Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / DataBinder.cs / 1 / DataBinder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Reflection; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DataBinder { private static readonly char[] expressionPartSeparator = new char[] { '.'}; private static readonly char[] indexExprStartChars = new char[] { '[', '('}; private static readonly char[] indexExprEndChars = new char[] { ']', ')'}; ///Provides design-time support for RAD designers to /// generate and parse ///. This class cannot be inherited. // public DataBinder() { } /// /// public static object Eval(object container, string expression) { if (expression == null) { throw new ArgumentNullException("expression"); } expression = expression.Trim(); if (expression.Length == 0) { throw new ArgumentNullException("expression"); } if (container == null) { return null; } string[] expressionParts = expression.Split(expressionPartSeparator); return DataBinder.Eval(container, expressionParts); } ///Evaluates data binding expressions at runtime. While /// this method is automatically called when you create data bindings in a RAD /// designer, you can also use it declaratively if you want to simplify the casting /// to a text string to be displayed on a browser. To do so, you must place the /// <%# and %> tags, which are also used in standard ASP.NET data binding, around the data binding expression. ///This method is particularly useful when data binding against controls that /// are in a templated list. ////// Since this method is called at runtime, it can cause performance /// to noticeably slow compared to standard ASP.NET databinding syntax. /// Use this method judiciously. /// ////// private static object Eval(object container, string[] expressionParts) { Debug.Assert((expressionParts != null) && (expressionParts.Length != 0), "invalid expressionParts parameter"); object prop; int i; for (prop = container, i = 0; (i < expressionParts.Length) && (prop != null); i++) { string expr = expressionParts[i]; bool indexedExpr = expr.IndexOfAny(indexExprStartChars) >= 0; if (indexedExpr == false) { prop = DataBinder.GetPropertyValue(prop, expr); } else { prop = DataBinder.GetIndexedPropertyValue(prop, expr); } } return prop; } ////// public static string Eval(object container, string expression, string format) { object value = DataBinder.Eval(container, expression); if ((value == null) || (value == System.DBNull.Value)) { return String.Empty; } else { if (String.IsNullOrEmpty(format)) { return value.ToString(); } else { return String.Format(format, value); } } } ///Evaluates data binding expressions at runtime and /// formats the output as text to be displayed in the requesting browser. While this /// method is automatically called when you create data bindings in a RAD designer, /// you can also use it declaratively if you want to simplify the casting to a text /// string to be displayed on a browser. To do so, you must place the <%# and %> tags, which are also used in standard ASP.NET data binding, around /// the data binding expression. ///This method is particularly useful when data binding against controls that /// are in a templated list. ////// Since this method is called at /// runtime, it can cause performance to noticeably slow compared to standard ASP.NET /// databinding syntax. Use this method judiciously, particularly when string /// formatting is not required. /// ////// public static object GetPropertyValue(object container, string propName) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(propName)) { throw new ArgumentNullException("propName"); } object prop = null; // get a PropertyDescriptor using case-insensitive lookup PropertyDescriptor pd = TypeDescriptor.GetProperties(container).Find(propName, true); if (pd != null) { prop = pd.GetValue(container); } else { throw new HttpException(SR.GetString(SR.DataBinder_Prop_Not_Found, container.GetType().FullName, propName)); } return prop; } ////// public static string GetPropertyValue(object container, string propName, string format) { object value = DataBinder.GetPropertyValue(container, propName); if ((value == null) || (value == System.DBNull.Value)) { return string.Empty; } else { if (String.IsNullOrEmpty(format)) { return value.ToString(); } else { return string.Format(format, value); } } } ////// public static object GetIndexedPropertyValue(object container, string expr) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(expr)) { throw new ArgumentNullException("expr"); } object prop = null; bool intIndex = false; int indexExprStart = expr.IndexOfAny(indexExprStartChars); int indexExprEnd = expr.IndexOfAny(indexExprEndChars, indexExprStart + 1); if ((indexExprStart < 0) || (indexExprEnd < 0) || (indexExprEnd == indexExprStart + 1)) { throw new ArgumentException(SR.GetString(SR.DataBinder_Invalid_Indexed_Expr, expr)); } string propName = null; object indexValue = null; string index = expr.Substring(indexExprStart + 1, indexExprEnd - indexExprStart - 1).Trim(); if (indexExprStart != 0) propName = expr.Substring(0, indexExprStart); if (index.Length != 0) { if (((index[0] == '"') && (index[index.Length - 1] == '"')) || ((index[0] == '\'') && (index[index.Length - 1] == '\''))) { indexValue = index.Substring(1, index.Length - 2); } else { if (Char.IsDigit(index[0])) { // treat it as a number int parsedIndex; intIndex = Int32.TryParse(index, NumberStyles.Integer, CultureInfo.InvariantCulture, out parsedIndex); if (intIndex) { indexValue = parsedIndex; } else { indexValue = index; } } else { // treat as a string indexValue = index; } } } if (indexValue == null) { throw new ArgumentException(SR.GetString(SR.DataBinder_Invalid_Indexed_Expr, expr)); } object collectionProp = null; if ((propName != null) && (propName.Length != 0)) { collectionProp = DataBinder.GetPropertyValue(container, propName); } else { collectionProp = container; } if (collectionProp != null) { Array arrayProp = collectionProp as Array; if (arrayProp != null && intIndex) { prop = arrayProp.GetValue((int)indexValue); } else if ((collectionProp is IList) && intIndex) { prop = ((IList)collectionProp)[(int)indexValue]; } else { PropertyInfo propInfo = collectionProp.GetType().GetProperty("Item", BindingFlags.Public | BindingFlags.Instance, null, null, new Type[] { indexValue.GetType() }, null); if (propInfo != null) { prop = propInfo.GetValue(collectionProp, new object[] { indexValue}); } else { throw new ArgumentException(SR.GetString(SR.DataBinder_No_Indexed_Accessor, collectionProp.GetType().FullName)); } } } return prop; } ////// public static string GetIndexedPropertyValue(object container, string propName, string format) { object value = DataBinder.GetIndexedPropertyValue(container, propName); if ((value == null) || (value == System.DBNull.Value)) { return String.Empty; } else { if (String.IsNullOrEmpty(format)) { return value.ToString(); } else { return string.Format(format, value); } } } ////// public static object GetDataItem(object container) { bool foundDataItem; return DataBinder.GetDataItem(container, out foundDataItem); } ////// public static object GetDataItem(object container, out bool foundDataItem) { // if (container == null) { foundDataItem = false; return null; } // If object implements IDataItemContainer, get value directly from interface IDataItemContainer dataItemContainer = container as IDataItemContainer; if (dataItemContainer != null) { foundDataItem = true; return dataItemContainer.DataItem; } // Otherwise, look for a property named "DataItem" string dataItemPropertyName = "DataItem"; // Check whether the property exists PropertyInfo propInfo = container.GetType().GetProperty(dataItemPropertyName, BindingFlags.Public | BindingFlags.Instance | BindingFlags.IgnoreCase); // If not, return null // if (propInfo == null) { foundDataItem = false; return null; } // If so, get the value foundDataItem = true; return propInfo.GetValue(container, null); } ////// Returns true if the value is null, DBNull, or INullableValue.HasValue is false. /// internal static bool IsNull(object value) { if (value == null || Convert.IsDBNull(value)) { return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Data; using System.Reflection; using System.Security.Permissions; using System.Web.Util; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class DataBinder { private static readonly char[] expressionPartSeparator = new char[] { '.'}; private static readonly char[] indexExprStartChars = new char[] { '[', '('}; private static readonly char[] indexExprEndChars = new char[] { ']', ')'}; ///Provides design-time support for RAD designers to /// generate and parse ///. This class cannot be inherited. // public DataBinder() { } /// /// public static object Eval(object container, string expression) { if (expression == null) { throw new ArgumentNullException("expression"); } expression = expression.Trim(); if (expression.Length == 0) { throw new ArgumentNullException("expression"); } if (container == null) { return null; } string[] expressionParts = expression.Split(expressionPartSeparator); return DataBinder.Eval(container, expressionParts); } ///Evaluates data binding expressions at runtime. While /// this method is automatically called when you create data bindings in a RAD /// designer, you can also use it declaratively if you want to simplify the casting /// to a text string to be displayed on a