Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Xml / System / Xml / Core / QueryOutputWriter.cs / 1 / QueryOutputWriter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.Globalization; using System.IO; using System.Collections.Generic; using System.Xml.Schema; using System.Diagnostics; ////// This writer wraps an XmlRawWriter and inserts additional lexical information into the resulting /// Xml 1.0 document: /// 1. CData sections /// 2. DocType declaration /// /// It also performs well-formed document checks if standalone="yes" and/or a doc-type-decl is output. /// internal class QueryOutputWriter : XmlRawWriter { private XmlRawWriter wrapped; private bool inCDataSection; private DictionarylookupCDataElems; private BitStack bitsCData; private XmlQualifiedName qnameCData; private bool outputDocType, checkWellFormedDoc, hasDocElem, inAttr; private string systemId, publicId; private int depth; public QueryOutputWriter(XmlRawWriter writer, XmlWriterSettings settings) { this.wrapped = writer; this.systemId = settings.DocTypeSystem; this.publicId = settings.DocTypePublic; if (settings.OutputMethod == XmlOutputMethod.Xml) { // Xml output method shouldn't output doc-type-decl if system ID is not defined (even if public ID is) // Only check for well-formed document if output method is xml if (this.systemId != null) { this.outputDocType = true; this.checkWellFormedDoc = true; } // Check for well-formed document if standalone="yes" in an auto-generated xml declaration if (settings.AutoXmlDeclaration && settings.Standalone == XmlStandalone.Yes) this.checkWellFormedDoc = true; if (settings.CDataSectionElements.Count > 0) { this.bitsCData = new BitStack(); this.lookupCDataElems = new Dictionary (); this.qnameCData = new XmlQualifiedName(); // Add each element name to the lookup table foreach (XmlQualifiedName name in settings.CDataSectionElements) { this.lookupCDataElems[name] = 0; } this.bitsCData.PushBit(false); } } else if (settings.OutputMethod == XmlOutputMethod.Html) { // Html output method should output doc-type-decl if system ID or public ID is defined if (this.systemId != null || this.publicId != null) this.outputDocType = true; } } //----------------------------------------------- // XmlWriter interface //----------------------------------------------- /// /// Get and set the namespace resolver that's used by this RawWriter to resolve prefixes. /// internal override IXmlNamespaceResolver NamespaceResolver { get { return this.resolver; } set { this.resolver = value; this.wrapped.NamespaceResolver = value; } } ////// Write the xml declaration. This must be the first call. /// internal override void WriteXmlDeclaration(XmlStandalone standalone) { this.wrapped.WriteXmlDeclaration(standalone); } internal override void WriteXmlDeclaration(string xmldecl) { this.wrapped.WriteXmlDeclaration(xmldecl); } ////// Return settings provided to factory. /// public override XmlWriterSettings Settings { get { XmlWriterSettings settings = this.wrapped.Settings; settings.ReadOnly = false; settings.DocTypeSystem = this.systemId; settings.DocTypePublic = this.publicId; settings.ReadOnly = true; return settings; } } ////// Suppress this explicit call to WriteDocType if information was provided by XmlWriterSettings. /// public override void WriteDocType(string name, string pubid, string sysid, string subset) { if (this.publicId == null && this.systemId == null) { Debug.Assert(!this.outputDocType); this.wrapped.WriteDocType(name, pubid, sysid, subset); } } ////// Check well-formedness, possibly output doc-type-decl, and determine whether this element is a /// CData section element. /// public override void WriteStartElement(string prefix, string