Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Duration.cs / 1 / Duration.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: Duration.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using System.ComponentModel; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// Duration struct provides sentinel values that are not included in TimeSpan. /// This structure may represent a TimeSpan, Automatic, or Forever value. /// [TypeConverter(typeof(DurationConverter))] public struct Duration { private TimeSpan _timeSpan; private DurationType _durationType; ////// Creates a Duration from a TimeSpan. /// /// public Duration(TimeSpan timeSpan) { if (timeSpan < TimeSpan.Zero) { throw new ArgumentException(SR.Get(SRID.Timing_InvalidArgNonNegative), "timeSpan"); } _durationType = DurationType.TimeSpan; _timeSpan = timeSpan; } #region Operators // // Since Duration has two special values, for comparison purposes // Duration.Forever behaves like Double.PositiveInfinity and // Duration.Automatic behaves almost entirely like Double.NaN // Any comparision with Automatic returns false, except for ==. // Unlike NaN, Automatic == Automatic is true. // ////// Implicitly creates a Duration from a TimeSpan. /// /// ///public static implicit operator Duration(TimeSpan timeSpan) { if (timeSpan < TimeSpan.Zero) { throw new ArgumentException(SR.Get(SRID.Timing_InvalidArgNonNegative), "timeSpan"); } return new Duration(timeSpan); } /// /// Adds two Durations together. /// /// /// ///If both Durations have values then this returns a Duration /// representing the sum of those two values; otherwise a Duration representing null. public static Duration operator +(Duration t1, Duration t2) { if (t1.HasTimeSpan && t2.HasTimeSpan) { return new Duration(t1._timeSpan + t2._timeSpan); } else if (t1._durationType != DurationType.Automatic && t2._durationType != DurationType.Automatic) { // Neither t1 nor t2 are Automatic, so one is Forever // while the other is Forever or a TimeSpan. Either way // the sum is Forever. return Duration.Forever; } else { // Automatic + anything is Automatic return Duration.Automatic; } } ////// Subracts one Duration from another. /// /// /// ///If both Durations have values then this returns a Duration /// representing the value of the second subtracted from the first; otherwise a Duration representing null. public static Duration operator -(Duration t1, Duration t2) { if (t1.HasTimeSpan && t2.HasTimeSpan) { return new Duration(t1._timeSpan - t2._timeSpan); } else if (t1._durationType == DurationType.Forever && t2.HasTimeSpan) { // The only way for the result to be Forever is // if t1 is Forever and t2 is a TimeSpan return Duration.Forever; } else { // This covers the following conditions: // Forever - Forever // TimeSpan - Forever // TimeSpan - Automatic // Forever - Automatic // Automatic - Automatic // Automatic - Forever // Automatic - TimeSpan return Duration.Automatic; } } ////// Indicates whether two Durations are equal. /// /// /// ///true if both of the Durations have values and those values are equal or if both /// Durations represent null; otherwise false. public static bool operator ==(Duration t1, Duration t2) { return t1.Equals(t2); } ////// Indicates whether two Durations are not equal. /// /// /// ///true if exactly one of t1 and t2 represents a value or if they both represent /// values and those values are not equal; otherwise false. public static bool operator !=(Duration t1, Duration t2) { return !