Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Shapes / ellipse.cs / 1 / ellipse.cs
//---------------------------------------------------------------------------- // File: Ellipse.cs // // Description: // Implementation of Ellipse shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System.ComponentModel; using System; namespace System.Windows.Shapes { ////// The ellipse shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// ///public sealed class Ellipse : Shape { #region Constructors /// /// Instantiates a new instance of a Ellipse. /// ///public Ellipse() { } // The default stretch mode of Ellipse is Fill static Ellipse() { StretchProperty.OverrideMetadata(typeof(Ellipse), new FrameworkPropertyMetadata(Stretch.Fill)); } #endregion Constructors #region Dynamic Properties // For an Ellipse, RenderedGeometry = defining geometry and GeometryTransform = Identity /// /// The RenderedGeometry property returns the final rendered geometry /// public override Geometry RenderedGeometry { get { // RenderedGeometry = defining geometry return DefiningGeometry; } } ////// Return the transformation applied to the geometry before rendering /// public override Transform GeometryTransform { get { return Transform.Identity; } } #endregion Dynamic Properties #region Protected ////// Updates DesiredSize of the Ellipse. Called by parent UIElement. This is the first pass of layout. /// /// Constraint size is an "upper limit" that Ellipse should not exceed. ///Ellipse's desired size. protected override Size MeasureOverride(Size constraint) { if (Stretch == Stretch.UniformToFill) { double width = constraint.Width; double height = constraint.Height; if (Double.IsInfinity(width) && Double.IsInfinity(height)) { return GetNaturalSize(); } else if (Double.IsInfinity(width) || Double.IsInfinity(height)) { width = Math.Min(width, height); } else { width = Math.Max(width, height); } return new Size(width, width); } return GetNaturalSize(); } ////// Returns the final size of the shape and caches the bounds. /// protected override Size ArrangeOverride(Size finalSize) { // We construct the rectangle to fit finalSize with the appropriate Stretch mode. The rendering // transformation will thus be the identity. double penThickness = GetStrokeThickness(); double margin = penThickness / 2; _rect = new Rect( margin, // X margin, // Y Math.Max(0, finalSize.Width - penThickness), // Width Math.Max(0, finalSize.Height - penThickness)); // Height switch (Stretch) { case Stretch.None: // A 0 Rect.Width and Rect.Height rectangle _rect.Width = _rect.Height = 0; break; case Stretch.Fill: // The most common case: a rectangle that fills the box. // _rect has already been initialized for that. break; case Stretch.Uniform: // The maximal square that fits in the final box if (_rect.Width > _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width <= _rect.Height { _rect.Height = _rect.Width; } break; case Stretch.UniformToFill: // The minimal square that fills the final box if (_rect.Width < _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width >= _rect.Height { _rect.Height = _rect.Width; } break; } ResetRenderedGeometry(); return finalSize; } ////// Get the ellipse that defines this shape /// protected override Geometry DefiningGeometry { get { if (_rect.IsEmpty) { return Geometry.Empty; } return new EllipseGeometry(_rect); } } ////// Render callback. /// protected override void OnRender(DrawingContext drawingContext) { if (!