Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / SystemNet / Net / PeerToPeer / UnsafePeerToPeerMethods.cs / 2 / UnsafePeerToPeerMethods.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Security.Permissions; namespace System.Net.PeerToPeer { using System; using System.Collections.Generic; using System.Text; using Microsoft.Win32.SafeHandles; using System.Security; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using System.Threading; using System.Net.Sockets; [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)] internal struct PEER_PNRP_CLOUD_INFO { internal IntPtr pwzCloudName; internal UInt32 dwScope; internal UInt32 dwScopeId; } [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)] internal struct PEER_PNRP_REGISTRATION_INFO { internal string pwszCloudName; internal string pwszPublishingIdentity; internal UInt32 cAddresses; internal IntPtr ArrayOfSOCKADDRIN6Pointers; internal ushort wport; internal string pwszComment; internal PEER_DATA payLoad; } [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)] internal struct PEER_PNRP_ENDPOINT_INFO { internal IntPtr pwszPeerName; internal UInt32 cAddresses; internal IntPtr ArrayOfSOCKADDRIN6Pointers; internal IntPtr pwszComment; internal PEER_DATA payLoad; } [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)] internal struct PEER_DATA { internal UInt32 cbPayload; internal IntPtr pbPayload; } [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal static class UnsafeP2PNativeMethods { internal const string P2P = "p2p.dll"; [DllImport(P2P, CharSet = CharSet.Unicode)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal extern static void PeerFreeData(IntPtr dataToFree); [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerPnrpGetCloudInfo(out UInt32 pNumClouds, out SafePeerData pArrayOfClouds); [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerPnrpStartup(ushort versionRequired); [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerCreatePeerName(string identity, string classfier, out SafePeerData peerName); //[DllImport(P2P, CharSet = CharSet.Unicode)] //internal extern static Int32 PeerCreatePeerName(string identity, string classfier, out SafePeerData peerName); [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerIdentityGetDefault(out SafePeerData defaultIdentity); /* [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerIdentityCreate(string classifier, string friendlyName, IntPtr hCryptoProv, out SafePeerData defaultIdentity); */ [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerNameToPeerHostName(string peerName, out SafePeerData peerHostName); [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerHostNameToPeerName(string peerHostName, out SafePeerData peerName); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpRegister(string pcwzPeerName, ref PEER_PNRP_REGISTRATION_INFO registrationInfo, out SafePeerNameUnregister handle); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpUnregister(IntPtr handle); [System.Security.SecurityCritical] [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpUpdateRegistration(SafePeerNameUnregister hRegistration, ref PEER_PNRP_REGISTRATION_INFO registrationInfo); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpResolve(string pcwzPeerNAme, string pcwzCloudName, ref UInt32 pcEndPoints, out SafePeerData pEndPoints); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpStartResolve(string pcwzPeerNAme, string pcwzCloudName, UInt32 cEndPoints, SafeWaitHandle hEvent, out SafePeerNameEndResolve safePeerNameEndResolve); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpGetEndpoint(IntPtr Handle, out SafePeerData pEndPoint); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpEndResolve(IntPtr Handle); private static object s_InternalSyncObject; private static bool s_Initialized; private const int PNRP_VERSION = 2; private static object InternalSyncObject { get { if (s_InternalSyncObject == null) { object o = new object(); Interlocked.CompareExchange(ref s_InternalSyncObject, o, null); } return s_InternalSyncObject; } } //// [System.Security.SecurityCritical] internal static void PnrpStartup() { if (!s_Initialized) { lock (InternalSyncObject) { if (!s_Initialized) { Int32 result = PeerPnrpStartup(PNRP_VERSION); if (result != 0) { throw new PeerToPeerException(SR.GetString(SR.Pnrp_StartupFailed), Marshal.GetExceptionForHR(result)); } s_Initialized = true; } } } } //end of method PnrpStartup } //// // // [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal sealed class SafePeerData : SafeHandleZeroOrMinusOneIsInvalid { private SafePeerData() : base(true) { } //private SafePeerData(bool ownsHandle) : base(ownsHandle) { } internal string UnicodeString { get { return Marshal.PtrToStringUni(handle); } } protected override bool ReleaseHandle() { UnsafeP2PNativeMethods.PeerFreeData(handle); SetHandleAsInvalid(); //Mark it closed - This does not change the value of the handle it self SetHandle(IntPtr.Zero); //Mark it invalid - Change the value to Zero return true; } } //// // [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal sealed class SafePeerNameUnregister : SafeHandleZeroOrMinusOneIsInvalid { internal SafePeerNameUnregister() : base(true) { } //internal SafePeerNameUnregister(bool ownsHandle) : base(ownsHandle) { } protected override bool ReleaseHandle() { UnsafeP2PNativeMethods.PeerPnrpUnregister(handle); SetHandleAsInvalid(); //Mark it closed - This does not change the value of the handle it self SetHandle(IntPtr.Zero); //Mark it invalid - Change the value to Zero return true; } } //// // [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal sealed class SafePeerNameEndResolve : SafeHandleZeroOrMinusOneIsInvalid { internal SafePeerNameEndResolve() : base(true) { } //internal SafePeerNameEndResolve(bool ownsHandle) : base(ownsHandle) { } protected override bool ReleaseHandle() { UnsafeP2PNativeMethods.PeerPnrpEndResolve(handle); SetHandleAsInvalid(); //Mark it closed - This does not change the value of the handle it self SetHandle(IntPtr.Zero); //Mark it invalid - Change the value to Zero return true; } } ///// /// Determines whether P2P is installed /// Note static constructors are guaranteed to be /// run in a thread safe manner. so no locks are necessary /// internal static class PeerToPeerOSHelper { private static bool s_supportsP2P = false; private static SafeLoadLibrary s_P2PLibrary = null; //// [System.Security.SecurityCritical] static PeerToPeerOSHelper() { s_P2PLibrary = SafeLoadLibrary.LoadLibraryEx(UnsafeP2PNativeMethods.P2P); if (!s_P2PLibrary.IsInvalid) { IntPtr Address = UnsafeSystemNativeMethods.GetProcAddress(s_P2PLibrary, "PeerCreatePeerName"); if (Address != IntPtr.Zero) { s_supportsP2P = true; } } //else --> the SafeLoadLibrary would have already been marked // closed by the LoadLibraryEx call above. } internal static bool SupportsP2P { get { return s_supportsP2P; } } internal static IntPtr P2PModuleHandle { //// // // // // [System.Security.SecurityCritical] [SecurityPermissionAttribute(SecurityAction.LinkDemand, UnmanagedCode=true)] get { if (!s_P2PLibrary.IsClosed && !s_P2PLibrary.IsInvalid) return s_P2PLibrary.DangerousGetHandle(); return IntPtr.Zero; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// // // // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Security.Permissions; namespace System.Net.PeerToPeer { using System; using System.Collections.Generic; using System.Text; using Microsoft.Win32.SafeHandles; using System.Security; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using System.Threading; using System.Net.Sockets; [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)] internal struct PEER_PNRP_CLOUD_INFO { internal IntPtr pwzCloudName; internal UInt32 dwScope; internal UInt32 dwScopeId; } [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)] internal struct PEER_PNRP_REGISTRATION_INFO { internal string pwszCloudName; internal string pwszPublishingIdentity; internal UInt32 cAddresses; internal IntPtr ArrayOfSOCKADDRIN6Pointers; internal ushort wport; internal string pwszComment; internal PEER_DATA payLoad; } [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)] internal struct PEER_PNRP_ENDPOINT_INFO { internal IntPtr pwszPeerName; internal UInt32 cAddresses; internal IntPtr ArrayOfSOCKADDRIN6Pointers; internal IntPtr pwszComment; internal PEER_DATA payLoad; } [StructLayout(LayoutKind.Sequential, CharSet = CharSet.Unicode)] internal struct PEER_DATA { internal UInt32 cbPayload; internal