browser. To do so, you must place the /// <%# and %> tags, which are also used in standard ASP.NET data binding, around the data binding expression. ///This method is particularly useful when data binding against controls that /// are in a templated list. ////// Since this method is called at runtime, it can cause performance /// to noticeably slow compared to standard ASP.NET databinding syntax. /// Use this method judiciously. /// ////// private static object Eval(object container, string[] expressionParts) { Debug.Assert((expressionParts != null) && (expressionParts.Length != 0), "invalid expressionParts parameter"); object prop; int i; for (prop = container, i = 0; (i < expressionParts.Length) && (prop != null); i++) { string expr = expressionParts[i]; bool indexedExpr = expr.IndexOfAny(indexExprStartChars) >= 0; if (indexedExpr == false) { prop = DataBinder.GetPropertyValue(prop, expr); } else { prop = DataBinder.GetIndexedPropertyValue(prop, expr); } } return prop; } ////// public static string Eval(object container, string expression, string format) { object value = DataBinder.Eval(container, expression); if ((value == null) || (value == System.DBNull.Value)) { return String.Empty; } else { if (String.IsNullOrEmpty(format)) { return value.ToString(); } else { return String.Format(format, value); } } } ///Evaluates data binding expressions at runtime and /// formats the output as text to be displayed in the requesting browser. While this /// method is automatically called when you create data bindings in a RAD designer, /// you can also use it declaratively if you want to simplify the casting to a text /// string to be displayed on a browser. To do so, you must place the <%# and %> tags, which are also used in standard ASP.NET data binding, around /// the data binding expression. ///This method is particularly useful when data binding against controls that /// are in a templated list. ////// Since this method is called at /// runtime, it can cause performance to noticeably slow compared to standard ASP.NET /// databinding syntax. Use this method judiciously, particularly when string /// formatting is not required. /// ////// public static object GetPropertyValue(object container, string propName) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(propName)) { throw new ArgumentNullException("propName"); } object prop = null; // get a PropertyDescriptor using case-insensitive lookup PropertyDescriptor pd = TypeDescriptor.GetProperties(container).Find(propName, true); if (pd != null) { prop = pd.GetValue(container); } else { throw new HttpException(SR.GetString(SR.DataBinder_Prop_Not_Found, container.GetType().FullName, propName)); } return prop; } ////// public static string GetPropertyValue(object container, string propName, string format) { object value = DataBinder.GetPropertyValue(container, propName); if ((value == null) || (value == System.DBNull.Value)) { return string.Empty; } else { if (String.IsNullOrEmpty(format)) { return value.ToString(); } else { return string.Format(format, value); } } } ////// public static object GetIndexedPropertyValue(object container, string expr) { if (container == null) { throw new ArgumentNullException("container"); } if (String.IsNullOrEmpty(expr)) { throw new ArgumentNullException("expr"); } object prop = null; bool intIndex = false; int indexExprStart = expr.IndexOfAny(indexExprStartChars); int indexExprEnd = expr.IndexOfAny(indexExprEndChars, indexExprStart + 1); if ((indexExprStart < 0) || (indexExprEnd < 0) || (indexExprEnd == indexExprStart + 1)) { throw new ArgumentException(SR.GetString(SR.DataBinder_Invalid_Indexed_Expr, expr)); } string propName = null; object indexValue = null; string index = expr.Substring(indexExprStart + 1, indexExprEnd - indexExprStart - 1).Trim(); if (indexExprStart != 0) propName = expr.Substring(0, indexExprStart); if (index.Length != 0) { if (((index[0] == '"') && (index[index.Length - 1] == '"')) || ((index[0] == '\'') && (index[index.Length - 1] == '\''))) { indexValue = index.Substring(1, index.Length - 2); } else { if (Char.IsDigit(index[0])) { // treat it as a number int parsedIndex; intIndex = Int32.TryParse(index, NumberStyles.Integer, CultureInfo.InvariantCulture, out parsedIndex); if (intIndex) { indexValue = parsedIndex; } else { indexValue = index; } } else { // treat as a string indexValue = index; } } } if (indexValue == null) { throw new ArgumentException(SR.GetString(SR.DataBinder_Invalid_Indexed_Expr, expr)); } object collectionProp = null; if ((propName != null) && (propName.Length != 0)) { collectionProp = DataBinder.GetPropertyValue(container, propName); } else { collectionProp = container; } if (collectionProp != null) { Array arrayProp = collectionProp as Array; if (arrayProp != null && intIndex) { prop = arrayProp.GetValue((int)indexValue); } else if ((collectionProp is IList) && intIndex) { prop = ((IList)collectionProp)[(int)indexValue]; } else { PropertyInfo propInfo = collectionProp.GetType().GetProperty("Item", BindingFlags.Public | BindingFlags.Instance, null, null, new Type[] { indexValue.GetType() }, null); if (propInfo != null) { prop = propInfo.GetValue(collectionProp, new object[] { indexValue}); } else { throw new ArgumentException(SR.GetString(SR.DataBinder_No_Indexed_Accessor, collectionProp.GetType().FullName)); } } } return prop; } ////// public static string GetIndexedPropertyValue(object container, string propName, string format) { object value = DataBinder.GetIndexedPropertyValue(container, propName); if ((value == null) || (value == System.DBNull.Value)) { return String.Empty; } else { if (String.IsNullOrEmpty(format)) { return value.ToString(); } else { return string.Format(format, value); } } } ////// public static object GetDataItem(object container) { bool foundDataItem; return DataBinder.GetDataItem(container, out foundDataItem); } ////// public static object GetDataItem(object container, out bool foundDataItem) { // if (container == null) { foundDataItem = false; return null; } // If object implements IDataItemContainer, get value directly from interface IDataItemContainer dataItemContainer = container as IDataItemContainer; if (dataItemContainer != null) { foundDataItem = true; return dataItemContainer.DataItem; } // Otherwise, look for a property named "DataItem" string dataItemPropertyName = "DataItem"; // Check whether the property exists PropertyInfo propInfo = container.GetType().GetProperty(dataItemPropertyName, BindingFlags.Public | BindingFlags.Instance | BindingFlags.IgnoreCase); // If not, return null // if (propInfo == null) { foundDataItem = false; return null; } // If so, get the value foundDataItem = true; return propInfo.GetValue(container, null); } ////// Returns true if the value is null, DBNull, or INullableValue.HasValue is false. /// internal static bool IsNull(object value) { if (value == null || Convert.IsDBNull(value)) { return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
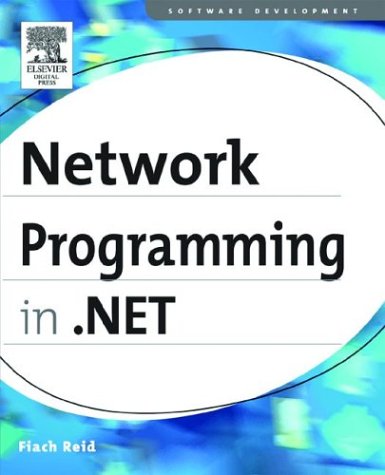
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StateDesigner.TransitionInfo.cs
- Helper.cs
- _UriSyntax.cs
- MaterialCollection.cs
- XmlSchemaSet.cs
- CAGDesigner.cs
- FileSystemWatcher.cs
- WindowsListViewGroup.cs
- SecUtil.cs
- Style.cs
- EntityDataSourceUtil.cs
- RemotingException.cs
- MimeMapping.cs
- ButtonBaseAutomationPeer.cs
- InputScope.cs
- CustomError.cs
- TextCollapsingProperties.cs
- Activity.cs
- ServiceHttpModule.cs
- ContentType.cs
- TextDecorationLocationValidation.cs
- ObjectDataSource.cs
- IgnoreFileBuildProvider.cs
- TargetInvocationException.cs
- CodeMethodReturnStatement.cs
- ProcessModelInfo.cs
- DataKeyCollection.cs
- unitconverter.cs
- FormatException.cs
- OperationAbortedException.cs
- InvokeWebServiceDesigner.cs
- printdlgexmarshaler.cs
- FormsAuthenticationUser.cs
- XmlQueryCardinality.cs
- PopOutPanel.cs
- StringInfo.cs
- MimeMapping.cs
- StickyNote.cs
- PropertyPath.cs
- SubMenuStyleCollection.cs
- WsdlImporterElement.cs
- ErrorInfoXmlDocument.cs
- MembershipPasswordException.cs
- ListChunk.cs
- MetadataItem_Static.cs
- CharAnimationBase.cs
- SafePEFileHandle.cs
- DnsEndPoint.cs
- Int32AnimationBase.cs
- CompilerError.cs
- InsufficientMemoryException.cs
- ToolboxCategoryItems.cs
- XhtmlBasicImageAdapter.cs
- SendMessageChannelCache.cs
- __Filters.cs
- CompositeScriptReferenceEventArgs.cs
- PenLineJoinValidation.cs
- serverconfig.cs
- XmlKeywords.cs
- TagNameToTypeMapper.cs
- ExtendLockAsyncResult.cs
- HttpPostLocalhostServerProtocol.cs
- X509Chain.cs
- Component.cs
- DelegatingTypeDescriptionProvider.cs
- CfgParser.cs
- IpcServerChannel.cs
- GridViewCellAutomationPeer.cs
- ItemAutomationPeer.cs
- ProcessThread.cs
- TextCharacters.cs
- GeneralTransform3DGroup.cs
- TaskFormBase.cs
- TCPListener.cs
- DoWorkEventArgs.cs
- ItemAutomationPeer.cs
- WebPartChrome.cs
- DataGridColumn.cs
- TaiwanCalendar.cs
- XmlConverter.cs
- MobileControl.cs
- OpenFileDialog.cs
- basemetadatamappingvisitor.cs
- ProcessModelSection.cs
- PerformanceCounterScope.cs
- ProfilePropertyNameValidator.cs
- ListChangedEventArgs.cs
- WorkflowMarkupSerializationManager.cs
- StaticResourceExtension.cs
- WindowsListView.cs
- TriggerAction.cs
- HtmlPhoneCallAdapter.cs
- InstanceDataCollection.cs
- EnterpriseServicesHelper.cs
- PermissionToken.cs
- StylusPointPropertyId.cs
- _RequestCacheProtocol.cs
- SuppressMergeCheckAttribute.cs
- EventArgs.cs
- sortedlist.cs