_rect.IsEmpty) { Pen pen = GetPen(); drawingContext.DrawGeometry(Fill, pen, new EllipseGeometry(_rect)); } } #endregion Protected #region Internal Methods internal override void CacheDefiningGeometry() { double margin = GetStrokeThickness() / 2; _rect = new Rect(margin, margin, 0, 0); } ////// Get the natural size of the geometry that defines this shape /// internal override Size GetNaturalSize() { double strokeThickness = GetStrokeThickness(); return new Size(strokeThickness, strokeThickness); } ////// Get the bonds of the rectangle that defines this shape /// internal override Rect GetDefiningGeometryBounds() { return _rect; } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 13; } } #endregion Internal Methods #region Private Fields private Rect _rect = Rect.Empty; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // File: Ellipse.cs // // Description: // Implementation of Ellipse shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System.ComponentModel; using System; namespace System.Windows.Shapes { ////// The ellipse shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// ///public sealed class Ellipse : Shape { #region Constructors /// /// Instantiates a new instance of a Ellipse. /// ///public Ellipse() { } // The default stretch mode of Ellipse is Fill static Ellipse() { StretchProperty.OverrideMetadata(typeof(Ellipse), new FrameworkPropertyMetadata(Stretch.Fill)); } #endregion Constructors #region Dynamic Properties // For an Ellipse, RenderedGeometry = defining geometry and GeometryTransform = Identity /// /// The RenderedGeometry property returns the final rendered geometry /// public override Geometry RenderedGeometry { get { // RenderedGeometry = defining geometry return DefiningGeometry; } } ////// Return the transformation applied to the geometry before rendering /// public override Transform GeometryTransform { get { return Transform.Identity; } } #endregion Dynamic Properties #region Protected ////// Updates DesiredSize of the Ellipse. Called by parent UIElement. This is the first pass of layout. /// /// Constraint size is an "upper limit" that Ellipse should not exceed. ///Ellipse's desired size. protected override Size MeasureOverride(Size constraint) { if (Stretch == Stretch.UniformToFill) { double width = constraint.Width; double height = constraint.Height; if (Double.IsInfinity(width) && Double.IsInfinity(height)) { return GetNaturalSize(); } else if (Double.IsInfinity(width) || Double.IsInfinity(height)) { width = Math.Min(width, height); } else { width = Math.Max(width, height); } return new Size(width, width); } return GetNaturalSize(); } ////// Returns the final size of the shape and caches the bounds. /// protected override Size ArrangeOverride(Size finalSize) { // We construct the rectangle to fit finalSize with the appropriate Stretch mode. The rendering // transformation will thus be the identity. double penThickness = GetStrokeThickness(); double margin = penThickness / 2; _rect = new Rect( margin, // X margin, // Y Math.Max(0, finalSize.Width - penThickness), // Width Math.Max(0, finalSize.Height - penThickness)); // Height switch (Stretch) { case Stretch.None: // A 0 Rect.Width and Rect.Height rectangle _rect.Width = _rect.Height = 0; break; case Stretch.Fill: // The most common case: a rectangle that fills the box. // _rect has already been initialized for that. break; case Stretch.Uniform: // The maximal square that fits in the final box if (_rect.Width > _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width <= _rect.Height { _rect.Height = _rect.Width; } break; case Stretch.UniformToFill: // The minimal square that fills the final box if (_rect.Width < _rect.Height) { _rect.Width = _rect.Height; } else // _rect.Width >= _rect.Height { _rect.Height = _rect.Width; } break; } ResetRenderedGeometry(); return finalSize; } ////// Get the ellipse that defines this shape /// protected override Geometry DefiningGeometry { get { if (_rect.IsEmpty) { return Geometry.Empty; } return new EllipseGeometry(_rect); } } ////// Render callback. /// protected override void OnRender(DrawingContext drawingContext) { if (!