Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Query / ResultAssembly / BitVec.cs / 3 / BitVec.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- namespace System.Data.Query.ResultAssembly { using System.Diagnostics; using System.Text; ////// BitVector helper class; used to keep track of the used columns /// in the result assembly. /// ////// BitVec can be a struct because it contains a readonly reference to an int[]. /// This code is a copy of System.Collections.BitArray so that we can have an efficient implementation of Minus. /// internal struct BitVec { private readonly int[] m_array; private readonly int m_length; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal BitVec(int length) { Debug.Assert(0 < length, "zero length"); m_array = new int[(length + 31) / 32]; m_length = length; } internal int Count { get { return m_length; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Set(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); m_array[index / 32] |= (1 << (index % 32)); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void ClearAll() { for (int i = 0; i < m_array.Length; i++) { m_array[i] = 0; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal bool IsEmpty() { for (int i = 0; i < m_array.Length; i++) { if (0 != m_array[i]) { return false; } } return true; } internal bool IsSet(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); return (m_array[index / 32] & (1 << (index % 32))) != 0; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Or(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] |= value.m_array[i]; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Minus(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] &= ~value.m_array[i]; } } public override string ToString() { StringBuilder sb = new StringBuilder(3 * Count); string separator = string.Empty; for (int i = 0; i < Count; i++) { if (IsSet(i)) { sb.Append(separator); sb.Append(i); separator = ","; } } return sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- namespace System.Data.Query.ResultAssembly { using System.Diagnostics; using System.Text; ////// BitVector helper class; used to keep track of the used columns /// in the result assembly. /// ////// BitVec can be a struct because it contains a readonly reference to an int[]. /// This code is a copy of System.Collections.BitArray so that we can have an efficient implementation of Minus. /// internal struct BitVec { private readonly int[] m_array; private readonly int m_length; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal BitVec(int length) { Debug.Assert(0 < length, "zero length"); m_array = new int[(length + 31) / 32]; m_length = length; } internal int Count { get { return m_length; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Set(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); m_array[index / 32] |= (1 << (index % 32)); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void ClearAll() { for (int i = 0; i < m_array.Length; i++) { m_array[i] = 0; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal bool IsEmpty() { for (int i = 0; i < m_array.Length; i++) { if (0 != m_array[i]) { return false; } } return true; } internal bool IsSet(int index) { Debug.Assert(unchecked((uint)index < (uint)m_length), "index out of range"); return (m_array[index / 32] & (1 << (index % 32))) != 0; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Or(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] |= value.m_array[i]; } } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal void Minus(BitVec value) { Debug.Assert(m_length == value.m_length, "unequal sized bitvec"); for (int i = 0; i < m_array.Length; i++) { m_array[i] &= ~value.m_array[i]; } } public override string ToString() { StringBuilder sb = new StringBuilder(3 * Count); string separator = string.Empty; for (int i = 0; i < Count; i++) { if (IsSet(i)) { sb.Append(separator); sb.Append(i); separator = ","; } } return sb.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
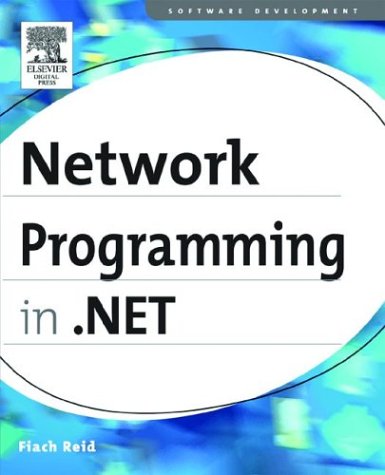
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlUnspecifiedAttribute.cs
- WindowsListViewItemCheckBox.cs
- CellTreeNode.cs
- VarRemapper.cs
- PrintController.cs
- InstanceNameConverter.cs
- DataStreamFromComStream.cs
- QueryableFilterUserControl.cs
- DataGridViewControlCollection.cs
- CompilerLocalReference.cs
- Size3DConverter.cs
- WindowsEditBoxRange.cs
- TextTreeDeleteContentUndoUnit.cs
- Publisher.cs
- UnmanagedBitmapWrapper.cs
- ControlIdConverter.cs
- InvalidateEvent.cs
- TextRangeAdaptor.cs
- SqlDataSourceCommandEventArgs.cs
- WebPartMenu.cs
- TraceSection.cs
- XsltException.cs
- WebPartEventArgs.cs
- Query.cs
- CodeTypeDeclarationCollection.cs
- XappLauncher.cs
- XmlComplianceUtil.cs
- VisualSerializer.cs
- EntityProxyFactory.cs
- IncrementalCompileAnalyzer.cs
- DbSourceCommand.cs
- CultureInfoConverter.cs
- ApplicationBuildProvider.cs
- SafeHandle.cs
- CodeGen.cs
- SerializableAttribute.cs
- InstalledVoice.cs
- VisualTreeHelper.cs
- ScriptDescriptor.cs
- CurrencyWrapper.cs
- ObjectParameterCollection.cs
- ProcessInfo.cs
- ProfileSettings.cs
- WeakReadOnlyCollection.cs
- LinkedList.cs
- ContractNamespaceAttribute.cs
- QilVisitor.cs
- ExtendedProtectionPolicy.cs
- DataSourceProvider.cs
- ContainerFilterService.cs
- __Error.cs
- _IPv4Address.cs
- ThreadExceptionEvent.cs
- Pen.cs
- TranslateTransform.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- UserControlCodeDomTreeGenerator.cs
- ToolBarTray.cs
- UrlPropertyAttribute.cs
- EntityContainerEntitySetDefiningQuery.cs
- MediaElement.cs
- SapiRecoContext.cs
- RoleManagerSection.cs
- StylusPointCollection.cs
- DockPatternIdentifiers.cs
- MarshalByRefObject.cs
- ButtonChrome.cs
- Action.cs
- X509ChainPolicy.cs
- VideoDrawing.cs
- MarkupCompilePass1.cs
- ArrayElementGridEntry.cs
- RelationshipSet.cs
- ReadingWritingEntityEventArgs.cs
- GridViewUpdateEventArgs.cs
- PeerCollaboration.cs
- DetailsViewInsertEventArgs.cs
- RelatedView.cs
- TraceHandlerErrorFormatter.cs
- RepeatButtonAutomationPeer.cs
- ServiceProviders.cs
- ContainsRowNumberChecker.cs
- RSAPKCS1SignatureFormatter.cs
- SyncMethodInvoker.cs
- ListViewSortEventArgs.cs
- DrawingBrush.cs
- BindingList.cs
- InputProcessorProfilesLoader.cs
- newitemfactory.cs
- SessionParameter.cs
- PrintControllerWithStatusDialog.cs
- WinCategoryAttribute.cs
- SettingsPropertyValue.cs
- TemplateXamlParser.cs
- SessionParameter.cs
- NestedContainer.cs
- LightweightCodeGenerator.cs
- SessionStateContainer.cs
- QuaternionValueSerializer.cs
- ProcessingInstructionAction.cs