Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / ColorContextHelper.cs / 1 / ColorContextHelper.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2001, 2002, 2003 // // File: ColorContextHelper.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Imaging; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Diagnostics; using System.Globalization; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { #region SafeProfileHandle internal class SafeProfileHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// [SecurityCritical] internal SafeProfileHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// This code calls SetHandle /// [SecurityCritical] internal SafeProfileHandle(IntPtr profile) : base(true) { SetHandle(profile); } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid color context handle. /// [SecurityCritical] protected override bool ReleaseHandle() { if (!UnsafeNativeMethods.Mscms.CloseColorProfile(handle)) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } return true; } } #endregion #region ColorContextHelper ////// Class to call into MSCMS color context APIs /// internal class ColorContextHelper { /// Constructor internal ColorContextHelper() { } /// Opens a color profile ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void OpenColorProfile(IntPtr pProfile) { // No need to get rid of the old handle as it will get GC'ed _profileHandle = UnsafeNativeMethods.Mscms.OpenColorProfile( pProfile, NativeMethods.PROFILE_READ, // DesiredAccess NativeMethods.FILE_SHARE_READ, // ShareMode NativeMethods.OPEN_EXISTING // CreationMode ); if (_profileHandle == null || _profileHandle.IsInvalid) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } } /// Retrieves the profile header ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileHeader(IntPtr pHeader) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileHeader(_profileHandle, pHeader)); } /// Retrieves the color profile from handle ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileFromHandle(IntPtr pBuffer, IntPtr pdwSize) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileFromHandle(_profileHandle, pBuffer, pdwSize)); } ////// Critical - Accesses critical resource _profileHandle /// TreatAsSafe - No inputs and just checks if SafeHandle is valid or not. /// internal bool IsInvalid { [SecurityCritical, SecurityTreatAsSafe] get { return (_profileHandle == null || _profileHandle.IsInvalid); } } ////// ProfileHandle /// ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// internal SafeProfileHandle ProfileHandle { [SecurityCritical] get { return _profileHandle; } } #region Data members ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// [SecurityCritical] SafeProfileHandle _profileHandle; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2001, 2002, 2003 // // File: ColorContextHelper.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Imaging; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Diagnostics; using System.Globalization; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { #region SafeProfileHandle internal class SafeProfileHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// [SecurityCritical] internal SafeProfileHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// This code calls SetHandle /// [SecurityCritical] internal SafeProfileHandle(IntPtr profile) : base(true) { SetHandle(profile); } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid color context handle. /// [SecurityCritical] protected override bool ReleaseHandle() { if (!UnsafeNativeMethods.Mscms.CloseColorProfile(handle)) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } return true; } } #endregion #region ColorContextHelper ////// Class to call into MSCMS color context APIs /// internal class ColorContextHelper { /// Constructor internal ColorContextHelper() { } /// Opens a color profile ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void OpenColorProfile(IntPtr pProfile) { // No need to get rid of the