Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Controls / Primitives / ToolBarPanel.cs / 1 / ToolBarPanel.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows.Controls.Primitives; using System.Windows.Data; using System.Windows.Media; using System.Windows.Threading; namespace System.Windows.Controls.Primitives { ////// ToolBarPanel is used to arrange children within the ToolBar. It is used in the ToolBar style as items host. /// public class ToolBarPanel : StackPanel { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default constructor. /// public ToolBarPanel() { } #endregion Constructors #region Override methods ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// Need to lock down Visual tree during the callbacks. /// During this virtual call it is not valid to modify the Visual tree. /// protected override Visual GetVisualChild(int index) { if(index < 0 || index >= VisualChildrenCount) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } return _uiElementCollection != null ? _uiElementCollection[index] : base.GetVisualChild(index); } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected override int VisualChildrenCount { get { return _uiElementCollection != null ? _uiElementCollection.Count : base.VisualChildrenCount; } } ////// Measure the content and store the desired size of the content /// /// ///protected override Size MeasureOverride(Size constraint) { GenerateItems(); if (IsItemsHost) { // Clear the current visual collection _uiElementCollection.Clear(); // Clear the ToolBarOverflowPanel collection ToolBarOverflowPanel tbop = ToolBarOverflowPanel; if (tbop != null) tbop.Children.Clear(); ToolBar toolBar = ToolBar; bool calcMinSizeMode = !toolBar.IsValidMinLength; bool calcMaxSizeMode = !calcMinSizeMode && !toolBar.IsValidMaxLength; for (int i = 0; i < _generatedItemsCollection.Count; i++) { UIElement uiElement = _generatedItemsCollection[i]; bool addElement = false; OverflowMode overflowMode = ToolBar.GetOverflowMode(uiElement); // In case we measure minimum toolbar size - add only Never items if (calcMinSizeMode) { addElement = overflowMode == OverflowMode.Never; } // In case we measure maximum toolbar size - add Never and AsNeeded items else if (calcMaxSizeMode) { addElement = overflowMode != OverflowMode.Always; } else // place only the items that fit { // For now we add all items different from OverflowMode.Always // then we measure them we remove those don't fit addElement = overflowMode != OverflowMode.Always; } // place in the main panel (this) if (addElement) { _uiElementCollection.Add(uiElement); ToolBar.SetIsOverflowItem(uiElement, BooleanBoxes.FalseBox); } // place in the overflow panel (ToolBarOverflowPanel) else { // We only generate the items but they are not attached to the visual tree at this point // When overflow panel measures it adds all items which set this property: ToolBar.SetIsOverflowItem(uiElement, BooleanBoxes.TrueBox); } } } UIElementCollection children = _uiElementCollection; Size stackDesiredSize = new Size(); Size layoutSlotSize = constraint; bool fHorizontal = (Orientation == Orientation.Horizontal); double logicalAvailableWidth, logicalDesiredWidth; // // Initialize child sizing and iterator data // Allow children as much size as they want along the stack. // if (fHorizontal) { layoutSlotSize.Width = Double.PositiveInfinity; logicalAvailableWidth = constraint.Width; } else { layoutSlotSize.Height = Double.PositiveInfinity; logicalAvailableWidth = constraint.Height; } // // Iterate through children. // for (int i = 0, count = children.Count; i < count; ++i) { // Get next child. UIElement