(t1.Equals(t2)); } ////// Indicates whether one Duration is greater than another. /// /// /// ///true if both t1 and t2 have values and the value of t1 /// is greater than the value of t2; otherwise false. Forever is /// considered greater than all finite values and any comparison /// with Automatic returns false. public static bool operator >(Duration t1, Duration t2) { if (t1.HasTimeSpan && t2.HasTimeSpan) { return t1._timeSpan > t2._timeSpan; } else if (t1.HasTimeSpan && t2._durationType == DurationType.Forever) { // TimeSpan > Forever is false; return false; } else if (t1._durationType == DurationType.Forever && t2.HasTimeSpan) { // Forever > TimeSpan is true; return true; } else { // Cases covered: // Either t1 or t2 are Automatic, // or t1 and t2 are both Forever return false; } } ////// Indicates whether one Duration is greater than or equal to another. /// /// /// ///true if both t1 and t2 have values and the value of t1 /// is greater than or equal to the value of t2; otherwise false. /// Forever is considered greater than all finite values and any /// comparison with Automatic returns false. public static bool operator >=(Duration t1, Duration t2) { if (t1._durationType == DurationType.Automatic && t2._durationType == DurationType.Automatic) { // Automatic == Automatic return true; } else if (t1._durationType == DurationType.Automatic || t2._durationType == DurationType.Automatic) { // Automatic compared to anything else is false return false; } else { return !(t1 < t2); } } ////// Indicates whether one Duration is less than another. /// /// /// ///true if both t1 and t2 have values and the value of t1 /// is less than the value of t2; otherwise false. Forever is /// considered greater than all finite values and any comparison /// with Automatic returns false public static bool operator <(Duration t1, Duration t2) { if (t1.HasTimeSpan && t2.HasTimeSpan) { return t1._timeSpan < t2._timeSpan; } else if (t1.HasTimeSpan && t2._durationType == DurationType.Forever) { // TimeSpan < Forever is true; return true; } else if (t1._durationType == DurationType.Forever && t2.HasTimeSpan) { // Forever < TimeSpan is true; return false; } else { // Cases covered: // Either t1 or t2 are Automatic, // or t1 and t2 are both Forever return false; } } ////// Indicates whether one Duration is less than or equal to another. /// /// /// ///true if both t1 and t2 have values and the value of t1 /// is less than or equal to the value of t2; otherwise false. /// Forever is considered greater than all finite values and any /// comparison with Automatic returns false. public static bool operator <=(Duration t1, Duration t2) { if (t1._durationType == DurationType.Automatic && t2._durationType == DurationType.Automatic) { // Automatic == Automatic return true; } else if (t1._durationType == DurationType.Automatic || t2._durationType == DurationType.Automatic) { // Automatic compared to anything else is false return false; } else { return !(t1 > t2); } } ////// Compares one Duration value to another. /// /// /// ////// A negative value, zero or a positive value, respectively, if t1 is /// less than, equal or greater than t2. /// /// Duration.Automatic is a special case and has the following return values: /// /// -1 if t1 is Automatic and t2 is not Automatic /// 0 if t1 and t2 are Automatic /// 1 if t1 is not Automatic and t2 is Automatic /// /// This mirrors Double.CompareTo()'s treatment of Double.NaN /// public static int Compare(Duration t1, Duration t2) { if (t1._durationType == DurationType.Automatic) { if (t2._durationType == DurationType.Automatic) { return 0; } else { return -1; } } else if (t2._durationType == DurationType.Automatic) { return 1; } else // Neither are Automatic, do a standard comparison { if (t1 < t2) { return -1; } else if (t1 > t2) { return 1; } else // Neither is greater than the other { return 0; } } } ////// Returns the specified instance of Duration. /// /// ///Returns duration. public static Duration Plus(Duration duration) { return duration; } ////// Returns the specified instance of Duration. /// /// ///Returns duration. public static Duration operator +(Duration duration) { return duration; } #endregion #region Properties ////// Indicates whether this Duration is a TimeSpan value. /// ///true if this Duration is a TimeSpan value. public bool HasTimeSpan { get { return (_durationType == DurationType.TimeSpan); } } ////// Returns a Duration that represents an Automatic value. /// ///A Duration that represents an Automatic value. public static Duration Automatic { get { Duration duration = new Duration(); duration._durationType = DurationType.Automatic; return duration; } } ////// Returns a Duration that represents a Forever value. /// ///A Duration that represents a Forever value. public static Duration Forever { get { Duration duration = new Duration(); duration._durationType = DurationType.Forever; return duration; } } ////// Returns the TimeSpan value that this Duration represents. /// ///The TimeSpan value that this Duration represents. ///Thrown if this Duration represents null. public TimeSpan TimeSpan { get { if (HasTimeSpan) { return _timeSpan; } else { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Timing_NotTimeSpan, this)); } } } #endregion #region Methods ////// Adds the specified Duration to this instance. /// /// ///A Duration that represents the value of this instance plus the value of duration. public Duration Add(Duration duration) { return this + duration; } ////// Indicates whether the specified Object is equal to this Duration. /// /// ///true if value is a Duration and is equal to this instance; otherwise false. public override bool Equals(Object value) { if (value == null) { return false; } else if (value is Duration) { return Equals((Duration)value); } else { return false; } } ////// Indicates whether the specified Duration is equal to this Duration. /// /// ///true if duration is equal to this instance; otherwise false. public bool Equals(Duration duration) { if (HasTimeSpan) { if (duration.HasTimeSpan) { return _timeSpan == duration._timeSpan; } else { return false; } } else { return _durationType == duration._durationType; } } ////// Indicates whether the specified Durations are equal. /// /// /// ///true if t1 equals t2; otherwise false. public static bool Equals(Duration t1, Duration t2) { return t1.Equals(t2); } ////// Returns a hash code for this instance. /// ///A 32-bit signed integer hash code. public override int GetHashCode() { if (HasTimeSpan) { return _timeSpan.GetHashCode(); } else { return _durationType.GetHashCode() + 17; } } ////// Subtracts the specified Duration from this instance. /// /// ///A Duration whose value is the result of the value of this instance minus the value of duration. public Duration Subtract(Duration duration) { return this - duration; } ////// Creates a string representation of this Duration. /// ///A string representation of this Duration. public override string ToString() { if (HasTimeSpan) { return TypeDescriptor.GetConverter(_timeSpan).ConvertToString(_timeSpan); } else if (_durationType == DurationType.Forever) { return "Forever"; } else // IsAutomatic { return "Automatic"; } } #endregion ////// An enumeration of the different types of Duration behaviors. /// private enum DurationType { Automatic, TimeSpan, Forever } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: Duration.cs //----------------------------------------------------------------------------- // Allow suppression of certain presharp messages #pragma warning disable 1634, 1691 using System.ComponentModel; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// Duration struct provides sentinel values that are not included in TimeSpan. /// This structure may represent a TimeSpan, Automatic, or Forever value. /// [TypeConverter(typeof(DurationConverter))] public struct Duration { private TimeSpan _timeSpan; private DurationType _durationType; ////// Creates a Duration from a TimeSpan. /// /// public Duration(TimeSpan timeSpan) { if (timeSpan < TimeSpan.Zero) { throw new ArgumentException(SR.Get(SRID.Timing_InvalidArgNonNegative), "timeSpan"); } _durationType = DurationType.TimeSpan; _timeSpan = timeSpan; } #region Operators // // Since Duration has two special values, for comparison purposes // Duration.Forever behaves like Double.PositiveInfinity and // Duration.Automatic behaves almost entirely like Double.NaN // Any comparision with Automatic returns false, except for ==. // Unlike NaN, Automatic == Automatic is true. // ////// Implicitly creates a Duration from a TimeSpan. /// /// ///public static implicit operator Duration(TimeSpan timeSpan) { if (timeSpan < TimeSpan.Zero) { throw new ArgumentException(SR.Get(SRID.Timing_InvalidArgNonNegative), "timeSpan"); } return new Duration(timeSpan); } /// /// Adds two Durations together. /// /// /// ///If both Durations have values then this returns a Duration /// representing the sum of those two values; otherwise a Duration representing null. public static Duration operator +(Duration t1, Duration t2) { if (t1.HasTimeSpan && t2.HasTimeSpan) { return new Duration(t1._timeSpan + t2._timeSpan); } else if (t1._durationType != DurationType.Automatic && t2._durationType != DurationType.Automatic) { // Neither t1 nor t2 are Automatic, so one is Forever // while the other is Forever or a TimeSpan. Either way // the sum is Forever. return Duration.Forever; } else { // Automatic + anything is Automatic return Duration.Automatic; } } ////// Subracts one Duration from another. /// /// /// ///If both Durations have values then this returns a Duration /// representing the value of the second subtracted from the first; otherwise a Duration representing null. public static Duration operator -(Duration t1, Duration t2) { if (t1.HasTimeSpan && t2.HasTimeSpan) { return new Duration(t1._timeSpan - t2._timeSpan); } else if (t1._durationType == DurationType.Forever && t2.HasTimeSpan) { // The only way for the result to be Forever is // if t1 is Forever and t2 is a TimeSpan return Duration.Forever; } else { // This covers the following conditions: // Forever - Forever // TimeSpan - Forever // TimeSpan - Automatic // Forever - Automatic // Automatic - Automatic // Automatic - Forever // Automatic - TimeSpan return Duration.Automatic; } } ////// Indicates whether two Durations are equal. /// /// /// ///true if both of the Durations have values and those values are equal or if both /// Durations represent null; otherwise false. public static bool operator ==(Duration t1, Duration t2) { return t1.Equals(t2); } ////// Indicates whether two Durations are not equal. /// /// /// ///true if exactly one of t1 and t2 represents a value or if they both represent /// values and those values are not equal; otherwise false. public static bool operator !=(Duration t1, Duration t2) { return !(t1.Equals(t2)); } ////// Indicates whether one Duration is greater than another. /// /// /// ///true if both t1 and t2 have values and the value of t1 /// is greater than the value of t2; otherwise false. Forever is /// considered greater than all finite values and any comparison /// with Automatic returns false. public static bool operator >(Duration t1, Duration t2) { if (t1.HasTimeSpan && t2.HasTimeSpan) { return t1._timeSpan > t2._timeSpan; } else if (t1.HasTimeSpan && t2._durationType == DurationType.Forever) { // TimeSpan > Forever is false; return false; } else if (t1._durationType == DurationType.Forever && t2.HasTimeSpan) { // Forever > TimeSpan is true; return true; } else { // Cases covered: // Either t1 or t2 are Automatic, // or t1 and t2 are both Forever return false; } } ////// Indicates whether one Duration is greater than or equal to another. /// /// /// ///true if both t1 and t2 have values and the value of t1 /// is greater than or equal to the value of t2; otherwise false. /// Forever is considered greater than all finite values and any /// comparison with Automatic returns false. public static bool operator >=(Duration t1, Duration t2) { if (t1._durationType == DurationType.Automatic && t2._durationType == DurationType.Automatic) { // Automatic == Automatic return true; } else if (t1._durationType == DurationType.Automatic || t2._durationType == DurationType.Automatic) { // Automatic compared to anything else is false return false; } else { return !