localName, string ns) { EndCDataSection(); if (this.checkWellFormedDoc) { // Don't allow multiple document elements if (this.depth == 0 && this.hasDocElem) throw new XmlException(Res.Xml_NoMultipleRoots, string.Empty); this.depth++; this.hasDocElem = true; } // Output doc-type declaration immediately before first element is output if (this.outputDocType) { this.wrapped.WriteDocType( prefix.Length != 0 ? prefix + ":" + localName : localName, this.publicId, this.systemId, null); this.outputDocType = false; } this.wrapped.WriteStartElement(prefix, localName, ns); if (this.lookupCDataElems != null) { // Determine whether this element is a CData section element this.qnameCData.Init(localName, ns); this.bitsCData.PushBit(this.lookupCDataElems.ContainsKey(this.qnameCData)); } } internal override void WriteEndElement(string prefix, string localName, string ns) { EndCDataSection(); this.wrapped.WriteEndElement(prefix, localName, ns); if (this.checkWellFormedDoc) this.depth--; if (this.lookupCDataElems != null) this.bitsCData.PopBit(); } internal override void WriteFullEndElement(string prefix, string localName, string ns) { EndCDataSection(); this.wrapped.WriteFullEndElement(prefix, localName, ns); if (this.checkWellFormedDoc) this.depth--; if (this.lookupCDataElems != null) this.bitsCData.PopBit(); } internal override void StartElementContent() { this.wrapped.StartElementContent(); } public override void WriteStartAttribute(string prefix, string localName, string ns) { this.inAttr = true; this.wrapped.WriteStartAttribute(prefix, localName, ns); } public override void WriteEndAttribute() { this.inAttr = false; this.wrapped.WriteEndAttribute(); } internal override void WriteNamespaceDeclaration(string prefix, string ns) { this.wrapped.WriteNamespaceDeclaration(prefix, ns); } public override void WriteCData(string text) { this.wrapped.WriteCData(text); } public override void WriteComment(string text) { EndCDataSection(); this.wrapped.WriteComment(text); } public override void WriteProcessingInstruction(string name, string text) { EndCDataSection(); this.wrapped.WriteProcessingInstruction(name, text); } public override void WriteWhitespace(string ws) { if (!this.inAttr && (this.inCDataSection || StartCDataSection())) this.wrapped.WriteCData(ws); else this.wrapped.WriteWhitespace(ws); } public override void WriteString(string text) { if (!this.inAttr && (this.inCDataSection || StartCDataSection())) this.wrapped.WriteCData(text); else this.wrapped.WriteString(text); } public override void WriteChars(char[] buffer, int index, int count) { if (!this.inAttr && (this.inCDataSection || StartCDataSection())) this.wrapped.WriteCData(new string(buffer, index, count)); else this.wrapped.WriteChars(buffer, index, count); } public override void WriteEntityRef(string name) { EndCDataSection(); this.wrapped.WriteEntityRef(name); } public override void WriteCharEntity(char ch) { EndCDataSection(); this.wrapped.WriteCharEntity(ch); } public override void WriteSurrogateCharEntity(char lowChar, char highChar) { EndCDataSection(); this.wrapped.WriteSurrogateCharEntity(lowChar, highChar); } public override void WriteRaw(char[] buffer, int index, int count) { if (!this.inAttr && (this.inCDataSection || StartCDataSection())) this.wrapped.WriteCData(new string(buffer, index, count)); else this.wrapped.WriteRaw(buffer, index, count); } public override void WriteRaw(string data) { if (!this.inAttr && (this.inCDataSection || StartCDataSection())) this.wrapped.WriteCData(data); else this.wrapped.WriteRaw(data); } public override void Close() { this.wrapped.Close(); if (this.checkWellFormedDoc && !this.hasDocElem) { // Need at least one document element throw new