Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / PtsHost / Section.cs / 1 / Section.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: Section.cs // // Description: Section is representing a portion of a document in which // certain page formatting properties can be changed, such as line numbering, // number of columns, headers and footers. // // History: // 05/05/2003 : grzegorz - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Security; using System.Windows.Documents; using System.Windows.Media; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// Section is representing a portion of a document in which certain page /// formatting properties can be changed, such as line numbering, /// number of columns, headers and footers. /// internal sealed class Section : UnmanagedHandle { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Constructor /// /// /// Content's structural cache /// internal Section(StructuralCache structuralCache) : base(structuralCache.PtsContext) { _structuralCache = structuralCache; } ////// Dispose unmanaged resources. /// public override void Dispose() { DestroyStructure(); base.Dispose(); } #endregion Constructors // ------------------------------------------------------------------ // // PTS callbacks // // ----------------------------------------------------------------- #region PTS callbacks ////// Indicates whether to skip a page /// /// /// OUT: skip page due to odd/even page issue /// internal void FSkipPage( out int fSkip) { // Never skip a page fSkip = PTS.False; } // ------------------------------------------------------------------ // GetPageDimensions // ------------------------------------------------------------------ ////// Get page dimensions /// /// /// OUT: direction of main text /// /// /// OUT: header/footer position on the page /// /// /// OUT: page width /// /// /// OUT: page height /// /// /// OUT: rectangle within page margins /// internal void GetPageDimensions( out uint fswdir, out int fHeaderFooterAtTopBottom, out int durPage, out int dvrPage, ref PTS.FSRECT fsrcMargin) { // Set page dimentions Size pageSize = _structuralCache.CurrentFormatContext.PageSize; durPage = TextDpi.ToTextDpi(pageSize.Width); dvrPage = TextDpi.ToTextDpi(pageSize.Height); // Set page margin Thickness pageMargin = _structuralCache.CurrentFormatContext.PageMargin; TextDpi.EnsureValidPageMargin(ref pageMargin, pageSize); fsrcMargin.u = TextDpi.ToTextDpi(pageMargin.Left); fsrcMargin.v = TextDpi.ToTextDpi(pageMargin.Top); fsrcMargin.du = durPage - TextDpi.ToTextDpi(pageMargin.Left + pageMargin.Right); fsrcMargin.dv = dvrPage - TextDpi.ToTextDpi(pageMargin.Top + pageMargin.Bottom); StructuralCache.PageFlowDirection = (FlowDirection)_structuralCache.PropertyOwner.GetValue(FrameworkElement.FlowDirectionProperty); fswdir = PTS.FlowDirectionToFswdir(StructuralCache.PageFlowDirection); // fHeaderFooterAtTopBottom = PTS.False; } ////// Get justification properties /// /// /// IN: array of the section names on the page /// /// /// IN: number of sections on the page /// /// /// IN: is last section on the page broken? /// /// /// OUT: apply justification/alignment to the page? /// /// /// OUT: kind of vertical alignment for the page /// /// /// OUT: cancel justification for the last column of the page? /// ////// Critical, because it is unsafe method. /// [SecurityCritical] internal unsafe void GetJustificationProperties( IntPtr* rgnms, int cnms, int fLastSectionNotBroken, out int fJustify, out PTS.FSKALIGNPAGE fskal, out int fCancelAtLastColumn) { // NOTE: use the