(t1 < t2); } } ////// Indicates whether one Duration is less than another. /// /// /// ///true if both t1 and t2 have values and the value of t1 /// is less than the value of t2; otherwise false. Forever is /// considered greater than all finite values and any comparison /// with Automatic returns false public static bool operator <(Duration t1, Duration t2) { if (t1.HasTimeSpan && t2.HasTimeSpan) { return t1._timeSpan < t2._timeSpan; } else if (t1.HasTimeSpan && t2._durationType == DurationType.Forever) { // TimeSpan < Forever is true; return true; } else if (t1._durationType == DurationType.Forever && t2.HasTimeSpan) { // Forever < TimeSpan is true; return false; } else { // Cases covered: // Either t1 or t2 are Automatic, // or t1 and t2 are both Forever return false; } } ////// Indicates whether one Duration is less than or equal to another. /// /// /// ///true if both t1 and t2 have values and the value of t1 /// is less than or equal to the value of t2; otherwise false. /// Forever is considered greater than all finite values and any /// comparison with Automatic returns false. public static bool operator <=(Duration t1, Duration t2) { if (t1._durationType == DurationType.Automatic && t2._durationType == DurationType.Automatic) { // Automatic == Automatic return true; } else if (t1._durationType == DurationType.Automatic || t2._durationType == DurationType.Automatic) { // Automatic compared to anything else is false return false; } else { return !(t1 > t2); } } ////// Compares one Duration value to another. /// /// /// ////// A negative value, zero or a positive value, respectively, if t1 is /// less than, equal or greater than t2. /// /// Duration.Automatic is a special case and has the following return values: /// /// -1 if t1 is Automatic and t2 is not Automatic /// 0 if t1 and t2 are Automatic /// 1 if t1 is not Automatic and t2 is Automatic /// /// This mirrors Double.CompareTo()'s treatment of Double.NaN /// public static int Compare(Duration t1, Duration t2) { if (t1._durationType == DurationType.Automatic) { if (t2._durationType == DurationType.Automatic) { return 0; } else { return -1; } } else if (t2._durationType == DurationType.Automatic) { return 1; } else // Neither are Automatic, do a standard comparison { if (t1 < t2) { return -1; } else if (t1 > t2) { return 1; } else // Neither is greater than the other { return 0; } } } ////// Returns the specified instance of Duration. /// /// ///Returns duration. public static Duration Plus(Duration duration) { return duration; } ////// Returns the specified instance of Duration. /// /// ///Returns duration. public static Duration operator +(Duration duration) { return duration; } #endregion #region Properties ////// Indicates whether this Duration is a TimeSpan value. /// ///true if this Duration is a TimeSpan value. public bool HasTimeSpan { get { return (_durationType == DurationType.TimeSpan); } } ////// Returns a Duration that represents an Automatic value. /// ///A Duration that represents an Automatic value. public static Duration Automatic { get { Duration duration = new Duration(); duration._durationType = DurationType.Automatic; return duration; } } ////// Returns a Duration that represents a Forever value. /// ///A Duration that represents a Forever value. public static Duration Forever { get { Duration duration = new Duration(); duration._durationType = DurationType.Forever; return duration; } } ////// Returns the TimeSpan value that this Duration represents. /// ///The TimeSpan value that this Duration represents. ///Thrown if this Duration represents null. public TimeSpan TimeSpan { get { if (HasTimeSpan) { return _timeSpan; } else { #pragma warning suppress 56503 // Suppress presharp warning: Follows a pattern similar to Nullable. throw new InvalidOperationException(SR.Get(SRID.Timing_NotTimeSpan, this)); } } } #endregion #region Methods ////// Adds the specified Duration to this instance. /// /// ///A Duration that represents the value of this instance plus the value of duration. public Duration Add(Duration duration) { return this + duration; } ////// Indicates whether the specified Object is equal to this Duration. /// /// ///true if value is a Duration and is equal to this instance; otherwise false. public override bool Equals(Object value) { if (value == null) { return false; } else if (value is Duration) { return Equals((Duration)value); } else { return false; } } ////// Indicates