XmlException(Res.Xml_NoRoot, string.Empty); } } public override void Flush() { this.wrapped.Flush(); } //----------------------------------------------- // Helper methods //----------------------------------------------- ////// Write CData text if element is a CData element. Return true if text should be written /// within a CData section. /// private bool StartCDataSection() { Debug.Assert(!this.inCDataSection); if (this.lookupCDataElems != null && this.bitsCData.PeekBit()) { this.inCDataSection = true; return true; } return false; } ////// No longer write CData text. /// private void EndCDataSection() { this.inCDataSection = false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.Globalization; using System.IO; using System.Collections.Generic; using System.Xml.Schema; using System.Diagnostics; ////// This writer wraps an XmlRawWriter and inserts additional lexical information into the resulting /// Xml 1.0 document: /// 1. CData sections /// 2. DocType declaration /// /// It also performs well-formed document checks if standalone="yes" and/or a doc-type-decl is output. /// internal class QueryOutputWriter : XmlRawWriter { private XmlRawWriter wrapped; private bool inCDataSection; private DictionarylookupCDataElems; private BitStack bitsCData; private XmlQualifiedName qnameCData; private bool outputDocType, checkWellFormedDoc, hasDocElem, inAttr; private string systemId, publicId; private int depth; public QueryOutputWriter(XmlRawWriter writer, XmlWriterSettings settings) { this.wrapped = writer; this.systemId = settings.DocTypeSystem; this.publicId = settings.DocTypePublic; if (settings.OutputMethod == XmlOutputMethod.Xml) { // Xml output method shouldn't output doc-type-decl if system ID is not defined (even if public ID is) // Only check for well-formed document if output method is xml if (this.systemId != null) { this.outputDocType = true; this.checkWellFormedDoc = true; } // Check for well-formed document if standalone="yes" in an auto-generated xml declaration if (settings.AutoXmlDeclaration && settings.Standalone == XmlStandalone.Yes) this.checkWellFormedDoc = true; if (settings.CDataSectionElements.Count > 0) { this.bitsCData = new BitStack(); this.lookupCDataElems = new Dictionary (); this.qnameCData = new XmlQualifiedName(); // Add each element name to the lookup table foreach (XmlQualifiedName name in settings.CDataSectionElements) { this.lookupCDataElems[name] = 0; } this.bitsCData.PushBit(false); } } else if (settings.OutputMethod == XmlOutputMethod.Html) { // Html output method should output doc-type-decl if system ID or public ID is defined if (this.systemId != null || this.publicId != null) this.outputDocType = true; } } //----------------------------------------------- // XmlWriter interface //----------------------------------------------- /// /// Get and set the namespace resolver that's used by this RawWriter to resolve prefixes. /// internal override IXmlNamespaceResolver NamespaceResolver { get { return this.resolver; } set { this.resolver = value; this.wrapped.NamespaceResolver = value; } } ////// Write the xml declaration. This must be the first call. /// internal override void WriteXmlDeclaration(XmlStandalone standalone) { this.wrapped.WriteXmlDeclaration(standalone); } internal override void WriteXmlDeclaration(string xmldecl) { this.wrapped.WriteXmlDeclaration(xmldecl); } ////// Return settings provided to factory. /// public override XmlWriterSettings Settings { get { XmlWriterSettings settings = this.wrapped.Settings; settings.ReadOnly = false; settings.DocTypeSystem = this.systemId; settings.DocTypePublic = this.publicId; settings.ReadOnly = true; return settings; } } ////// Suppress this