Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / Diagnostics / CorrelationManager.cs / 1 / CorrelationManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Specialized; using System.Threading; using System.Runtime.Remoting.Messaging; namespace System.Diagnostics { public class CorrelationManager { private const string transactionSlotName = "System.Diagnostics.Trace.CorrelationManagerSlot"; private const string activityIdSlotName = "E2ETrace.ActivityID"; internal CorrelationManager() { } public Guid ActivityId { get { Object id = CallContext.LogicalGetData(activityIdSlotName); if (id != null) return (Guid) id; else return Guid.Empty; } set { CallContext.LogicalSetData(activityIdSlotName, value); } } public Stack LogicalOperationStack { get { return GetLogicalOperationStack(); } } public void StartLogicalOperation(object operationId) { if (operationId == null) throw new ArgumentNullException("operationId"); Stack idStack = GetLogicalOperationStack(); idStack.Push(operationId); } public void StartLogicalOperation() { StartLogicalOperation(Guid.NewGuid()); } public void StopLogicalOperation() { Stack idStack = GetLogicalOperationStack(); idStack.Pop(); } private Stack GetLogicalOperationStack() { Stack idStack = CallContext.LogicalGetData(transactionSlotName) as Stack; if (idStack == null) { idStack = new Stack(); CallContext.LogicalSetData(transactionSlotName, idStack); } return idStack; } } }
Link Menu
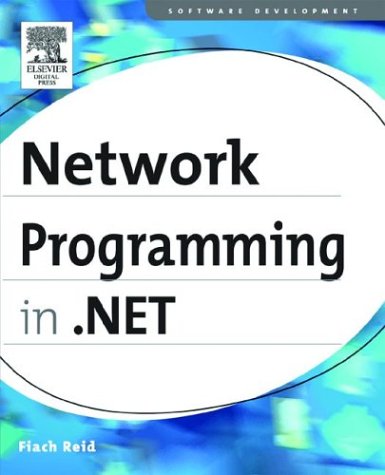
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Panel.cs
- MinMaxParagraphWidth.cs
- RoutingSection.cs
- COM2ColorConverter.cs
- RubberbandSelector.cs
- BitmapPalette.cs
- PersonalizableTypeEntry.cs
- TextDecorationCollection.cs
- XsdDateTime.cs
- TextFormatterHost.cs
- NullReferenceException.cs
- LocatorBase.cs
- ServiceModelDictionary.cs
- EdmProperty.cs
- Parser.cs
- PieceDirectory.cs
- WebContext.cs
- RelatedCurrencyManager.cs
- InternalUserCancelledException.cs
- InfoCardCryptoHelper.cs
- XmlHierarchyData.cs
- SvcMapFileSerializer.cs
- PersonalizableAttribute.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- RunClient.cs
- MultiView.cs
- CompilerCollection.cs
- DataGridViewImageColumn.cs
- PropertyGridEditorPart.cs
- DecimalAnimation.cs
- documentsequencetextpointer.cs
- PowerStatus.cs
- Substitution.cs
- NetNamedPipeSecurity.cs
- Container.cs
- ComponentCommands.cs
- TreeView.cs
- HttpListenerRequest.cs
- TypedRowHandler.cs
- TransformDescriptor.cs
- QuadraticBezierSegment.cs
- ConfigurationSectionCollection.cs
- RtfControlWordInfo.cs
- EncryptedXml.cs
- BitmapData.cs
- DataGridItemAutomationPeer.cs
- CodeEventReferenceExpression.cs
- VariantWrapper.cs
- BamlRecordHelper.cs
- RightsManagementInformation.cs
- CmsInterop.cs
- BufferedGraphicsContext.cs
- RegexFCD.cs
- GlobalizationAssembly.cs
- ServiceOperation.cs
- IMembershipProvider.cs
- SingleAnimationBase.cs
- EdmProviderManifest.cs
- OptimizedTemplateContent.cs
- XPathNodeInfoAtom.cs
- TreeViewAutomationPeer.cs
- SamlConstants.cs
- RecognizedAudio.cs
- NameValueCollection.cs
- GridView.cs
- UpDownEvent.cs
- ExclusiveHandle.cs
- InfoCardArgumentException.cs
- CodeDelegateCreateExpression.cs
- ReaderWriterLockWrapper.cs
- DataFormats.cs
- Calendar.cs
- RequestCacheManager.cs
- Signature.cs
- MetadataArtifactLoaderFile.cs
- HttpFileCollection.cs
- ConfigXmlCDataSection.cs
- Assembly.cs
- DisplayMemberTemplateSelector.cs
- DocumentReference.cs
- CheckBoxStandardAdapter.cs
- Type.cs
- ThrowHelper.cs
- CTreeGenerator.cs
- DataGridViewCellStyle.cs
- LocatorPartList.cs
- TextDecorationCollection.cs
- XamlSerializer.cs
- ListViewDataItem.cs
- DescriptionAttribute.cs
- WebConfigurationHostFileChange.cs
- UidPropertyAttribute.cs
- UrlAuthFailedErrorFormatter.cs
- WindowsTooltip.cs
- XmlSchemaImporter.cs
- EmptyStringExpandableObjectConverter.cs
- RMEnrollmentPage1.cs
- ToolStripHighContrastRenderer.cs
- MenuCommand.cs
- ConfigurationException.cs