whether the specified Duration is equal to this Duration. /// /// ///true if duration is equal to this instance; otherwise false. public bool Equals(Duration duration) { if (HasTimeSpan) { if (duration.HasTimeSpan) { return _timeSpan == duration._timeSpan; } else { return false; } } else { return _durationType == duration._durationType; } } ////// Indicates whether the specified Durations are equal. /// /// /// ///true if t1 equals t2; otherwise false. public static bool Equals(Duration t1, Duration t2) { return t1.Equals(t2); } ////// Returns a hash code for this instance. /// ///A 32-bit signed integer hash code. public override int GetHashCode() { if (HasTimeSpan) { return _timeSpan.GetHashCode(); } else { return _durationType.GetHashCode() + 17; } } ////// Subtracts the specified Duration from this instance. /// /// ///A Duration whose value is the result of the value of this instance minus the value of duration. public Duration Subtract(Duration duration) { return this - duration; } ////// Creates a string representation of this Duration. /// ///A string representation of this Duration. public override string ToString() { if (HasTimeSpan) { return TypeDescriptor.GetConverter(_timeSpan).ConvertToString(_timeSpan); } else if (_durationType == DurationType.Forever) { return "Forever"; } else // IsAutomatic { return "Automatic"; } } #endregion ////// An enumeration of the different types of Duration behaviors. /// private enum DurationType { Automatic, TimeSpan, Forever } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
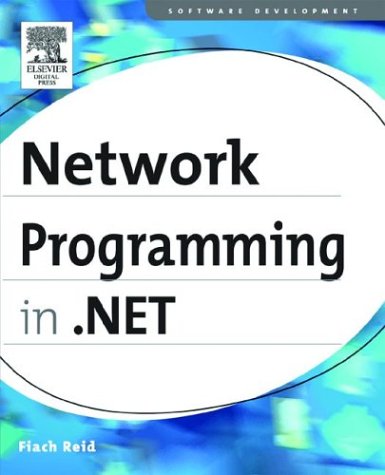
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlDataRecord.cs
- GreaterThanOrEqual.cs
- ServicePointManager.cs
- Int16.cs
- XmlRootAttribute.cs
- DataGridBoolColumn.cs
- FillErrorEventArgs.cs
- SaveFileDialog.cs
- ExpressionBindings.cs
- DtrList.cs
- ListBox.cs
- StaticTextPointer.cs
- WinFormsComponentEditor.cs
- CombinedGeometry.cs
- AssertFilter.cs
- InputMethodStateChangeEventArgs.cs
- TimeSpan.cs
- ReadOnlyActivityGlyph.cs
- HasCopySemanticsAttribute.cs
- ListSourceHelper.cs
- XmlTypeAttribute.cs
- SqlFunctionAttribute.cs
- AccessorTable.cs
- XmlLoader.cs
- InternalConfigRoot.cs
- ToolboxItemSnapLineBehavior.cs
- TypeResolver.cs
- XmlTypeAttribute.cs
- SingleResultAttribute.cs
- Int32Animation.cs
- CheckBoxAutomationPeer.cs
- OpenTypeLayoutCache.cs
- XamlPointCollectionSerializer.cs
- WindowsTokenRoleProvider.cs
- TreeViewItem.cs
- SqlDependencyListener.cs
- KeyMatchBuilder.cs
- XmlAnyElementAttributes.cs
- SqlTriggerContext.cs
- COM2ICategorizePropertiesHandler.cs
- Binding.cs
- ContextMenuAutomationPeer.cs
- ControlIdConverter.cs
- DataPagerFieldCollection.cs
- ModelEditingScope.cs
- WebPartMinimizeVerb.cs
- RowTypeElement.cs
- BindingCompleteEventArgs.cs
- ReadContentAsBinaryHelper.cs
- Action.cs
- HostProtectionException.cs
- XsltException.cs
- DocumentReferenceCollection.cs
- DependencyPropertyAttribute.cs
- WeakReadOnlyCollection.cs
- MultiView.cs
- ListBindingHelper.cs
- ContainerVisual.cs
- DateTimeOffsetStorage.cs
- FocusChangedEventArgs.cs
- PerformanceCounterManager.cs
- DBDataPermission.cs
- ValidatingReaderNodeData.cs
- WebConfigurationHostFileChange.cs
- XmlNamespaceMapping.cs
- CharacterHit.cs
- GridViewColumn.cs
- QueryContinueDragEvent.cs
- HtmlInputImage.cs
- CommonDialog.cs
- TabItemAutomationPeer.cs
- RepeaterItem.cs
- ZipIOExtraFieldPaddingElement.cs
- MetadataProperty.cs
- Label.cs
- ThreadAbortException.cs
- BindingCompleteEventArgs.cs
- pingexception.cs
- RsaKeyIdentifierClause.cs
- HttpModulesSection.cs
- ResourceContainer.cs
- EqualityArray.cs
- CssTextWriter.cs
- DataGridAutomationPeer.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- SpecialNameAttribute.cs
- XmlSchemaParticle.cs
- LeaseManager.cs
- ToggleButton.cs
- ViewStateException.cs
- TrackingRecord.cs
- prompt.cs
- RegexCompilationInfo.cs
- TextEndOfSegment.cs
- ObjectDataSourceView.cs
- SqlDataSourceSummaryPanel.cs
- Compensation.cs
- TypeValidationEventArgs.cs
- DataGridViewRowPostPaintEventArgs.cs
- XmlnsDictionary.cs