Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / PtsHost / PageBreakRecord.cs / 1 / PageBreakRecord.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: PageBreakRecord.cs // // Description: Break records for PTS pages. // //--------------------------------------------------------------------------- using System; // IntPtr, IDisposable, ... using System.Threading; // Interlocked using System.Security; // SecurityCritical using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS namespace MS.Internal.PtsHost { ////// PageBreakRecord is used to represent a break record for top level PTS /// page. Points to break record structure of PTS page. /// internal sealed class PageBreakRecord : IDisposable { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// Current PTS Context. /// PTS page break record. /// Page number. internal PageBreakRecord(PtsContext ptsContext, SecurityCriticalDataForSetbr, int pageNumber) { Invariant.Assert(ptsContext != null, "Invalid PtsContext object."); Invariant.Assert(br.Value != IntPtr.Zero, "Invalid break record object."); _br = br; _pageNumber = pageNumber; // In the finalizer we may need to reference an object instance // to do the right cleanup. For this reason store a WeakReference // to such object. _ptsContext = new WeakReference(ptsContext); // BreakRecord contains unmanaged resources that need to be released when // BreakRecord is destroyed or Dispatcher is closing. For this reason keep // track of this BreakRecord in PtsContext. ptsContext.OnPageBreakRecordCreated(_br); } /// /// Finalizer - releases unmanaged resources. /// ~PageBreakRecord() { Dispose(false); } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Dispose allocated resources. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } #endregion Public Methods //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- #region Internal Properties ////// PTS page break record. /// internal IntPtr BreakRecord { get { return _br.Value; } } ////// Page number of the page starting at the break position. /// internal int PageNumber { get { return _pageNumber; } } #endregion Internal Properties //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods ////// Destroys all unmanaged resources. /// /// Whether dispose is caused by explicit call to Dispose. ////// Finalizer needs to follow rules below: /// a) Your Finalize method must tolerate partially constructed instances. /// b) Your Finalize method must consider the consequence of failure. /// c) Your object is callable after Finalization. /// d) Your object is callable during Finalization. /// e) Your Finalizer could be called multiple times. /// f) Your Finalizer runs in a delicate security context. /// See: http://blogs.msdn.com/[....]/archive/2004/02/20/77460.aspx /// ////// Critical, because sets SecurityCriticalDataForSet. /// Safe, because sets SecurityCriticalDataForSet to IntPtr.Zero, which is safe. /// [SecurityCritical, SecurityTreatAsSafe] private void Dispose(bool disposing) { PtsContext ptsContext = null; // Do actual dispose only once. if (Interlocked.CompareExchange(ref _disposed, 1, 0) == 0) { // Dispose PTS break record. // According to following article the entire reachable graph from // a finalizable object is promoted, and it is safe to access its // members if they do not have their own finalizers. // Hence it is OK to access _ptsContext during finalization. // See: http://blogs.msdn.com/[....]/archive/2004/02/20/77460.aspx ptsContext = _ptsContext.Target as PtsContext; if (ptsContext != null && !ptsContext.Disposed) { // Notify PtsContext that BreakRecord is not used anymore, so all // associated resources can by destroyed. ptsContext.OnPageBreakRecordDisposed(_br, disposing); } // Cleanup the state. _br.Value = IntPtr.Zero; _ptsContext = null; } } #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields ////// PTS page break record. /// private SecurityCriticalDataForSet_br; /// /// Page number of the page starting at the break position. /// private readonly int _pageNumber; ////// In the finalizer we may need to reference an instance of PtsContext /// to do the right cleanup. For this reason store a WeakReference /// to it. /// private WeakReference _ptsContext; ////// Whether object is already disposed. /// private int _disposed; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: PageBreakRecord.cs // // Description: Break records for PTS pages. // //--------------------------------------------------------------------------- using System; // IntPtr, IDisposable, ... using System.Threading; // Interlocked using System.Security; // SecurityCritical using MS.Internal.PtsHost.UnsafeNativeMethods; // PTS namespace MS.Internal.PtsHost { ////// PageBreakRecord is used to represent a break record for top level PTS /// page. Points to break record structure of PTS page. /// internal sealed class PageBreakRecord : IDisposable { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// Current PTS Context. /// PTS page break record. /// Page number. internal PageBreakRecord(PtsContext ptsContext, SecurityCriticalDataForSetbr, int pageNumber) { Invariant.Assert(ptsContext != null, "Invalid PtsContext object."); Invariant.Assert(br.Value != IntPtr.Zero, "Invalid break record object."); _br = br; _pageNumber = pageNumber; // In the finalizer we may need to reference an object instance // to do the right cleanup. For this reason store a WeakReference // to such object. _ptsContext = new WeakReference(ptsContext); // BreakRecord contains unmanaged resources that need to be released when // BreakRecord is destroyed or Dispatcher is closing. For this reason keep // track of this BreakRecord in PtsContext. ptsContext.OnPageBreakRecordCreated(_br); } /// /// Finalizer - releases unmanaged resources. /// ~PageBreakRecord() { Dispose(false); } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Dispose allocated resources. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } #endregion Public Methods //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- #region Internal Properties ////// PTS page break record. /// internal IntPtr BreakRecord { get { return _br.Value; } } ////// Page number of the page starting at the break position. /// internal int PageNumber { get { return _pageNumber; } } #endregion Internal Properties //------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------- #region Private Methods ////// Destroys all unmanaged resources. /// /// Whether dispose is caused by explicit call to Dispose. ////// Finalizer needs to follow rules below: /// a) Your Finalize method must tolerate partially constructed instances. /// b) Your Finalize method must consider the consequence of failure. /// c) Your object is callable after Finalization. /// d) Your object is callable during Finalization. /// e) Your Finalizer could be called multiple times. /// f) Your Finalizer runs in a delicate security context. /// See: http://blogs.msdn.com/[....]/archive/2004/02/20/77460.aspx /// ////// Critical, because sets SecurityCriticalDataForSet. /// Safe, because sets SecurityCriticalDataForSet to IntPtr.Zero, which is safe. /// [SecurityCritical, SecurityTreatAsSafe] private void Dispose(bool disposing) { PtsContext ptsContext = null; // Do actual dispose only once. if (Interlocked.CompareExchange(ref _disposed, 1, 0) == 0) { // Dispose PTS break record. // According to following article the entire reachable graph from // a finalizable object is promoted, and it is safe to access its // members if they do not have their own finalizers. // Hence it is OK to access _ptsContext during finalization. // See: http://blogs.msdn.com/[....]/archive/2004/02/20/77460.aspx ptsContext = _ptsContext.Target as PtsContext; if (ptsContext != null && !ptsContext.Disposed) { // Notify PtsContext that BreakRecord is not used anymore, so all // associated resources can by destroyed. ptsContext.OnPageBreakRecordDisposed(_br, disposing); } // Cleanup the state. _br.Value = IntPtr.Zero; _ptsContext = null; } } #endregion Private Methods //------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields ////// PTS page break record. /// private SecurityCriticalDataForSet_br; /// /// Page number of the page starting at the break position. /// private readonly int _pageNumber; ////// In the finalizer we may need to reference an instance of PtsContext /// to do the right cleanup. For this reason store a WeakReference /// to it. /// private WeakReference _ptsContext; ////// Whether object is already disposed. /// private int _disposed; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
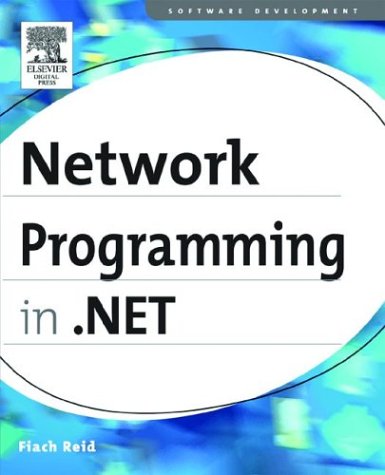
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UndirectedGraph.cs
- DesignerActionUI.cs
- CurrentTimeZone.cs
- EnvironmentPermission.cs
- ObjectDataSourceView.cs
- HyperLinkField.cs
- DataGridAddNewRow.cs
- Selection.cs
- GregorianCalendar.cs
- ListViewCommandEventArgs.cs
- ValidatingPropertiesEventArgs.cs
- GeometryConverter.cs
- ArrayListCollectionBase.cs
- COM2FontConverter.cs
- AsyncPostBackTrigger.cs
- SID.cs
- PassportIdentity.cs
- Set.cs
- ProgressBarRenderer.cs
- DataSourceHelper.cs
- SQLBoolean.cs
- DrawingBrush.cs
- PointAnimationClockResource.cs
- PropertyMapper.cs
- TextEvent.cs
- ObjectSet.cs
- TCPListener.cs
- _FtpDataStream.cs
- ElapsedEventArgs.cs
- SiteMapDataSourceDesigner.cs
- ValidationSummary.cs
- EditorZone.cs
- SettingsSavedEventArgs.cs
- TrackingMemoryStreamFactory.cs
- MimeParameters.cs
- GacUtil.cs
- CurrencyWrapper.cs
- LinkConverter.cs
- FacetChecker.cs
- PolyBezierSegment.cs
- WindowHideOrCloseTracker.cs
- UIPropertyMetadata.cs
- DynamicUpdateCommand.cs
- UnsafeNativeMethods.cs
- Control.cs
- ResourceWriter.cs
- FontNameEditor.cs
- VSWCFServiceContractGenerator.cs
- TimelineGroup.cs
- FileLoadException.cs
- DesignerSerializerAttribute.cs
- ObjectHandle.cs
- EndpointDesigner.cs
- HashHelper.cs
- EnumerableCollectionView.cs
- OrderedDictionary.cs
- CodeObject.cs
- CompilerGlobalScopeAttribute.cs
- AuthorizationRule.cs
- EdmToObjectNamespaceMap.cs
- embossbitmapeffect.cs
- Padding.cs
- DataSourceControl.cs
- DataGridItemEventArgs.cs
- CodeDomDecompiler.cs
- SimpleLine.cs
- TableStyle.cs
- GraphicsContext.cs
- XmlWrappingReader.cs
- Timeline.cs
- ObjectQueryState.cs
- BuildManagerHost.cs
- sqlmetadatafactory.cs
- TemplatePartAttribute.cs
- ProgressBar.cs
- PathGeometry.cs
- RoleProviderPrincipal.cs
- PathSegment.cs
- OleCmdHelper.cs
- QueueProcessor.cs
- Mapping.cs
- Peer.cs
- XPathException.cs
- TextEndOfParagraph.cs
- NativeMsmqMessage.cs
- SpanIndex.cs
- PersonalizablePropertyEntry.cs
- TableSectionStyle.cs
- ColorDialog.cs
- Renderer.cs
- Ticks.cs
- RadioButtonBaseAdapter.cs
- FilteredDataSetHelper.cs
- ListenerConnectionDemuxer.cs
- Encoder.cs
- CompiledQuery.cs
- DirectoryRootQuery.cs
- GeneralTransform3D.cs
- DataServiceRequest.cs
- ServicePointManager.cs