child = (UIElement)children[i]; // Measure the child. child.Measure(layoutSlotSize); Size childDesiredSize = child.DesiredSize; // Accumulate child size. if (fHorizontal) { stackDesiredSize.Width += childDesiredSize.Width; stackDesiredSize.Height = Math.Max(stackDesiredSize.Height, childDesiredSize.Height); } else { stackDesiredSize.Width = Math.Max(stackDesiredSize.Width, childDesiredSize.Width); stackDesiredSize.Height += childDesiredSize.Height; } } logicalDesiredWidth = fHorizontal ? stackDesiredSize.Width : stackDesiredSize.Height; // After we measure all the children - if they don't fit within constraint size // - we need to remove AsNeeded items from the end of the collection if (logicalDesiredWidth > logicalAvailableWidth) { for (int i = children.Count - 1; i >= 0; i--) { UIElement uiElement = (UIElement)children[i]; OverflowMode overflowMode = ToolBar.GetOverflowMode(uiElement); if (overflowMode == OverflowMode.AsNeeded) { logicalDesiredWidth -= fHorizontal ? uiElement.DesiredSize.Width : uiElement.DesiredSize.Height; ToolBar.SetIsOverflowItem(uiElement, BooleanBoxes.TrueBox); // Marked for overflow children.Remove(uiElement); // If the rest of the items fit - exit the loop if (logicalDesiredWidth <= logicalAvailableWidth) break; } } logicalDesiredWidth = Math.Max(logicalDesiredWidth, 0d); if (fHorizontal) { stackDesiredSize.Width = logicalDesiredWidth; } else { stackDesiredSize.Height = logicalDesiredWidth; } } // Update ToolBar.HasOverflowItemsProperty depend on number of overflow children ToolBar tb = ToolBar; if (tb != null) { tb.SetValue(ToolBar.HasOverflowItemsPropertyKey, tb.Items.Count > children.Count); } return stackDesiredSize; } /// /// Content arrangement. /// /// Arrange size protected override Size ArrangeOverride(Size arrangeSize) { UIElementCollection children = _uiElementCollection; bool fHorizontal = (Orientation == Orientation.Horizontal); Rect rcChild = new Rect(arrangeSize); double previousChildSize = 0.0d; // // Arrange and Position Children. // for (int i = 0, count = children.Count; i < count; ++i) { UIElement child = (UIElement)children[i]; if (fHorizontal) { rcChild.X += previousChildSize; previousChildSize = child.DesiredSize.Width; rcChild.Width = previousChildSize; rcChild.Height = Math.Max(arrangeSize.Height, child.DesiredSize.Height); } else { rcChild.Y += previousChildSize; previousChildSize = child.DesiredSize.Height; rcChild.Height = previousChildSize; rcChild.Width = Math.Max(arrangeSize.Width, child.DesiredSize.Width); } child.Arrange(rcChild); } return arrangeSize; } ////// ToolBarPanel sets bindings a on its Orientation property to its TemplatedParent if the /// property is not already set. /// internal override void OnPreApplyTemplate() { base.OnPreApplyTemplate(); if (TemplatedParent is ToolBar && !HasNonDefaultValue(OrientationProperty)) { Binding binding = new Binding(); binding.RelativeSource = RelativeSource.TemplatedParent; binding.Path = new PropertyPath(ToolBar.OrientationProperty); SetBinding(OrientationProperty, binding); } } #endregion Override methods // ItemsControl needs to access this collection for removing children internal UIElementCollection UIElementCollection { get { if (_uiElementCollection == null) { GenerateItems(); } return _uiElementCollection; } } #region private implementation private void OnItemsChanged(object sender, ItemsChangedEventArgs args) { switch (args.Action) { case NotifyCollectionChangedAction.Add: case NotifyCollectionChangedAction.Remove: case NotifyCollectionChangedAction.Reset: _childrenGenerated = false; GenerateItems(); break; } InvalidateMeasure(); } // Items