Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CompMod / System / ComponentModel / Design / Serialization / ContextStack.cs / 1 / ContextStack.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.Collections; using System.Security.Permissions; ////// A context stack is an object that can be used by serializers /// to push various context objects. Serialization is often /// a deeply nested operation, involving many different /// serialization classes. These classes often need additional /// context information when performing serialization. As /// an example, an object with a property named "Enabled" may have /// a data type of System.Boolean. If a serializer is writing /// this value to a data stream it may want to know what property /// it is writing. It won't have this information, however, because /// it is only instructed to write the boolean value. In this /// case the parent serializer may push a PropertyDescriptor /// pointing to the "Enabled" property on the context stack. /// What objects get pushed on this stack are up to the /// individual serializer objects. /// [HostProtection(SharedState = true)] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name = "FullTrust")] public sealed class ContextStack { private ArrayList contextStack; ////// Retrieves the current object on the stack, or null /// if no objects have been pushed. /// public object Current { get { if (contextStack != null && contextStack.Count > 0) { return contextStack[contextStack.Count - 1]; } return null; } } ////// Retrieves the object on the stack at the given /// level, or null if no object exists at that level. /// public object this[int level] { get { if (level < 0) { throw new ArgumentOutOfRangeException("level"); } if (contextStack != null && level < contextStack.Count) { return contextStack[contextStack.Count - 1 - level]; } return null; } } ////// Retrieves the first object on the stack that /// inherits from or implements the given type, or /// null if no object on the stack implements the type. /// public object this[Type type] { get { if (type == null) { throw new ArgumentNullException("type"); } if (contextStack != null) { int level = contextStack.Count; while(level > 0) { object value = contextStack[--level]; if (type.IsInstanceOfType(value)) { return value; } } } return null; } } ////// Appends an object to the end of the stack, rather than pushing it /// onto the top of the stack. This method allows a serializer to communicate /// with other serializers by adding contextual data that does not have to /// be popped in order. There is no way to remove an object that was /// appended to the end of the stack without popping all other objects. /// public void Append(object context) { if (context == null) { throw new ArgumentNullException("context"); } if (contextStack == null) { contextStack = new ArrayList(); } contextStack.Insert(0, context); } ////// Pops the current object off of the stack, returning /// its value. /// public object Pop() { object context = null; if (contextStack != null && contextStack.Count > 0) { int idx = contextStack.Count - 1; context = contextStack[idx]; contextStack.RemoveAt(idx); } return context; } ////// Pushes the given object onto the stack. /// public void Push(object context) { if (context == null) { throw new ArgumentNullException("context"); } if (contextStack == null) { contextStack = new ArrayList(); } contextStack.Add(context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.Collections; using System.Security.Permissions; ////// A context stack is an object that can be used by serializers /// to push various context objects. Serialization is often /// a deeply nested operation, involving many different /// serialization classes. These classes often need additional /// context information when performing serialization. As /// an example, an object with a property named "Enabled" may have /// a data type of System.Boolean. If a serializer is writing /// this value to a data stream it may want to know what property /// it is writing. It won't have this information, however, because /// it is only instructed to write the boolean value. In this /// case the parent serializer may push a PropertyDescriptor /// pointing to the "Enabled" property on the context stack. /// What objects get pushed on this stack are up to the /// individual serializer objects. /// [HostProtection(SharedState = true)] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name = "FullTrust")] public sealed class ContextStack { private ArrayList contextStack; ////// Retrieves the current object on the stack, or null /// if