Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / MaskDescriptor.cs / 1 / MaskDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System; using System.ComponentModel; using System.Design; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Threading; ////// MaskDescriptor abstract class defines the set of methods mask descriptors need to implement for the /// MaskedTextBox.Mask UITypeEditor to include as options in the property editor. MaskDescriptor /// types are discovered at designed time by querying the ITypeDiscoveryService service provider from /// the UITypeEditor object. /// public abstract class MaskDescriptor { ////// The mask being described. /// public abstract string Mask { get; } ////// The friendly name of the mask descriptor. /// Used also as the description for the mask. /// public abstract string Name { get; } ////// A sample text following the mask specification. /// public abstract string Sample { get; } ////// A Type representing the type providing validation for this mask. /// public abstract Type ValidatingType { get; } ////// The CultureInfo representing the locale the mask is designed for. /// public virtual CultureInfo Culture { get{ return Thread.CurrentThread.CurrentCulture; } } ////// Determines whether the specified mask descriptor is valid and hence can be added to the canned masks list. /// A valid MaskDescriptor must meet the following conditions: /// 1. Not null. /// 2. Not null or empty mask. /// 3. Not null or empty name. /// 4. Not null or empty sample. /// 5. The sample is correct based on the mask and all required edit characters have been provided (mask completed - not necessarily full). /// 6. The sample is valid based on the ValidatingType object (if any). /// public static bool IsValidMaskDescriptor( MaskDescriptor maskDescriptor ) { string dummy; return IsValidMaskDescriptor(maskDescriptor, out dummy); } public static bool IsValidMaskDescriptor( MaskDescriptor maskDescriptor, out string validationErrorDescription) { validationErrorDescription = string.Empty; if ( maskDescriptor == null ) { validationErrorDescription = SR.GetString(SR.MaskDescriptorNull); return false; } if ( string.IsNullOrEmpty(maskDescriptor.Mask) || string.IsNullOrEmpty(maskDescriptor.Name) || string.IsNullOrEmpty(maskDescriptor.Sample) ) { validationErrorDescription = SR.GetString(SR.MaskDescriptorNullOrEmptyRequiredProperty); return false; } MaskedTextProvider mtp = new MaskedTextProvider( maskDescriptor.Mask, maskDescriptor.Culture ); MaskedTextBox mtb = new MaskedTextBox(mtp); mtb.SkipLiterals = true; mtb.ResetOnPrompt = true; mtb.ResetOnSpace = true; mtb.ValidatingType = maskDescriptor.ValidatingType; mtb.FormatProvider = maskDescriptor.Culture; mtb.Culture = maskDescriptor.Culture; mtb.TypeValidationCompleted += new System.Windows.Forms.TypeValidationEventHandler(maskedTextBox1_TypeValidationCompleted); mtb.MaskInputRejected += new System.Windows.Forms.MaskInputRejectedEventHandler(maskedTextBox1_MaskInputRejected); // Add sample. If it fails we are done. mtb.Text = maskDescriptor.Sample; if (mtb.Tag == null) // Sample was added successfully (MaskInputRejected event handler did not change the mtb tag). { if( maskDescriptor.ValidatingType != null ) { mtb.ValidateText(); } // } if (mtb.Tag != null) // Validation failed. { validationErrorDescription = mtb.Tag.ToString(); } return validationErrorDescription.Length == 0; } private static void maskedTextBox1_MaskInputRejected(object sender, MaskInputRejectedEventArgs e) { MaskedTextBox mtb = sender as MaskedTextBox; mtb.Tag = MaskedTextBoxDesigner.GetMaskInputRejectedErrorMessage(e); } private static void maskedTextBox1_TypeValidationCompleted(object sender, TypeValidationEventArgs e) { if (!e.IsValidInput) { MaskedTextBox mtb = sender as MaskedTextBox; mtb.Tag = e.Message; } } ////// Determines whether this mask descriptor and the passed object describe the same mask. /// True if the following conditions are met in both, this and the passed object: /// 1. Mask property is the same. /// 2. Validating type is the same /// Observe that the Name property is not considered since MaskedTextProvider/Box are not /// aware of it. /// public override bool Equals( object maskDescriptor ) { MaskDescriptor descriptor = maskDescriptor as MaskDescriptor; if( !IsValidMaskDescriptor(descriptor) || !IsValidMaskDescriptor(this) ) { return this == maskDescriptor; // shallow comparison. } return ((this.Mask == descriptor.Mask) && (this.ValidatingType == descriptor.ValidatingType)); } ////// override. /// public override int GetHashCode() { string hash = this.Mask; if (this.ValidatingType != null ) { hash += this.ValidatingType.ToString(); } return hash.GetHashCode(); } ////// ToString override. /// public override string ToString() { return string.Format(CultureInfo.CurrentCulture, "{0}/// Implements the manual sorting