first section to report values (array is only for word compat). fJustify = PTS.False; fCancelAtLastColumn = PTS.False; fskal = PTS.FSKALIGNPAGE.fskalpgTop; } ////// Get next section /// /// /// OUT: next section exists /// /// /// OUT: name of the next section /// internal void GetNextSection( out int fSuccess, out IntPtr nmsNext) { fSuccess = PTS.False; nmsNext = IntPtr.Zero; } // ----------------------------------------------------------------- // GetSectionProperties // ------------------------------------------------------------------ ////// Get section properties /// /// /// OUT: stop page before this section? /// /// /// OUT: direction of this section /// /// /// OUT: apply column balancing to this section? /// /// /// OUT: number of columns in the main text segment /// /// /// OUT: number of segment-defined columnspan areas /// /// /// OUT: number of height-defined columnsapn areas /// internal void GetSectionProperties( out int fNewPage, out uint fswdir, out int fApplyColumnBalancing, out int ccol, out int cSegmentDefinedColumnSpanAreas, out int cHeightDefinedColumnSpanAreas) { ColumnPropertiesGroup columnProperties = new ColumnPropertiesGroup(Element); Size pageSize = _structuralCache.CurrentFormatContext.PageSize; double lineHeight = DynamicPropertyReader.GetLineHeightValue(Element); Thickness pageMargin = _structuralCache.CurrentFormatContext.PageMargin; double pageFontSize = (double)_structuralCache.PropertyOwner.GetValue(Block.FontSizeProperty); FontFamily pageFontFamily = (FontFamily)_structuralCache.PropertyOwner.GetValue(Block.FontFamilyProperty); bool enableColumns = _structuralCache.CurrentFormatContext.FinitePage; fNewPage = PTS.False; // Since only one section is supported, don't force page break before. fswdir = PTS.FlowDirectionToFswdir((FlowDirection)_structuralCache.PropertyOwner.GetValue(FrameworkElement.FlowDirectionProperty)); fApplyColumnBalancing = PTS.False; ccol = PtsHelper.CalculateColumnCount(columnProperties, lineHeight, pageSize.Width - (pageMargin.Left + pageMargin.Right), pageFontSize, pageFontFamily, enableColumns); cSegmentDefinedColumnSpanAreas = 0; cHeightDefinedColumnSpanAreas = 0; } ////// Get main TextSegment /// /// /// OUT: name of the main text segment for this section /// internal void GetMainTextSegment( out IntPtr nmSegment) { if (_mainTextSegment == null) { // Create the main text segment _mainTextSegment = new ContainerParagraph(Element, _structuralCache); } nmSegment = _mainTextSegment.Handle; } ////// Get header segment /// /// /// IN: ptr to page break record of main page /// /// /// IN: direction for dvrMaxHeight/dvrFromEdge /// /// /// OUT: is there header on this page? /// /// /// OUT: does margin increase with header? /// /// /// OUT: maximum size of header /// /// /// OUT: distance from top edge of the paper /// /// /// OUT: direction for header /// /// /// OUT: name of header segment /// internal void GetHeaderSegment( IntPtr pfsbrpagePrelim, uint fswdir, out int fHeaderPresent, out int fHardMargin, out int dvrMaxHeight, out int dvrFromEdge, out uint fswdirHeader, out IntPtr nmsHeader) { fHeaderPresent = PTS.False; fHardMargin = PTS.False; dvrMaxHeight = dvrFromEdge = 0; fswdirHeader = fswdir; nmsHeader = IntPtr.Zero; } ////// Get footer segment /// /// /// IN: ptr to page break record of main page /// /// /// IN: direction for dvrMaxHeight/dvrFromEdge /// /// /// OUT: is there footer on this page? /// /// /// OUT: does margin increase with footer? /// /// /// OUT: maximum size of footer /// /// /// OUT: distance from bottom edge of the