generation is executed when we call this method for the first time // or if items collection changes // All generated items are cached in _generatedItemsCollection private void GenerateItems() { // If items have been generated already if (_childrenGenerated) return; ToolBar toolBar = ToolBar; if (IsItemsHost && toolBar != null) { if (_itemUIGenerator == null) { _itemUIGenerator = ((IItemContainerGenerator)toolBar.ItemContainerGenerator).GetItemContainerGeneratorForPanel(this); _itemUIGenerator.ItemsChanged += new ItemsChangedEventHandler(OnItemsChanged); } IItemContainerGenerator generator = (IItemContainerGenerator)_itemUIGenerator; generator.RemoveAll(); if (_uiElementCollection == null) _uiElementCollection = CreateUIElementCollection(null); else _uiElementCollection.Clear(); GeneratedItemsCollection.Clear(); // Clear the ToolBarOverflowPanel collection ToolBarOverflowPanel tbop = ToolBarOverflowPanel; if (tbop != null) tbop.Children.Clear(); // Generate all items and keep them in _generatedItemsCollection using (generator.StartAt(new GeneratorPosition(-1, 0), GeneratorDirection.Forward)) { UIElement uiElement; while ((uiElement = generator.GenerateNext() as UIElement) != null) { _generatedItemsCollection.Add(uiElement); _uiElementCollection.Add(uiElement); ToolBar.SetIsOverflowItem(uiElement, BooleanBoxes.FalseBox); generator.PrepareItemContainer(uiElement); } } _childrenGenerated = true; } else { _uiElementCollection = InternalChildren; } } private ToolBar ToolBar { get { return TemplatedParent as ToolBar; } } private ToolBarOverflowPanel ToolBarOverflowPanel { get { ToolBar tb = ToolBar; return tb == null ? null : tb.ToolBarOverflowPanel; } } internal ListGeneratedItemsCollection { get { if (_generatedItemsCollection == null) _generatedItemsCollection = new List (5); return _generatedItemsCollection; } } #endregion private implementation #region private data private List _generatedItemsCollection; private UIElementCollection _uiElementCollection; private ItemContainerGenerator _itemUIGenerator; private bool _childrenGenerated; #endregion private data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows.Controls.Primitives; using System.Windows.Data; using System.Windows.Media; using System.Windows.Threading; namespace System.Windows.Controls.Primitives { /// /// ToolBarPanel is used to arrange children within the ToolBar. It is used in the ToolBar style as items host. /// public class ToolBarPanel : StackPanel { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default constructor. /// public ToolBarPanel() { } #endregion Constructors #region Override methods ////// Derived class must implement to support Visual children. The method must return /// the child at the specified index. Index must be between 0 and GetVisualChildrenCount-1. /// /// By default a Visual does not have any children. /// /// Remark: /// Need to lock down Visual tree during the callbacks. /// During this virtual call it is not valid to modify the Visual tree. /// protected override Visual GetVisualChild(int index) { if(index < 0 || index >= VisualChildrenCount) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } return _uiElementCollection != null ? _uiElementCollection[index] : base.GetVisualChild(index); } ////// Derived classes override this property to enable the Visual code to enumerate /// the Visual children. Derived classes need to return the number of children /// from this method. /// /// By default a Visual does not have any children. /// /// Remark: During this virtual method the Visual tree must not be modified. /// protected override