of items by columns in the mask descriptor table. /// Used by the MaskDesignerDialog to sort the items in the mask descriptors list. /// internal class MaskDescriptorComparer : System.Collections.Generic.IComparer { private SortOrder sortOrder; private SortType sortType; public enum SortType { ByName, BySample, ByValidatingTypeName } public MaskDescriptorComparer(SortType sortType, SortOrder sortOrder) { this.sortType = sortType; this.sortOrder = sortOrder; } public int Compare(MaskDescriptor maskDescriptorA, MaskDescriptor maskDescriptorB) { if( maskDescriptorA == null || maskDescriptorB == null ) { // Since this is an internal class we cannot throw here, the user cannot do anything about this. Debug.Fail( "One or more parameters invalid" ); return 0; } string textA, textB; switch( sortType ) { default: Debug.Fail( "Invalid SortType, defaulting to SortType.ByName" ); goto case SortType.ByName; case SortType.ByName: textA = maskDescriptorA.Name; textB = maskDescriptorB.Name; break; case SortType.BySample: textA = maskDescriptorA.Sample; textB = maskDescriptorB.Sample; break; case SortType.ByValidatingTypeName: textA = maskDescriptorA.ValidatingType == null ? SR.GetString( SR.MaskDescriptorValidatingTypeNone ) : maskDescriptorA.ValidatingType.Name; textB = maskDescriptorB.ValidatingType == null ? SR.GetString( SR.MaskDescriptorValidatingTypeNone ) : maskDescriptorB.ValidatingType.Name; break; } int retVal = String.Compare(textA, textB); return sortOrder == SortOrder.Descending ? -retVal : retVal; } [SuppressMessage("Microsoft.Performance", "CA1801:AvoidUnusedParameters")] [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] public int GetHashCode(MaskDescriptor maskDescriptor) { if( maskDescriptor != null ) { return maskDescriptor.GetHashCode(); } Debug.Fail("Null maskDescriptor passed."); return 0; } [SuppressMessage("Microsoft.Performance", "CA1801:AvoidUnusedParameters")] [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] public bool Equals( MaskDescriptor maskDescriptorA, MaskDescriptor maskDescriptorB ) { if( !MaskDescriptor.IsValidMaskDescriptor(maskDescriptorA) || !MaskDescriptor.IsValidMaskDescriptor(maskDescriptorB) ) { return maskDescriptorA == maskDescriptorB; // shallow comparison. } return maskDescriptorA.Equals(maskDescriptorB); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
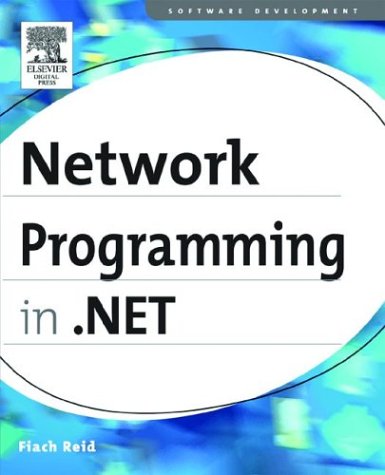
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConditionalExpression.cs
- EntityParameterCollection.cs
- RemoteCryptoSignHashRequest.cs
- Funcletizer.cs
- PathTooLongException.cs
- PersonalizationProviderHelper.cs
- HwndTarget.cs
- odbcmetadatacolumnnames.cs
- XmlDictionaryString.cs
- MdImport.cs
- EntityType.cs
- ListControlConvertEventArgs.cs
- MimeParameterWriter.cs
- FragmentQueryKB.cs
- AuthenticationService.cs
- ExternalCalls.cs
- ActivityWithResultWrapper.cs
- ImageListStreamer.cs
- ControlParameter.cs
- webbrowsersite.cs
- ThreadInterruptedException.cs
- RequestUriProcessor.cs
- EncoderExceptionFallback.cs
- BehaviorService.cs
- ADRole.cs
- COM2ComponentEditor.cs
- DataTableExtensions.cs
- Int32.cs
- TreeViewItem.cs
- DbConnectionInternal.cs
- MailHeaderInfo.cs
- DataGridViewTopRowAccessibleObject.cs
- DrawingCollection.cs
- TypeEnumerableViewSchema.cs
- _LazyAsyncResult.cs
- XPathScanner.cs
- OdbcHandle.cs
- StringReader.cs
- PropertyMappingExceptionEventArgs.cs
- TokenBasedSet.cs
- UnmanagedMarshal.cs
- GroupBox.cs
- ArgIterator.cs
- LifetimeServices.cs
- DomNameTable.cs
- StorageBasedPackageProperties.cs
- Serializer.cs
- Rect.cs
- Compiler.cs
- DynamicObjectAccessor.cs
- DoubleLinkList.cs
- SimpleType.cs
- HighlightVisual.cs
- EntityDataSourceSelectingEventArgs.cs
- RbTree.cs
- CreateUserErrorEventArgs.cs
- MailAddressCollection.cs
- connectionpool.cs
- CheckBoxList.cs
- EntityException.cs
- InternalsVisibleToAttribute.cs
- MeshGeometry3D.cs
- TimeStampChecker.cs
- AttributedMetaModel.cs
- BrowserCapabilitiesFactory35.cs
- XpsPackagingPolicy.cs
- BooleanSwitch.cs
- CreateUserWizard.cs
- TextTreeUndo.cs
- OdbcStatementHandle.cs
- SoapTypeAttribute.cs
- BindingCompleteEventArgs.cs
- AnimatedTypeHelpers.cs
- CodePrimitiveExpression.cs
- FontUnitConverter.cs
- LogAppendAsyncResult.cs
- CacheVirtualItemsEvent.cs
- SqlCacheDependency.cs
- StrongNameKeyPair.cs
- DataKeyArray.cs
- FlagsAttribute.cs
- NotImplementedException.cs
- CapiSafeHandles.cs
- PackageFilter.cs
- GridViewDeletedEventArgs.cs
- NullableDecimalMinMaxAggregationOperator.cs
- UIInitializationException.cs
- ReadOnlyObservableCollection.cs
- Mapping.cs
- ScalarOps.cs
- RepeaterCommandEventArgs.cs
- ComponentResourceKeyConverter.cs
- GridViewColumnCollectionChangedEventArgs.cs
- WindowShowOrOpenTracker.cs
- SelectionItemProviderWrapper.cs
- ConnectionPointCookie.cs
- ProfileModule.cs
- EventMappingSettingsCollection.cs
- _CookieModule.cs
- CombinedGeometry.cs