Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CqlGeneration / CqlBlock.cs / 2 / CqlBlock.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.Structures; using System.Collections.ObjectModel; using System.Text; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class that holds an expression of the form SELECT .. FROM .. WHERE // Essentially, it is a structure allows us to generate Cql query in a // localized manner, i.e., all global decisions about nulls, constants, // case statements, etc have already been made internal abstract class CqlBlock : InternalBase { // effects: Initializes a CqlBlock with the SELECT (slotinfos), FROM // (children), WHERE (whereClause), AS (blockAliasNum) protected CqlBlock(SlotInfo[] slotInfos, Listchildren, BoolExpression whereClause, CqlIdentifiers identifiers, int blockAliasNum) { m_slots = new ReadOnlyCollection (slotInfos); m_children = new ReadOnlyCollection (children); m_whereClause = whereClause; m_blockAlias = identifiers.GetBlockAlias(blockAliasNum); } #region Fields private ReadOnlyCollection m_slots; // essentially, SELECT private ReadOnlyCollection m_children; // FROM private BoolExpression m_whereClause; // WHERE private string m_blockAlias; // AS ... for cql generation #endregion #region Properties // effects: Returns the slot being projected at slotNum. If no slot // is being projected, returns null internal ProjectedSlot ProjectedSlot(int slotNum) { Debug.Assert(slotNum < m_slots.Count, "Slotnum too high"); return m_slots[slotNum].SlotValue; } // effects: Returns the whereclause of this block (WHERE) protected BoolExpression WhereClause { get { return m_whereClause; } } // effects: Returns all the child blocks of this block (FROM) protected ReadOnlyCollection Children { get { return m_children; } } // effects: Returns all the slots for this block (SELECT) internal ReadOnlyCollection Slots { get { return m_slots; } set { m_slots = value; } } #endregion #region Abstract Methods // effects: Returns a string corresponding to the Cql representation // of this block (and its children below). internal abstract StringBuilder AsCql(StringBuilder builder, bool isTopLevel, int indentLevel); #endregion #region Methods // effects: Returns a string that has an alias for the FROM part of // this block or the whole block itself that can be used for "AS" internal string CqlAlias { get { return m_blockAlias;} } // effects: Returns true iff slotNum is being projected by this block internal bool IsProjected(int slotNum) { Debug.Assert(slotNum < m_slots.Count, "Slotnum too high"); return m_slots[slotNum].IsProjected; } // effects: Generates a "SELECT A, B, C, .." for all the slots in this // if asForCaseStatementsOnly is true, generates aliases, ie., foo AS // bar for CaseStatements only. Otherwise, generates them for all slots protected void GenerateProjectedtList(StringBuilder builder, int indentLevel, string blockAlias, bool asForCaseStatementsOnly) { StringUtil.IndentNewLine(builder, indentLevel); builder.Append("SELECT "); List projectedExpressions = new List (); bool isFirst = true; foreach (SlotInfo slotInfo in Slots) { if (false == slotInfo.IsRequiredByParent) { // Ignore slots that are not needed continue; } if (isFirst == false) { builder.Append(", "); } // Note: We call AsCql and not AliasName on // slotInfo since we do want to pick the right reference to // the slot from the appropriate child if (false == asForCaseStatementsOnly) { StringUtil.IndentNewLine(builder, indentLevel + 1); } slotInfo.AsCql(builder, blockAlias, indentLevel); // Print the field alias for constants (booleans and regular constants). Also // case statements need the alias as well. // We also add it for extent cql blocks for all fields and // finally we need IS NOT NULL for boolean conditions if (slotInfo.SlotValue is CaseStatementSlot || slotInfo.SlotValue is BooleanProjectedSlot || slotInfo.SlotValue is ConstantSlot || asForCaseStatementsOnly == false || slotInfo.IsEnforcedNotNull) { builder.Append(" AS ") .Append(slotInfo.CqlFieldAlias); } isFirst = false; } // Get the slots as A, B, C, ... StringUtil.ToSeparatedString(builder, projectedExpressions, ", ", null); StringUtil.IndentNewLine(builder, indentLevel); } internal override void ToCompactString(StringBuilder builder) { for (int i = 0; i < m_slots.Count; i++) { StringUtil.FormatStringBuilder(builder, "{0}: ", i); m_slots[i].ToCompactString(builder); builder.Append(' '); } m_whereClause.ToCompactString(builder); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.Structures; using System.Collections.ObjectModel; using System.Text; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class that holds an expression of the form SELECT .. FROM .. WHERE // Essentially, it is a structure allows us to generate Cql query in a // localized manner, i.e., all global decisions about nulls, constants, // case statements, etc have already been made internal abstract class CqlBlock : InternalBase { // effects: Initializes a CqlBlock with the SELECT (slotinfos), FROM // (children), WHERE (whereClause), AS (blockAliasNum) protected CqlBlock(SlotInfo[] slotInfos, Listchildren, BoolExpression whereClause, CqlIdentifiers identifiers, int blockAliasNum) { m_slots = new ReadOnlyCollection (slotInfos); m_children = new ReadOnlyCollection (children); m_whereClause = whereClause; m_blockAlias = identifiers.GetBlockAlias(blockAliasNum); } #region Fields private ReadOnlyCollection m_slots; // essentially, SELECT private ReadOnlyCollection m_children; // FROM private BoolExpression m_whereClause; // WHERE private string m_blockAlias; // AS ... for cql generation #endregion #region Properties // effects: Returns the slot being projected at slotNum. If no slot // is being projected, returns null internal ProjectedSlot ProjectedSlot(int slotNum) { Debug.Assert(slotNum < m_slots.Count, "Slotnum too high"); return m_slots[slotNum].SlotValue; } // effects: Returns the whereclause of this block (WHERE) protected BoolExpression WhereClause { get { return m_whereClause; } } // effects: Returns all the child blocks of this block (FROM) protected ReadOnlyCollection Children { get { return m_children; } } // effects: Returns all the slots for this block (SELECT) internal ReadOnlyCollection Slots { get { return m_slots; } set { m_slots = value; } } #endregion #region Abstract Methods // effects: Returns a string corresponding to the Cql representation // of this block (and its children below). internal abstract StringBuilder AsCql(StringBuilder builder, bool isTopLevel, int indentLevel); #endregion #region Methods // effects: Returns a string that has an alias for the FROM part of // this block or the whole block itself that can be used for "AS" internal string CqlAlias { get { return m_blockAlias;} } // effects: Returns true iff slotNum is being projected by this block internal bool IsProjected(int slotNum) { Debug.Assert(slotNum < m_slots.Count, "Slotnum too high"); return m_slots[slotNum].IsProjected; } // effects: Generates a "SELECT A, B, C, .." for all the slots in this // if asForCaseStatementsOnly is true, generates aliases, ie., foo AS // bar for CaseStatements only. Otherwise, generates them for all slots protected void GenerateProjectedtList(StringBuilder builder, int indentLevel, string blockAlias, bool asForCaseStatementsOnly) { StringUtil.IndentNewLine(builder, indentLevel); builder.Append("SELECT "); List projectedExpressions = new List (); bool isFirst = true; foreach (SlotInfo slotInfo in Slots) { if (false == slotInfo.IsRequiredByParent) { // Ignore slots that are not needed continue; } if (isFirst == false) { builder.Append(", "); } // Note: We call AsCql and not AliasName