw new ArgumentNullException(key == null ? "key" : "val"); } _nameValues.Add(key, val); FireDictionaryChanged(); } ////// Removes all name/value pairs from the ContentLocatorPart. /// public void Clear() { int count = _nameValues.Count; if (count > 0) { _nameValues.Clear(); // Only fire changed event if the dictionary actually changed FireDictionaryChanged(); } } ////// Returns whether or not a value of the key exists in this ContentLocatorPart. /// /// the key to check for ///true - yes, false - no public bool ContainsKey(string key) { return _nameValues.ContainsKey(key); } ////// Removes the key and its value from the ContentLocatorPart. /// /// key to be removed ///true - the key was found in the ContentLocatorPart, false o- it wasn't public bool Remove(string key) { bool exists = _nameValues.Remove(key); // Only fire changed event if the key was actually removed if (exists) { FireDictionaryChanged(); } return exists; } ////// Returns an enumerator for the key/value pairs in this ContentLocatorPart. /// ///an enumerator for the key/value pairs; never returns null IEnumerator IEnumerable.GetEnumerator() { return _nameValues.GetEnumerator(); } ////// Returns an enumerator forthe key/value pairs in this ContentLocatorPart. /// ///an enumerator for the key/value pairs; never returns null public IEnumerator> GetEnumerator() { return ((IEnumerable >)_nameValues).GetEnumerator(); } /// /// /// /// /// ////// key is null public bool TryGetValue(string key, out string value) { if (key == null) throw new ArgumentNullException("key"); return _nameValues.TryGetValue(key, out value); } ////// /// /// ///pair is null void ICollection>.Add(KeyValuePair pair) { ((ICollection >)_nameValues).Add(pair); } /// /// /// /// ////// pair is null bool ICollection>.Contains(KeyValuePair pair) { return ((ICollection >)_nameValues).Contains(pair); } /// /// /// /// ////// pair is null bool ICollection>.Remove(KeyValuePair pair) { return ((ICollection >)_nameValues).Remove(pair); } /// /// /// /// /// ///target is null ///startIndex is less than zero or greater than the lenght of target void ICollection>.CopyTo(KeyValuePair [] target, int startIndex) { if (target == null) throw new ArgumentNullException("target"); if (startIndex < 0 || startIndex > target.Length) throw new ArgumentOutOfRangeException("startIndex"); ((ICollection >)_nameValues).CopyTo(target, startIndex); } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //------------------------------------------------------ //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- #region Public Properties /// /// The number of name/value pairs in this ContentLocatorPart. /// ///count of name/value pairs public int Count { get { return _nameValues.Count; } } ////// Indexer provides lookup of values by key. Gets or sets the value /// in the ContentLocatorPart for the specified key. If the key does not exist /// in the ContentLocatorPart, /// /// key ///the value stored in this locator part for key public string this[string key] { get { if (key == null) { throw new ArgumentNullException("key"); } string value = null; _nameValues.TryGetValue(key, out value); return value; } set { if (key == null) { throw new ArgumentNullException("key"); } if (value == null) { throw new ArgumentNullException("value"); } string oldValue = null; _nameValues.TryGetValue(key, out oldValue); // If the new value is actually different, then we add it and fire // a change notification if ((oldValue == null) || (oldValue != value)) { _nameValues[key] = value; FireDictionaryChanged(); } } } ////// /// public bool IsReadOnly { get { return