paper /// /// /// OUT: direction for footer /// /// /// OUT: name of footer segment /// internal void GetFooterSegment( IntPtr pfsbrpagePrelim, uint fswdir, out int fFooterPresent, out int fHardMargin, out int dvrMaxHeight, out int dvrFromEdge, out uint fswdirFooter, out IntPtr nmsFooter) { fFooterPresent = PTS.False; fHardMargin = PTS.False; dvrMaxHeight = dvrFromEdge = 0; fswdirFooter = fswdir; nmsFooter = IntPtr.Zero; } ////// Get section column info /// /// /// IN: direction of section /// /// /// IN: size of the preallocated fscolinfo array /// /// /// OUT: array of the colinfo structures /// /// /// OUT: actual number of the columns in the segment /// ////// Critical, because: /// a) calls Critical function GetColumnsInfo, /// b) it is unsafe method. /// [SecurityCritical] internal unsafe void GetSectionColumnInfo( uint fswdir, int ncol, PTS.FSCOLUMNINFO* pfscolinfo, out int ccol) { ColumnPropertiesGroup columnProperties = new ColumnPropertiesGroup(Element); Size pageSize = _structuralCache.CurrentFormatContext.PageSize; double lineHeight = DynamicPropertyReader.GetLineHeightValue(Element); Thickness pageMargin = _structuralCache.CurrentFormatContext.PageMargin; double pageFontSize = (double)_structuralCache.PropertyOwner.GetValue(Block.FontSizeProperty); FontFamily pageFontFamily = (FontFamily)_structuralCache.PropertyOwner.GetValue(Block.FontFamilyProperty); bool enableColumns = _structuralCache.CurrentFormatContext.FinitePage; ccol = ncol; PtsHelper.GetColumnsInfo(columnProperties, lineHeight, pageSize.Width - (pageMargin.Left + pageMargin.Right), pageFontSize, pageFontFamily, ncol, pfscolinfo, enableColumns); } ////// Get end note segment /// /// /// OUT: are there endnotes for this segment? /// /// /// OUT: name of endnote segment /// internal void GetEndnoteSegment( out int fEndnotesPresent, out IntPtr nmsEndnotes) { fEndnotesPresent = PTS.False; nmsEndnotes = IntPtr.Zero; } ////// Get end note separators /// /// /// OUT: name of the endnote separator segment /// /// /// OUT: name of endnote cont separator segment /// /// /// OUT: name of the endnote cont notice segment /// internal void GetEndnoteSeparators( out IntPtr nmsEndnoteSeparator, out IntPtr nmsEndnoteContSeparator, out IntPtr nmsEndnoteContNotice) { nmsEndnoteSeparator = IntPtr.Zero; nmsEndnoteContSeparator = IntPtr.Zero; nmsEndnoteContNotice = IntPtr.Zero; } #endregion PTS callbacks // ----------------------------------------------------------------- // // Internal Methods // // ----------------------------------------------------------------- #region Internal Methods ////// Invalidate format caches accumulated in the section. /// internal void InvalidateFormatCache() { if (_mainTextSegment != null) { _mainTextSegment.InvalidateFormatCache(); } } ////// Clear previously accumulated update info. /// internal void ClearUpdateInfo() { if (_mainTextSegment != null) { _mainTextSegment.ClearUpdateInfo(); } } ////// Invalidate content's structural cache. /// internal void InvalidateStructure() { if (_mainTextSegment != null) { DtrList dtrs = _structuralCache.DtrList; if (dtrs != null) { _mainTextSegment.InvalidateStructure(dtrs[0].StartIndex); } } } ////// Destroy content's structural cache. /// internal void DestroyStructure() { if (_mainTextSegment != null) { _mainTextSegment.Dispose(); _mainTextSegment = null; } } ////// Update number of characters consumed by the main text segment. /// internal void UpdateSegmentLastFormatPositions() { if(_mainTextSegment != null) { _mainTextSegment.UpdateLastFormatPositions(); } } ////// Can