int VisualChildrenCount { get { return _uiElementCollection != null ? _uiElementCollection.Count : base.VisualChildrenCount; } } ////// Measure the content and store the desired size of the content /// /// ///protected override Size MeasureOverride(Size constraint) { GenerateItems(); if (IsItemsHost) { // Clear the current visual collection _uiElementCollection.Clear(); // Clear the ToolBarOverflowPanel collection ToolBarOverflowPanel tbop = ToolBarOverflowPanel; if (tbop != null) tbop.Children.Clear(); ToolBar toolBar = ToolBar; bool calcMinSizeMode = !toolBar.IsValidMinLength; bool calcMaxSizeMode = !calcMinSizeMode && !toolBar.IsValidMaxLength; for (int i = 0; i < _generatedItemsCollection.Count; i++) { UIElement uiElement = _generatedItemsCollection[i]; bool addElement = false; OverflowMode overflowMode = ToolBar.GetOverflowMode(uiElement); // In case we measure minimum toolbar size - add only Never items if (calcMinSizeMode) { addElement = overflowMode == OverflowMode.Never; } // In case we measure maximum toolbar size - add Never and AsNeeded items else if (calcMaxSizeMode) { addElement = overflowMode != OverflowMode.Always; } else // place only the items that fit { // For now we add all items different from OverflowMode.Always // then we measure them we remove those don't fit addElement = overflowMode != OverflowMode.Always; } // place in the main panel (this) if (addElement) { _uiElementCollection.Add(uiElement); ToolBar.SetIsOverflowItem(uiElement, BooleanBoxes.FalseBox); } // place in the overflow panel (ToolBarOverflowPanel) else { // We only generate the items but they are not attached to the visual tree at this point // When overflow panel measures it adds all items which set this property: ToolBar.SetIsOverflowItem(uiElement, BooleanBoxes.TrueBox); } } } UIElementCollection children = _uiElementCollection; Size stackDesiredSize = new Size(); Size layoutSlotSize = constraint; bool fHorizontal = (Orientation == Orientation.Horizontal); double logicalAvailableWidth, logicalDesiredWidth; // // Initialize child sizing and iterator data // Allow children as much size as they want along the stack. // if (fHorizontal) { layoutSlotSize.Width = Double.PositiveInfinity; logicalAvailableWidth = constraint.Width; } else { layoutSlotSize.Height = Double.PositiveInfinity; logicalAvailableWidth = constraint.Height; } // // Iterate through children. // for (int i = 0, count = children.Count; i < count; ++i) { // Get next child. UIElement child = (UIElement)children[i]; // Measure the child. child.Measure(layoutSlotSize); Size childDesiredSize = child.DesiredSize; // Accumulate child size. if (fHorizontal) { stackDesiredSize.Width += childDesiredSize.Width; stackDesiredSize.Height = Math.Max(stackDesiredSize.Height, childDesiredSize.Height); } else { stackDesiredSize.Width = Math.Max(stackDesiredSize.Width, childDesiredSize.Width); stackDesiredSize.Height += childDesiredSize.Height; } } logicalDesiredWidth = fHorizontal ? stackDesiredSize.Width : stackDesiredSize.Height; // After we measure all the children - if they don't fit within constraint size // - we need to remove AsNeeded items from the end of the collection if (logicalDesiredWidth > logicalAvailableWidth) { for (int i = children.Count - 1; i >= 0; i--) { UIElement uiElement = (UIElement)children[i]; OverflowMode overflowMode = ToolBar.GetOverflowMode(uiElement); if (overflowMode == OverflowMode.AsNeeded) { logicalDesiredWidth -= fHorizontal ? uiElement.DesiredSize.Width : uiElement.DesiredSize.Height; ToolBar.SetIsOverflowItem(uiElement, BooleanBoxes.TrueBox); // Marked for overflow