old handle as it will get GC'ed _profileHandle = UnsafeNativeMethods.Mscms.OpenColorProfile( pProfile, NativeMethods.PROFILE_READ, // DesiredAccess NativeMethods.FILE_SHARE_READ, // ShareMode NativeMethods.OPEN_EXISTING // CreationMode ); if (_profileHandle == null || _profileHandle.IsInvalid) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } } /// Retrieves the profile header ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileHeader(IntPtr pHeader) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileHeader(_profileHandle, pHeader)); } /// Retrieves the color profile from handle ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileFromHandle(IntPtr pBuffer, IntPtr pdwSize) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileFromHandle(_profileHandle, pBuffer, pdwSize)); } ////// Critical - Accesses critical resource _profileHandle /// TreatAsSafe - No inputs and just checks if SafeHandle is valid or not. /// internal bool IsInvalid { [SecurityCritical, SecurityTreatAsSafe] get { return (_profileHandle == null || _profileHandle.IsInvalid); } } ////// ProfileHandle /// ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// internal SafeProfileHandle ProfileHandle { [SecurityCritical] get { return _profileHandle; } } #region Data members ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// [SecurityCritical] SafeProfileHandle _profileHandle; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
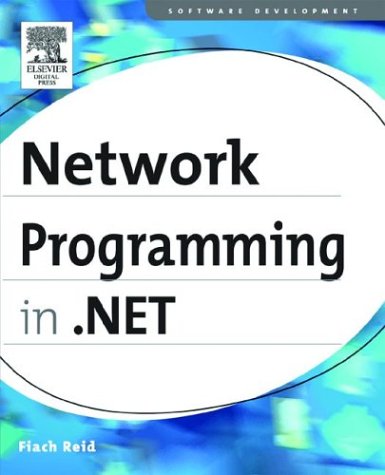
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataException.cs
- ExpressionConverter.cs
- ButtonField.cs
- GeneralTransform3DTo2DTo3D.cs
- DropDownList.cs
- GeometryHitTestParameters.cs
- ImageButton.cs
- ManipulationBoundaryFeedbackEventArgs.cs
- SapiAttributeParser.cs
- EditingCoordinator.cs
- InternalControlCollection.cs
- LocatorManager.cs
- LocalFileSettingsProvider.cs
- Int64AnimationUsingKeyFrames.cs
- SqlRowUpdatingEvent.cs
- ConnectionStringsExpressionBuilder.cs
- ToolStripItemRenderEventArgs.cs
- SessionPageStatePersister.cs
- XmlSchemaProviderAttribute.cs
- SecureUICommand.cs
- RouteParametersHelper.cs
- DesignerForm.cs
- RegexWorker.cs
- XPathSelfQuery.cs
- BamlTreeNode.cs
- DESCryptoServiceProvider.cs
- XmlSignatureManifest.cs
- HiddenField.cs
- SqlInfoMessageEvent.cs
- SafeFileMappingHandle.cs
- WebPartConnection.cs
- QuadTree.cs
- Ray3DHitTestResult.cs
- TextParagraphCache.cs
- WebPartManagerInternals.cs
- Schedule.cs
- XmlSecureResolver.cs
- CollectionEditVerbManager.cs
- CalendarDateRange.cs
- DurationConverter.cs
- Formatter.cs
- DocumentViewerAutomationPeer.cs
- StrongName.cs
- DbDataRecord.cs
- HeaderCollection.cs
- WsiProfilesElementCollection.cs
- SequentialWorkflowRootDesigner.cs
- PerformanceCounterPermissionAttribute.cs
- Rotation3DAnimation.cs
- StylusDevice.cs
- Automation.cs
- MTConfigUtil.cs
- MustUnderstandBehavior.cs
- UInt16Converter.cs
- ClientApiGenerator.cs
- ping.cs
- NetworkCredential.cs
- DescendantBaseQuery.cs
- ExpressionPrefixAttribute.cs
- WindowsGraphics.cs
- EventDescriptorCollection.cs
- IdentifierCollection.cs
- oledbconnectionstring.cs
- PKCS1MaskGenerationMethod.cs
- BuildResultCache.cs
- TypeConverters.cs
- Win32.cs
- ColorBlend.cs
- MDIControlStrip.cs
- AdornerDecorator.cs
- DurationConverter.cs
- ActiveXHelper.cs
- DeferredElementTreeState.cs
- FolderNameEditor.cs
- FormViewDeletedEventArgs.cs
- CacheChildrenQuery.cs
- EncodingFallbackAwareXmlTextWriter.cs
- ReadOnlyHierarchicalDataSource.cs
- XPathMessageContext.cs
- DataSourceCacheDurationConverter.cs
- DataGridCommandEventArgs.cs
- LocatorManager.cs
- SqlDataSourceSelectingEventArgs.cs
- TablePattern.cs
- NamespaceEmitter.cs
- ErrorEventArgs.cs
- SeekStoryboard.cs
- ObjectDataSource.cs
- CallSiteHelpers.cs
- InternalConfigRoot.cs
- XmlEncodedRawTextWriter.cs
- versioninfo.cs
- CodeIdentifiers.cs
- MemberNameValidator.cs
- RepeaterCommandEventArgs.cs
- __Filters.cs
- XmlTextReader.cs
- DataGridToolTip.cs
- ImageList.cs
- AssociationSetEnd.cs