update section? /// internal bool CanUpdate { get { return _mainTextSegment != null; } } ////// StructuralCache. /// internal StructuralCache StructuralCache { get { return _structuralCache; } } ////// Element owner. /// internal DependencyObject Element { get { return _structuralCache.PropertyOwner; } } #endregion Internal Methods //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields ////// Main text segment. /// private BaseParagraph _mainTextSegment; ////// Structural cache. /// private readonly StructuralCache _structuralCache; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: Section.cs // // Description: Section is representing a portion of a document in which // certain page formatting properties can be changed, such as line numbering, // number of columns, headers and footers. // // History: // 05/05/2003 : grzegorz - moving from Avalon branch. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Security; using System.Windows.Documents; using System.Windows.Media; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// Section is representing a portion of a document in which certain page /// formatting properties can be changed, such as line numbering, /// number of columns, headers and footers. /// internal sealed class Section : UnmanagedHandle { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Constructor /// /// /// Content's structural cache /// internal Section(StructuralCache structuralCache) : base(structuralCache.PtsContext) { _structuralCache = structuralCache; } ////// Dispose unmanaged resources. /// public override void Dispose() { DestroyStructure(); base.Dispose(); } #endregion Constructors // ------------------------------------------------------------------ // // PTS callbacks // // ----------------------------------------------------------------- #region PTS callbacks ////// Indicates whether to skip a page /// /// /// OUT: skip page due to odd/even page issue /// internal void FSkipPage( out int fSkip) { // Never skip a page fSkip = PTS.False; } // ------------------------------------------------------------------ // GetPageDimensions // ------------------------------------------------------------------ ////// Get page dimensions /// /// /// OUT: direction of main text /// /// /// OUT: header/footer position on the page /// /// /// OUT: page width /// /// /// OUT: page height /// /// /// OUT: rectangle within page margins /// internal void GetPageDimensions( out uint fswdir, out int fHeaderFooterAtTopBottom, out int durPage, out int dvrPage, ref PTS.FSRECT fsrcMargin) { // Set page dimentions Size pageSize = _structuralCache.CurrentFormatContext.PageSize; durPage = TextDpi.ToTextDpi(pageSize.Width); dvrPage = TextDpi.ToTextDpi(pageSize.Height); // Set page margin Thickness pageMargin = _structuralCache.CurrentFormatContext.PageMargin; TextDpi.EnsureValidPageMargin(ref pageMargin, pageSize); fsrcMargin.u = TextDpi.ToTextDpi(pageMargin.Left); fsrcMargin.v = TextDpi.ToTextDpi(pageMargin.Top); fsrcMargin.du = durPage - TextDpi.ToTextDpi(pageMargin.Left + pageMargin.Right); fsrcMargin.dv = dvrPage - TextDpi.ToTextDpi(pageMargin.Top + pageMargin.Bottom); StructuralCache.PageFlowDirection = (FlowDirection)_structuralCache.PropertyOwner.GetValue(FrameworkElement.FlowDirectionProperty); fswdir = PTS.FlowDirectionToFswdir(StructuralCache.PageFlowDirection); // fHeaderFooterAtTopBottom = PTS.False; } ////// Get justification properties /// /// /// IN: array of the section names on the page /// /// /// IN: number of sections on the page /// /// /// IN: is last