explicit call to WriteDocType if information was provided by XmlWriterSettings. /// public override void WriteDocType(string name, string pubid, string sysid, string subset) { if (this.publicId == null && this.systemId == null) { Debug.Assert(!this.outputDocType); this.wrapped.WriteDocType(name, pubid, sysid, subset); } } ////// Check well-formedness, possibly output doc-type-decl, and determine whether this element is a /// CData section element. /// public override void WriteStartElement(string prefix, string localName, string ns) { EndCDataSection(); if (this.checkWellFormedDoc) { // Don't allow multiple document elements if (this.depth == 0 && this.hasDocElem) throw new XmlException(Res.Xml_NoMultipleRoots, string.Empty); this.depth++; this.hasDocElem = true; } // Output doc-type declaration immediately before first element is output if (this.outputDocType) { this.wrapped.WriteDocType( prefix.Length != 0 ? prefix + ":" + localName : localName, this.publicId, this.systemId, null); this.outputDocType = false; } this.wrapped.WriteStartElement(prefix, localName, ns); if (this.lookupCDataElems != null) { // Determine whether this element is a CData section element this.qnameCData.Init(localName, ns); this.bitsCData.PushBit(this.lookupCDataElems.ContainsKey(this.qnameCData)); } } internal override void WriteEndElement(string prefix, string localName, string ns) { EndCDataSection(); this.wrapped.WriteEndElement(prefix, localName, ns); if (this.checkWellFormedDoc) this.depth--; if (this.lookupCDataElems != null) this.bitsCData.PopBit(); } internal override void WriteFullEndElement(string prefix, string localName, string ns) { EndCDataSection(); this.wrapped.WriteFullEndElement(prefix, localName, ns); if (this.checkWellFormedDoc) this.depth--; if (this.lookupCDataElems != null) this.bitsCData.PopBit(); } internal override void StartElementContent() { this.wrapped.StartElementContent(); } public override void WriteStartAttribute(string prefix, string localName, string ns) { this.inAttr = true; this.wrapped.WriteStartAttribute(prefix, localName, ns); } public override void WriteEndAttribute() { this.inAttr = false; this.wrapped.WriteEndAttribute(); } internal override void WriteNamespaceDeclaration(string prefix, string ns) { this.wrapped.WriteNamespaceDeclaration(prefix, ns); } public override void WriteCData(string text) { this.wrapped.WriteCData(text); } public override void WriteComment(string text) { EndCDataSection(); this.wrapped.WriteComment(text); } public override void WriteProcessingInstruction(string name, string text) { EndCDataSection(); this.wrapped.WriteProcessingInstruction(name, text); } public override void WriteWhitespace(string ws) { if (!this.inAttr && (this.inCDataSection || StartCDataSection())) this.wrapped.WriteCData(ws); else this.wrapped.WriteWhitespace(ws); } public override void WriteString(string text) { if (!this.inAttr && (this.inCDataSection || StartCDataSection())) this.wrapped.WriteCData(text); else this.wrapped.WriteString(text); } public override void WriteChars(char[] buffer, int index, int count) { if (!this.inAttr && (this.inCDataSection || StartCDataSection())) this.wrapped.WriteCData(new string(buffer, index, count)); else this.wrapped.WriteChars(buffer, index, count); } public override void WriteEntityRef(string name) { EndCDataSection(); this.wrapped.WriteEntityRef(name); } public override void WriteCharEntity(char ch) { EndCDataSection(); this.wrapped.WriteCharEntity(ch); } public override void WriteSurrogateCharEntity(char lowChar, char highChar) { EndCDataSection(); this.wrapped.WriteSurrogateCharEntity(lowChar, highChar); } public override void WriteRaw(char[] buffer, int index, int