IntPtr pbPayload; } [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal static class UnsafeP2PNativeMethods { internal const string P2P = "p2p.dll"; [DllImport(P2P, CharSet = CharSet.Unicode)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal extern static void PeerFreeData(IntPtr dataToFree); [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerPnrpGetCloudInfo(out UInt32 pNumClouds, out SafePeerData pArrayOfClouds); [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerPnrpStartup(ushort versionRequired); [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerCreatePeerName(string identity, string classfier, out SafePeerData peerName); //[DllImport(P2P, CharSet = CharSet.Unicode)] //internal extern static Int32 PeerCreatePeerName(string identity, string classfier, out SafePeerData peerName); [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerIdentityGetDefault(out SafePeerData defaultIdentity); /* [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerIdentityCreate(string classifier, string friendlyName, IntPtr hCryptoProv, out SafePeerData defaultIdentity); */ [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerNameToPeerHostName(string peerName, out SafePeerData peerHostName); [DllImport(P2P, CharSet = CharSet.Unicode)] internal extern static Int32 PeerHostNameToPeerName(string peerHostName, out SafePeerData peerName); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpRegister(string pcwzPeerName, ref PEER_PNRP_REGISTRATION_INFO registrationInfo, out SafePeerNameUnregister handle); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpUnregister(IntPtr handle); [System.Security.SecurityCritical] [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpUpdateRegistration(SafePeerNameUnregister hRegistration, ref PEER_PNRP_REGISTRATION_INFO registrationInfo); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpResolve(string pcwzPeerNAme, string pcwzCloudName, ref UInt32 pcEndPoints, out SafePeerData pEndPoints); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpStartResolve(string pcwzPeerNAme, string pcwzCloudName, UInt32 cEndPoints, SafeWaitHandle hEvent, out SafePeerNameEndResolve safePeerNameEndResolve); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpGetEndpoint(IntPtr Handle, out SafePeerData pEndPoint); [DllImport(P2P, CharSet = CharSet.Unicode)] public extern static Int32 PeerPnrpEndResolve(IntPtr Handle); private static object s_InternalSyncObject; private static bool s_Initialized; private const int PNRP_VERSION = 2; private static object InternalSyncObject { get { if (s_InternalSyncObject == null) { object o = new object(); Interlocked.CompareExchange(ref s_InternalSyncObject, o, null); } return s_InternalSyncObject; } } //// [System.Security.SecurityCritical] internal static void PnrpStartup() { if (!s_Initialized) { lock (InternalSyncObject) { if (!s_Initialized) { Int32 result = PeerPnrpStartup(PNRP_VERSION); if (result != 0) { throw new PeerToPeerException(SR.GetString(SR.Pnrp_StartupFailed), Marshal.GetExceptionForHR(result)); } s_Initialized = true; } } } } //end of method PnrpStartup } //// // // [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal sealed class SafePeerData : SafeHandleZeroOrMinusOneIsInvalid { private SafePeerData() : base(true) { } //private SafePeerData(bool ownsHandle) : base(ownsHandle) { } internal string UnicodeString { get { return Marshal.PtrToStringUni(handle); } } protected override bool ReleaseHandle() { UnsafeP2PNativeMethods.PeerFreeData(handle); SetHandleAsInvalid(); //Mark it closed - This does not change the value of the handle it self SetHandle(IntPtr.Zero); //Mark it invalid - Change the value to Zero return true; } } //// // [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal sealed class SafePeerNameUnregister : SafeHandleZeroOrMinusOneIsInvalid { internal SafePeerNameUnregister() : base(true) { } //internal SafePeerNameUnregister(bool ownsHandle) : base(ownsHandle) { } protected override bool ReleaseHandle() { UnsafeP2PNativeMethods.PeerPnrpUnregister(handle); SetHandleAsInvalid(); //Mark it closed - This does not change the value of the handle it self SetHandle(IntPtr.Zero); //Mark it invalid - Change the value to Zero return