section on the page broken? /// /// /// OUT: apply justification/alignment to the page? /// /// /// OUT: kind of vertical alignment for the page /// /// /// OUT: cancel justification for the last column of the page? /// ////// Critical, because it is unsafe method. /// [SecurityCritical] internal unsafe void GetJustificationProperties( IntPtr* rgnms, int cnms, int fLastSectionNotBroken, out int fJustify, out PTS.FSKALIGNPAGE fskal, out int fCancelAtLastColumn) { // NOTE: use the first section to report values (array is only for word compat). fJustify = PTS.False; fCancelAtLastColumn = PTS.False; fskal = PTS.FSKALIGNPAGE.fskalpgTop; } ////// Get next section /// /// /// OUT: next section exists /// /// /// OUT: name of the next section /// internal void GetNextSection( out int fSuccess, out IntPtr nmsNext) { fSuccess = PTS.False; nmsNext = IntPtr.Zero; } // ----------------------------------------------------------------- // GetSectionProperties // ------------------------------------------------------------------ ////// Get section properties /// /// /// OUT: stop page before this section? /// /// /// OUT: direction of this section /// /// /// OUT: apply column balancing to this section? /// /// /// OUT: number of columns in the main text segment /// /// /// OUT: number of segment-defined columnspan areas /// /// /// OUT: number of height-defined columnsapn areas /// internal void GetSectionProperties( out int fNewPage, out uint fswdir, out int fApplyColumnBalancing, out int ccol, out int cSegmentDefinedColumnSpanAreas, out int cHeightDefinedColumnSpanAreas) { ColumnPropertiesGroup columnProperties = new ColumnPropertiesGroup(Element); Size pageSize = _structuralCache.CurrentFormatContext.PageSize; double lineHeight = DynamicPropertyReader.GetLineHeightValue(Element); Thickness pageMargin = _structuralCache.CurrentFormatContext.PageMargin; double pageFontSize = (double)_structuralCache.PropertyOwner.GetValue(Block.FontSizeProperty); FontFamily pageFontFamily = (FontFamily)_structuralCache.PropertyOwner.GetValue(Block.FontFamilyProperty); bool enableColumns = _structuralCache.CurrentFormatContext.FinitePage; fNewPage = PTS.False; // Since only one section is supported, don't force page break before. fswdir = PTS.FlowDirectionToFswdir((FlowDirection)_structuralCache.PropertyOwner.GetValue(FrameworkElement.FlowDirectionProperty)); fApplyColumnBalancing = PTS.False; ccol = PtsHelper.CalculateColumnCount(columnProperties, lineHeight, pageSize.Width - (pageMargin.Left + pageMargin.Right), pageFontSize, pageFontFamily, enableColumns); cSegmentDefinedColumnSpanAreas = 0; cHeightDefinedColumnSpanAreas = 0; } ////// Get main TextSegment /// /// /// OUT: name of the main text segment for this section /// internal void GetMainTextSegment( out IntPtr nmSegment) { if (_mainTextSegment == null) { // Create the main text segment _mainTextSegment = new ContainerParagraph(Element, _structuralCache); } nmSegment = _mainTextSegment.Handle; } ////// Get header segment /// /// /// IN: ptr to page break record of main page /// /// /// IN: direction for dvrMaxHeight/dvrFromEdge /// /// /// OUT: is there header on this page? /// /// /// OUT: does margin increase with header? /// /// /// OUT: maximum size of header /// /// /// OUT: distance from top edge of the paper /// /// /// OUT: direction for header /// /// /// OUT: name of header segment /// internal void GetHeaderSegment( IntPtr pfsbrpagePrelim, uint fswdir, out int fHeaderPresent, out int fHardMargin, out int dvrMaxHeight, out int dvrFromEdge, out uint fswdirHeader, out