false; } } ////// Returns a collection of all the keys in this ContentLocatorPart. /// ///keys public ICollectionKeys { get { return _nameValues.Keys; } } /// /// Returns a collection of all the values in this ContentLocatorPart. /// ///values public ICollectionValues { get { return _nameValues.Values; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ //------------------------------------------------------ // // Internal Operators // //----------------------------------------------------- //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- #region Public Events /// /// /// public event PropertyChangedEventHandler PropertyChanged; #endregion Public Events //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods ////// Notify the owner this ContentLocatorPart has changed. /// private void FireDictionaryChanged() { if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(null)); } } #endregion Private Methods //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields ////// The internal data structure. /// private Dictionary_nameValues; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
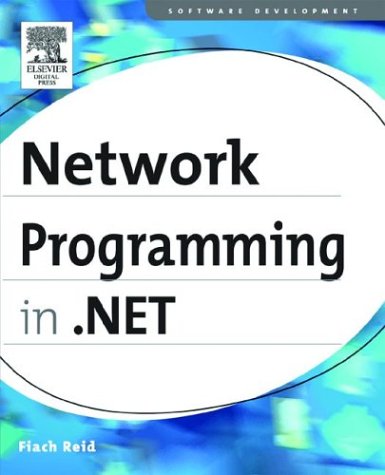
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResourceContainer.cs
- MetabaseServerConfig.cs
- MouseGestureConverter.cs
- ProfileModule.cs
- FileReservationCollection.cs
- TextEditorSelection.cs
- XDeferredAxisSource.cs
- SQLSingleStorage.cs
- DataTableCollection.cs
- SqlBulkCopyColumnMapping.cs
- NameGenerator.cs
- ConnectionStringSettings.cs
- DataSourceView.cs
- SafeArchiveContext.cs
- StatusStrip.cs
- OutOfProcStateClientManager.cs
- TreeNodeCollection.cs
- NetworkInformationPermission.cs
- SendingRequestEventArgs.cs
- Win32Exception.cs
- _FixedSizeReader.cs
- Rfc4050KeyFormatter.cs
- SynchronizedDispatch.cs
- NaturalLanguageHyphenator.cs
- ImpersonateTokenRef.cs
- ComplexLine.cs
- InstanceNormalEvent.cs
- AssemblyBuilderData.cs
- CompilationUnit.cs
- FixedFindEngine.cs
- XPathException.cs
- XslUrlEditor.cs
- SyndicationContent.cs
- LeaseManager.cs
- SkipQueryOptionExpression.cs
- ObsoleteAttribute.cs
- TextureBrush.cs
- InheritedPropertyChangedEventArgs.cs
- MemberHolder.cs
- AutomationElementCollection.cs
- WindowsSolidBrush.cs
- RegistryPermission.cs
- DbMetaDataFactory.cs
- BinaryMethodMessage.cs
- DispatcherExceptionFilterEventArgs.cs
- BitmapEffect.cs
- InProcStateClientManager.cs
- OrCondition.cs
- sqlser.cs
- SkinBuilder.cs
- ServerIdentity.cs
- Geometry.cs
- DBNull.cs
- ViewStateModeByIdAttribute.cs
- Closure.cs
- TcpTransportElement.cs
- PcmConverter.cs
- SchemaTableOptionalColumn.cs
- BmpBitmapDecoder.cs
- WindowsGraphicsWrapper.cs
- DataServiceQueryOfT.cs
- FrameSecurityDescriptor.cs
- WebServicesInteroperability.cs
- DesignerActionKeyboardBehavior.cs
- ListViewTableRow.cs
- PrintDialog.cs
- TypeConverterAttribute.cs
- WindowClosedEventArgs.cs
- PasswordBox.cs
- ColumnClickEvent.cs
- EntityDataSourceEntityTypeFilterItem.cs
- LinkArea.cs
- DigestComparer.cs
- DataColumn.cs
- SessionStateUtil.cs
- WSSecurityPolicy12.cs
- DataGridViewSelectedRowCollection.cs
- AnnotationAuthorChangedEventArgs.cs
- XPathNodeHelper.cs
- ObjectSet.cs
- IncrementalHitTester.cs
- AttachedAnnotation.cs
- HtmlContainerControl.cs
- HtmlEncodedRawTextWriter.cs
- XslVisitor.cs
- BitmapFrame.cs
- CodeTypeReferenceExpression.cs
- WebBrowserEvent.cs
- formatter.cs
- PageParser.cs
- GlobalAllocSafeHandle.cs
- Int32.cs
- StaticTextPointer.cs
- RecognitionEventArgs.cs
- WebFormDesignerActionService.cs
- VersionPair.cs
- RightsManagementEncryptedStream.cs
- StringResourceManager.cs
- GenericEnumConverter.cs
- PathSegmentCollection.cs