true; } } //// // [System.Security.SecurityCritical(System.Security.SecurityCriticalScope.Everything)] [System.Security.SuppressUnmanagedCodeSecurityAttribute()] internal sealed class SafePeerNameEndResolve : SafeHandleZeroOrMinusOneIsInvalid { internal SafePeerNameEndResolve() : base(true) { } //internal SafePeerNameEndResolve(bool ownsHandle) : base(ownsHandle) { } protected override bool ReleaseHandle() { UnsafeP2PNativeMethods.PeerPnrpEndResolve(handle); SetHandleAsInvalid(); //Mark it closed - This does not change the value of the handle it self SetHandle(IntPtr.Zero); //Mark it invalid - Change the value to Zero return true; } } ///// /// Determines whether P2P is installed /// Note static constructors are guaranteed to be /// run in a thread safe manner. so no locks are necessary /// internal static class PeerToPeerOSHelper { private static bool s_supportsP2P = false; private static SafeLoadLibrary s_P2PLibrary = null; //// [System.Security.SecurityCritical] static PeerToPeerOSHelper() { s_P2PLibrary = SafeLoadLibrary.LoadLibraryEx(UnsafeP2PNativeMethods.P2P); if (!s_P2PLibrary.IsInvalid) { IntPtr Address = UnsafeSystemNativeMethods.GetProcAddress(s_P2PLibrary, "PeerCreatePeerName"); if (Address != IntPtr.Zero) { s_supportsP2P = true; } } //else --> the SafeLoadLibrary would have already been marked // closed by the LoadLibraryEx call above. } internal static bool SupportsP2P { get { return s_supportsP2P; } } internal static IntPtr P2PModuleHandle { //// // // // // [System.Security.SecurityCritical] [SecurityPermissionAttribute(SecurityAction.LinkDemand, UnmanagedCode=true)] get { if (!s_P2PLibrary.IsClosed && !s_P2PLibrary.IsInvalid) return s_P2PLibrary.DangerousGetHandle(); return IntPtr.Zero; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.// // // //
Link Menu
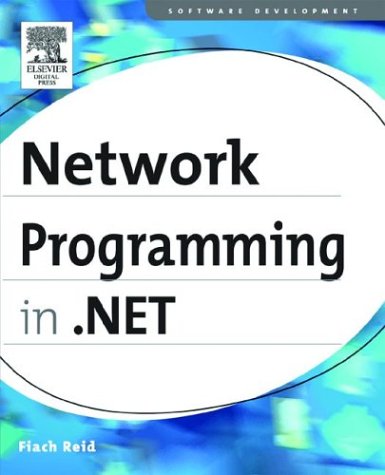
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeDirectoryCompiler.cs
- ConnectionConsumerAttribute.cs
- CharConverter.cs
- WorkflowInstanceContextProvider.cs
- KeyedQueue.cs
- UIElement3DAutomationPeer.cs
- DataGridViewCellValueEventArgs.cs
- MouseActionConverter.cs
- DataGridViewRowsRemovedEventArgs.cs
- _NestedMultipleAsyncResult.cs
- AggregateNode.cs
- TextBoxRenderer.cs
- DataSourceXmlSubItemAttribute.cs
- PreservationFileReader.cs
- ZoneMembershipCondition.cs
- ExpressionBuilderContext.cs
- DelayedRegex.cs
- DetailsViewUpdatedEventArgs.cs
- OletxCommittableTransaction.cs
- PropertySourceInfo.cs
- HttpApplicationFactory.cs
- MsmqChannelListenerBase.cs
- PathFigureCollectionConverter.cs
- RowVisual.cs
- AddInControllerImpl.cs
- TreeNode.cs
- SvcMapFileLoader.cs
- TableCellCollection.cs
- MetaModel.cs
- AssemblyBuilder.cs
- DesignerLabelAdapter.cs
- XmlSchemaProviderAttribute.cs
- XmlDocumentSurrogate.cs
- HitTestFilterBehavior.cs
- ReturnValue.cs
- TextTreeTextBlock.cs
- ButtonBase.cs
- ConstantExpression.cs
- ListCollectionView.cs
- ConditionedDesigner.cs
- ProtectedProviderSettings.cs
- SelectionEditor.cs
- ToolstripProfessionalRenderer.cs
- OleAutBinder.cs
- BindingBase.cs
- HtmlTable.cs
- DbConvert.cs
- ScalarOps.cs
- FileIOPermission.cs
- XmlSchemaChoice.cs
- TypeToTreeConverter.cs
- TdsRecordBufferSetter.cs
- Panel.cs
- DrawingContext.cs
- WS2007FederationHttpBinding.cs
- ColumnHeader.cs
- CodeLabeledStatement.cs
- ModelPropertyImpl.cs
- StringStorage.cs
- EntityConnectionStringBuilderItem.cs
- PerfCounters.cs
- SystemMulticastIPAddressInformation.cs
- SystemIPv6InterfaceProperties.cs
- TableLayoutPanelCellPosition.cs
- ContainerTracking.cs
- MaskedTextBox.cs
- PropertyRecord.cs
- Function.cs
- Focus.cs
- UpdatePanelTrigger.cs
- EncodingNLS.cs
- QilXmlReader.cs
- WinEventHandler.cs
- ZipIOExtraFieldPaddingElement.cs
- DataGridViewColumn.cs
- ZipIOExtraFieldZip64Element.cs
- InstancePersistenceEvent.cs
- HttpRawResponse.cs
- SoapMessage.cs
- ConfigurationSection.cs
- WebControlAdapter.cs
- BindingContext.cs
- BitSet.cs
- HtmlShimManager.cs
- ContainerVisual.cs
- UrlMapping.cs
- DirectionalLight.cs
- TypeConverter.cs
- Graph.cs
- RenderCapability.cs
- Cursors.cs
- TableDetailsCollection.cs
- COM2Properties.cs
- RecipientInfo.cs
- Tokenizer.cs
- Mouse.cs
- AssemblySettingAttributes.cs
- FileDialog.cs
- KeyTime.cs
- WebPartCatalogAddVerb.cs