no objects have been pushed. /// public object Current { get { if (contextStack != null && contextStack.Count > 0) { return contextStack[contextStack.Count - 1]; } return null; } } ////// Retrieves the object on the stack at the given /// level, or null if no object exists at that level. /// public object this[int level] { get { if (level < 0) { throw new ArgumentOutOfRangeException("level"); } if (contextStack != null && level < contextStack.Count) { return contextStack[contextStack.Count - 1 - level]; } return null; } } ////// Retrieves the first object on the stack that /// inherits from or implements the given type, or /// null if no object on the stack implements the type. /// public object this[Type type] { get { if (type == null) { throw new ArgumentNullException("type"); } if (contextStack != null) { int level = contextStack.Count; while(level > 0) { object value = contextStack[--level]; if (type.IsInstanceOfType(value)) { return value; } } } return null; } } ////// Appends an object to the end of the stack, rather than pushing it /// onto the top of the stack. This method allows a serializer to communicate /// with other serializers by adding contextual data that does not have to /// be popped in order. There is no way to remove an object that was /// appended to the end of the stack without popping all other objects. /// public void Append(object context) { if (context == null) { throw new ArgumentNullException("context"); } if (contextStack == null) { contextStack = new ArrayList(); } contextStack.Insert(0, context); } ////// Pops the current object off of the stack, returning /// its value. /// public object Pop() { object context = null; if (contextStack != null && contextStack.Count > 0) { int idx = contextStack.Count - 1; context = contextStack[idx]; contextStack.RemoveAt(idx); } return context; } ////// Pushes the given object onto the stack. /// public void Push(object context) { if (context == null) { throw new ArgumentNullException("context"); } if (contextStack == null) { contextStack = new ArrayList(); } contextStack.Add(context); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
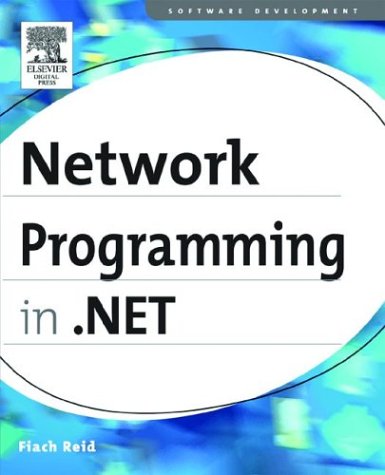
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DetailsViewModeEventArgs.cs
- AsymmetricSignatureDeformatter.cs
- XmlSigningNodeWriter.cs
- SymLanguageVendor.cs
- TdsParameterSetter.cs
- FocusWithinProperty.cs
- SafeMarshalContext.cs
- DataGridViewAdvancedBorderStyle.cs
- ComponentChangingEvent.cs
- TypedReference.cs
- ComplexTypeEmitter.cs
- CalendarTable.cs
- Viewport3DVisual.cs
- PathFigureCollectionValueSerializer.cs
- EncryptedData.cs
- HandoffBehavior.cs
- NativeBuffer.cs
- ValidatorCollection.cs
- SystemNetHelpers.cs
- HtmlLink.cs
- ExecutionEngineException.cs
- Inline.cs
- ButtonChrome.cs
- OperatingSystem.cs
- RuntimeConfigLKG.cs
- PrimaryKeyTypeConverter.cs
- CodeDirectoryCompiler.cs
- ConnectionStringEditor.cs
- RoleGroupCollection.cs
- EventSchemaTraceListener.cs
- MaskedTextBoxTextEditor.cs
- Helper.cs
- CrossAppDomainChannel.cs
- Helper.cs
- activationcontext.cs
- ToolboxService.cs
- MenuItemStyle.cs
- CodeGotoStatement.cs
- RegionData.cs
- ManagementScope.cs
- QueryCacheEntry.cs
- BamlBinaryWriter.cs
- HostedHttpContext.cs
- RepeatInfo.cs
- KeyEventArgs.cs
- DefaultShape.cs
- PrefixQName.cs
- TraceInternal.cs
- TextDecorationLocationValidation.cs
- RemoteWebConfigurationHost.cs
- XmlnsPrefixAttribute.cs
- SqlConnectionPoolGroupProviderInfo.cs
- DateTimeStorage.cs
- MailAddressCollection.cs
- FixUpCollection.cs
- PresentationAppDomainManager.cs
- DataSourceCache.cs
- DetailsViewDeleteEventArgs.cs
- HostVisual.cs
- WindowsTab.cs
- ProxyOperationRuntime.cs
- ColumnHeader.cs
- HistoryEventArgs.cs
- AccessDataSourceView.cs
- WhitespaceSignificantCollectionAttribute.cs
- VersionedStreamOwner.cs
- DbDeleteCommandTree.cs
- Brushes.cs
- Ipv6Element.cs
- FileUpload.cs
- webclient.cs
- DataPagerField.cs
- QueryOpeningEnumerator.cs
- EventBuilder.cs
- XmlAtomicValue.cs
- TextSelection.cs
- RewritingValidator.cs
- IDispatchConstantAttribute.cs
- TextAdaptor.cs
- SystemResourceHost.cs
- StatusBarPanel.cs
- PaperSource.cs
- ValidateNames.cs
- UnsafeNativeMethods.cs
- XsdBuildProvider.cs
- MsmqHostedTransportConfiguration.cs
- TextEditorThreadLocalStore.cs
- StringDictionary.cs
- DisableDpiAwarenessAttribute.cs
- SecureConversationServiceElement.cs
- ConfigXmlCDataSection.cs
- StatusBarPanelClickEvent.cs
- IconHelper.cs
- MessageHeaders.cs
- WmlValidationSummaryAdapter.cs
- PolyBezierSegment.cs
- TabletCollection.cs
- WorkflowRuntimeSection.cs
- Renderer.cs
- MultilineStringConverter.cs