_rect.IsEmpty) { Pen pen = GetPen(); drawingContext.DrawGeometry(Fill, pen, new EllipseGeometry(_rect)); } } #endregion Protected #region Internal Methods internal override void CacheDefiningGeometry() { double margin = GetStrokeThickness() / 2; _rect = new Rect(margin, margin, 0, 0); } ////// Get the natural size of the geometry that defines this shape /// internal override Size GetNaturalSize() { double strokeThickness = GetStrokeThickness(); return new Size(strokeThickness, strokeThickness); } ////// Get the bonds of the rectangle that defines this shape /// internal override Rect GetDefiningGeometryBounds() { return _rect; } // // This property // 1. Finds the correct initial size for the _effectiveValues store on the current DependencyObject // 2. This is a performance optimization // internal override int EffectiveValuesInitialSize { get { return 13; } } #endregion Internal Methods #region Private Fields private Rect _rect = Rect.Empty; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
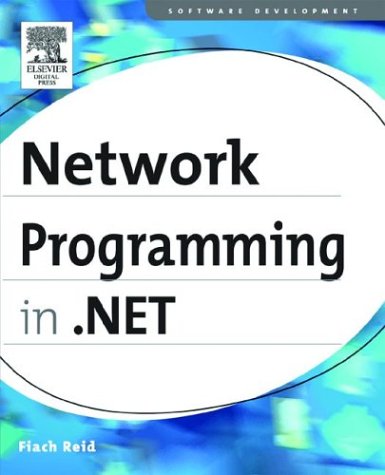
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BufferedGraphics.cs
- ResourcesChangeInfo.cs
- ExceptQueryOperator.cs
- EdgeProfileValidation.cs
- DriveNotFoundException.cs
- RecordConverter.cs
- HtmlElementErrorEventArgs.cs
- TiffBitmapDecoder.cs
- COM2IManagedPerPropertyBrowsingHandler.cs
- DropSourceBehavior.cs
- HtmlInputFile.cs
- LabelDesigner.cs
- SystemBrushes.cs
- SerializationSectionGroup.cs
- AttributeCollection.cs
- MemberPath.cs
- TagMapCollection.cs
- StorageConditionPropertyMapping.cs
- ParseChildrenAsPropertiesAttribute.cs
- Error.cs
- AuthenticatingEventArgs.cs
- SpellCheck.cs
- DiffuseMaterial.cs
- DesignerView.Commands.cs
- NavigationService.cs
- DataGridDetailsPresenterAutomationPeer.cs
- ListenerHandler.cs
- OSFeature.cs
- DataGridRowsPresenter.cs
- HyperLink.cs
- SystemResourceHost.cs
- XmlArrayAttribute.cs
- XmlWellformedWriter.cs
- QualifiedCellIdBoolean.cs
- IisTraceWebEventProvider.cs
- BamlLocalizableResourceKey.cs
- SchemaImporterExtensionElement.cs
- DrawingServices.cs
- ReferenceConverter.cs
- ConfigurationElement.cs
- ClientTargetCollection.cs
- ProcessThreadCollection.cs
- ToolBarPanel.cs
- Point.cs
- ObjectDisposedException.cs
- WindowClosedEventArgs.cs
- MenuCommands.cs
- Wildcard.cs
- Int32.cs
- IPGlobalProperties.cs
- VerificationException.cs
- LambdaCompiler.Lambda.cs
- HttpValueCollection.cs
- HwndSourceKeyboardInputSite.cs
- ProcessHostServerConfig.cs
- AppDomainUnloadedException.cs
- PaginationProgressEventArgs.cs
- XmlC14NWriter.cs
- DbSetClause.cs
- Utility.cs
- RtfNavigator.cs
- EntityCommandDefinition.cs
- MonitoringDescriptionAttribute.cs
- ClickablePoint.cs
- HtmlMobileTextWriter.cs
- SpellerHighlightLayer.cs
- PersonalizablePropertyEntry.cs
- FixedSOMLineRanges.cs
- ParserContext.cs
- Blend.cs
- XmlSchemaChoice.cs
- iisPickupDirectory.cs
- TransformConverter.cs
- ConstructorNeedsTagAttribute.cs
- TempFiles.cs
- DynamicRenderer.cs
- DefaultBindingPropertyAttribute.cs
- ImportContext.cs
- ObjectContext.cs
- ObjectDataSourceView.cs
- UnhandledExceptionEventArgs.cs
- Type.cs
- CommandEventArgs.cs
- CallSiteOps.cs
- CodeTypeDeclarationCollection.cs
- WebBrowserNavigatedEventHandler.cs
- FloaterParaClient.cs
- OraclePermission.cs
- rsa.cs
- NameValueCollection.cs
- PathFigureCollection.cs
- ProbeDuplexCD1AsyncResult.cs
- KnownAssemblyEntry.cs
- Positioning.cs
- TypefaceMap.cs
- BinaryCommonClasses.cs
- JsonFormatReaderGenerator.cs
- diagnosticsswitches.cs
- StringToken.cs
- HashLookup.cs