children.Remove(uiElement); // If the rest of the items fit - exit the loop if (logicalDesiredWidth <= logicalAvailableWidth) break; } } logicalDesiredWidth = Math.Max(logicalDesiredWidth, 0d); if (fHorizontal) { stackDesiredSize.Width = logicalDesiredWidth; } else { stackDesiredSize.Height = logicalDesiredWidth; } } // Update ToolBar.HasOverflowItemsProperty depend on number of overflow children ToolBar tb = ToolBar; if (tb != null) { tb.SetValue(ToolBar.HasOverflowItemsPropertyKey, tb.Items.Count > children.Count); } return stackDesiredSize; } /// /// Content arrangement. /// /// Arrange size protected override Size ArrangeOverride(Size arrangeSize) { UIElementCollection children = _uiElementCollection; bool fHorizontal = (Orientation == Orientation.Horizontal); Rect rcChild = new Rect(arrangeSize); double previousChildSize = 0.0d; // // Arrange and Position Children. // for (int i = 0, count = children.Count; i < count; ++i) { UIElement child = (UIElement)children[i]; if (fHorizontal) { rcChild.X += previousChildSize; previousChildSize = child.DesiredSize.Width; rcChild.Width = previousChildSize; rcChild.Height = Math.Max(arrangeSize.Height, child.DesiredSize.Height); } else { rcChild.Y += previousChildSize; previousChildSize = child.DesiredSize.Height; rcChild.Height = previousChildSize; rcChild.Width = Math.Max(arrangeSize.Width, child.DesiredSize.Width); } child.Arrange(rcChild); } return arrangeSize; } ////// ToolBarPanel sets bindings a on its Orientation property to its TemplatedParent if the /// property is not already set. /// internal override void OnPreApplyTemplate() { base.OnPreApplyTemplate(); if (TemplatedParent is ToolBar && !HasNonDefaultValue(OrientationProperty)) { Binding binding = new Binding(); binding.RelativeSource = RelativeSource.TemplatedParent; binding.Path = new PropertyPath(ToolBar.OrientationProperty); SetBinding(OrientationProperty, binding); } } #endregion Override methods // ItemsControl needs to access this collection for removing children internal UIElementCollection UIElementCollection { get { if (_uiElementCollection == null) { GenerateItems(); } return _uiElementCollection; } } #region private implementation private void OnItemsChanged(object sender, ItemsChangedEventArgs args) { switch (args.Action) { case NotifyCollectionChangedAction.Add: case NotifyCollectionChangedAction.Remove: case NotifyCollectionChangedAction.Reset: _childrenGenerated = false; GenerateItems(); break; } InvalidateMeasure(); } // Items generation is executed when we call this method for the first time // or if items collection changes // All generated items are cached in _generatedItemsCollection private void GenerateItems() { // If items have been generated already if (_childrenGenerated) return; ToolBar toolBar = ToolBar; if (IsItemsHost && toolBar != null) { if (_itemUIGenerator == null) { _itemUIGenerator = ((IItemContainerGenerator)toolBar.ItemContainerGenerator).GetItemContainerGeneratorForPanel(this); _itemUIGenerator.ItemsChanged += new ItemsChangedEventHandler(OnItemsChanged); } IItemContainerGenerator generator = (IItemContainerGenerator)_itemUIGenerator; generator.RemoveAll(); if (_uiElementCollection == null) _uiElementCollection = CreateUIElementCollection(null); else _uiElementCollection.Clear(); GeneratedItemsCollection.Clear(); // Clear the ToolBarOverflowPanel collection ToolBarOverflowPanel tbop = ToolBarOverflowPanel; if (tbop != null) tbop.Children.Clear(); // Generate all items and keep them in _generatedItemsCollection using (generator.StartAt(new GeneratorPosition(-1, 0), GeneratorDirection.Forward)) { UIElement