Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / StartUpEventArgs.cs / 1 / StartUpEventArgs.cs
//-------------------------------------------------------------------------------------------------- // File: StartupEventArgs.cs // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // Description: // This event is fired when the application starts - once that application’s Run() // method has been called. // // The developer will typically hook this event if they want to take action at startup time // // History: // 08/10/04: kusumav Moved out of Application.cs to its own separate file. // 05/09/05: hamidm Created StartupEventArgs.cs and renamed StartingUpCancelEventArgs to StartupEventArgs // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Interop; using MS.Internal.PresentationFramework; using System.Runtime.CompilerServices; using MS.Internal; using MS.Internal.AppModel; namespace System.Windows { ////// Event args for Startup event /// public class StartupEventArgs : EventArgs { ////// constructor /// internal StartupEventArgs() { _performDefaultAction = true; } ////// Command Line arguments /// public String[] Args { get { if (_args == null) { _args = GetCmdLineArgs(); } return _args; } } internal bool PerformDefaultAction { get { return _performDefaultAction; } set { _performDefaultAction = value; } } private string[] GetCmdLineArgs() { string[] retValue = null; if (!BrowserInteropHelper.IsBrowserHosted && ( ( Application.Current.MimeType != MimeType.Application ) || ! IsOnNetworkShareForDeployedApps() )) { string[] args = Environment.GetCommandLineArgs(); Invariant.Assert(args.Length >= 1); int newLength = args.Length - 1; newLength = (newLength >=0 ? newLength : 0); retValue = new string[newLength]; for (int i = 1; i < args.Length; i++) { retValue[i-1] = args[i]; } } else { retValue = new string[0]; } return retValue; } // // Put this into a separate Method to avoid loading of this code at JIT time. // // // Explicitly tell the compiler that we don't want to be inlined. // This will prevent loading of system.deployment unless we are a click-once app. // [MethodImplAttribute (MethodImplOptions.NoInlining )] private bool IsOnNetworkShareForDeployedApps() { return System.Deployment.Application.ApplicationDeployment.IsNetworkDeployed ; } private String[] _args; private bool _performDefaultAction; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //-------------------------------------------------------------------------------------------------- // File: StartupEventArgs.cs // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // Description: // This event is fired when the application starts - once that application’s Run() // method has been called. // // The developer will typically hook this event if they want to take action at startup time // // History: // 08/10/04: kusumav Moved out of Application.cs to its own separate file. // 05/09/05: hamidm Created StartupEventArgs.cs and renamed StartingUpCancelEventArgs to StartupEventArgs // //--------------------------------------------------------------------------- using System.ComponentModel; using System.Windows.Interop; using MS.Internal.PresentationFramework; using System.Runtime.CompilerServices; using MS.Internal; using MS.Internal.AppModel; namespace System.Windows { ////// Event args for Startup event /// public class StartupEventArgs : EventArgs { ////// constructor /// internal StartupEventArgs() { _performDefaultAction = true; } ////// Command Line arguments /// public String[] Args { get { if (_args == null) { _args = GetCmdLineArgs(); } return _args; } } internal bool PerformDefaultAction { get { return _performDefaultAction; } set { _performDefaultAction = value; } } private string[] GetCmdLineArgs() { string[] retValue = null; if (!BrowserInteropHelper.IsBrowserHosted && ( ( Application.Current.MimeType != MimeType.Application ) || ! IsOnNetworkShareForDeployedApps() )) { string[] args = Environment.GetCommandLineArgs(); Invariant.Assert(args.Length >= 1); int newLength = args.Length - 1; newLength = (newLength >=0 ? newLength : 0); retValue = new string[newLength]; for (int i = 1; i < args.Length; i++) { retValue[i-1] = args[i]; } } else { retValue = new string[0]; } return retValue; } // // Put this into a separate Method to avoid loading of this code at JIT time. // // // Explicitly tell the compiler that we don't want to be inlined. // This will prevent loading of system.deployment unless we are a click-once app. // [MethodImplAttribute (MethodImplOptions.NoInlining )] private bool IsOnNetworkShareForDeployedApps() { return System.Deployment.Application.ApplicationDeployment.IsNetworkDeployed ; } private String[] _args; private bool _performDefaultAction; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
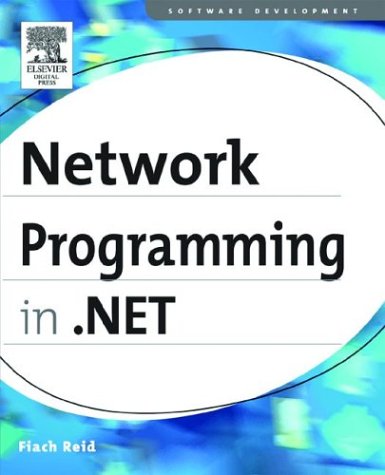
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Stack.cs
- XsltOutput.cs
- MasterPageBuildProvider.cs
- DbConnectionOptions.cs
- CompositeCollectionView.cs
- ContentDesigner.cs
- ViewBase.cs
- SqlDataSourceCustomCommandPanel.cs
- DataGridRow.cs
- ScrollChrome.cs
- ToolStripDropTargetManager.cs
- PeerContact.cs
- WebPartDescription.cs
- ActivationServices.cs
- ColumnMapProcessor.cs
- NavigationPropertyEmitter.cs
- mediaeventargs.cs
- ManagementScope.cs
- LifetimeServices.cs
- XPathScanner.cs
- RequestContext.cs
- ListManagerBindingsCollection.cs
- ByValueEqualityComparer.cs
- SwitchLevelAttribute.cs
- Trace.cs
- WebConfigurationHostFileChange.cs
- CodeAttachEventStatement.cs
- LinqDataSourceContextEventArgs.cs
- SoapFormatterSinks.cs
- MediaElement.cs
- TreeViewBindingsEditorForm.cs
- Vector3DAnimationBase.cs
- IncrementalCompileAnalyzer.cs
- ConnectionOrientedTransportChannelListener.cs
- LineProperties.cs
- EventLogPermission.cs
- MethodAccessException.cs
- Function.cs
- GeneratedContractType.cs
- ListViewSelectEventArgs.cs
- ApplicationException.cs
- BufferAllocator.cs
- ProcessModuleCollection.cs
- DesignTimeType.cs
- HttpWebRequestElement.cs
- ApplicationDirectory.cs
- ToolStripDropDownClosingEventArgs.cs
- RowVisual.cs
- AppDomainAttributes.cs
- HMACMD5.cs
- SchemaMapping.cs
- UnsafeNativeMethods.cs
- Menu.cs
- WebPartDescription.cs
- Point3DAnimation.cs
- DataControlLinkButton.cs
- xmlsaver.cs
- AddInStore.cs
- BinaryFormatterWriter.cs
- DataGridPagerStyle.cs
- ClientRolePrincipal.cs
- HttpGetServerProtocol.cs
- InputScopeNameConverter.cs
- OutOfProcStateClientManager.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- ClockGroup.cs
- PageThemeParser.cs
- FormsAuthenticationUser.cs
- ListViewCancelEventArgs.cs
- ThumbButtonInfo.cs
- XmlUTF8TextWriter.cs
- ModelPropertyImpl.cs
- EncryptedPackageFilter.cs
- GroupLabel.cs
- TracePayload.cs
- PartManifestEntry.cs
- WebPart.cs
- FileRecordSequence.cs
- MembershipValidatePasswordEventArgs.cs
- QilVisitor.cs
- AnnotationDocumentPaginator.cs
- ByteStream.cs
- XomlCompilerHelpers.cs
- SystemResources.cs
- CodeThrowExceptionStatement.cs
- HtmlTernaryTree.cs
- XmlSchemaParticle.cs
- MsmqMessage.cs
- WebPartConnection.cs
- SnapshotChangeTrackingStrategy.cs
- PolicyUtility.cs
- SchemaObjectWriter.cs
- StateMachineWorkflow.cs
- SafeNativeMethods.cs
- OrderedParallelQuery.cs
- SqlCommandSet.cs
- SqlExpander.cs
- OleDbPermission.cs
- DataViewManagerListItemTypeDescriptor.cs
- MimeXmlReflector.cs