Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / ControlValuePropertyAttribute.cs / 2 / ControlValuePropertyAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.ComponentModel; using System.Diagnostics; using System.Security.Permissions; using System.Web.Util; ////// Specifies the default value property for a control. /// [AttributeUsage(AttributeTargets.Class)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class ControlValuePropertyAttribute : Attribute { private readonly string _name; private readonly object _defaultValue; ////// Initializes a new instance of the public ControlValuePropertyAttribute(string name) { _name = name; } ///class. /// /// Initializes a new instance of the class, using the specified value as the default value. /// public ControlValuePropertyAttribute(string name, object defaultValue) { _name = name; _defaultValue = defaultValue; } ////// Initializes a new instance of the class, converting the specified value to the /// specified type. /// public ControlValuePropertyAttribute(string name, Type type, string defaultValue) { _name = name; // The try/catch here is because attributes should never throw exceptions. We would fail to // load an otherwise normal class. try { _defaultValue = TypeDescriptor.GetConverter(type).ConvertFromInvariantString(defaultValue); } catch { System.Diagnostics.Debug.Fail("ControlValuePropertyAttribute: Default value of type " + type.FullName + " threw converting from the string '" + defaultValue + "'."); } } ////// Gets the name of the default value property for the control this attribute is bound to. /// public string Name { get { return _name; } } ////// Gets the value of the default value property for the control this attribute is bound to. /// public object DefaultValue { get { return _defaultValue; } } public override bool Equals(object obj) { ControlValuePropertyAttribute other = obj as ControlValuePropertyAttribute; if (other != null) { if (String.Equals(_name, other.Name, StringComparison.Ordinal)) { if (_defaultValue != null) { return _defaultValue.Equals(other.DefaultValue); } else { return (other.DefaultValue == null); } } } return false; } public override int GetHashCode() { return System.Web.Util.HashCodeCombiner.CombineHashCodes( ((Name != null) ? Name.GetHashCode() : 0), ((DefaultValue != null) ? DefaultValue.GetHashCode() : 0)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.ComponentModel; using System.Diagnostics; using System.Security.Permissions; using System.Web.Util; ////// Specifies the default value property for a control. /// [AttributeUsage(AttributeTargets.Class)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class ControlValuePropertyAttribute : Attribute { private readonly string _name; private readonly object _defaultValue; ////// Initializes a new instance of the public ControlValuePropertyAttribute(string name) { _name = name; } ///class. /// /// Initializes a new instance of the class, using the specified value as the default value. /// public ControlValuePropertyAttribute(string name, object defaultValue) { _name = name; _defaultValue = defaultValue; } ////// Initializes a new instance of the class, converting the specified value to the /// specified type. /// public ControlValuePropertyAttribute(string name, Type type, string defaultValue) { _name = name; // The try/catch here is because attributes should never throw exceptions. We would fail to // load an otherwise normal class. try { _defaultValue = TypeDescriptor.GetConverter(type).ConvertFromInvariantString(defaultValue); } catch { System.Diagnostics.Debug.Fail("ControlValuePropertyAttribute: Default value of type " + type.FullName + " threw converting from the string '" + defaultValue + "'."); } } ////// Gets the name of the default value property for the control this attribute is bound to. /// public string Name { get { return _name; } } ////// Gets the value of the default value property for the control this attribute is bound to. /// public object DefaultValue { get { return _defaultValue; } } public override bool Equals(object obj) { ControlValuePropertyAttribute other = obj as ControlValuePropertyAttribute; if (other != null) { if (String.Equals(_name, other.Name, StringComparison.Ordinal)) { if (_defaultValue != null) { return _defaultValue.Equals(other.DefaultValue); } else { return (other.DefaultValue == null); } } } return false; } public override int GetHashCode() { return System.Web.Util.HashCodeCombiner.CombineHashCodes( ((Name != null) ? Name.GetHashCode() : 0), ((DefaultValue != null) ? DefaultValue.GetHashCode() : 0)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
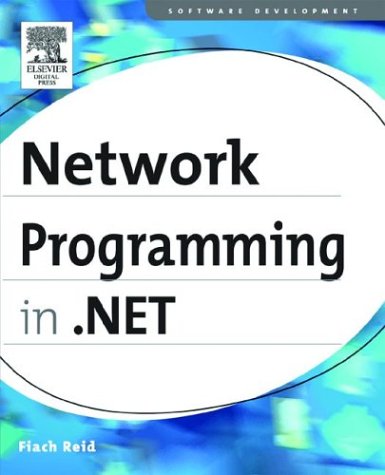
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MdImport.cs
- WindowsToolbar.cs
- XslException.cs
- HttpFileCollection.cs
- GPPOINT.cs
- Roles.cs
- LayoutExceptionEventArgs.cs
- ModuleBuilder.cs
- CombinedGeometry.cs
- InstanceKeyNotReadyException.cs
- DetailsViewInsertedEventArgs.cs
- OleDbParameterCollection.cs
- EventHandlersStore.cs
- TransformGroup.cs
- ElementProxy.cs
- GetPageNumberCompletedEventArgs.cs
- LineProperties.cs
- HyperLinkField.cs
- GetPageNumberCompletedEventArgs.cs
- DataRowChangeEvent.cs
- DragCompletedEventArgs.cs
- TypedDataSourceCodeGenerator.cs
- PersianCalendar.cs
- TableCellAutomationPeer.cs
- Properties.cs
- KeyEventArgs.cs
- AQNBuilder.cs
- ContentValidator.cs
- MachineSettingsSection.cs
- ClipboardData.cs
- ProxyDataContractResolver.cs
- MetadataArtifactLoaderComposite.cs
- ColorEditor.cs
- ListenerElementsCollection.cs
- SamlSubjectStatement.cs
- cryptoapiTransform.cs
- FormsAuthenticationUserCollection.cs
- ImageMetadata.cs
- DrawItemEvent.cs
- FormCollection.cs
- EllipseGeometry.cs
- SeekStoryboard.cs
- DocumentReferenceCollection.cs
- TakeQueryOptionExpression.cs
- XmlSchemaSequence.cs
- SoapTypeAttribute.cs
- XPathScanner.cs
- Root.cs
- SqlDataSourceSelectingEventArgs.cs
- TouchesOverProperty.cs
- SeverityFilter.cs
- SecurityAlgorithmSuite.cs
- ChannelPoolSettings.cs
- AlphaSortedEnumConverter.cs
- Function.cs
- BitSet.cs
- HttpListenerPrefixCollection.cs
- CustomAttributeSerializer.cs
- ReferencedAssembly.cs
- ClientScriptItem.cs
- FormsAuthenticationUser.cs
- MethodBuilder.cs
- BitmapEffectInputConnector.cs
- HtmlContainerControl.cs
- COM2IPerPropertyBrowsingHandler.cs
- LocatorPart.cs
- FieldNameLookup.cs
- DecoderNLS.cs
- ResetableIterator.cs
- Transform.cs
- ProviderConnectionPoint.cs
- DateTimeOffsetAdapter.cs
- XmlNode.cs
- TransactionScope.cs
- CodeDomLoader.cs
- InputMethod.cs
- SystemGatewayIPAddressInformation.cs
- SessionStateItemCollection.cs
- CryptoConfig.cs
- coordinatorfactory.cs
- DataPagerFieldCollection.cs
- EventToken.cs
- AnimationLayer.cs
- ClientTargetCollection.cs
- CngUIPolicy.cs
- ToolboxBitmapAttribute.cs
- PageOutputColor.cs
- GacUtil.cs
- TargetControlTypeCache.cs
- FirstMatchCodeGroup.cs
- Stroke.cs
- RoutedEvent.cs
- WebPartDescription.cs
- EndpointBehaviorElement.cs
- PreservationFileReader.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- AmbientLight.cs
- WindowInteractionStateTracker.cs
- ObjectDisposedException.cs
- KeyboardNavigation.cs