uiElement; while ((uiElement = generator.GenerateNext() as UIElement) != null) { _generatedItemsCollection.Add(uiElement); _uiElementCollection.Add(uiElement); ToolBar.SetIsOverflowItem(uiElement, BooleanBoxes.FalseBox); generator.PrepareItemContainer(uiElement); } } _childrenGenerated = true; } else { _uiElementCollection = InternalChildren; } } private ToolBar ToolBar { get { return TemplatedParent as ToolBar; } } private ToolBarOverflowPanel ToolBarOverflowPanel { get { ToolBar tb = ToolBar; return tb == null ? null : tb.ToolBarOverflowPanel; } } internal ListGeneratedItemsCollection { get { if (_generatedItemsCollection == null) _generatedItemsCollection = new List (5); return _generatedItemsCollection; } } #endregion private implementation #region private data private List _generatedItemsCollection; private UIElementCollection _uiElementCollection; private ItemContainerGenerator _itemUIGenerator; private bool _childrenGenerated; #endregion private data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
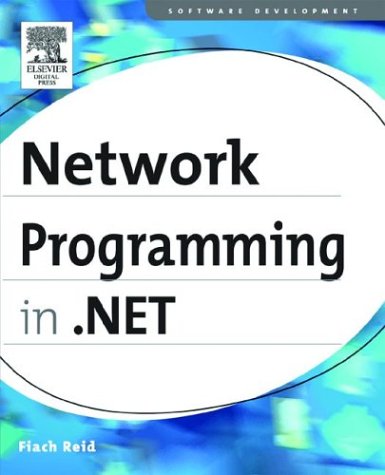
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerUtils.cs
- UniqueIdentifierService.cs
- ControlHelper.cs
- StringDictionaryCodeDomSerializer.cs
- ImpersonateTokenRef.cs
- TextSearch.cs
- ReceiveErrorHandling.cs
- ConsoleEntryPoint.cs
- WhitespaceRule.cs
- EntityDataSourceSelectingEventArgs.cs
- WmpBitmapEncoder.cs
- WebPartsSection.cs
- GridSplitter.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- ProtectedProviderSettings.cs
- DbMetaDataCollectionNames.cs
- WizardStepBase.cs
- DesignerSerializerAttribute.cs
- TileBrush.cs
- XPathQilFactory.cs
- AssignDesigner.xaml.cs
- QilInvokeLateBound.cs
- BuildProvider.cs
- MapPathBasedVirtualPathProvider.cs
- WebHttpSecurityModeHelper.cs
- CodeAttributeDeclaration.cs
- StickyNoteHelper.cs
- HtmlLink.cs
- LinkedResourceCollection.cs
- MaskPropertyEditor.cs
- MailDefinition.cs
- Compilation.cs
- CodeIdentifiers.cs
- CheckBoxPopupAdapter.cs
- DocumentReferenceCollection.cs
- DesignerSerializerAttribute.cs
- PasswordValidationException.cs
- XPathNodeList.cs
- FontUnitConverter.cs
- BufferedWebEventProvider.cs
- ColorAnimation.cs
- XmlBaseReader.cs
- MultiTrigger.cs
- ExtendedProtectionPolicy.cs
- ConfigurationElement.cs
- SamlAssertionDirectKeyIdentifierClause.cs
- PreviousTrackingServiceAttribute.cs
- PrintControllerWithStatusDialog.cs
- ErrorActivity.cs
- MessageBox.cs
- MachineKeyConverter.cs
- httpstaticobjectscollection.cs
- __Filters.cs
- OpCodes.cs
- StorageTypeMapping.cs
- BitArray.cs
- NavigationCommands.cs
- ResXResourceReader.cs
- X509CertificateCollection.cs
- SimpleWebHandlerParser.cs
- Vector.cs
- EDesignUtil.cs
- DefaultPropertiesToSend.cs
- GuidTagList.cs
- ToolStripManager.cs
- AndCondition.cs
- ReferencedAssembly.cs
- DeflateEmulationStream.cs
- BooleanExpr.cs
- PreviewPrintController.cs
- ParallelQuery.cs
- TearOffProxy.cs
- PrivateFontCollection.cs
- _RegBlobWebProxyDataBuilder.cs
- ServiceOperationDetailViewControl.cs
- ProfileProvider.cs
- TextEndOfParagraph.cs
- SchemaImporterExtension.cs
- OutputCacheProfile.cs
- ToolStripDropDownItem.cs
- InkSerializer.cs
- ExpressionEditor.cs
- DataTablePropertyDescriptor.cs
- ControlBuilderAttribute.cs
- XmlSchemaSequence.cs
- HttpSessionStateWrapper.cs
- FindCriteriaElement.cs
- PersonalizablePropertyEntry.cs
- BeginEvent.cs
- TrustManager.cs
- Message.cs
- XNameTypeConverter.cs
- AsymmetricKeyExchangeDeformatter.cs
- DBSchemaTable.cs
- X509Extension.cs
- SignedInfo.cs
- AuthenticationModuleElementCollection.cs
- AnyReturnReader.cs
- UIElement3DAutomationPeer.cs
- ReliabilityContractAttribute.cs