Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / GrammarBuilding / GrammarBuilderPhrase.cs / 1 / GrammarBuilderPhrase.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System.Diagnostics; using System.Speech.Internal.SrgsCompiler; using System.Speech.Internal.SrgsParser; using System.Speech.Recognition; namespace System.Speech.Internal.GrammarBuilding { #if VSCOMPILE [DebuggerDisplay ("{DebugSummary}")] #endif internal sealed class GrammarBuilderPhrase : GrammarBuilderBase { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// /// /// internal GrammarBuilderPhrase (string phrase) : this (phrase, false, SubsetMatchingMode.OrderedSubset) { } ////// /// /// /// internal GrammarBuilderPhrase (string phrase, SubsetMatchingMode subsetMatchingCriteria) : this (phrase, true, subsetMatchingCriteria) { } ////// /// /// /// /// private GrammarBuilderPhrase (string phrase, bool subsetMatching, SubsetMatchingMode subsetMatchingCriteria) { _phrase = string.Copy (phrase); _subsetMatching = subsetMatching; switch (subsetMatchingCriteria) { case SubsetMatchingMode.OrderedSubset: _matchMode = MatchMode.OrderedSubset; break; case SubsetMatchingMode.OrderedSubsetContentRequired: _matchMode = MatchMode.OrderedSubsetContentRequired; break; case SubsetMatchingMode.Subsequence: _matchMode = MatchMode.Subsequence; break; case SubsetMatchingMode.SubsequenceContentRequired: _matchMode = MatchMode.SubsequenceContentRequired; break; } } ////// /// /// /// /// private GrammarBuilderPhrase (string phrase, bool subsetMatching, MatchMode matchMode) { _phrase = string.Copy (phrase); _subsetMatching = subsetMatching; _matchMode = matchMode; } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region Public Methods /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.Equals"]/*' /> public override bool Equals (object obj) { GrammarBuilderPhrase refObj = obj as GrammarBuilderPhrase; if (refObj == null) { return false; } return _phrase == refObj._phrase && _matchMode == refObj._matchMode && _subsetMatching == refObj._subsetMatching; } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.GetHashCode"]/*' /> public override int GetHashCode () { return _phrase.GetHashCode (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************** #region Internal Methods ////// /// ///internal override GrammarBuilderBase Clone () { return new GrammarBuilderPhrase (_phrase, _subsetMatching, _matchMode); } /// /// /// /// /// /// /// ///internal override IElement CreateElement (IElementFactory elementFactory, IElement parent, IRule rule, IdentifierCollection ruleIds) { return CreatePhraseElement (elementFactory, parent); } #endregion //******************************************************************* // // Internal Properties // //******************************************************************** #region Internal Properties override internal string DebugSummary { get { return "‘" + _phrase + "’"; } } #endregion //******************************************************************* // // Private Methods // //******************************************************************* #region Private Methods /// /// /// /// /// ///private IElement CreatePhraseElement (IElementFactory elementFactory, IElement parent) { if (_subsetMatching) { // Create and return the ISubset representing the current phrase return elementFactory.CreateSubset (parent, _phrase, _matchMode); } else { if (elementFactory is SrgsElementCompilerFactory) { XmlParser.ParseText (parent, _phrase, null, null, -1f, new CreateTokenCallback (elementFactory.CreateToken)); } else { // Create and return the IElementText representing the current phrase return elementFactory.CreateText (parent, _phrase); } } return null; } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private readonly string _phrase; private readonly bool _subsetMatching; private readonly MatchMode _matchMode; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System.Diagnostics; using System.Speech.Internal.SrgsCompiler; using System.Speech.Internal.SrgsParser; using System.Speech.Recognition; namespace System.Speech.Internal.GrammarBuilding { #if VSCOMPILE [DebuggerDisplay ("{DebugSummary}")] #endif internal sealed class GrammarBuilderPhrase : GrammarBuilderBase { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// /// /// internal GrammarBuilderPhrase (string phrase) : this (phrase, false, SubsetMatchingMode.OrderedSubset) { } ////// /// /// /// internal GrammarBuilderPhrase (string phrase, SubsetMatchingMode subsetMatchingCriteria) : this (phrase, true, subsetMatchingCriteria) { } ////// /// /// /// /// private GrammarBuilderPhrase (string phrase, bool subsetMatching, SubsetMatchingMode subsetMatchingCriteria) { _phrase = string.Copy (phrase); _subsetMatching = subsetMatching; switch (subsetMatchingCriteria) { case SubsetMatchingMode.OrderedSubset: _matchMode = MatchMode.OrderedSubset; break; case SubsetMatchingMode.OrderedSubsetContentRequired: _matchMode = MatchMode.OrderedSubsetContentRequired; break; case SubsetMatchingMode.Subsequence: _matchMode = MatchMode.Subsequence; break; case SubsetMatchingMode.SubsequenceContentRequired: _matchMode = MatchMode.SubsequenceContentRequired; break; } } ////// /// /// /// /// private