count) { if (!this.inAttr && (this.inCDataSection || StartCDataSection())) this.wrapped.WriteCData(new string(buffer, index, count)); else this.wrapped.WriteRaw(buffer, index, count); } public override void WriteRaw(string data) { if (!this.inAttr && (this.inCDataSection || StartCDataSection())) this.wrapped.WriteCData(data); else this.wrapped.WriteRaw(data); } public override void Close() { this.wrapped.Close(); if (this.checkWellFormedDoc && !this.hasDocElem) { // Need at least one document element throw new XmlException(Res.Xml_NoRoot, string.Empty); } } public override void Flush() { this.wrapped.Flush(); } //----------------------------------------------- // Helper methods //----------------------------------------------- ////// Write CData text if element is a CData element. Return true if text should be written /// within a CData section. /// private bool StartCDataSection() { Debug.Assert(!this.inCDataSection); if (this.lookupCDataElems != null && this.bitsCData.PeekBit()) { this.inCDataSection = true; return true; } return false; } ////// No longer write CData text. /// private void EndCDataSection() { this.inCDataSection = false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
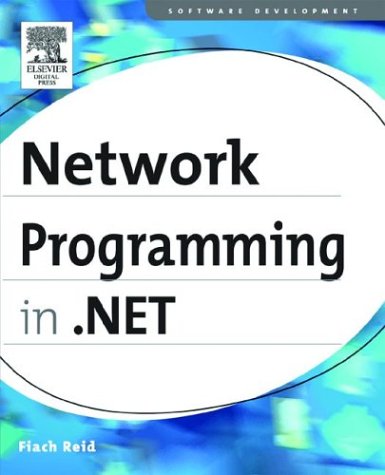
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IndexedSelectQueryOperator.cs
- DescendantQuery.cs
- Underline.cs
- HtmlInputCheckBox.cs
- ApplicationActivator.cs
- MatrixAnimationUsingKeyFrames.cs
- DataBoundControl.cs
- XPathNavigatorKeyComparer.cs
- SafeCancelMibChangeNotify.cs
- DataGridViewLinkColumn.cs
- DataSourceControlBuilder.cs
- ConfigurationConverterBase.cs
- SiteMembershipCondition.cs
- MetabaseReader.cs
- PathNode.cs
- Point3DAnimation.cs
- ScriptingAuthenticationServiceSection.cs
- NetMsmqSecurityElement.cs
- TabControl.cs
- Completion.cs
- BlurEffect.cs
- Shared.cs
- __FastResourceComparer.cs
- URL.cs
- RbTree.cs
- NameValueConfigurationElement.cs
- UnknownBitmapDecoder.cs
- CorrelationTokenInvalidatedHandler.cs
- ContractComponent.cs
- DispatchWrapper.cs
- WebPartsSection.cs
- TimeoutValidationAttribute.cs
- DEREncoding.cs
- XmlTextWriter.cs
- MultitargetingHelpers.cs
- OperatingSystem.cs
- TextElementEnumerator.cs
- RequestQueue.cs
- SmtpClient.cs
- TableRowsCollectionEditor.cs
- TagPrefixCollection.cs
- EntityClientCacheKey.cs
- CmsUtils.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- CollectionChangeEventArgs.cs
- UrlMapping.cs
- CustomWebEventKey.cs
- XhtmlBasicPanelAdapter.cs
- SoapException.cs
- RelationshipDetailsCollection.cs
- ListViewItem.cs
- TimelineGroup.cs
- StoryFragments.cs
- ToolStripRendererSwitcher.cs
- CompatibleComparer.cs
- ObjectSet.cs
- InternalPermissions.cs
- BitStack.cs
- TextRangeEditTables.cs
- PTConverter.cs
- EntityParameterCollection.cs
- TraceListener.cs
- MessageAction.cs
- DBParameter.cs
- EpmTargetPathSegment.cs
- QilXmlWriter.cs
- DesignObjectWrapper.cs
- InternalConfigConfigurationFactory.cs
- BufferCache.cs
- XmlSchemaComplexContentRestriction.cs
- UserControlDesigner.cs
- AuthenticationService.cs
- InvalidOperationException.cs
- DockPanel.cs
- connectionpool.cs
- PageThemeCodeDomTreeGenerator.cs
- StandardCommands.cs
- ToolStripDropDownDesigner.cs
- DataGridPreparingCellForEditEventArgs.cs
- MutableAssemblyCacheEntry.cs
- KnowledgeBase.cs
- Frame.cs
- GridViewDeletedEventArgs.cs
- StaticExtension.cs
- UnknownBitmapEncoder.cs
- FixedSOMSemanticBox.cs
- SystemIPv4InterfaceProperties.cs
- Delegate.cs
- GrabHandleGlyph.cs
- Crypto.cs
- DesignerSerializerAttribute.cs
- TransformCollection.cs
- ArgumentNullException.cs
- FontSourceCollection.cs
- PathGeometry.cs
- Transform.cs
- RoutedEvent.cs
- WindowsRegion.cs
- Exception.cs
- Misc.cs