on // slotInfo since we do want to pick the right reference to // the slot from the appropriate child if (false == asForCaseStatementsOnly) { StringUtil.IndentNewLine(builder, indentLevel + 1); } slotInfo.AsCql(builder, blockAlias, indentLevel); // Print the field alias for constants (booleans and regular constants). Also // case statements need the alias as well. // We also add it for extent cql blocks for all fields and // finally we need IS NOT NULL for boolean conditions if (slotInfo.SlotValue is CaseStatementSlot || slotInfo.SlotValue is BooleanProjectedSlot || slotInfo.SlotValue is ConstantSlot || asForCaseStatementsOnly == false || slotInfo.IsEnforcedNotNull) { builder.Append(" AS ") .Append(slotInfo.CqlFieldAlias); } isFirst = false; } // Get the slots as A, B, C, ... StringUtil.ToSeparatedString(builder, projectedExpressions, ", ", null); StringUtil.IndentNewLine(builder, indentLevel); } internal override void ToCompactString(StringBuilder builder) { for (int i = 0; i < m_slots.Count; i++) { StringUtil.FormatStringBuilder(builder, "{0}: ", i); m_slots[i].ToCompactString(builder); builder.Append(' '); } m_whereClause.ToCompactString(builder); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
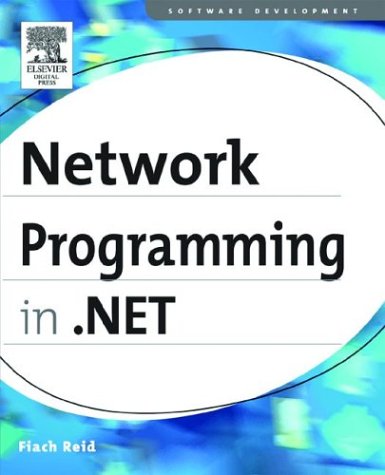
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProviderCollection.cs
- VerticalAlignConverter.cs
- ObjectCloneHelper.cs
- AnnotationObservableCollection.cs
- SymbolMethod.cs
- DataTablePropertyDescriptor.cs
- DictionarySectionHandler.cs
- MILUtilities.cs
- RMPublishingDialog.cs
- PersonalizationStateInfoCollection.cs
- unsafenativemethodsother.cs
- StreamReader.cs
- WebPartConnectVerb.cs
- EntityDataSourceChangingEventArgs.cs
- TextEditorCopyPaste.cs
- ModelProperty.cs
- ToolStripScrollButton.cs
- PathGeometry.cs
- PropertyManager.cs
- AccessDataSource.cs
- ImportContext.cs
- OptimizerPatterns.cs
- KeyedCollection.cs
- NativeCppClassAttribute.cs
- TextTreeNode.cs
- OutputCacheProfileCollection.cs
- __Filters.cs
- rsa.cs
- ToolStripDropTargetManager.cs
- Setter.cs
- BuilderPropertyEntry.cs
- OutputCacheProfileCollection.cs
- ConditionalBranch.cs
- IndexerNameAttribute.cs
- SerialPort.cs
- ButtonFlatAdapter.cs
- UserNameSecurityToken.cs
- SafeLibraryHandle.cs
- ComponentChangingEvent.cs
- MappedMetaModel.cs
- OdbcReferenceCollection.cs
- HttpConfigurationSystem.cs
- SamlAssertion.cs
- TextTreeInsertElementUndoUnit.cs
- SystemIcmpV6Statistics.cs
- CorePropertiesFilter.cs
- AddInStore.cs
- DocumentViewerAutomationPeer.cs
- TextEndOfParagraph.cs
- ClosableStream.cs
- X509Certificate2Collection.cs
- DesignerSerializationVisibilityAttribute.cs
- Win32.cs
- BuilderPropertyEntry.cs
- LineServicesRun.cs
- FormsAuthenticationConfiguration.cs
- GCHandleCookieTable.cs
- XmlSortKey.cs
- EntryWrittenEventArgs.cs
- EntityContainerEntitySetDefiningQuery.cs
- DetailsViewInsertEventArgs.cs
- RequestUriProcessor.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- SendMailErrorEventArgs.cs
- HtmlInputReset.cs
- RankException.cs
- RemoteWebConfigurationHostStream.cs
- ParserExtension.cs
- _UriTypeConverter.cs
- LookupNode.cs
- RenderDataDrawingContext.cs
- SspiNegotiationTokenAuthenticator.cs
- ZipIOCentralDirectoryBlock.cs
- HttpPostedFile.cs
- XamlVector3DCollectionSerializer.cs
- listitem.cs
- XmlBindingWorker.cs
- PropertyCondition.cs
- StatusBarDrawItemEvent.cs
- XmlUTF8TextReader.cs
- RtType.cs
- BinaryNode.cs
- LocationChangedEventArgs.cs
- DataListItem.cs
- ExpandSegment.cs
- DiagnosticTrace.cs
- ButtonChrome.cs
- EnvironmentPermission.cs
- InstanceCollisionException.cs
- FilteredReadOnlyMetadataCollection.cs
- Int32KeyFrameCollection.cs
- HelpInfo.cs
- IDQuery.cs
- XamlBrushSerializer.cs
- HtmlButton.cs
- XmlChildEnumerator.cs
- IIS7ConfigurationLoader.cs
- DefaultPrintController.cs
- Regex.cs
- HitTestDrawingContextWalker.cs