GrammarBuilderPhrase (string phrase, bool subsetMatching, MatchMode matchMode) { _phrase = string.Copy (phrase); _subsetMatching = subsetMatching; _matchMode = matchMode; } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region Public Methods /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.Equals"]/*' /> public override bool Equals (object obj) { GrammarBuilderPhrase refObj = obj as GrammarBuilderPhrase; if (refObj == null) { return false; } return _phrase == refObj._phrase && _matchMode == refObj._matchMode && _subsetMatching == refObj._subsetMatching; } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.GetHashCode"]/*' /> public override int GetHashCode () { return _phrase.GetHashCode (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************** #region Internal Methods ////// /// ///internal override GrammarBuilderBase Clone () { return new GrammarBuilderPhrase (_phrase, _subsetMatching, _matchMode); } /// /// /// /// /// /// /// ///internal override IElement CreateElement (IElementFactory elementFactory, IElement parent, IRule rule, IdentifierCollection ruleIds) { return CreatePhraseElement (elementFactory, parent); } #endregion //******************************************************************* // // Internal Properties // //******************************************************************** #region Internal Properties override internal string DebugSummary { get { return "‘" + _phrase + "’"; } } #endregion //******************************************************************* // // Private Methods // //******************************************************************* #region Private Methods /// /// /// /// /// ///private IElement CreatePhraseElement (IElementFactory elementFactory, IElement parent) { if (_subsetMatching) { // Create and return the ISubset representing the current phrase return elementFactory.CreateSubset (parent, _phrase, _matchMode); } else { if (elementFactory is SrgsElementCompilerFactory) { XmlParser.ParseText (parent, _phrase, null, null, -1f, new CreateTokenCallback (elementFactory.CreateToken)); } else { // Create and return the IElementText representing the current phrase return elementFactory.CreateText (parent, _phrase); } } return null; } #endregion //******************************************************************* // // Private Fields // //******************************************************************** #region Private Fields private readonly string _phrase; private readonly bool _subsetMatching; private readonly MatchMode _matchMode; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
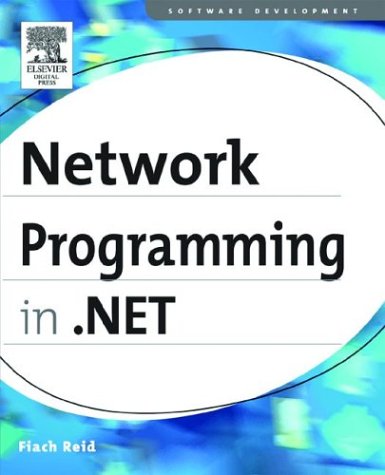
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PathFigureCollectionConverter.cs
- BitmapEffectGroup.cs
- XpsSerializationManager.cs
- ContentPlaceHolder.cs
- MaskedTextBox.cs
- SettingsPropertyValueCollection.cs
- VectorAnimationUsingKeyFrames.cs
- PageHandlerFactory.cs
- DesignerDataTable.cs
- DataGridViewUtilities.cs
- ConfigurationManager.cs
- DBNull.cs
- HostProtectionPermission.cs
- XmlAttribute.cs
- TableProviderWrapper.cs
- ValidationService.cs
- TabPanel.cs
- PersonalizationProviderHelper.cs
- ExtensionDataReader.cs
- DesignTimeTemplateParser.cs
- CodeVariableDeclarationStatement.cs
- wgx_commands.cs
- DbDataSourceEnumerator.cs
- XmlWriterTraceListener.cs
- TextRangeEditTables.cs
- UInt64.cs
- TraceUtility.cs
- PermissionToken.cs
- SimpleWorkerRequest.cs
- SpellerInterop.cs
- TextEditorTyping.cs
- MsmqIntegrationBinding.cs
- LabelLiteral.cs
- ListItemCollection.cs
- DebugManager.cs
- ConfigurationElement.cs
- XmlSchemaCompilationSettings.cs
- EditorZoneAutoFormat.cs
- ClientConvert.cs
- MethodExpr.cs
- GridViewDeletedEventArgs.cs
- TemplatePartAttribute.cs
- PageThemeBuildProvider.cs
- ResourceManager.cs
- Brush.cs
- DockPatternIdentifiers.cs
- WebPermission.cs
- DataControlFieldCollection.cs
- ContainerAction.cs
- ConnectorRouter.cs
- ActivityXamlServices.cs
- ToolStripKeyboardHandlingService.cs
- DataContractJsonSerializerOperationFormatter.cs
- KeyInterop.cs
- UserControlAutomationPeer.cs
- SoapReflectionImporter.cs
- GlyphingCache.cs
- ServerIdentity.cs
- DecoderReplacementFallback.cs
- FunctionQuery.cs
- ServiceDurableInstanceContextProvider.cs
- CollectionChangedEventManager.cs
- DesignBindingValueUIHandler.cs
- ListBoxItem.cs
- DockPatternIdentifiers.cs
- WorkflowInlining.cs
- URIFormatException.cs
- UnionCodeGroup.cs
- Normalization.cs
- FixedSOMFixedBlock.cs
- DateTimePicker.cs
- ComponentSerializationService.cs
- WorkflowExecutor.cs
- ItemsPresenter.cs
- RadioButtonPopupAdapter.cs
- BindingCompleteEventArgs.cs
- ImageMap.cs
- CodeDOMProvider.cs
- GestureRecognizer.cs
- OptimalBreakSession.cs
- HttpWrapper.cs
- TypeConverterAttribute.cs
- SecurityTokenParametersEnumerable.cs
- OutputCacheModule.cs
- BindingEntityInfo.cs
- UInt32Converter.cs
- XmlSerializerFaultFormatter.cs
- PathTooLongException.cs
- BulletedList.cs
- WasEndpointConfigContainer.cs
- IsolatedStorageSecurityState.cs
- TreeViewItem.cs
- QueryCacheManager.cs
- DesignerEventService.cs
- BasicExpandProvider.cs
- TreeViewImageIndexConverter.cs
- XmlSignatureManifest.cs
- ListViewInsertedEventArgs.cs
- PagesSection.cs
- DateTimePicker.cs