Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Effects / BitmapEffectGroup.cs / 1 / BitmapEffectGroup.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapEffectGroup.cs //----------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Windows.Media.Animation; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Windows.Markup; using System.Windows.Media.Composition; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Effects { ////// This contains the managed effect object and /// a corresponding unmanaged handle /// internal struct BitmapEffectHandle { public BitmapEffect effect; public SafeHandle handle; public BitmapEffectHandle(BitmapEffect e, SafeHandle h) { effect = e; handle = h; } } ////// The class defintion for BitmapEffectGroup /// [ContentProperty("Children")] public sealed partial class BitmapEffectGroup : BitmapEffect { List_childrenHandles; Dictionary > _mapGroupToChildren; /// /// /// public BitmapEffectGroup() { _mapGroupToChildren = new Dictionary>(); } /// /// 1. Updates (propagates) the properties to the unmanaged handle of all /// the child effects /// 2. Sets up all the connections /// 3. Wraps the list with the aggregate effect /// /// Unmanaged handle for aggregate effect protected override void UpdateUnmanagedPropertyState(SafeHandle unmanagedEffect) { // if the group has no children, there is nothing to update if (Children == null || Children.Count <= 0) return; Listchildren = GetChildrenHandles(unmanagedEffect); for (int i = 0; i < children.Count; i++) { children[i].effect.Internal_UpdateUnmanagedPropertyState(children[i].handle); } SetupConnections(unmanagedEffect, children); } /// /// Setup the connections /// private void SetupConnections(SafeHandle unmanagedEffect, Listchildren) { if (!_connectionsSetup) { // find the first effect that has both inputs and outputs BitmapEffectHandle firstEffect = children[0]; int index = 0; for (; index < children.Count; index++) { if (BitmapEffect.GetNumberInputs(children[index].handle) >= 1 && BitmapEffect.GetNumberOutputs(children[index].handle) >= 1) { firstEffect = children[index]; break; } } if (index >= children.Count) return; BitmapEffectHandle lastEffect = firstEffect; // update the connections for (int i = index + 1; i < children.Count; i++) { // skip any effects that don't have inputs or outputs if (BitmapEffect.GetNumberInputs(children[i].handle) < 1 || BitmapEffect.GetNumberOutputs(children[i].handle) < 1) continue; BitmapEffect.GetInputConnector(children[i].handle, 0).ConnectTo( BitmapEffect.GetOutputConnector(lastEffect.handle, 0)); lastEffect = children[i]; } // we should have already handled these cases Debug.Assert(BitmapEffect.GetNumberInputs(firstEffect.handle) >= 1); Debug.Assert(BitmapEffect.GetNumberOutputs(lastEffect.handle) >= 1); BitmapEffect.GetInputConnector(firstEffect.handle, 0).ConnectTo( BitmapEffect.GetInteriorInputConnector(unmanagedEffect, 0)); BitmapEffect.GetInteriorOutputConnector(unmanagedEffect, 0).ConnectTo( BitmapEffect.GetOutputConnector(lastEffect.handle, 0)); BitmapEffect.Add(unmanagedEffect, lastEffect.handle); _connectionsSetup = true; } } /// /// Resets the cache of unmanaged effects /// internal void ChildrenPropertyChangedHook(DependencyPropertyChangedEventArgs e) { // we are caching the unmanaged effects, so if the collection changes // we want to throw away the cache _childrenHandles = null; UnmanagedEffectGroup = null; _mapGroupToChildren.Clear(); _connectionsSetup = false; } ////// Returns a list of the child effect handles, given the unmanaged /// aggregate handle. /// /// unmanaged aggregate handle ///private List GetChildrenHandles(SafeHandle unmanagedEffect) { if (UnmanagedEffectGroup == unmanagedEffect) { if (_childrenHandles == null) { _childrenHandles = CreateEffectHandleList(); } return _childrenHandles; } if (_mapGroupToChildren[unmanagedEffect] == null) { _mapGroupToChildren[unmanagedEffect] = CreateEffectHandleList(); } return _mapGroupToChildren[unmanagedEffect]; } /// /// Creates a list of unmanaged effect handles from the list of BitmapEffect children /// ///private List CreateEffectHandleList() { if (Children == null) return null; List handles = new List (Children.Count); foreach (BitmapEffect e in Children) { handles.Add(new BitmapEffectHandle(e, e.Internal_CreateUnmanagedEffect())); } return handles; } /// /// Updates (propagates) the properties for the aggreagate effect. /// /// This is called only if GetOutput is called directly on the BitmapEffectGroup /// object. /// ///internal override bool UpdateUnmanagedGroupState() { if (Children == null || Children.Count <= 0) return false; UpdateUnmanagedPropertyState(UnmanagedEffectGroup); if (BitmapEffect.GetNumberInputs(UnmanagedEffectGroup) < 1 || BitmapEffect.GetNumberOutputs(UnmanagedEffectGroup) < 1) { return false; } return true; } /// /// Create an unmanaged handle for the group effect /// ///protected override unsafe SafeHandle CreateUnmanagedEffect() { SafeMILHandle effect = Create(new Guid("AC9C1A9A-7E18-4F64-AC7E-47CF7F051E95")); _mapGroupToChildren.Add(effect, null); return effect; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: BitmapEffectGroup.cs //----------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Windows.Media.Animation; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Windows.Markup; using System.Windows.Media.Composition; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Effects { /// /// This contains the managed effect object and /// a corresponding unmanaged handle /// internal struct BitmapEffectHandle { public BitmapEffect effect; public SafeHandle handle; public BitmapEffectHandle(BitmapEffect e, SafeHandle h) { effect = e; handle = h; } } ////// The class defintion for BitmapEffectGroup /// [ContentProperty("Children")] public sealed partial class BitmapEffectGroup : BitmapEffect { List_childrenHandles; Dictionary > _mapGroupToChildren; /// /// /// public BitmapEffectGroup() { _mapGroupToChildren = new Dictionary>(); } /// /// 1. Updates (propagates) the properties to the unmanaged handle of all /// the child effects /// 2. Sets up all the connections /// 3. Wraps the list with the aggregate effect /// /// Unmanaged handle for aggregate effect protected override void UpdateUnmanagedPropertyState(SafeHandle unmanagedEffect) { // if the group has no children, there is nothing to update if (Children == null || Children.Count <= 0) return; Listchildren = GetChildrenHandles(unmanagedEffect); for (int i = 0; i < children.Count; i++) { children[i].effect.Internal_UpdateUnmanagedPropertyState(children[i].handle); } SetupConnections(unmanagedEffect, children); } /// /// Setup the connections /// private void SetupConnections(SafeHandle unmanagedEffect, Listchildren) { if (!_connectionsSetup) { // find the first effect that has both inputs and outputs BitmapEffectHandle firstEffect = children[0]; int index = 0; for (; index < children.Count; index++) { if (BitmapEffect.GetNumberInputs(children[index].handle) >= 1 && BitmapEffect.GetNumberOutputs(children[index].handle) >= 1) { firstEffect = children[index]; break; } } if (index >= children.Count) return; BitmapEffectHandle lastEffect = firstEffect; // update the connections for (int i = index + 1; i < children.Count; i++) { // skip any effects that don't have inputs or outputs if (BitmapEffect.GetNumberInputs(children[i].handle) < 1 || BitmapEffect.GetNumberOutputs(children[i].handle) < 1) continue; BitmapEffect.GetInputConnector(children[i].handle, 0).ConnectTo( BitmapEffect.GetOutputConnector(lastEffect.handle, 0)); lastEffect = children[i]; } // we should have already handled these cases Debug.Assert(BitmapEffect.GetNumberInputs(firstEffect.handle) >= 1); Debug.Assert(BitmapEffect.GetNumberOutputs(lastEffect.handle) >= 1); BitmapEffect.GetInputConnector(firstEffect.handle, 0).ConnectTo( BitmapEffect.GetInteriorInputConnector(unmanagedEffect, 0)); BitmapEffect.GetInteriorOutputConnector(unmanagedEffect, 0).ConnectTo( BitmapEffect.GetOutputConnector(lastEffect.handle, 0)); BitmapEffect.Add(unmanagedEffect, lastEffect.handle); _connectionsSetup = true; } } /// /// Resets the cache of unmanaged effects /// internal void ChildrenPropertyChangedHook(DependencyPropertyChangedEventArgs e) { // we are caching the unmanaged effects, so if the collection changes // we want to throw away the cache _childrenHandles = null; UnmanagedEffectGroup = null; _mapGroupToChildren.Clear(); _connectionsSetup = false; } ////// Returns a list of the child effect handles, given the unmanaged /// aggregate handle. /// /// unmanaged aggregate handle ///private List GetChildrenHandles(SafeHandle unmanagedEffect) { if (UnmanagedEffectGroup == unmanagedEffect) { if (_childrenHandles == null) { _childrenHandles = CreateEffectHandleList(); } return _childrenHandles; } if (_mapGroupToChildren[unmanagedEffect] == null) { _mapGroupToChildren[unmanagedEffect] = CreateEffectHandleList(); } return _mapGroupToChildren[unmanagedEffect]; } /// /// Creates a list of unmanaged effect handles from the list of BitmapEffect children /// ///private List CreateEffectHandleList() { if (Children == null) return null; List handles = new List (Children.Count); foreach (BitmapEffect e in Children) { handles.Add(new BitmapEffectHandle(e, e.Internal_CreateUnmanagedEffect())); } return handles; } /// /// Updates (propagates) the properties for the aggreagate effect. /// /// This is called only if GetOutput is called directly on the BitmapEffectGroup /// object. /// ///internal override bool UpdateUnmanagedGroupState() { if (Children == null || Children.Count <= 0) return false; UpdateUnmanagedPropertyState(UnmanagedEffectGroup); if (BitmapEffect.GetNumberInputs(UnmanagedEffectGroup) < 1 || BitmapEffect.GetNumberOutputs(UnmanagedEffectGroup) < 1) { return false; } return true; } /// /// Create an unmanaged handle for the group effect /// ///protected override unsafe SafeHandle CreateUnmanagedEffect() { SafeMILHandle effect = Create(new Guid("AC9C1A9A-7E18-4F64-AC7E-47CF7F051E95")); _mapGroupToChildren.Add(effect, null); return effect; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
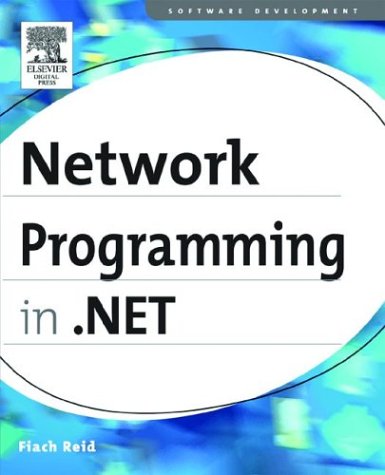
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OutputCacheSettingsSection.cs
- DataSourceHelper.cs
- CultureInfo.cs
- FocusWithinProperty.cs
- OverflowException.cs
- MenuItemBinding.cs
- HttpServerChannel.cs
- Knowncolors.cs
- HoistedLocals.cs
- ToolboxDataAttribute.cs
- TimeSpanHelper.cs
- ScanQueryOperator.cs
- MatchAttribute.cs
- InstanceHandleReference.cs
- WebPartCollection.cs
- XmlArrayItemAttributes.cs
- Schema.cs
- ComponentResourceKeyConverter.cs
- DialogResultConverter.cs
- SQLInt64Storage.cs
- SingleAnimationBase.cs
- BitmapEffect.cs
- XmlDownloadManager.cs
- NavigationExpr.cs
- XsltSettings.cs
- HtmlInputControl.cs
- StrokeCollectionConverter.cs
- Types.cs
- UnknownWrapper.cs
- RangeBase.cs
- CompositionAdorner.cs
- xmlglyphRunInfo.cs
- IISMapPath.cs
- IResourceProvider.cs
- UrlMapping.cs
- MailAddressCollection.cs
- RowToFieldTransformer.cs
- VisualTreeHelper.cs
- XPathCompileException.cs
- Storyboard.cs
- RightsManagementPermission.cs
- XPathScanner.cs
- WSSecureConversationDec2005.cs
- ComEventsInfo.cs
- DataGridViewDataErrorEventArgs.cs
- XmlTextReaderImpl.cs
- CapabilitiesRule.cs
- CellLabel.cs
- SqlNotificationRequest.cs
- SizeKeyFrameCollection.cs
- ThicknessAnimationBase.cs
- StrokeNodeOperations2.cs
- Label.cs
- MeasureItemEvent.cs
- CommandExpr.cs
- DbProviderServices.cs
- PageRequestManager.cs
- InternalBufferOverflowException.cs
- Viewport3DAutomationPeer.cs
- WmfPlaceableFileHeader.cs
- TypeUsage.cs
- Authorization.cs
- isolationinterop.cs
- SocketException.cs
- AnimatedTypeHelpers.cs
- ComboBox.cs
- SortKey.cs
- XsdDuration.cs
- GeometryGroup.cs
- ExpandedWrapper.cs
- OptimizerPatterns.cs
- SecurityElement.cs
- OverflowException.cs
- ListViewItemSelectionChangedEvent.cs
- HttpHeaderCollection.cs
- HostingPreferredMapPath.cs
- UserControlBuildProvider.cs
- MediaElement.cs
- MenuBindingsEditor.cs
- FileDialogPermission.cs
- TextBlock.cs
- Select.cs
- GridViewEditEventArgs.cs
- GraphicsPathIterator.cs
- ControlParameter.cs
- QueryUtil.cs
- DiagnosticStrings.cs
- OraclePermission.cs
- BitmapCache.cs
- _SecureChannel.cs
- StatusBar.cs
- TransportationConfigurationTypeInstallComponent.cs
- BulletedList.cs
- ContextItemManager.cs
- EpmContentSerializerBase.cs
- SelectionItemPattern.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- XdrBuilder.cs
- HttpModuleActionCollection.cs
- ParameterToken.cs