IntPtr nmsHeader) { fHeaderPresent = PTS.False; fHardMargin = PTS.False; dvrMaxHeight = dvrFromEdge = 0; fswdirHeader = fswdir; nmsHeader = IntPtr.Zero; } ////// Get footer segment /// /// /// IN: ptr to page break record of main page /// /// /// IN: direction for dvrMaxHeight/dvrFromEdge /// /// /// OUT: is there footer on this page? /// /// /// OUT: does margin increase with footer? /// /// /// OUT: maximum size of footer /// /// /// OUT: distance from bottom edge of the paper /// /// /// OUT: direction for footer /// /// /// OUT: name of footer segment /// internal void GetFooterSegment( IntPtr pfsbrpagePrelim, uint fswdir, out int fFooterPresent, out int fHardMargin, out int dvrMaxHeight, out int dvrFromEdge, out uint fswdirFooter, out IntPtr nmsFooter) { fFooterPresent = PTS.False; fHardMargin = PTS.False; dvrMaxHeight = dvrFromEdge = 0; fswdirFooter = fswdir; nmsFooter = IntPtr.Zero; } ////// Get section column info /// /// /// IN: direction of section /// /// /// IN: size of the preallocated fscolinfo array /// /// /// OUT: array of the colinfo structures /// /// /// OUT: actual number of the columns in the segment /// ////// Critical, because: /// a) calls Critical function GetColumnsInfo, /// b) it is unsafe method. /// [SecurityCritical] internal unsafe void GetSectionColumnInfo( uint fswdir, int ncol, PTS.FSCOLUMNINFO* pfscolinfo, out int ccol) { ColumnPropertiesGroup columnProperties = new ColumnPropertiesGroup(Element); Size pageSize = _structuralCache.CurrentFormatContext.PageSize; double lineHeight = DynamicPropertyReader.GetLineHeightValue(Element); Thickness pageMargin = _structuralCache.CurrentFormatContext.PageMargin; double pageFontSize = (double)_structuralCache.PropertyOwner.GetValue(Block.FontSizeProperty); FontFamily pageFontFamily = (FontFamily)_structuralCache.PropertyOwner.GetValue(Block.FontFamilyProperty); bool enableColumns = _structuralCache.CurrentFormatContext.FinitePage; ccol = ncol; PtsHelper.GetColumnsInfo(columnProperties, lineHeight, pageSize.Width - (pageMargin.Left + pageMargin.Right), pageFontSize, pageFontFamily, ncol, pfscolinfo, enableColumns); } ////// Get end note segment /// /// /// OUT: are there endnotes for this segment? /// /// /// OUT: name of endnote segment /// internal void GetEndnoteSegment( out int fEndnotesPresent, out IntPtr nmsEndnotes) { fEndnotesPresent = PTS.False; nmsEndnotes = IntPtr.Zero; } ////// Get end note separators /// /// /// OUT: name of the endnote separator segment /// /// /// OUT: name of endnote cont separator segment /// /// /// OUT: name of the endnote cont notice segment /// internal void GetEndnoteSeparators( out IntPtr nmsEndnoteSeparator, out IntPtr nmsEndnoteContSeparator, out IntPtr nmsEndnoteContNotice) { nmsEndnoteSeparator = IntPtr.Zero; nmsEndnoteContSeparator = IntPtr.Zero; nmsEndnoteContNotice = IntPtr.Zero; } #endregion PTS callbacks // ----------------------------------------------------------------- // // Internal Methods // // ----------------------------------------------------------------- #region Internal Methods ////// Invalidate format caches accumulated in the section. /// internal void InvalidateFormatCache() { if (_mainTextSegment != null) { _mainTextSegment.InvalidateFormatCache(); } } ////// Clear previously accumulated update info. /// internal void ClearUpdateInfo() { if (_mainTextSegment != null) { _mainTextSegment.ClearUpdateInfo(); } } ////// Invalidate content's structural cache. /// internal void InvalidateStructure() { if (_mainTextSegment != null) { DtrList dtrs = _structuralCache.DtrList; if (dtrs != null) { _mainTextSegment.InvalidateStructure(dtrs[0].StartIndex); } } } ////// Destroy content's structural cache. /// internal void DestroyStructure() { if (_mainTextSegment != null) { _mainTextSegment.Dispose(); _mainTextSegment = null; } } ////// Update number of characters consumed by the main text segment. /// internal void UpdateSegmentLastFormatPositions() { if(_mainTextSegment != null) { _mainTextSegment.UpdateLastFormatPositions(); } } ////// Can update section? /// internal bool CanUpdate { get { return _mainTextSegment != null; } } ////// StructuralCache. /// internal StructuralCache StructuralCache { get { return _structuralCache; } } ////// Element owner. /// internal DependencyObject Element { get { return _structuralCache.PropertyOwner; } } #endregion Internal Methods //------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------- #region Private Fields ////// Main text segment. /// private BaseParagraph _mainTextSegment; ////// Structural cache. /// private readonly StructuralCache _structuralCache; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
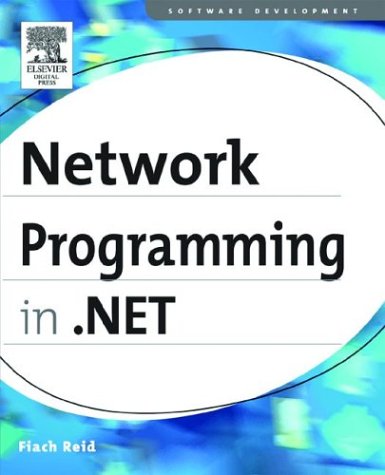
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MaskInputRejectedEventArgs.cs
- SqlCacheDependencyDatabaseCollection.cs
- PolicyLevel.cs
- JavaScriptObjectDeserializer.cs
- ObjectIDGenerator.cs
- UriTemplateLiteralQueryValue.cs
- WpfWebRequestHelper.cs
- WebPartDeleteVerb.cs
- SectionVisual.cs
- BitStream.cs
- Rect3D.cs
- TemplateBindingExtensionConverter.cs
- StateMachine.cs
- SqlTriggerContext.cs
- ChannelSinkStacks.cs
- ValueOfAction.cs
- BitStack.cs
- PageHandlerFactory.cs
- KnownBoxes.cs
- ZoneButton.cs
- WebPartConnectionsCloseVerb.cs
- CfgRule.cs
- View.cs
- GPRECTF.cs
- PageAction.cs
- EnumValidator.cs
- SystemPens.cs
- SafeViewOfFileHandle.cs
- StreamGeometry.cs
- ChangesetResponse.cs
- AmbientProperties.cs
- CanonicalXml.cs
- SmtpFailedRecipientException.cs
- ListViewItemSelectionChangedEvent.cs
- AppDomainAttributes.cs
- ProviderSettings.cs
- MasterPageCodeDomTreeGenerator.cs
- TypeUsage.cs
- ConfigXmlCDataSection.cs
- RoutedUICommand.cs
- TransactionManager.cs
- RowToParametersTransformer.cs
- RtfFormatStack.cs
- XmlNodeReader.cs
- XmlCollation.cs
- SingletonConnectionReader.cs
- CodeRegionDirective.cs
- CFStream.cs
- EventManager.cs
- DateTimeConverter.cs
- CollectionViewSource.cs
- SchemaImporterExtension.cs
- PrintDialogDesigner.cs
- Events.cs
- DriveNotFoundException.cs
- JoinSymbol.cs
- DecoderReplacementFallback.cs
- TouchEventArgs.cs
- IndexedEnumerable.cs
- TypeConstant.cs
- JulianCalendar.cs
- WorkItem.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- DuplicateWaitObjectException.cs
- XmlImplementation.cs
- Socket.cs
- ContainerUIElement3D.cs
- TextEditorParagraphs.cs
- TimeSpan.cs
- FormsAuthenticationUser.cs
- WebPartConnectionsCancelEventArgs.cs
- LexicalChunk.cs
- TextAdaptor.cs
- NameNode.cs
- Message.cs
- WebControlParameterProxy.cs
- Padding.cs
- HttpCapabilitiesSectionHandler.cs
- MemoryMappedView.cs
- DataGridViewTopLeftHeaderCell.cs
- _ConnectOverlappedAsyncResult.cs
- SubMenuStyleCollectionEditor.cs
- SecurityContext.cs
- HttpListenerException.cs
- shaperfactoryquerycacheentry.cs
- MasterPage.cs
- ZipIOExtraField.cs
- ModuleElement.cs
- MdImport.cs
- DesignerLinkAdapter.cs
- AuthenticationService.cs
- ObsoleteAttribute.cs
- PageBuildProvider.cs
- CounterCreationDataConverter.cs
- MemberAccessException.cs
- UnaryNode.cs
- LocatorManager.cs
- WebBrowserHelper.cs
- Int32AnimationBase.cs
- SecurityValidationBehavior.cs